In the last few years TypeScript has seen an absolute explosion in adoption and popularity. Some of the tools you’re probably very familiar with as web developers, such as VSCode, Chrome DevTools, and more, are all now authored in TypeScript.
TypeScript brings many benefits to a project that help explain its widespread adoption:
1. Being able to add types to your code increases its robustness; the compiler is able to spot a whole set of errors that would otherwise result in your application breaking when a user interacts with it.
2. Adding types is a great way to try to make the code easier to understand for developers, and particularly those who are new to the codebase.
3. Having the compiler with you makes it easier and safer to modify your code; now every refactoring you tackle has a compiler behind it ensuring that nothing gets forgotten.
If you’ve decided that 2022 is the year you dive into TypeScript, then stay right where you are, because in this article I’ll suggest a few ways in which you can dip your toes into the type-safe, compiler-checked water.
Where To Learn TypeScript
1. Use TypeScript on a new project
There’s no denying that the easiest way to try a new technology is often to have a brand new project - either at work, or your own side-project, to try it on.
It couldn’t be any easier to get up and running either; popular build tool Vite (which I highly recommend) makes it really easy to get up and running - when starting a new project it will let you pick TypeScript and provide you with a starter template ready to go.
2. Add TypeScript to existing project
Unfortunately most of us don’t get the chance to start brand new projects on a regular basis. At work you’re probably working on more established codebases that have built up over time, and outside of work you may quite understandably not have the time or inclination to start your own side projects. The good news is that there’s a myriad of ways to adopt TypeScript into existing projects and use it more and more as you get confident with it.
3. Using TypeScript in JavaScript files
The first step of adopting TypeScript used to be to create a .ts
file and start writing. You would then have to update your build system to support those files, and make sure they worked alongside JavaScript files.
TypeScript now has support for JSDoc, a format in which you can annotate your code with comments. These comments are used to document what arguments a function might take, and what it returns, but you can also use them to add type information, and this is supported by the TypeScript compiler.
Whilst it is slightly more cumbersome to define and add types in this system, the huge benefit that it has is that you don’t have to rewrite any code, make any build system changes or rename your file extensions from “js” to “ts”. It’s a fantastic way to lightly sprinkle some TypeScript into your code.
You can find the TypeScript guide to using JSDoc here to get a sense for how to use it.
4. Use the TypeScript playground to debug and share examples
The TypeScript Playground is a fantastic resource for understanding TypeScript. If you hit upon a compiler error in your codebase, or a situation where you can’t figure out the best types to use, the TS Playground is a great tool where you can reduce the problem down to a few lines of code. It will update the URL as you write code, so you can share your code with a colleague to work on it together. You can also use this when asking for help online to give people an easy reproduction of your problem.
The TS Playground will configure TypeScript, but you can also change the settings to see how the various configuration options impact how TypeScript checks and builds your code. There are a lot of options and ways to configure TypeScript - this handy list on the TS docs site is worth keeping handy! It’s also the reason I recommend using the aforementioned Vite to create new TypeScript projects, it will set it up with reasonable defaults.
5. Use TypeScript separately to your build tool to type check only
We’ve talked about adopting TypeScript via JSDoc comments, and that’s a great way to get started. Ultimately long term you’ll want to migrate to writing code in TypeScript directly. If you’re trying to adopt TypeScript on a large project, it’s likely you have your build tooling setup directly; whether that be Vite, Webpack, Parcel, Rollup or any other tool. It’s worth seeing if those tools have TypeScript support (the answer is almost certainly yes - just google “
If you don’t want to dive into restructuring your build tool, another option is to run the TypeScript compiler in what’s called “no emit” mode. In this mode TypeScript doesn’t try to compile your code and create any output, it will just type check your code. This means you can have all the benefits of TypeScript’s compiler without impacting your build system, and is a great way to take small steps towards full TypeScript adoption.
The TypeScript compiler can also be run against JS files, so if you start adopting TypeScript and have a codebase that mixes JS and TS files, that’s not a problem.
