Server-Side Rendering (SSR) has transformed web development improving SEO, faster page load times, and user experiences. In this dynamic landscape, Next.js and Nuxt.js have emerged as leading frameworks for JavaScript developers seeking to leverage SSR.
Next.js and Nuxt.js are robust, versatile frameworks, each specializing in compatibility with a specific JavaScript library—Next.js with React and Nuxt.js with Vue.js. They simplify SSR implementation, enabling developers to create high-performance web applications optimized for SEO and user satisfaction.
This article provides a comprehensive comparison of Next.js and Nuxt.js, highlighting their strengths, weaknesses, and use cases. By the end, JavaScript developers will be equipped to make informed decisions for their web development projects.
Next.js
Next.js is a powerful web framework built on top of React, one of the most popular JavaScript libraries for building user interfaces. It is designed to simplify and enhance the development of web applications by providing a comprehensive set of features.
Features of Next.js
Server-Side Rendering (SSR): Next.js excels at SSR, enabling you to generate web pages on the server and deliver them to clients. This results in faster initial page loads and improved SEO.
Automatic Code Splitting: Next.js automatically splits your JavaScript bundles, loading only the code necessary for a particular page. This feature improves performance and reduces load times.
File-Based Routing: Next.js offers a straightforward file-based routing system. When you create a file in the "pages" directory, it automatically becomes a route, simplifying route creation.
Static Site Generation (SSG): In addition to SSR, Next.js supports SSG, allowing you to generate static HTML files at build time. This is useful for content-heavy websites, blogs, and documentation.
Developer-Friendly: Next.js offers a great development experience with features like hot module replacement (HMR) for fast code updates during development.
Hybrid Applications: You can build hybrid applications that combine client-side rendering with server-side rendering or static site generation, providing flexibility to suit your project's needs.
React Ecosystem: Being built on React, Next.js seamlessly integrates with the extensive React ecosystem, allowing you to leverage existing components and libraries.
Use-Cases for Next.js
Next.js, with its close integration with React, is a versatile and popular choice for developers aiming to build high-performance web applications with the benefits of SSR and automatic code splitting.
Web Applications: Next.js is well-suited for building a wide range of web applications, including e-commerce sites, dashboards, and interactive web apps.
Blogs and Content Websites: Its SSG capabilities make it an excellent choice for blogs, documentation sites, and content-heavy platforms.
SEO-Optimized Sites: Next.js is favoured for projects that require strong SEO performance, as it provides SSR out of the box.
Nuxt.js
Nuxt.js is a powerful framework built on top of Vue.js, a popular JavaScript library for building user interfaces. It simplifies the development of web applications by providing conventions and a modular architecture.
Features of Nuxt.js
Server-Side Rendering (SSR): Nuxt.js offers robust SSR capabilities, allowing you to render Vue components on the server, which results in faster initial page loads and enhanced SEO.
Static Site Generation (SSG): In addition to SSR, Nuxt.js supports SSG, enabling you to generate static HTML files at build time. This is beneficial for content-focused websites and blogs.
File-Based Routing: Nuxt.js follows a file-based routing approach, where the directory structure and file names define routes. This convention simplifies route creation.
Automatic Code Splitting: Like Next.js, Nuxt.js automatically splits your JavaScript bundles, loading only the code needed for a specific page, leading to better performance.
Vue Devtools Integration: Nuxt.js seamlessly integrates with Vue Devtools for improved development and debugging experiences.
Use-Cases for Nuxt.js
Nuxt.js convention-based approach streamlines development processes and makes it an attractive choice for JavaScript developers looking to create high-performance web applications and content-driven websites.
Web Applications: Nuxt.js is well-suited for building various web applications, including e-commerce platforms, dashboards, and interactive web apps.
Blogs and Content Websites: Its support for SSR and SSG makes it an ideal choice for content-rich websites, blogs, and documentation sites.
Vue.js-Powered Apps: For developers who prefer Vue.js, Nuxt.js offers an excellent way to build SSR and SSG applications while adhering to Vue's conventions.
