In the realm of web development, creating visually appealing and interactive experiences is paramount. One effective way to engage users is through the use of image galleries. By employing CSS, we can elevate these galleries to new heights, transforming them into dynamic and captivating elements on your website. In this comprehensive guide, we will delve into the art of crafting interactive image galleries using CSS, exploring the reasons behind their importance, the benefits they offer, and practical tips and methods to implement them.
Why Interactive Image Galleries Matter
Interactive image galleries are more than just a collection of pictures; they are a powerful tool to enhance user engagement and elevate the overall user experience. Here's why they matter:
1. Enhanced User Experience: Interactive elements, such as hover effects, transitions, and lightboxes, create a more immersive and enjoyable browsing experience for visitors.
2. Improved Visual Appeal: Well-designed image galleries can significantly enhance the aesthetic appeal of your website, making it more visually appealing and memorable.
3. Better Storytelling: By strategically organizing and presenting images, you can effectively convey a narrative or message, guiding users through a visual journey.
4. Increased User Engagement: Interactive elements encourage users to explore your content further, leading to longer website visits and increased conversions.
5. Mobile-Friendly Design: By optimizing your image galleries for mobile devices, you can ensure a seamless experience across various screen sizes.
Benefits of Using CSS for Interactive Image Galleries
CSS offers a versatile and efficient way to create stunning interactive image galleries. Here are some key benefits:
1. Cross-Browser Compatibility: CSS is widely supported by modern browsers, ensuring that your image galleries will work consistently across different platforms.
2. Performance Optimization: CSS-based solutions are generally lightweight and can significantly improve website performance, especially when compared to JavaScript-heavy approaches.
3. Customization Flexibility: CSS provides granular control over the styling and behavior of your image galleries, allowing you to create unique and tailored experiences.
4. Accessibility: By adhering to accessibility best practices, you can make your image galleries inclusive for users with disabilities.
Tips and Methods for Creating Interactive Image Galleries
Now that we understand the importance and benefits of interactive image galleries, let's explore practical tips and methods to create them using CSS:
1. Basic Image Gallery Structure
To create a basic image gallery, we'll use HTML to structure the images and CSS to style them.
HTML Structure:
<div class="image-gallery">
<img src="image1.jpg" alt="Image 1">
<img src="image2.jpg" alt="Image 2">
<img src="image3.jpg" alt="Image 3">
</div>
Explanation:
<div class="image-gallery">
: This is a container element that will hold all the images. Theclass="image-gallery"
attribute is used to target this element with CSS.<img src="image1.jpg" alt="Image 1">
: This is an image element. Thesrc
attribute specifies the path to the image file, and thealt
attribute provides alternative text for screen readers and browsers that can't display images.
CSS Styling:
.image-gallery {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
.image-gallery img {
width: calc(33.33% - 20px);
margin: 10px;
}
Explanation:
.image-gallery
: This CSS rule targets the image gallery container.display: flex;
: This sets the display property to flex, allowing us to arrange the images flexibly.flex-wrap: wrap;
: This ensures that the images wrap to the next line if they don't fit in a single row.justify-content: space-between;
: This distributes the images evenly within the container, with space between them.
.image-gallery img
: This CSS rule targets the images within the gallery container.width: calc(33.33% - 20px);
: This sets the width of each image to one-third of the container width, minus 20px of margin on both sides.margin: 10px;
: This adds a 10px margin around each image.
This basic structure provides a foundation for creating more complex image galleries with various interactive effects and layouts.
2. Hover Effects
Let's enhance our basic image gallery with some interactive hover effects. We'll use CSS to achieve these effects without relying on JavaScript.
Image Zoom:
When you hover over an image, it zooms in slightly.
CSS Styling:
.image-gallery img:hover {
transform: scale(1.1);
}
Explanation:
transform: scale(1.1);
: This CSS property scales the image to 110% of its original size when hovered over.
Image Gray Scale:
The image turns grayscale on hover.
