In JavaScript, the DOM (Document Object Model) is a concept or a means by which HTML documents can be manipulated using JavaScript or other programming languages to perform several tasks.
DOM is one of the most important topics to learn or understand when learning how to create websites or web applications because JavaScript relies heavily on it to change styles, content, and document structure.
In this article, you’ll get to understand how JavaScript can be used to perform tasks using the DOM. There will be several examples provided to learn firsthand and understand how the DOM represents a webpage and how JavaScript communicates with HTML elements via the DOM.
What is DOM?
The Document Object Model, or DOM, as it’s commonly referred to, is a programming interface for web documents. It represents the structure and content of the page for us to manipulate or interact with using JavaScript.
It’s common to hear the term DOM manipulation. This is because JavaScript or other programming languages are used to find elements to work with, handle events, and overall manipulate them, allowing developers to create a dynamic and interactive web application.
The DOM is a JavaScript structure that represents HTML as a JavaScript object.
Several operations can be performed in JavaScript by using DOM, and they include:
- Access and modify the content of HTML elements, e.g. paragraphs, headings,
- Create or delete HTML elements from the document.
- Change the style of HTML elements, e.g. color, font, etc.
- Change attributes of HTML documents, e.g. class, ID, etc.
- Create HTML events and react to events on the page.
Structure of Document Object Model
The foundation of every web page is HTML. When the browser loads a webpage, it receives HTML files from the web server, and the content of the HTML can be seen on our web page as content.
The Document Object Model [DOM] represents HTML or XML documents, and it’s called the DOM tree. Why is it called a DOM tree? The reason it's termed a DOM tree is that its structure resembles a tree; that is, it is organized in a hierarchy with the document as the root node and several child nodes representing the document's texts, components, and attributes.
The HTML or XML document is represented or loaded inside the browser as the DOM tree. The browser gets the HTML/XML and passes it, making it an object, which is the structure of your site and what is referred to as the Document Object Model.
Within the framework of an HTML document, there are;
Document: This represents the entire HTML document, - The document represents web pages displayed in the browser; this is a point of entry into the web page content. E.g., document.body, document.createElement, document.clear()
HTML: <html> <html>
is the root and it contains two child elements, which include;
<head> </head>
which contains meta information and resources necessary for the page’s behavior, e.g.<meta>
,<link>
,<title>
, etc.
<body> </body>
Contains the main content of the web page that will be visible for users to see. This element contains other elements, e.g., text, images, forms, etc.
<!DOCTYPE html>
<html>
<head>
<meta>
<link>
</head>
<body>
<p> A paragraph </p>
<h1> Heading 1 </h1>
</body>
</html>
Note: Each child element contains other child elements. e.g. <head>
contains elements, i.e. <meta>, <link>
Let’s take a look at the browser. If you right-click on a page, an inspect option will appear; select it and click. When you click on the element tab, it shows the representation of the HTML as it’s rendered in the document.
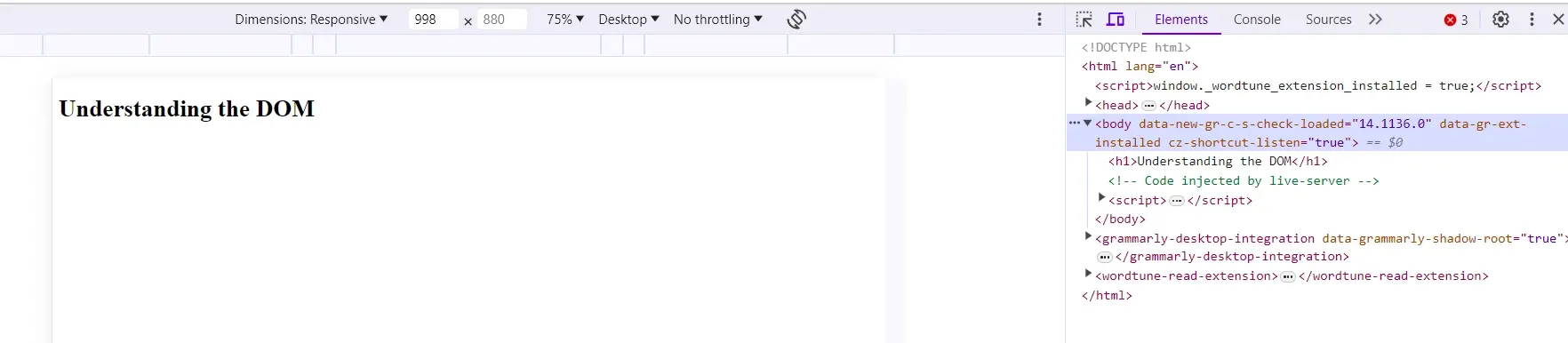
This is not the DOM, but a representation of the DOM. It’s called the Render Tree, and it includes information about how the page should display the visible DOM content and all CSS Object Model (CSSOM) information for different nodes. Including styles, layout, and rendering properties to display the page visually.