SEO-Optimized Sites: Nuxt.js, with its SSR capabilities, is a strong candidate for projects that prioritize SEO.
Installation and Setup
Setting up a basic Next.js project is straightforward and can be done in a few simple steps.
Step 1: Create a New Directory
- Open your terminal and navigate to the directory where you want to create your Next.js project. You can do this using the cd command.
cd path/to/your/directory
Step 2: Initialize a New Node.js Project
- Run the following command to initialize a new Node.js project in your chosen directory. This will create a package.json file.
npm init -y
Step 3: Install Next.js and React
- Next, you need to install Next.js and React as project dependencies. Run the following command:
npm install next react react-dom
Step 4: Create a Pages Directory
- Next.js uses a convention-based routing system. Create a pages directory in your project root. Inside this directory, you will place your page components, and Next.js will automatically generate routes based on the file names.
mkdir pages
Step 5: Create Your First Page
- Inside the page directory, create an index.js file. This will be your homepage.
touch pages/index.js
- Open the index.js file in your code editor and add a basic React component to it. For example:
// pages/index.js
import React from 'react';
const Home = () => {
return (
<div>
<h1>Hello, Next.js!</h1>
</div>
);
};
export default Home;
Step 6: Start the Development Server
- To start your Next.js development server, run the following command:
npm run dev
- This command will compile your project and start a development server. You should see output that says "Ready on http://localhost:3000." Open your web browser and navigate to http://localhost:3000 to see your Next.js app in action.
Congratulations! You have successfully set up a basic Next.js project. You can continue building your application by adding more pages and components to the pages directory and exploring the many features Next.js offers for server-side rendering, routing, and more.
Setting Up a Nuxt.js Project
Setting up a basic Nuxt.js project is straightforward, and you can get started quickly by following these steps:
Step 1: Create a New Directory
- Open your terminal and navigate to the directory where you want to create your Nuxt.js project. You can use the cd command to change directories.
cd path/to/your/directory
Step 2: Initialize a New Node.js Project
- To create a new Node.js project in your chosen directory, run the following command. This will generate a package.json file.
npm init -y
Step 3: Install Nuxt.js and Vue.js
- Next, you need to install Nuxt.js and Vue.js as project dependencies. Run the following command:
npm install nuxt vue
Step 4: Create a Nuxt.js Project
- Run the following command to create a new Nuxt.js project:
npx create-nuxt-app .
- This command will initiate the Nuxt.js project setup process interactively. It will ask you several questions about your project configuration. You can choose the default options or customize them according to your project's needs.
Step 5: Start the Development Server
- Once the project setup is complete, you can start the Nuxt.js development server by running:
npm run dev
- This command will compile your Nuxt.js project and start the development server. You will see output indicating that the server is running and accessible at a specific URL (usually http://localhost:3000).
Step 6: Access Your Nuxt.js App
- Open your web browser and navigate to the URL provided in the terminal (typically http://localhost:3000). You should see your Nuxt.js app's default landing page.
Congratulations! You have successfully set up a basic Nuxt.js project. From here, you can start building your application by adding pages, components, and exploring Nuxt.js features like server-side rendering (SSR) and static site generation (SSG) to create powerful web applications with Vue.js.
Feature Comparison
Server-Side Rendering (SSR)
Server-Side Rendering (SSR) in Next.js
Next.js has been designed with SSR as one of its core features, making it relatively easy to implement and customize.
Ease of Implementation: SSR in Next.js is straightforward to set up. Simply create a page component in the pages directory, and Next.js automatically takes care of the server-side rendering. You do not need to write server-side code explicitly.
Customization: Next.js offers a high degree of customization for SSR. You can control when and how data is fetched on the server and passed to the client. This flexibility allows you to optimize SSR for your specific application's needs.
Dynamic Importing: Next.js supports dynamic imports with getServerSideProps and getInitialProps. These functions enable you to fetch data on the server and pass it to your components for rendering.
SEO Benefits: SSR in Next.js provides strong SEO benefits out of the box, as it generates fully formed HTML on the server, which is more search-engine-friendly compared to client-rendered applications.