CSS Styling:
.image-gallery img:hover {
filter: grayscale(100%);
}
Explanation:
filter: grayscale(100%);
: This CSS filter converts the image to grayscale when hovered over.
Image Border Animation:
A border appears and animates on hover.
CSS Styling:
.image-gallery img:hover {
border: 2px solid #007bff;
border-radius: 5px;
transition: border 0.3s ease-in-out;
}
Explanation:
border: 2px solid #007bff;
: This adds a 2-pixel solid border with the color #007bff.border-radius: 5px;
: This rounds the corners of the border.transition: border 0.3s ease-in-out;
: This creates a smooth transition effect for the border when the image is hovered over. Theease-in-out
timing function specifies the speed curve of the transition.
3. Image Transitions
Let's add some dynamic transitions to our image gallery. We'll use CSS to create smooth animations as images appear on the page. By combining these techniques and experimenting with different timing functions and delays, you can create a variety of engaging image transition effects to enhance your website's visual appeal.
Fade-in Effect:
Images fade in sequentially when the page loads.
CSS Styling:
.image-gallery img {
opacity: 0;
transition: opacity 0.5s ease-in-out;
}
.image-gallery img:nth-child(1) {
animation-delay: 0.2s;
}
.image-gallery img:nth-child(2) {
animation-delay: 0.4s;
}
/* ... and so on ... */
Explanation:
opacity: 0;
: Initially, the images are set to be completely transparent.transition:
opacity 0.5s ease-in-out;: This property specifies a smooth transition for theopacity
property over 0.5 seconds, using an ease-in-out timing function for a natural acceleration and deceleration.animation-delay: 0.2s;
: This delays the start of the transition for the specified image. By adjusting the delay for each image, we can create a staggered fade-in effect.
Slide-in Effect:
Images slide in from the left when the page loads.
CSS Styling:
.image-gallery img {
transform: translateX(-20px);
opacity: 0;
transition: transform 0.5s ease-in-out, opacity 0.5s ease-in-out;
}
.image-gallery img:hover {
transform: translateX(0);
opacity: 1;
}
Explanation:
transform: translateX(-20px);
: Initially, the images are positioned 20 pixels to the left of their final position.opacity: 0;
: Initially, the images are completely transparent.transition: transform 0.5s ease-in-out, opacity 0.5s ease-in-out;
: This specifies smooth transitions for both thetransform
andopacity
properties over 0.5 seconds, using an ease-in-out timing function.transform: translateX(0);
: When the image is hovered over, it slides to its original position.opacity: 1;
: The image becomes fully visible.
4. Image Scrolling
A scrolling image gallery is a dynamic visual element that allows users to scroll through a series of images horizontally or vertically. We can create this effect using CSS Grid or Flexbox.
CSS Grid Approach:
HTML Structure:
<div class="image-gallery">
<img src="image1.jpg" alt="Image 1">
<img src="image2.jpg" alt="Image 2">
<img src="image3.jpg" alt="Image 3">
</div>
CSS Styling:
.image-gallery {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
gap: 20px;
overflow-x: scroll;
scroll-snap-type: x mandatory;
}
.image-gallery img {
width: 100%;
height: auto;
}
Explanation:
display: grid;
: Sets the display property to grid.grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
: Creates a grid with columns that automatically adjust to fit the container, with a minimum width of 200px.gap: 20px;
: Sets the gap between grid items.overflow-x: scroll;
: Enables horizontal scrolling when the content exceeds the container width.scroll-snap-type: x mandatory;
: Makes the scrolling snap to the nearest grid item.
Flexbox Approach:
HTML Structure: (Same as the CSS Grid approach)
<div class="image-gallery">
<img src="image1.jpg" alt="Image 1">
<img src="image2.jpg" alt="Image 2">
<img src="image3.jpg" alt="Image 3">
</div>
CSS Styling:
.image-gallery {
display: flex;
overflow-x: scroll;
scroll-snap-type: x mandatory;
}
.image-gallery img {
flex: 0 0 auto;
margin: 0 20px;
}
Explanation:
display: flex;
: Sets the display property to flex.overflow-x: scroll;
: Enables horizontal scrolling when the content exceeds the container width.scroll-snap-type: x mandatory;
: Makes the scrolling snap to the nearest flex item.flex: 0 0 auto;
: Sets the flex properties to ensure each image maintains its original size and doesn't stretch.