In simpler terms, it's a combination of information from both the DOM and the CSSOM.
Note: Everything in the DOM is an object that has properties. Also, if you check the properties tab, you’ll find all the properties you can access.
Let’s see a brief example of how to modify the DOM using JavaScript directly in the console.
The color of the text “Understanding the DOM” was changed in the console by accessing the body via the document object and styling the text.

Now that you know the DOM is made up of objects, these objects are called nodes. The DOM is made up of nodes, which include:
- Element Node
- Attribute Node
- Text Node
- Comment Node
- Processing Instruction Node
- Document Fragment Node
- Document Type Node
- Entity Node
- Entity Reference Node
- Notation Node
However, there are three main types, namely- element, attribute, and text node, which will be explained briefly.
Element Node: Represents HTML elements such as paragraphs, forms, images, tables, etc.
Attribute Node: The attribute node represents properties that are connected to HTML elements. These properties provide additional information on how you can access or modify the element. E.g. ‘src’ attribute of IMG element
<img src=” # ” >
Text Node: Represents the text content within an HTML element, e.g.
<p>
I am the text content within the paragraph element.</p>
To get started with accessing elements and manipulating their descendants, let’s see some primary data types that can be interacted with in the DOM.
- Elements
- Attributes
- TextNodes
- Events
- Functions
- Booleans
- Numbers
- Strings
Here are a few examples of how to interact with the document using the above-mentioned data types.
Assessing Elements in the DOM using JavaScript
There are methods to retrieve or search for elements; they are;
getElementById: Gets elements based on the ID.
getElementByClassName: Gets elements based on the class.
getElementByTagName: Gets elements based on tag names.
getElementByName: Gets elements based on name value.
querySelector & querySelectorAll: These methods are used to select elements based on certain criteria, which include tag names, IDs, classes, and other attributes.
There are also JavaScript methods and properties that can be used to manipulate the elements after accessing them, e.g.
innerHTML: This property allows us to modify or access HTML contents, e.g elements, including child elements
removeChild(): This method is used to remove a child element from its parent element.
replaceChild(): This method is used to replace a child element with another within the parent element.
appendChild(): Appends a child or attaches an element as a child to a parent element.
setAttribute: This method allows us to set the value of an attribute for an element. For example, you can set the ‘src’ attribute for our img element by adding an image like so; imageElement.setAttribute(‘src’, ‘dom.png’)
textContent: This property allows us to access or modify the text content of an element.
Style: CSS style can be used to change or control the appearance of DOM elements, e.g. background color, font color, font size, font weight, font type, etc.
DOM Manipulation using the following methods;
document.getElementById
In this example, we have two elements, a paragraph and a button. The paragraph has the initial text “Test me," which would be changed by accessing the DOM.
<p id="test">Test me </p>
<button id="changeText> Change Text </button>
<script>
let testElement = document. getElementById("test")
let btnElement = document.getElementById("changeText")
btnElement.addEventListener("click", function() {
testElement.textContent = "This text was changed using DOM manipulation."
}
</script>
Key points:
- The HTML file contains a paragraph and button with their respective ID
- In the script file, each element is saved in a variable that is referenced to the HTML element using "getElementById."
- An eventListener is added to the button
- In the addEventListener function, there is a callback function that defines an action when the button is clicked.
- In the callback function, the "textContent" property changes the paragraph's content when the button is clicked.
ATTRIBUTES
// In the HTML File
<h1> Let's toggle an Image </h1>
<img id="img1" src="img1.jpg" alt="#">
<button id="toggleImg" > Change Me </button>
<script>
let toggleElement = document.querySelector("#toggleImg");
let imgElement = document.querySelector("#img1");
toggleElement.addEventListener("click", function () {
let currentImg = imgElement.getAttribute("src");
if (currentImg === "jim.jpeg") {
imgElement.setAttribute("src", "pexels.jpg");
} else {
imgElement.setAttribute("src", "jim.jpeg");
}
});
</script>
The example above is simple image logic that changes one image to another by clicking a button.
toggleElement: This represents the button with the ID "toggleImg” accessed by the document.querySelector
imgElement: Represents the image attribute with ID “img1”
Event Listener: The eventLister is attached to the “toggleElement” (which represents the button), which responds to the “click” event.
getAttribute and setAttribute: The current image src is retrieved using getAttribute. Using a traditional if statement, check if the current image src is “jim.jpeg” ;
If true, it sets the image src to “pexels.jpeg” using setAttribute
If false, it sets the image src to “jim.jpeg” using setAttribute
Summary
The DOM helps tie HTML, CSS, and JavaScript together, allowing developers to manipulate web page content using JavaScript or other programming languages, ultimately creating reactive and interactive web applications. The browser loads HTML into JavaScript objects, and JavaScript can be used to access the DOM and make changes to the behavior of the HTML or XML documents by accessing or retrieving the objects you want to change.