Server-Side Rendering (SSR) in Nuxt.js
Nuxt.js also excels in SSR, as it is built with this in mind.
Ease of Implementation: Nuxt.js simplifies SSR by providing automatic server-side rendering for Vue.js components. Like Next.js, you do not need to write server-side code explicitly for basic SSR.
Customization: Nuxt.js allows customization of SSR through its asyncData and fetch methods. These methods enable you to fetch data on the server and populate your components with server-rendered data.
Layouts and Middleware: Nuxt.js provides layout and middleware support, making it easy to apply server-side logic and templates to different parts of your application.
SEO Benefits: Nuxt.js, like Next.js, offers SEO advantages through its SSR capabilities. It generates SEO-friendly HTML content on the server, contributing to better search engine discoverability.
Static Site Generation (SSG)
Static Site Generation (SSG) in Next.js
Next.js offers robust support for static site generation, making it a powerful choice for websites that require pre-rendering of content at build time.
Static Pages: Next.js allows you to create static pages by placing React components in the pages directory. These pages are pre-rendered at build time and can be served as static HTML files.
Dynamic Routes: While Next.js is known for SSR, it also provides dynamic route capabilities for generating static pages with dynamic data. You can use the [param] syntax to create dynamic routes and generate static pages for different content.
Data Fetching: Next.js supports data fetching during the build process using the getStaticProps function. This enables you to fetch data from APIs, databases, or other sources and pre-render pages with that data.
Incremental Static Regeneration (ISR): Next.js introduces ISR, a feature that allows you to re-generate static pages on-demand without rebuilding the entire site. This is useful for frequently updated content.
SEO Benefits: SSG in Next.js provides strong SEO benefits, as it generates optimized static HTML files that are easily indexed by search engines.
Static Site Generation (SSG) in Nuxt.js
Nuxt.js also offers robust support for static site generation, making it well-suited for content-driven websites.
Static Pages: Nuxt.js allows you to create static pages using Vue.js components. These pages are pre-rendered at build time and can be served as static HTML files.
Dynamic Routes: Similar to Next.js, Nuxt.js supports dynamic routes for generating static pages with dynamic data. You can use the _slug.vue syntax for dynamic routes.
Data Fetching: Nuxt.js provides several methods for data fetching during the build process, including asyncData, fetch, and generate. These methods enable you to fetch data from APIs and databases and pre-render pages with that data.
Full Static Mode: Nuxt.js offers a full static mode, where the entire site can be generated as static files. This mode is suitable for websites with minimal dynamic content.
SEO Benefits: SSG in Nuxt.js provides strong SEO benefits, as it generates optimized static HTML content that is search engine-friendly.
Routing
Routing in Next.js
Next.js provides a straightforward and intuitive routing system.
File-Based Routing: Next.js follows a file-based routing system. Each .js file in the pages directory corresponds to a route. For example, if you create an about.js file in the pages directory, it automatically becomes accessible at /about.
Custom Routing: While file-based routing is convenient, you can also implement custom routing in Next.js. You can create complex route patterns and parameterized routes using brackets (e.g., [id].js).
Nested Routes: Next.js supports nested routes by creating subdirectories within the pages directory. For example, a file at pages/blog/post.js would correspond to the route /blog/post.
Link Component: Next.js provides a Link component for client-side navigation between pages. It automatically pre-fetches linked pages for faster navigation.
Programmatic Routing: You can perform programmatic routing using the Router object from the next/router module. This allows you to navigate to different pages in response to events or user interactions.
Routing in Nuxt.js
Nuxt.js offers a flexible and convention-based routing system that simplifies the creation of routes.
File-Based Routing: Similar to Next.js, Nuxt.js uses a file-based routing system. Vue components placed in the pages directory correspond to routes. For instance, a contact.vue component in the pages directory creates a /contact route.
Dynamic Routes: Nuxt.js supports dynamic routes using the pages directory structure and dynamic parameters within square brackets (e.g., pages/users/_id.vue for a dynamic user route).
Nested Routes: Nuxt.js supports nested routes through directories and layout components. You can create nested route structures by using layouts in the layouts directory.