Customization:
- Vertical Scrolling: Replace
overflow-x: scroll;
withoverflow-y: scroll;
. - Infinite Scrolling: Use JavaScript to dynamically load more images as the user scrolls.
- Custom Scrollbars: Use CSS to style the scrollbars.
- Transition Effects: Add CSS transitions to the images as they scroll into view.
Examples of Interactive CSS Image Gallery
Interactive Engagement in a Simple Image Gallery
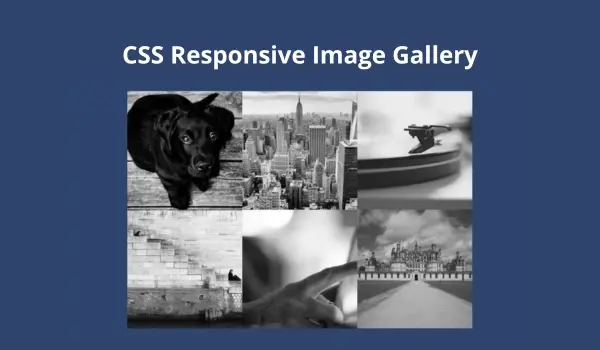
This responsive image gallery, built with just HTML and CSS by aledebarba on CodePen, showcases a clean and functional approach to interactivity. The gallery utilizes a grid layout to display images in a visually appealing arrangement that adapts seamlessly across various screen sizes. While the interactivity itself might be considered basic, it effectively enhances the user experience.
Upon hovering over an image, a subtle yet impactful grayscale effect is applied. This provides immediate visual feedback, indicating that the image is interactive and clickable. Clicking on an image triggers a smooth transition as it expands to fill the available space, revealing a larger version. Additionally, the image becomes linked, directing users to a potentially more detailed view of the image or a related webpage. This combination of hover effects and click-through functionality creates a sense of user control and exploration, making the gallery feel dynamic and engaging.
Embed this codepen >> https://codepen.io/aledebarba/pen/RwrNdOO
A Unique Approach to Image Display: Interactive Rhombus Gallery
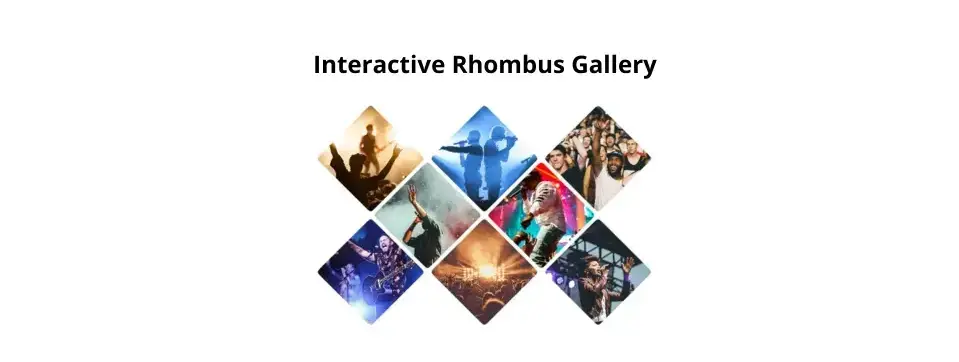
This CodePen example by alvaromontoro takes a captivating approach to showcasing images in a rhombus-shaped layout. The unconventional design and subtle hover effect spark curiosity and encourage users to explore the images within this visually intriguing gallery.
The rhombus shape deviates from the standard grid format, creating a unique and eye-catching display. Additionally, a subtle hover effect activates a slight opacity change, making the selected image stand out from the rest.
