In a previous article, we made a lot of cool and fancy effects for images. We considered modern CSS tricks and only the <img>
tag to reach for stunning results. In this article, we will do the same!
We will create even more effects with the same requirements: Only the <img>
and a few lines of CSS. No extra element and no pseudo-element.
Overlay Effect
Previously, we did an overlay effect where we have a semi-transparent layer above the image that disappears on hover.
We are going to improve this effect by adding some radius to the image and a circular reveal effect on hover.
Cool right? I am using a solid color this time to hide the image but you can also use a semi-transparent color like the previous demo.
The effect relies on two tricks. First I am going to rely on the outline
property to create the overlay and then animate the outline-offset
to make it disappear:
img {
--s: 300px; /* size of the image*/
width: var(--s);
aspect-ratio: 1;
border-radius: 50%;
outline: var(--s) solid #FFC48C;
outline-offset: calc(var(--s)/-2);
}
img:hover {
outline-offset: 0;
}
Note how I am using border-radius: 50%
to create a rounded image which is also what will make the outline rounded and get the circular animation on hover.
It looks a bit ugly because the outline is too big but don’t worry, it will get fixed with the second trick when we will introduce clip-path
.
Using clip-path
allows us to hide the non-need outline and at the same time define the radius of the image. Don’t forget that the main idea is to have an image with a specific radius and not a rounded image.
clip-path: inset(14.65% round 20px);
Here is a step-by-step illustration to understand what the clip-path
is doing
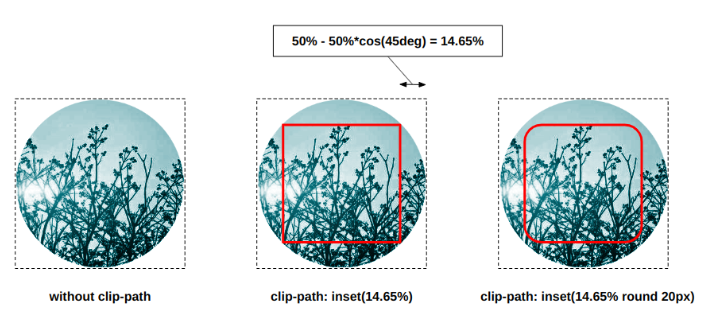
We start with the rounded image without clip-path
(the dotted box illustrates the area of the image). Then we apply the clip-path
in a way to remove the rounded part. In other words, we are going to extract the inscribed square from the image. We will only see what is inside the red square. Finally, we consider the round
option of clip-path
to round the clipped area and get the radius we want.
The use of clip-path
will also hide the outline outside the image and our effect is perfect. The only drawback of this effect is that we are going to hide some parts of the image so it may not be suitable for all the images.
Overlapping Frame
Let’s move to some fancy frames. In the previous article, we added a frame behind the image that moves on hover to create a full border around it.
This time we are going to make the frame above the image and create a slightly different animation:
We don’t need an extra element or a pseudo-element to create that frame. An outline trick combined with padding can do the job.
img {
--c: #F9CDAD; /* the main color */
--b: 8px; /* thickness of the border */
--o: 25px; /* control the offset */
width: 200px; /* the image size */
--_p: calc(2*var(--o) + var(--b));
padding: var(--_p) var(--_p) 0 0;
outline: var(--b) solid var(--c);
outline-offset: calc(var(--o) - var(--_p));
}
img:hover {
padding: calc(var(--_p)/2);
}
The code may look complex but if we add a background coloration the trick will be easier to understand:
We create an outline with a negative offset which will add that frame above the image in the middle. The padding is creating the illusion of an offset frame but with the background coloration, we can notice that the frame is always in the center of the image area.
Then, by adjusting the padding, we create the moving animation while keeping the frame fixed. The total padding remains the same so the overall size of the image won’t change and the frame won’t move.
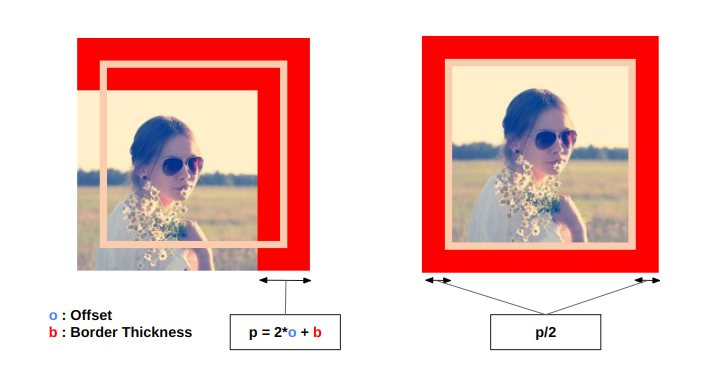
Initially, we define padding on two sides only (the left figure) then on hover we define half the padding on all the sides (the right figure).
This configuration allows us to have 4 variations for the same effect. I already show 2 of them so I let you try the others by adjusting the padding configuration.
Corner-only borders
What about adding corner-only borders to your image? Yes, it’s possible and you don’t need any extra element to do it. Only a few lines of CSS are needed and we can also get a nice animation on hover.