Link Component: Nuxt.js provides a nuxt-link component, similar to Next.js's Link, for client-side navigation between pages. It also offers features like prefetching for faster navigation.
Middleware: Nuxt.js allows you to apply middleware functions to routes, providing a way to add custom logic before rendering a page. This is useful for tasks like authentication checks.
State Management
State Management in Next.js
Next.js itself does not include a built-in state management solution, as it primarily focuses on routing, server-side rendering, and other frontend concerns. However, Next.js can work seamlessly with various state management libraries and patterns.
React State Management: Next.js works seamlessly with React state management solutions like React's built-in useState, useReducer, and libraries such as Redux, Mobx, and Recoil. You can integrate these libraries into your Next.js application to manage client-side state.
Server-Side State: Since Next.js supports server-side rendering (SSR), you can pass server-generated state to the client using getServerSideProps or other data-fetching methods. This allows you to hydrate your components with initial server-rendered state.
API Routes: Next.js makes it easy to create API routes that can handle server-side logic and state management. You can use these routes to fetch or update data on the server, which can then be used to update client-side state.
Context API: React's Context API can be used for sharing state across components, including those within a Next.js application. This is a simple way to manage state without external libraries.
State Management in Nuxt.js
Nuxt.js offers more opinionated state management options due to its close integration with Vue.js.
VueX: Nuxt.js integrates seamlessly with VueX, which is Vue.js's official state management library. You can define stores, mutations, and actions to manage application state centrally. VueX is well-suited for medium to large-scale Vue.js applications.
Local Component State: For smaller applications or component-level state management, you can use Vue.js's built-in component state using the data and computed properties. This is handy for managing state within individual components.
NuxtServerInit: Nuxt.js provides a special action called nuxtServerInit that you can use to initialize state on the server side before rendering the application. This is useful for pre-populating state when using SSR.
Plugins: You can use Vue.js plugins to manage state or integrate external state management solutions. Plugins allow you to encapsulate and reuse state management logic across your Nuxt.js application.
Performance
General Performance
Both Next.js and Nuxt.js are designed to optimize the performance of web applications.
Server-Side Rendering (SSR): Both frameworks offer SSR capabilities, which can lead to faster initial page loads and improved SEO. SSR allows content to be pre-rendered on the server and sent to the client as fully formed HTML.
Code Splitting: Both Next.js and Nuxt.js support automatic code splitting, ensuring that only the necessary JavaScript code is loaded for each page. This reduces initial load times and improves the user experience.
Caching: Caching strategies can significantly impact performance. Both frameworks provide options for caching rendered pages, API responses, and assets to reduce server load and improve response times.
Client-Side Navigation: While SSR is important for the initial load, client-side navigation is also crucial for optimizing the user experience as users interact with your application. Both frameworks offer client-side routing for seamless navigation.
Development Experience
Learning Curve
The ease of learning and the learning curve for Next.js and Nuxt.js can be influenced by several factors.
Next.js:
Ease of Learning for React Developers: If you are already familiar with React, Next.js can be relatively easy to grasp. Next.js builds on top of React and extends its capabilities, so React developers can leverage their existing knowledge.
React Ecosystem: Next.js benefits from the extensive React ecosystem, including a vast number of resources, tutorials, and third-party libraries. This rich ecosystem can be a valuable learning resource for newcomers.
Routing Simplicity: Next.js offers a simple file-based routing system, which can be intuitive for beginners. Creating pages and routes in the pages directory follows a natural and straightforward pattern.
Documentation: Next.js has comprehensive documentation with clear examples and guides, making it accessible for learners. The documentation covers essential topics like routing, data fetching, and deployment.
Community Support: Next.js has a large and active community of developers that provides ample resources for both beginners and experienced developers.
Nuxt.js:
Ease of Learning for Vue.js Developers: If you are already familiar with Vue.js, Nuxt.js can be a natural choice. Nuxt.js follows Vue's conventions and leverages Vue.js concepts, making it accessible to Vue developers.
