There are principles for coding and programming, these principles are necessary for readability and maintainability amongst many other advantages. Interaction with code is second nature to developers, and collaborating on different projects with different developers is unavoidable.
It is possible to write illegible, disorganized code that will leave you and your team members perplexed and frustrated, or code that your collaborators are unable to understand. Apart from writing code that is hard to read, you can also write code that is unnecessarily bulky, such as writing ten lines of code instead of three. So, how can we build clean, readable code? Why should JavaScript developers be aware of the concept of clean code? In this post, we'll discuss common principles to answer these questions. Let's get started.
What is Clean Code?
The nature of software is “continuous integration and continuous deployment (CI/CD),” and having a clean code is of utmost importance. Clean code is simply a technique of writing code that is readable and self-explanatory. It can also be defined as code that is free from unnecessary complexity, and clutter with a meaningful/clear structure, and logic. Clean code in JavaScript just like any other programming language makes the software easy to maintain, extend and debug. Let’s take a look at some clean practices for writing clean code in JavaScript.
Naming Conventions
Naming variables in a concise manner is of great importance in JavaScript. The importance of descriptive variable names includes
Readability: Properly named variables make code easier to read and understand, particularly when working on projects that will be maintained by other software engineers.
Maintainability: Clear variable names make it easy to maintain code and prevent errors.
Self-Documentation: You’ll always come back to your code, therefore, you should be able to understand why it was named a certain way to perform a specific task. Self-documentation can also be helpful for collaboration and maintenance long term.
Let’s take a look at a few examples:
If you have a variable to store a username, you cannot name the variable as Const x; or Const y; because there’s no context. This will confuse you and your collaborators, it is also crucial not to rely on your own memory.
Further examples;
const user = true; // This can be anything
// The better approach would be to write;
const isUserActive = true // This gives you a clearer description
The alternative would be to write;
In this case, what does cnt mean? this type of naming can be very hectic to decipher.
const cnt = prompt( do something);
There are general rules to name variables in JavaScript;
- Camel Case: userName
- Snake Case: user_name
- Kebab Case: user-name
- PascalCase: UserName
The camel case is the most used and accepted naming convention in the JavaScript community and is the most likely recommended style to use.
Unnecessary Naming:
Apart from naming variables that are “unclear,” you can also name variables that are unnecessary.
Let’s create an object;
Const author = {
authorId: 1
authorName: “Charles Dickens”
AuthorDob: “7 February 1812”
}
If a collaborator wants to retrieve the data, for instance, these steps have to be taken: author.authorId or author.authorName. This makes it unnecessary; instead, it should be written as follows:
Const author = {
id: 1
name: “Charles Dickens”
dateOfBirth: “7 February 1812”
}
Only use lengthy names if it is necessary; also, all variable names must be unique to avoid errors.
Comment:
There are different ways to write comments in JavaScript: single-line and multiple-line comments.
// This is a single line comment
/*
This is a multiple-line comment
*/
Adding comments to your code is useful; there are good and bad comments that you may be unaware of.
What makes a good code comment?
- A good comment must be concise.
- A good comment must provide value.
- A good comment must magnify or warn of importance.
- Display legal comment.
What makes a bad comment?
- Unnecessary and lengthy comment.
- Incomplete comment.
Limit Global Variables:
Global variables are defined outside of any function or block scope. A global variable can be accessed from anywhere in the code; therefore, declaring a global variable can be hard to manage and debug for large scripts, especially if various parts of the code can alter the global variable. However, it is considered good practice to use local variables instead.
The global variable looks like so;
let userName = “Alexandr Wang” //global variable
Function displayName(){
console.log(`Hello ${userName}`)
}
The local variable looks like so;
Function displayName(){
let userName = “Alexandr Wang” //local variable
console.log(`Hello ${userName}`)
}
displayName();
It may seem that the code is limited because it can only be accessed locally in the code block where it is defined. However, locally scoped variables provide security for our code.
Standardize Formatting:
Standardized formatting is a way of authoring code that incorporates indentation, spacing, capitalization, line breaks, and other elements. Using specific formats gives your code a consistent appearance and enhances its overall look and feel. Prettier https://prettier.io/ is an example of a JavaScript formatter that can make your code unified. Also, you can simply learn the rules of formatting JavaScript if you do not want to rely on a tool for assistance.