Embed this codepen >> https://codepen.io/alvaromontoro/pen/yLZVJRR
Interactive Scrolling Meets Image Gallery: A User-Friendly Experience
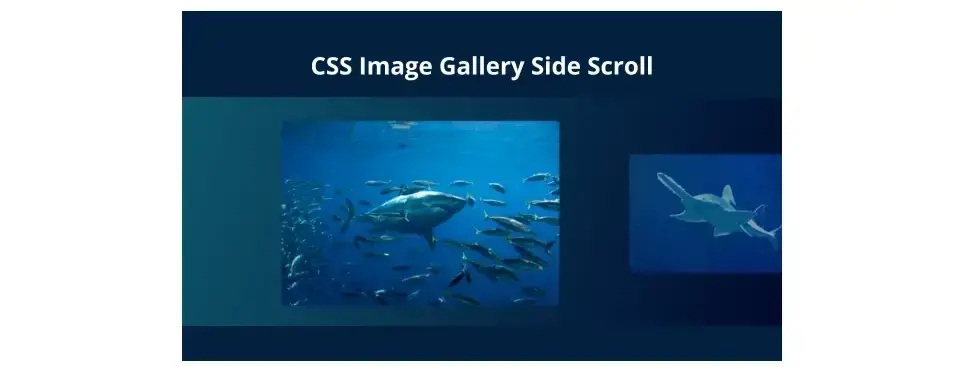
This CSS image gallery with side scroll functionality, created by christian-thorvik on CodePen, prioritizes user interaction for effortless exploration. The gallery utilizes a horizontal scroll, allowing users to effortlessly navigate through a collection of images, making it particularly suitable for showcasing large sets of pictures.
The interactivity goes beyond the scrolling mechanism. Hovering over an image triggers a subtle zoom effect, providing a closer look at the selected image without obstructing the overall view. Additionally, the gallery employs a "snap-to-scroll" feature, ensuring a smooth and controlled browsing experience. By seamlessly combining side-scrolling with hover zoom and snap-to-scroll functionality, this gallery prioritizes user control and fosters a pleasant browsing experience.
Embed this codepen >> https://codepen.io/christian-thorvik/pen/QWRqpgK
Conclusion
Our websites' overall user experience can be improved by using CSS to create aesthetically beautiful and dynamic image galleries. From basic hover effects to intricate animations and transitions, we may create a vast array of effects by comprehending the foundational ideas of CSS and experimenting with different approaches.
Aesthetics and utility must be balanced in order to create great image galleries. By putting accessibility and user experience first, we can make sure that our galleries are not only aesthetically pleasing but also easy to use.
Frequently Asked Questions
Can I host multiple websites?
Yes. Some packages even give you unlimited domains. However, the more you host, the slower your speeds will be.
Can gamification improve website loyalty?
Yes, gamification can contribute to improved website loyalty. By creating engaging and rewarding experiences, gamification encourages users to return to the website, participate in activities, and build a sense of loyalty. Loyalty programs, rewards, and achievements enhance the overall user experience and foster lasting connections.
Can Google host my website?
Yes, Google can host your website through services like Google Cloud Platform (GCP) and Google Sites. GCP offers scalable cloud hosting for various types of websites, while Google Sites is a simpler option for basic site creation and hosting.
Why should I create a website?
There are many reasons why you should create a website if you’re an artist. You can use it to create a place where people can learn about you, talk about your art, or show off your work.
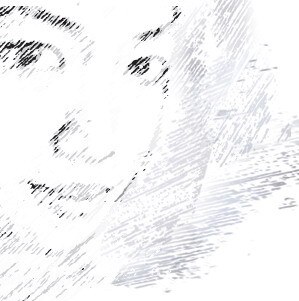
Audee Mirza is a graphic designer and WordPress developer at audeemirza.com who resides in Surabaya, Indonesia. She's also the author of Graphic Identity Blog, a professional logo designer, and often creates vector illustrations for clients and marketplaces. She enjoys good typography design and all kinds of animation.
View all posts by Audee Mirza