Vue Ecosystem: Nuxt.js benefits from the Vue.js ecosystem, which includes a supportive community and a range of Vue-specific resources and libraries. This ecosystem can be helpful for learners.
Convention-Based Approach: Nuxt.js's convention-based approach can be advantageous for beginners. By following naming conventions and directory structures, developers can quickly create routes, layouts, and components without complex configuration.
Official Guides: Nuxt.js provides official guides and documentation that cover essential concepts, making it easier for newcomers to understand how to build Vue.js applications with SSR and SSG.
Community Support:Nuxt.js has a strong and active community of developers and Vue.js enthusiasts that is passionate about Vue.js and its ecosystem.
Documentation: The combination of well-structured documentation and a vibrant community makes it accessible to developers of all levels.
Tooling and Ecosystem
Tooling and Ecosystem for Next.js:
Next.js benefits from a robust tooling ecosystem and seamless integration with other technologies, primarily in the React ecosystem.
Next.js CLI: Next.js provides a command-line interface (CLI) for initializing projects, running development servers, building applications, and deploying to various platforms.
Vercel: Next.js has a strong association with Vercel, a cloud platform for hosting frontend applications. The Vercel platform offers features like serverless functions, automatic deployments, and a global content delivery network (CDN) designed to work seamlessly with Next.js.
-React Ecosystem: Since Next.js is built on top of React, it seamlessly integrates with the entire React ecosystem, including tools like Create React App (CRA), React Router, and state management libraries like Redux and Recoil.
API Routes: Next.js includes a built-in API routing system, allowing you to create serverless functions and RESTful APIs within your application. These routes can be used for server-side logic and data fetching.
Deployment Platforms: Next.js projects can be easily deployed to various hosting platforms, including Vercel, Netlify, AWS Amplify, and others. Deployment is often as simple as connecting your Git repository.
React Devtools: Next.js integrates well with React Devtools, making it easy to debug React components and inspect the component tree during development.
Tooling and Ecosystem for Nuxt.js:
Nuxt.js is closely associated with the Vue.js ecosystem and provides a set of tools that streamline Vue.js development.
Nuxt.js CLI: Nuxt.js offers a feature-rich CLI that facilitates project setup, development server management, building, and deployment. The CLI provides commands for generating pages, layouts, and components.
Vue.js Ecosystem: Nuxt.js is built on Vue.js, so it seamlessly integrates with Vue.js libraries and tools. This includes Vue Router for client-side routing and VueX for state management.
Vue Devtools: Nuxt.js works well with Vue Devtools, allowing you to inspect and debug Vue components during development.
Nuxt Content: Nuxt Content is a module for managing content in Nuxt.js applications. It provides a simple way to create and manage content for blogs, documentation sites, and more.
Deployment Platforms: Nuxt.js projects can be deployed to various hosting platforms, including Vercel, Netlify, AWS Amplify, and others. Like Next.js, deployment is often straightforward, thanks to integrations with Git.
Server Middleware: Nuxt.js allows you to define server middleware for custom server-side logic. This can be useful for tasks like authentication or handling specific API routes.
Feature | Next.js | Nuxt.js |
---|---|---|
Server-Side Rendering (SSR) | Designed with SSR as a core feature | Built with SSR in mind |
Ease of Implementation: Straightforward setup | Ease of Implementation: Automatic SSR for Vue.js | |
Customization: High degree of flexibility | Customization: asyncData and fetch methods | |
Dynamic Importing: getServerSideProps and getInitialProps | Layouts and Middleware support | |
SEO Benefits: Strong SEO advantages | SEO Benefits: SEO-friendly HTML content | |
Static Site Generation (SSG) | Robust support for SSG | Robust support for SSG |
Static Pages, Dynamic Routes, Data Fetching | Static Pages, Dynamic Routes, Data Fetching | |
Incremental Static Regeneration (ISR) | Full Static Mode | |
SEO Benefits: Strong SEO advantages | SEO Benefits: SEO-friendly HTML content | |
Routing | File-Based Routing, Custom Routing, Nested Routes | File-Based Routing, Dynamic Routes, Nested Routes |
Link Component, Programmatic Routing | Link Component, Middleware | |
State Management | No built-in state management, compatible with React, API Routes, Context API | VueX, Local Component State, NuxtServerInit, Plugins |
Performance | SSR, Code Splitting, Caching, Client-Side Navigation | SSR, Code Splitting, Caching, Client-Side Navigation |
Development Experience | Learning Curve | Learning Curve |
Ease of Learning for React Developers, React Ecosystem | Ease of Learning for Vue.js Developers, Vue Ecosystem | |
Routing Simplicity, Documentation, Community Support | Convention-Based Approach, Official Guides | |
Tooling and Ecosystem | Tooling and Ecosystem |
Feature comparison between Next.js and Nuxt.js
Server-Side Rendering (SSR):
Next.js:
Designed with SSR as a core feature.