Conditional Short-hand:
Conditional short-hand syntax is used to write concise conditional statements. The most common conditional short-hand is called the Ternary Operator. The ternary operator syntax is represented as so; a?b:c or condition?exprIfTrue:expIfFalse
Let’s check if two numbers are equal or not
function checkNum(a,b){
return a===b? “Equal” : “not equal”
}
checkNum(1,2);
// This would return the statement “not equal”
Instead of chaining if else statements, we can simply check with one line of code, and this reduces your code significantly.
Template Literals:
Template literals are literals that are a new feature in ES6 used for interpolation and concatenation.
const firstName = “Julia”, lastName = “Robert”
const greeting = “Hello” + firstName + “ ” + lastName
console.log(greeting)
Using template literals, we’ll write this as;
const firstName = “Julia”, lastName = “Robert”
const greeting = `Hello
${firstName}
${lastName}
`
// Note, this can be written on one line, but it’s spread out for clarity sake.
Arrow Function:
The arrow function with an implicit return, is an example of why the arrow function should be considered; however, it only works for a single expression to be evaluated and returned. Let’s write a function called SUM that takes in two arguments. Using the arrow function, we can write our function on a single line. Implicit return looks like so;
const sum=(a,b)=> a+b;
Explicit return looks like so;
function sum (a,b) {
return a + b;
}
This is especially useful for short lines of code, and not required in most cases.
Loops:
In JavaScript, loops are used to perform repeated tasks. There are numerous ways to write loops;
In this example, we’re looping through an array of animals,
const animals=[‘Tiger’, ‘Lion’, ‘Wolf’, ‘Hippo’]
for(let i=0; i< animals.length; i++){
console.table(i,animals[i]
}
You could write this;
const animals=[‘Tiger’, ‘Lion’, ‘Wolf’, ‘Hippo’]
for(animal of animals){
console.log(animal)
}
Arrays are iterable by default, an array is iterable if it provides a way for you to create an iterator. In this case, the iterator is “animal”. The Iterator “animal” loops through the block of code for each value in the array.
Or, the use of a forEach method
const animals=[‘Tiger’, ‘Lion’, ‘Wolf’, ‘Hippo’]
animals.forEach(animal => {
console.log(animal)
});
This would print out all the animals in the array just like the first example, and this method is considered a newer way to write for loops.
Error Handling:
There are different types of errors that are likely to occur in JavaScript, a few examples include; syntax error, type error, reference error, range error, and reference error, etc. Error handling identifies and fixes issues quickly delivering a code that is robust and unsusceptible to bugs. Errors are hard to escape in JavaScript, an example would be using a == operator instead of a === operator. How do you identify and fix errors likely to occur? try
… catch
method is one way to handle errors that are thrown to prevent your code from crashing. try
block contains code that will throw an error, and the catch
block contains the code that’ll be executed if an error is thrown.
Linter tool:
A linter tool enforces consistent coding techniques and catches potential errors that may cause issues in your code. Using a linter tool catches errors like missing white spaces and typographical errors, and basically enforces standards. The Linter tool is very well supported in the JavaScript community; you can customize the linter tool according to your requirements or you can also import a predefined tool instead of defining one yourself.
Package Manager for dependencies
Using a node package manager to handle dependencies is good practice for writing clean code in JavaScript. Node package allows you to install and manage dependencies, and keep track of versions of dependencies. You can share your code and dependencies, which will allow others to collaborate on projects. Also, the node package manager manages updates and upgrades of dependencies, reducing the danger of breaking your code. To use npm, you have to create a package.json
file in the project directory, which will contain the dependencies and other information related to the project.
The Conclusion
There are a ton of reasons why you should consider following certain principles for creating clean code in JavaScript. Clean code takes a lot of practice, and following the principles for clean code would make you more efficient and also make your code readable, manageable, and easy to understand.
Iif you need a solid environment to host your JavaScript projects, our JavaScript Hosting solutions offer exceptional reliability and performance.
Frequently Asked Questions
Why should I create a website?
There are many reasons why you should create a website if you’re an artist. You can use it to create a place where people can learn about you, talk about your art, or show off your work.
How do I choose a design for my website?
One of the most important things when creating a website for your art is the design. Even though your pieces of art might be amazing, people will leave if your site is hard to navigate. This is why it’s important that the site is easy on the eyes and easy to navigate.
Do I need to know how to code to use WordPress?
Definitely not. There’s no need to learn coding, since most WordPress users aren’t developers. There’s many plugins and themes you can use to customize your website without coding.
