The evolution of JavaScript from a simple scripting language for adding interactivity to a webpage, to one that can be used to build more scalable and complex applications in front-end and back-end environments has been remarkable to watch. However, growth comes with complexities and the need for better organisation and maintainability.
This is why there are a lot of techniques or concepts that support the complexities of JavaScript. Among the many are JavaScript Modules; in this article, we’ll explore what JavaScript Modules are and their importance in modern web development.
What are JavaScript Modules?
Modules are not exclusive to JavaScript alone; it is a concept that can be found in modern programming; however, the applications may differ.
The Cambridge University dictionary defines a module as a set of separate parts that, when combined, form a complete whole. A Module can be defined as a software component that contains one or more routines, which could be a function or a method.
Modules refer to a collection of individual components within a software system, and a collection of these components make up a program.
The goal of modules is to support modular designs, meaning we can break large systems into pieces. We do not need to understand the internal workings of each component; all we need to know is the interface between them.
Modularity manages complexity by ensuring that the program is broken into pieces small enough that a programmer can understand. Additionally, there has to be a connection between modules to enable their interaction either via protocols or interfaces.
Modules help structure code into independent and reusable components necessary in software development. The features of Modules are:
Encapsulation: Encapsulation keeps certain parts of the module private so they cannot be overridden. This keeps important details safe and allows other parts of the program to connect with the module without the need to know how it functions.
Separation of Concerns: Separation logic keeps the index.html file from loading all the scripts. In this approach, code is separated, and import and export statements are used to foster communication between modules.
Collaboration: This feature promotes code sharing, allowing multiple developers or teams to work on a project independently or dependently.
Reusability: Modules can be reused across different projects, promoting collaboration via shared code bases, saving time, and improving efficiency.
Evolution of JavaScript Code Management
When JavaScript was newly introduced as a way to make web pages dynamic, all application logic was placed into a single file, and then programmers would import JavaScript into an HTML file using a script tag.
<script src=" "> </script>
In the past, people used JavaScript to build smaller and less complex applications than in recent times, now we have interactive applications that are more complex.
Collaboration is a common concept in web development which means developers must share their code especially when working in a team. If a developer writes all the functions or logic in one file and a teammate requires it in their code, how would they access it?
This led to the creation of code modules that are small in size and reusable, to fix the issue of working in a huge JavaScript file. Splitting JavaScript files into bits makes them reusable and easy to share assuming these files need something from each other.
Modules were introduced in JavaScript with ES6 (the 6th version of ECMAScript published in 2015) to keep code short and reusable. This approach makes the codebase cleaner and easier to manage as the project grows. For Instance, when new features are added, developers create separate files for each feature instead of adding everything into one file.
Two important terms for modules we’ll be discussing are, import and export. They are crucial because they enable sharing between modules.
Export and Import
The benefits of import and export in modular programming include code organisation, reusability, and encapsulation.
Export
Export allows you to make specific variables, functions, or objects from a module (JavaScript File) available to use in other modules.
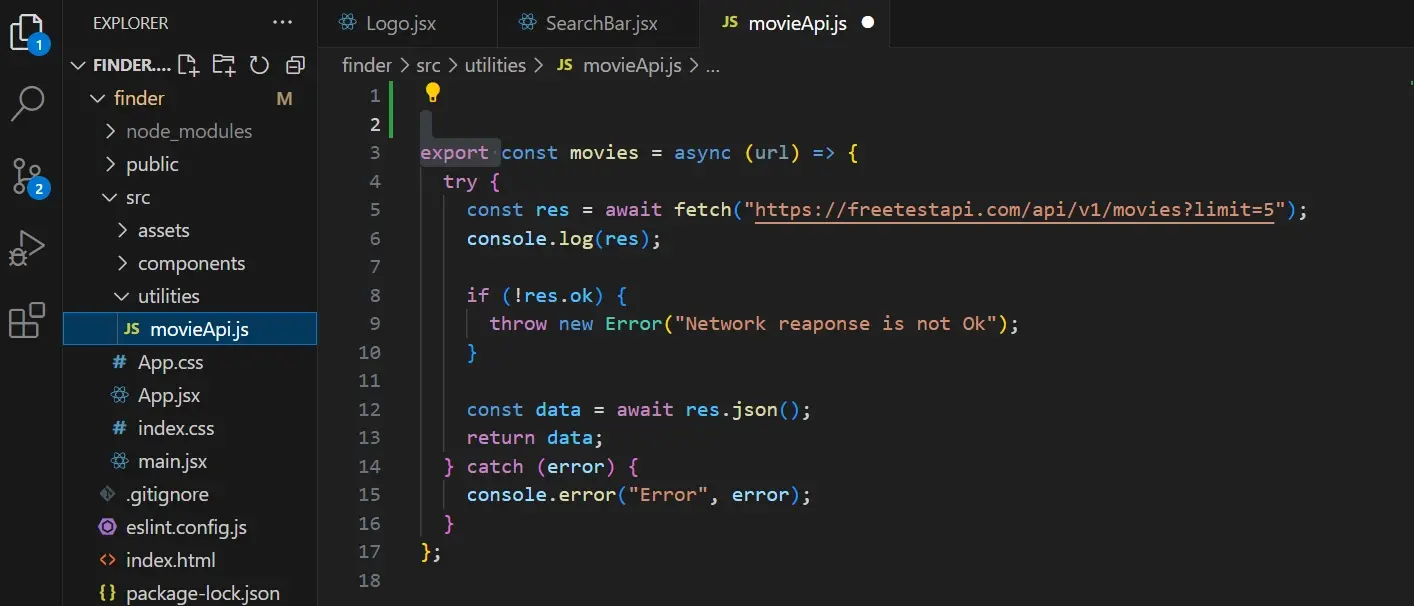
Import
Import allows you to bring exported variables, functions, or objects from other modules (JavaScript files) into a current module.