Ease of Implementation: Straightforward setup with automatic server-side rendering for React components.
Customization: Offers a high degree of customization for SSR, allowing control over data fetching and rendering.
Dynamic Importing: Supports dynamic imports for efficient data fetching and rendering.
SEO Benefits: Provides strong SEO advantages by generating SEO-friendly HTML content on the server.
Nuxt.js:
Built with SSR in mind, providing automatic server-side rendering for Vue.js components.
Customization: Allows customization of SSR through asyncData and fetch methods.
Layouts and Middleware: Provides layout and middleware support for server-side logic and templates.
SEO Benefits: Offers SEO advantages through its SSR capabilities, generating SEO-friendly HTML content on the server.
Static Site Generation (SSG):
Next.js:
Robust support for SSG with features like static pages, dynamic routes, and data fetching during the build process.
Incremental Static Regeneration (ISR) allows on-demand regeneration of static pages.
Generates optimized static HTML files for better search engine indexing.
Nuxt.js:
Strong support for SSG with static pages, dynamic routes, and multiple data-fetching methods during the build process.
Full Static Mode for websites with minimal dynamic content.
Generates SEO-friendly static HTML content.
Routing:
Next.js:
File-Based Routing: Follows a file-based routing [system}(https://verpex.com/blog/cloud-hosting/operating-systems-common-in-cloud-data-centers) for simplicity.
Custom Routing: Allows implementation of custom route patterns.
Nested Routes: Supports nested routes within the pages directory.
Link Component: Provides a Link component for client-side navigation.
Programmatic Routing: Enables programmatic routing using the Router object.
Nuxt.js:
File-Based Routing: Similar to Next.js, it follows a file-based routing system.
Dynamic Routes: Supports dynamic routes with parameters.
Nested Routes: Supports nested routes through directories and layouts.
Link Component: Offers a nuxt-link component for client-side navigation.
Middleware: Allows applying middleware functions to routes for custom logic.
State Management:
Next.js:
No built-in state management but integrates seamlessly with React state management solutions and libraries.
Server-Side State: Supports passing server-generated state to the client.
API Routes: It makes creating API routes for server-side logic and state management easy.
Context API: Allows using React's Context API for sharing state.
Nuxt.js:
Integrates well with VueX for Vue.js's official state management.
Local Component State: Supports component-level state management.
NuxtServerInit: Provides a special action for initializing the state on the server side.
Plugins: Allows use of Vue.js plugins for state management.
Performance:
Both frameworks offer strong performance through SSR, code splitting, caching, and client-side navigation. Specific benchmarks may vary depending on project requirements and configurations.
Development Experience:
Learning Curve:
Next.js:
Easier for React developers due to its alignment with React's concepts.
Benefits from React's extensive ecosystem and resources.
Nuxt.js:
Easier for Vue.js developers, following Vue's conventions.
Benefits from Vue.js's ecosystem and Vue-specific resources.
Documentation and Community Support:
Both frameworks have comprehensive documentation and active communities, making them accessible to developers of all levels. Next.js benefits from the React ecosystem, while Nuxt.js benefits from the Vue.js ecosystem, with extensive resources and libraries available for each.
Advantages and Disadvantages
Advantages of Next.js
Server-Side Rendering (SSR): Next.js excels in SSR, allowing you to render pages on the server. This improves SEO, initial page load times, and the overall user experience.