How is the website maintenance carried out?
On a daily basis, software, hardware, vulnerability, MYSQL, CloudLinux paths and cPanel updates are carried out on our servers without a reboot. However, if we have to carry out any maintenance that includes some downtime, we schedule in advance and update our status page
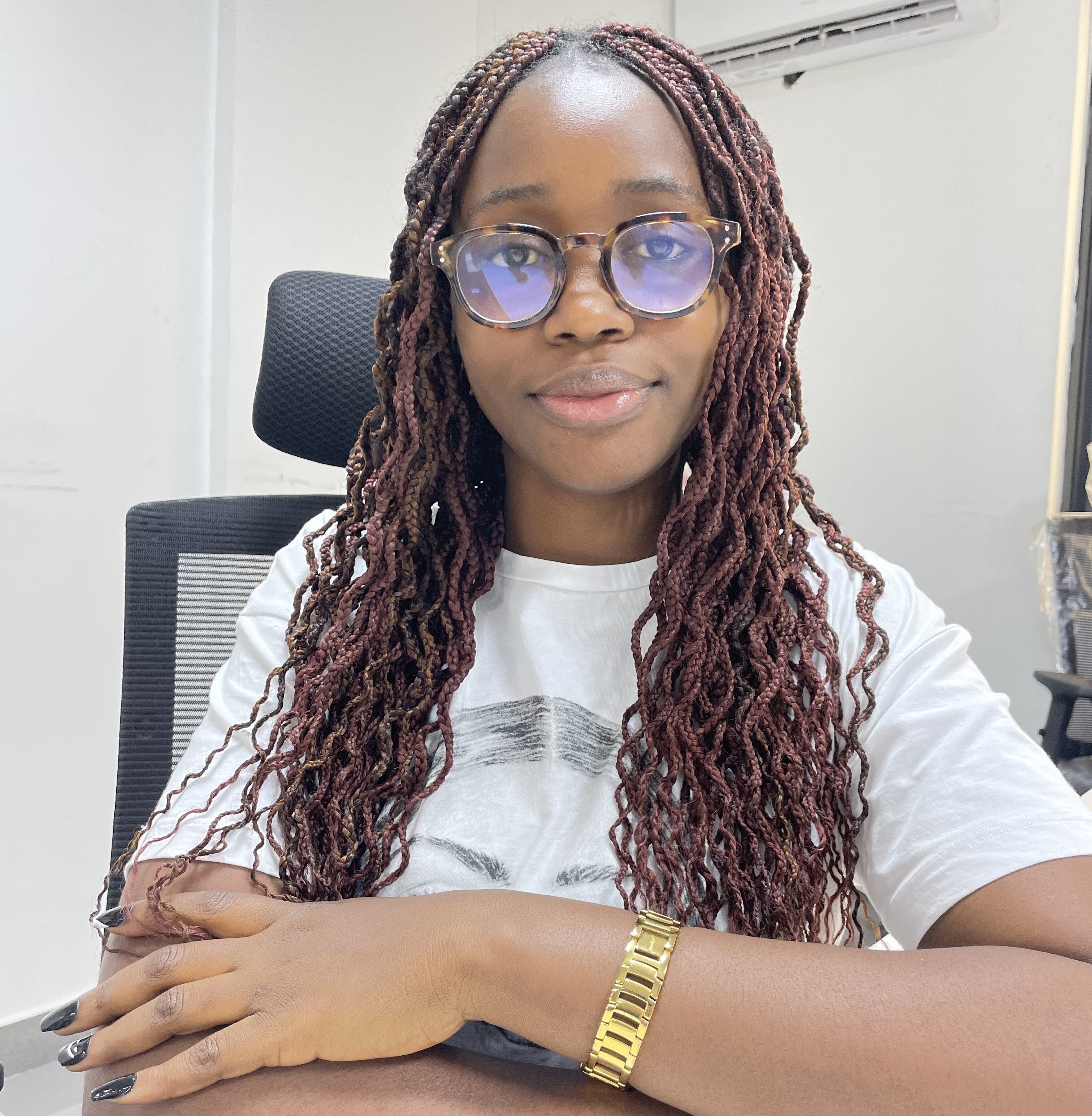
Jessica Agorye is a developer based in Lagos, Nigeria. A witty creative with a love for life, she is dedicated to sharing insights and inspiring others through her writing. With over 5 years of writing experience, she believes that content is king.
View all posts by Jessica Agorye