First, we will add an outline. Yes, outline again. This underrated property is powerful and allows us to create a lot of fancy decorations.
img {
--t: 5px; /* the thickness of the border */
--g: 20px; /* the gap between the border and image */
padding: calc(var(--g) + var(--t));
outline: var(--t) solid #B38184; /* the color here */
outline-offset: calc(-1*var(--t));
}
img:hover {
outline-offset: calc(var(--g)/-1);
}
Nothing complex so far. We are animating the offset of the outline on hover to get the following result:
I am adding a background coloration to illustrate the area of the image. You can see that the outline is within that area which is important for the next step where will introduce the mask property. The idea is to hide parts of the image area to keep only the corner visible (and the image itself).
I will be using two gradients to achieve this:
img {
--s: 50px;
mask:
conic-gradient(at var(--s) var(--s),#0000 75%,#000 0)
0 0/calc(100% - var(--s)) calc(100% - var(--s)),
linear-gradient(#000 0 0) content-box;
}
The linear-gradient
covers only the content area (thanks to content-box). This will make sure the image is visible. The conic-gradient
will cover the corners. Here is an illustration to better see each gradient:
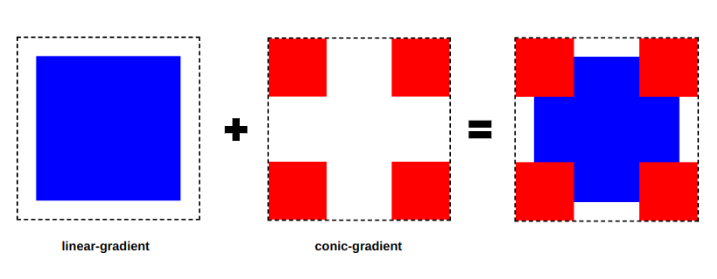
The red and blue areas are the visible part of the image. The outline will move within the red area and the illusion is perfect! We have corner-only borders:
img {
--s: 50px; /* the size on the corner */
--t: 5px; /* the thickness of the border */
--g: 20px; /* the gap between the border and image */
padding: calc(var(--g) + var(--t));
outline: var(--t) solid #B38184; /* the color here */
outline-offset: calc(-1*var(--t));
-webkit-mask:
conic-gradient(at var(--s) var(--s),#0000 75%,#000 0)
0 0/calc(100% - var(--s)) calc(100% - var(--s)),
linear-gradient(#000 0 0) content-box;
transition: .4s;
cursor: pointer;
}
img:hover {
outline-offset: calc(var(--g)/-1);
}
If you are not familiar with gradients, I highly recommend reading one of my previous articles
where I am detailing how to use conic-gradient
to create different CSS patterns.
Zoom Effect
I will end this article with a simple zoom effect on hover.
Such an effect is pretty easy. Only two properties are required.
img {
--f: 1.15; /* the scale factor */
clip-path: inset(0);
}
img:hover {
clip-path: inset(calc((1 - 1/var(--f)) * 50%));
scale: var(--f)
}
We apply a scale transformation which will make the image bigger. Then we will consider clip-path
to trim the image and make it looks like the image is growing within a fixed area.
Here is a demo to illustrate the trick. Hover the screen to see the effect:
The first image grows, the second one is trimmed and the third one combines both effects to get our zoom effect.
If you want more detail about the calculation, I am doing inside the clip-path
check my article: How to make a zoom effect using CSS. I am using the same technique to create a similar zoom effect on images.
Conclusion
I hope you enjoyed this second part where we created cool effects and decorations using only the <img>
tag. Don’t forget to check the first part and stay tuned for a third one. Yes, we can still make even more fancy effects!
Until then, here are a few articles if you want to explore more CSS tricks around images:
Frequently Asked Questions
Will removing unused images affect running containers?
No, removing unused images does not impact running containers. Containers are based on the images that were used to create them. Removing images only affects containers created from those images if they were stopped and not in use.
Can free HTML editors support other languages like CSS and JavaScript?
Yes, free HTML editors often support languages like CSS and JavaScript, enabling integrated web development.
What is the impact of server location on CSS hosting performance?
Server location significantly affects the loading speed of CSS files. Hosting your CSS closer to your user base ensures faster download times, reducing website latency and improving user experience.
How does CSS hosting handle scalability for high-traffic websites?
CSS hosting typically employs scalable infrastructure, like cloud hosting, which can dynamically allocate resources to handle increased traffic. This ensures your site remains fast and reliable, even during traffic surges.
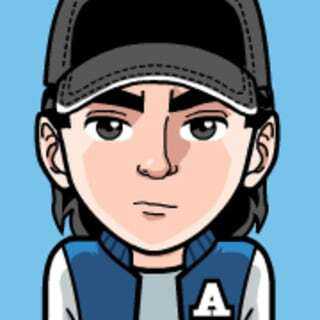
Temani Afif is an expert web developer, a content creator, and a CSS addict. He is the mastermind behind CSS Loaders, CSS Generators, CSS Tip and a lot of CSS stuff.
View all posts by Temani Afif