Ease of Use: Next.js offers a simple file-based routing system and a clear project structure, making it easy to get started and maintain projects.
React Integration: It seamlessly integrates with React, leveraging the extensive React ecosystem, including components, libraries, and developer tools.
Serverless Functions: Next.js supports serverless functions, enabling you to create APIs and serverless endpoints within your application.
Incremental Static Regeneration (ISR): ISR allows you to re-generate static pages on-demand, keeping content fresh without rebuilding the entire site.
Strong Community: Next.js has a large and active community, providing ample resources, documentation, and support.
Disadvantages of Next.js
Learning Curve: For developers new to React, Next.js may have a learning curve, especially when working with React-specific concepts.
Complexity for Simple Projects: For very simple projects, Next.js's powerful features like SSR and ISR might be overkill and add unnecessary complexity.
Framework Size: Including all Next.js features in your project may result in a larger bundle size, which can affect initial load times.
Advantages of Nuxt.js
Vue.js Integration: Nuxt.js is built on Vue.js, offering seamless integration with Vue components, Vue Router, and VueX for state management.
Convenient Convention: Nuxt.js follows conventions for routing and project structure, simplifying development and reducing configuration overhead.
Static Site Generation (SSG): Nuxt.js supports SSG, allowing you to generate static pages for improved performance and SEO.
Content Module: Nuxt Content is a built-in module for content management, making it easy to create and manage content for websites and blogs.
Official Modules: Nuxt.js provides official modules for common tasks like authentication, proxying, and loading CSS resources, streamlining development.
Vue Devtools Compatibility: You can use Vue Devtools to inspect and debug Vue components in Nuxt.js applications.
Disadvantages of Nuxt.js
Vue.js Dependency: If you are not already familiar with Vue.js, there may be a learning curve associated with adopting Nuxt.js.
Flexibility: While Nuxt.js's conventions are convenient for most projects, they may not fit well with highly customized or unconventional project requirements.
Smaller Ecosystem: The Vue.js ecosystem is robust but may not be as extensive as the React ecosystem in terms of available libraries and tools.
It is essential to consider these advantages and disadvantages in the context of your project's specific needs and your team's expertise when choosing between Next.js and Nuxt.js. Both frameworks offer powerful features for building modern web applications.
Recommendation
Choosing between Next.js and Nuxt.js ultimately depends on your specific project requirements and your familiarity with React or Vue.js.
Choose Next.js if:
You prefer React and want to leverage the React ecosystem.
You need strong SSR capabilities for SEO or content that frequently changes.
You want the flexibility to choose your state management solution.
You are building a project where serverless functions or ISR are essential.
Choose Nuxt.js if:
You prefer Vue.js or are already experienced with Vue.js.
Your project involves content management, such as a blog or documentation site.
You appreciate convention-based development and want to minimize configuration.
You want built-in modules for common tasks like authentication or proxying.
Conclusion
Next.js and Nuxt.js are both powerful frameworks for SSR and SSG in JavaScript. Next.js, based on React, offers flexibility and integration with the React ecosystem. Nuxt.js, based on Vue.js, follows conventions and provides Vue.js's Vuex for state management.
The choice between these two frameworks largely depends on your familiarity with React or Vue.js, as well as the specific needs of your project.
Frequently Asked Questions
Are there any enterprise-level features or support plans for either framework?
Both Next.js and Nuxt.js have enterprise-level features and support plans available. For example, Next.js offers Next.js Enterprise, and Nuxt.js provides Nuxt.js Enterprise. These offerings may include features like enhanced support, performance optimizations, and custom solutions tailored to enterprise needs.
How do Next.js and Nuxt.js handle internationalization and localization?
Both frameworks have solutions for internationalization (i18n) and localization (l10n). They offer plugins and modules that simplify the process of translating content and managing multilingual applications.
Can I use third-party libraries or packages with both frameworks?
Yes, you can use third-party libraries and packages with both Next.js and Nuxt.js. They are compatible with the broader JavaScript ecosystem, and you can incorporate external libraries and packages as needed.