You can also export multiple functions like so;
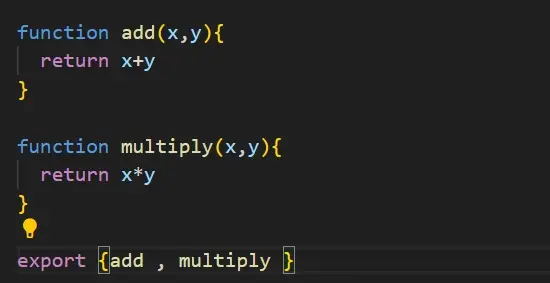
And import the functions where you want to use them like so;

There are two types of exports, namely default and named exports. Let’s take a look at the difference between the two.
Default Export
When using a default export, you can export only one function, variable or object per module as the default export.
You can add other functions in the module, but they cannot be default exports. The default export is the primary item being exported, or the main thing the module is supposed to provide.

Default Import
The subtract function is imported without curly braces; Like so;

Also, you can assign it any name when importing since it’s the default export it will always point or refer to the exported value.
Named Export
Allows you to export variables, functions, or objects from a module by giving them specific names so that other parts of the program or other modules can import them by their names.
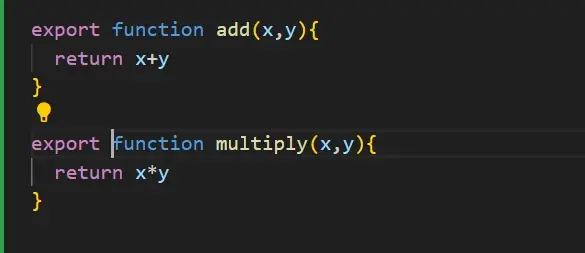
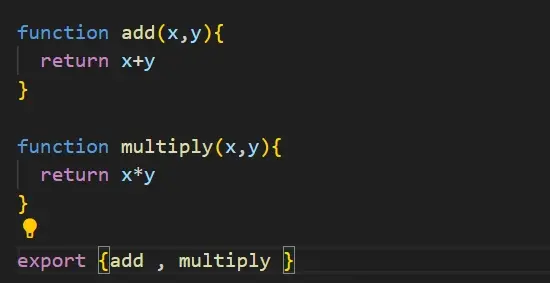
Named Import
Named import allows you to import items that have been exported from a module by their specific name. When importing named exports, you use the curly braces to match the names of items that are being imported.