6. Use an editor with good TypeScript support
One of the huge benefits of TypeScript is its auto completion and editor integration. Because TypeScript can deduce the types of your variables and functions, that means it can give you smarter autocomplete suggestions, show you the arguments a function takes, and highlight where you’ve written unsafe code (such as trying to call a method like toLowerCase()
on a number, not a string).
The most popular editor today is VSCode. Not only is it built by Microsoft, the same folks who build and maintain TypeScript, but it’s also written in TypeScript and has fantastic support right out of the box. If you’re looking for an editor that just works with TypeScript, this is the one.
Alternatively if you have another editor of choice, it’s highly likely that it has great TypeScript support either baked in or via a plugin. “
7. Using eslint with TypesScript
If you’ve written any JavaScript over the last five plus years, you’ll almost certainly have come across ESLint. ESLint has become the definitive tool for automatic code quality checks in JavaScript, and typescript-eslint has added TypeScript support to it. This lets you enable any number of rules to enforce conventions and standards across your TypeScript, and you can use the recommended rule set to start from a great foundation. If you’re new to TypeScript, using these rules is a great way to learn the common conventions that you’ll find across most codebases and get called out by the tooling when you’re doing something that could be done in a better way.
8. TypeScript tutorials, books and courses
As TypeScript’s popularity has exploded, so has the amount of content that’s been created to help people learn the language. Here’s a pick of some of my favourites, but you’ll find plenty more online. My one word of warning is to be careful when any article you find was written - TypeScript has changed a little over the years and articles from a few years ago may now be obsolete or slightly outdated.
Bonus: my favourite online and offline resources for learning TypeScript:
- The official TypeScript docs recently received a large revamp and is now a great place to start. They have customised quick start guides depending on your background - for example, if you’re a Java developer, you can go through the guide specifically for you. You’ll also find the TypeScript handbook, which is really required reading once you’re beyond the quick start tutorial. You can also download the handbook contents as an eBook if you’d prefer so you have access to it offline.
- If you’re using VSCode, I highly recommend giving the VSCode & TypeScript tutorial a read. It’ll help you understand the extra editor capabilities available to you and how to make use of them.
- If, like me, you prefer offline resources, “TypeScript in 50 Lessons” by Stefan Baumgartner comes highly recommended and has excellent reviews. There’s even a copy sat on my bookshelf! It’s a great read and does a great job of breaking TypeScript down into short sections highlighting all the functionality packed into it. You can get a physical copy or stick to the eBook version too. Stefan’s blog is also one that’s worth having in your list of regularly visited websites as he regularly posts great TypeScript articles there too.
- If video content is your preferred medium, I don’t think you can go wrong with the content that Egghead provides. There are a vast amount of TypeScript courses on here, ranging from beginner through to advanced.
Conclusion
Over the last few years TypeScript has exploded in popularity. I’ve ridden that wave, and in that time have migrated nearly all the projects I work on to TypeScript. Whilst there was a little bit of a learning curve, the productivity benefits that TypeScript and its compiler provide have made me more efficient, quicker to spot bugs and more likely to build robust applications that won’t fail as often. I can’t speak highly enough of the impact TypeScript has had on my work, and I hope with the information and resources linked above, you’ll experience the same in 2022.
Frequently Asked Questions
Can you migrate my existing website over?
Yes, and without issue. Regardless of how many websites you’re managing, we’ll bring all of them under the Verpex wing free of charge. Just send us the details of your websites when you’ve signed up to get started.
Do I need web developer skills to use Shopify or WooCommerce?
No. Both platforms welcome beginners, but you might get more out of WooCommerce’s extensions if you’ve got some previous experience.
Can I migrate my store to Verpex?
Yes; these days, it’s a fairly straightforward process to migrate your store in full. Simply get in touch with one of our service agents and they can migrate the store for you.