Which framework has a more straightforward learning curve?
The learning curve can vary depending on your prior experience with React or Vue.js. If you are already familiar with React, Next.js may have a gentler learning curve, and the same applies to Vue.js and Nuxt.js. However, both frameworks offer accessible documentation and communities to support learning.
Do both frameworks support Progressive Web Apps (PWAs)?
Yes, both Next.js and Nuxt.js provide features and tools that support the creation of Progressive Web Apps (PWAs). You can implement service workers, cache assets, and follow best practices for creating PWAs in your projects.
What are the deployment options available for Next.js and Nuxt.js?
Both frameworks are versatile when it comes to deployment. You can deploy them to various hosting platforms, including Vercel, Netlify, AWS Amplify, traditional web hosts, or your own server infrastructure. Deployment is often straightforward, especially when using platforms like Vercel and Netlify.
Can I use GraphQL easily with both frameworks?
Yes, you can use GraphQL with both Next.js and Nuxt.js. They do not include GraphQL by default but can integrate with GraphQL clients and servers seamlessly. Popular libraries like Apollo Client and Relay are commonly used for GraphQL integration.
How often do Next.js and Nuxt.js receive updates?
Both frameworks receive regular updates, including bug fixes, new features, and performance improvements. The frequency of updates can vary, but both communities are committed to maintaining and enhancing their frameworks.
Are there any built-in state management solutions in either framework?
Nuxt.js provides built-in support for VueX, Vue.js's official state management library. Next.js, on the other hand, leaves the choice of state management to developers, allowing flexibility in selecting a state management solution like React's Context API, Redux, or Recoil.
Which framework offers better SEO features out of the box?
Both Next.js and Nuxt.js are strong contenders for SEO-friendly applications due to their SSR capabilities. However, Next.js is often recognized for its SSR optimization, which can be advantageous for SEO. Ultimately, SEO effectiveness depends on your implementation and content strategy.
Can I migrate an existing React or Vue project to Next.js or Nuxt.js?
Yes, it is possible to migrate an existing React or Vue project to Next.js or Nuxt.js. However, the migration process may involve adjustments to the project structure, routing, and codebase to align with the conventions of the chosen framework. It is recommended to consult the migration guides provided by Next.js and Nuxt.js for detailed instructions.
Which framework has better performance?
The performance of Next.js and Nuxt.js can vary depending on your project's specific implementation and optimizations. Both frameworks are designed with performance in mind, and their performance is often comparable. The actual performance of your application will depend on factors like code quality, server infrastructure, and content delivery.
Can I use TypeScript with both Next.js and Nuxt.js?
Yes, both Next.js and Nuxt.js have good support for TypeScript. You can set up TypeScript in your projects and enjoy benefits like static typing, code completion, and better developer tooling.
Is Next.js only for React developers and Nuxt.js only for Vue developers?
While Next.js is primarily associated with React, and Nuxt.js is built on Vue.js, you are not limited to using them exclusively with their respective libraries. Both frameworks can be used to build applications in their native library (Next.js with React, Nuxt.js with Vue.js), but they also offer flexibility for mixing and matching with other technologies if needed. For instance, you can use Vue components within a Next.js project or React components within a Nuxt.js project.
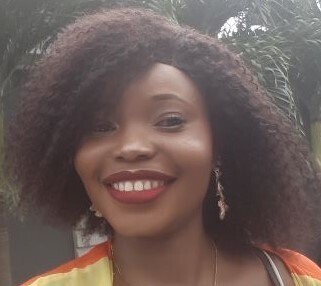
Yetunde Salami is a seasoned technical writer with expertise in the hosting industry. With 8 years of experience in the field, she has a deep understanding of complex technical concepts and the ability to communicate them clearly and concisely to a wide range of audiences. At Verpex Hosting, she is responsible for writing blog posts, knowledgebase articles, and other resources that help customers understand and use the company's products and services. When she is not writing, Yetunde is an avid reader of romance novels and enjoys fine dining.
View all posts by Yetunde Salami