Frequently Asked Questions
Are website builders easy to use?
One of the easiest ways to build a website is with a website builder. Using a website builder doesn't require any programming and coding skills.
Can I afford a website builder?
Yes. Besides paid website builders, there are also free ones; however, they come with fewer options.
Is a website on WordPress safe?
Websites on WordPress are safe, however to avoid hacking keep your website up to date.
Why should I create a website?
There are many reasons why you should create a website if you’re an artist. You can use it to create a place where people can learn about you, talk about your art, or show off your work.
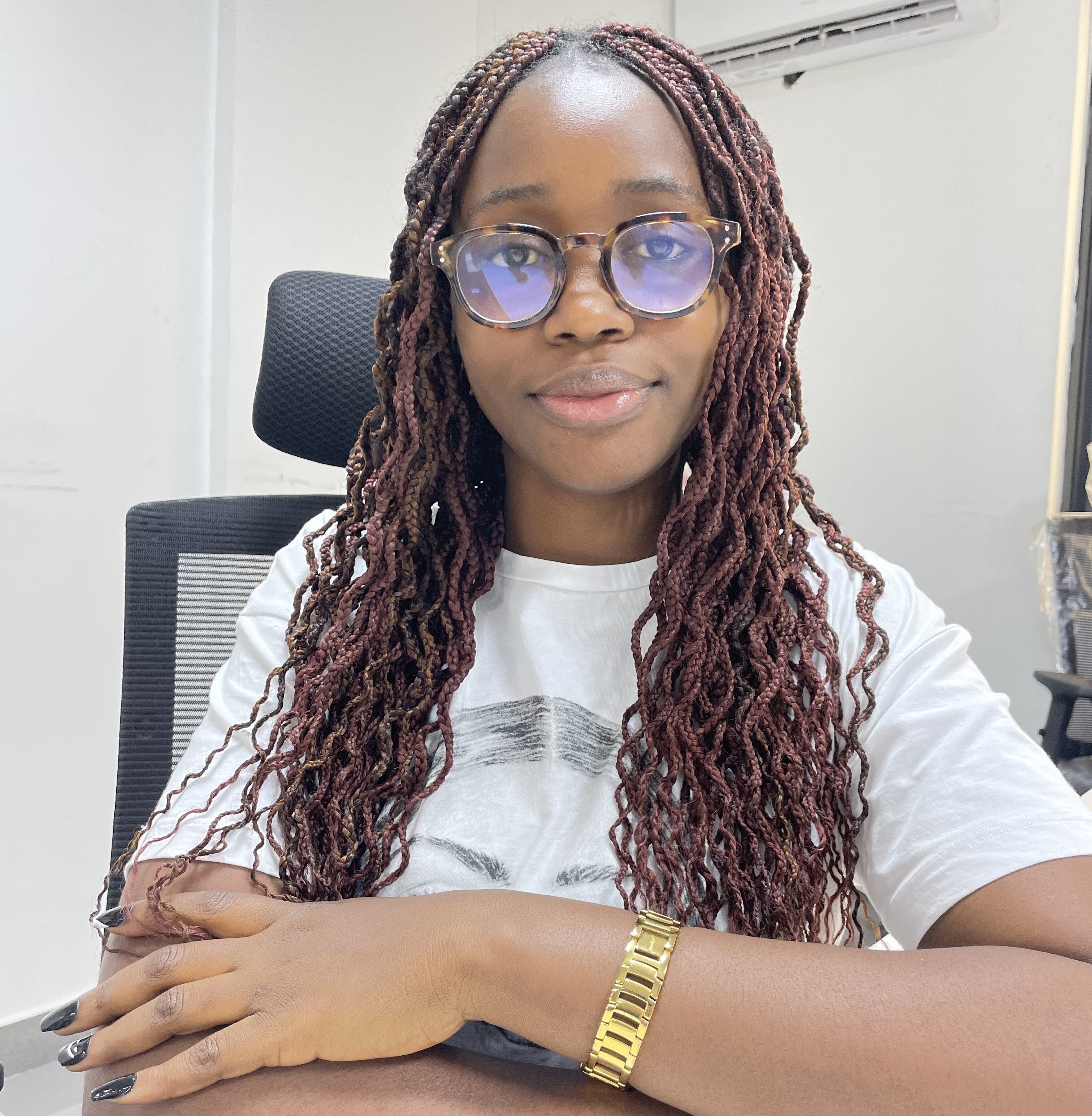
Jessica Agorye is a developer based in Lagos, Nigeria. A witty creative with a love for life, she is dedicated to sharing insights and inspiring others through her writing. With over 5 years of writing experience, she believes that content is king.
View all posts by Jessica Agorye