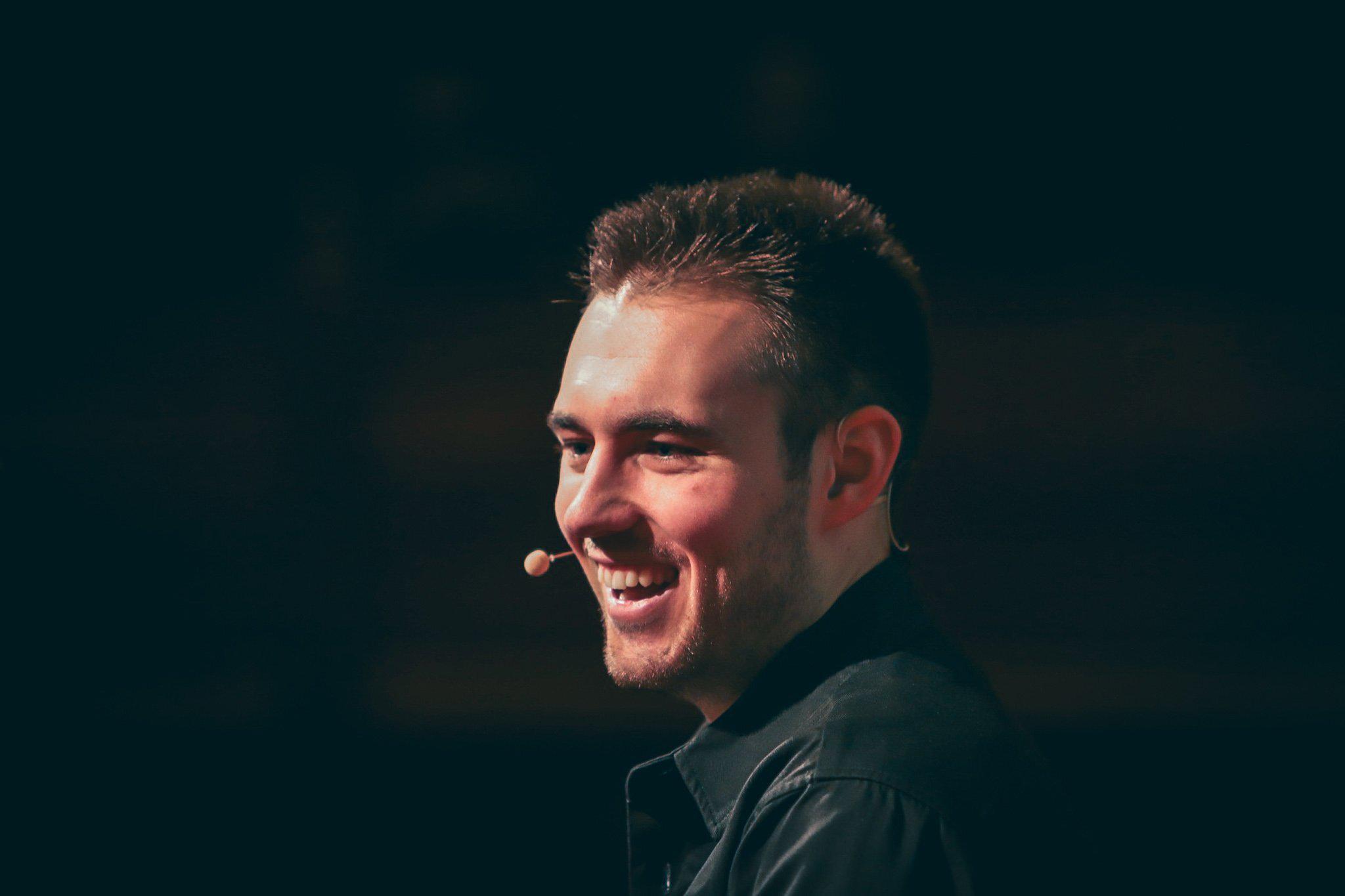
Jack is a Software Engineer at Google where he spends most of his time working on Chrome DevTools, writing HTML, CSS and TypeScript.
View all posts by Jack Franklin