When importing items that have been exported from a module, you can import multiple items, making it easy to share functions or variables across large projects.
In the above image, you can see that the import contains more than one function. This shows that you can export multiple functions from a single module at once.
Import using Alias
Alias can help avoid conflicts if you have multiple imports with the same name. When you import a function, for instance, you can give it a different name using a keyword.

In the above image, instead of using the original name “add” which we exported, we are using another name “sum” to reference “add”.
Modules NameSpaces
If you want to import a large amount of data from a module, it can be tedious to import them using curly braces and to separate them using commas like so; {sum, mul, division}

Using namespace, you can Import everything using * then, you can give it a namespace “calc” and then access each function using the namespace “calc.add”
In other words, all (*) the exports from the module located in the utility directory are imported and grouped under the alias named calc.
Combined Export and Import
The process of combined export and import involves having a centralised module where we can import functions and variables from different modules and then re-export them as a whole to another module.
For instance, if we have two different files in the util.js directory and we have another module that needs items from the two different files, instead of importing them separately (imagine if we have over 10 modules and each module has a different functions within them, which means we would have to import them one at a time), what we can do is create another file and then export the modules just like you see in the image below.

To import both modules into another module where they are needed, we can import everything from the combined file using * and namespace like so;

We can see from the image above that when we use the dot notation to summon calc from the combined module.
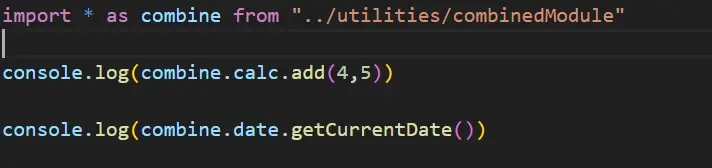
In the above image, we import everything from the combinedModule.js and assign it to the alias name combine.
combine.calc.add (4,5) tells you there is a module exported from combinedModule.js named calc which contains an add function.
combine.date.getCurrentDate() tells you there is a module called date exported from combinedModule.js that contains a getCurrentDate function
Best Practices for Using JavaScript Modules
Several practices are considered best when working with JavaScript Modules, here are a few:
- Keep the module small and give it a clear task or responsibility. For instance, in creating a module for PI calculation or other mathematical logic ensure that the module doesn’t contain anything unrelated, this makes it easier to maintain and debug.
The use of named exports for utility functions is when you have multiple variables in a module.
Consistent naming for files is necessary for understanding the purpose of the module.
Keep related functions that are in the same module instead of putting them in multiple files.
Always import only things you need this reduces memory storage and improves performance
Summary
Module in JavaScript is simply a JavaScript file that has been broken down into smaller bits. They are necessary to promote modularity, code reusability, and maintainability in web development.
Modules also allow developers to break down large codebases into smaller manageable pieces, creating better code organisation and making it easier to work within a codebase and maintain it as the codebase grows.
There are different practices involved in working with modules that are essential for providing developers with better experiences when writing and maintaining their code.
Frequently Asked Questions
How does JavaScript hosting differ from traditional web hosting?
Traditional web hosting is typically optimized for hosting static websites and may not offer the necessary resources or environment for running JavaScript-based applications, especially on the server side. JavaScript hosting, on the other hand, is specifically optimized for JavaScript applications, offering features like Node.js support, database integration, and specialized security measures.
How does Verpex Hosting handle databases for JavaScript hosting?
We support both SQL (like MySQL, PostgreSQL) and NoSQL (such as MongoDB) databases for JavaScript hosting, ensuring seamless integration and management for Node.js applications.
Can I use a CDN with JavaScript hosting?
Yes, it's common to integrate a CDN (Content Delivery Network) with JavaScript hosting to improve load times and reduce latency for users across different geographical locations. Verpex hosting includes CDN services in their plans.
Can I use JavaScript frameworks like React or Vue.js in my WordPress plugin?
Yes, you can. Enqueue your scripts properly using wp_enqueue_script(). Ensure compatibility with WordPress core and consider using the REST API for seamless communication.
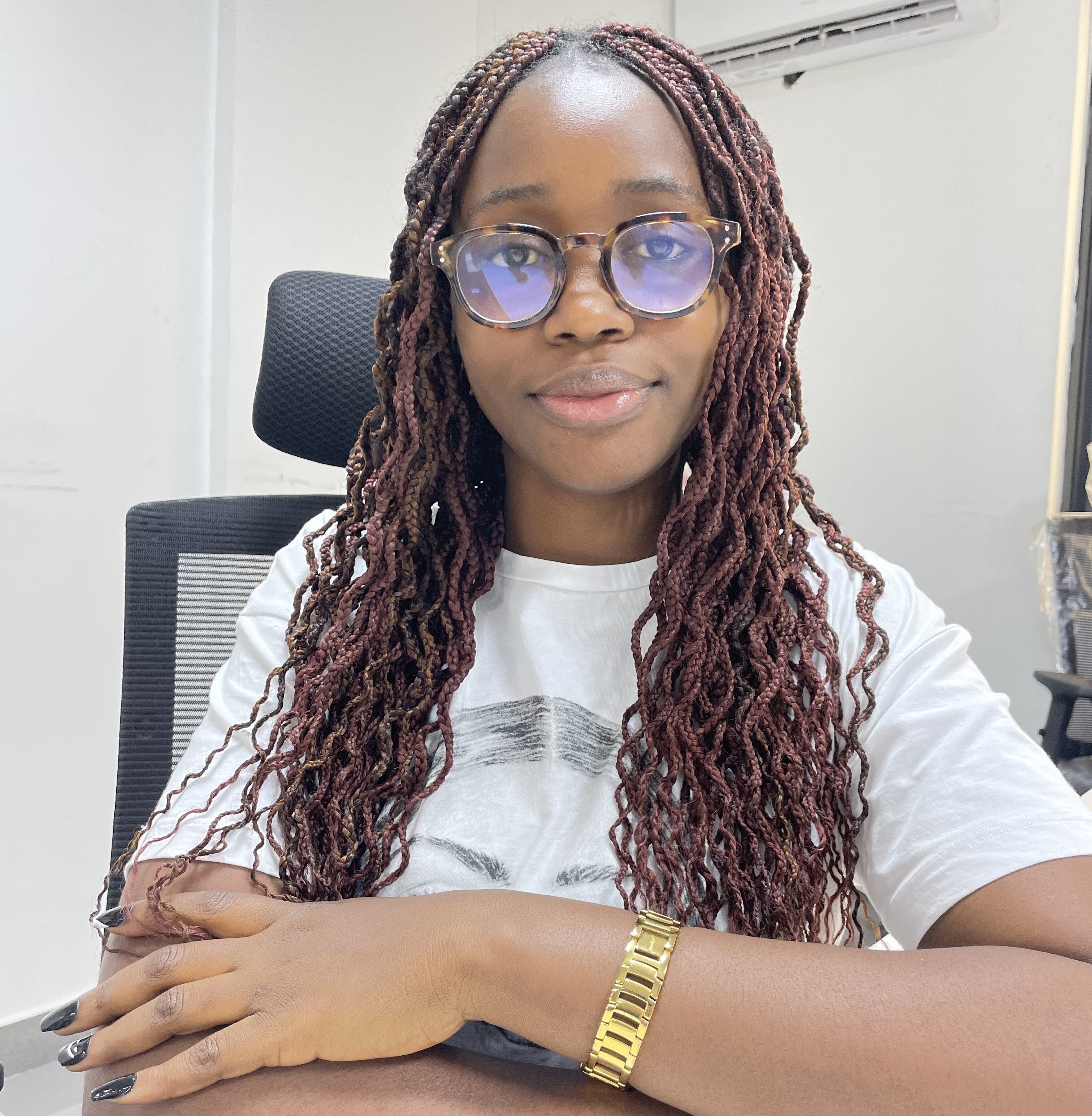
Jessica Agorye is a developer based in Lagos, Nigeria. A witty creative with a love for life, she is dedicated to sharing insights and inspiring others through her writing. With over 5 years of writing experience, she believes that content is king.
View all posts by Jessica Agorye