Programming languages are an integral part of software development. A programming language is a formal language used to create software programs that communicate or instruct computers.
There are different languages commonly used by developers, such as Python, Java, C#, C++, JavaScript, TypeScript, Ruby, Swift, Go, etc.
In this article, we’ll highlight two programming or coding languages named JavaScript and Typescript. JavaScript programming language was released in 1995, which means it’s been in the game for a while. TypeScript, on the other hand, is relatively new and is now being adopted by many developers.
Let’s get started!
Type Checking in Programming Languages
Programming languages fall into two categories, and they can be either dynamic or static. JavaScript and Typescript are closely related. However, JavaScript is considered a dynamic language while Typescript is a static language.
Programming languages apply a method of checking assigned values in a variable called “type checking”. Type checking verifies and enforces constraints of types in values and ensures that the code or program follows the constraints of the programming language.
The “type of “operator is used in JavaScript for type checking. For instance, let’s use “typeof” to check what “type” of value is stored in the name variable.
let name = "Verpex"
console.log (name, typeof name)
Verpex string // response

Key Point:
The categories of type checking used by programming languages are static or dynamic. This is why we often hear phrases like “JavaScript is dynamically typed or TypeScript is statically typed”.
What is a Dynamic Language?
A dynamic programming language involves a type system where variables are not explicitly declared with their types, and type checking is performed during runtime.
This means that variables are checked during program or code execution. Types of dynamically typed languages include; python, JavaScript, Ruby, etc.
For example, let’s create a variable that stores a number
let x = 2 , and try to convert the value 2 to a string
x=String(x) console.log (x)

The String function will convert the value to a string, and then update the “x” variable with the new value.
Advantages and Disadvantages of Dynamically typed language
Advantages
Disadvantages
What is a Static Language?
Static programming involves a type system where variables are declared explicitly with their types, and type checking occurs when the code is compiled. When we create a variable, we cannot change the “type “of the variable after it is declared unless we adjust the typing rule. TypeScript follows an optional-statically typed method that allows developers to type explicitly or rely on type inference.
Variable types are determined and checked before the program or code is executed, and there are different types of statically typed languages, including C, C++, C#, Java, etc.
If we create a variable using a static language like Java or TypeScript and define the “value type” as a string or a number, the variable is typed to that specific value.
In other words, the variable is associated with the value and can only hold the value of the same type. For example, let’s create a variable that stores a number.
let x = 2 , and try to convert 2 to a string
x=String(x) console.log (x)

This returned an error because we can only change the value of x to a number and not a string.
Advantages and Disadvantages of Statically Typed Languages
Advantages
Disadvantages
What is JavaScript?
JavaScript, an interpreted programming language, is one of the most used programming or scripting languages used to build dynamic features on web pages, applications, servers, or games.
It is famous not just cause of its lightweight nature, ease of learning, and flexibility but also because most browsers use it as the scripting language for performing interactive activities (e.g. animated graphics) on web pages to improve user experience.
JavaScript typically works hand-in-hand with HTML and CSS, which are the foundation of web development. It can be used to develop the frontend, and backend of an application, mobile apps, and desktop applications.
There are different JavaScript versions or standards of JavaScript examples including ECMAScript 5(ES 5), ECMAScript 6(ES 6), and these standards set features, syntax upgrades, etc. for JavaScript.
Significance of JavaScript
- Versatile in nature
- Easy to learn
- Converts static web pages to interactive pages
- Considerable resources and community support
These are just a few benefits of using JavaScript.
What is TypeScript?
According to the official TypeScript page, TypeScript is JavaScript with syntax for types. It is a strongly typed programming language that builds on JavaScript, providing better tooling at any scale. It was introduced in 2012 by Microsoft, and It is commonly referred to as a sub-set of JavaScript and can be stated as a stricter version of JavaScript.
TypeScript code is transpiled into native JavaScript using the transcript compiler. It’s safe to say that it goes through compilation to exist or function in a JavaScript environment. E.g. web browser, node.js, etc.
Significance of TypeScript
- Type enforcement
- Easy to learn if you already know JavaScript
- Adds static typing to JavaScript
- Error catching occurs earlier instead of debugging at runtime. This means that before the code or program is executed, you must fix the error if you want the code to run.
- Interchangeable with JavaScript- means it can be transpired into native code and vice-versa.
- Great for enterprise or any large-scale project.
The limitations of JavaScript led to the creation of TypeScript. For example, JavaScript has a flexible data type rule, and this is also the exact reason why JavaScript code may produce incorrect code.
Example:
Let's write a function to calculate the sum of two numbers;
function sum (a , b){
return a + b
}
console.log (sum (10, “10”))
When we call the sum function, you’ll notice that there’s a string and a number instead of two numbers.
JavaScript will do a type conversion and add the number and string together, which means that JavaScript will convert the number 10 to a string. This occurs when you add a string to a number using the + operator. JavaScript concatenates the number to the string. Instead of 20, we got a string “1010”
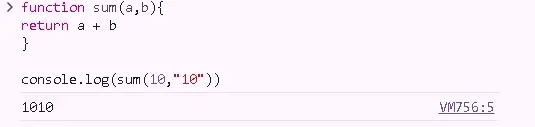
Now, we’ll perform this same function in typescript, and we’ll see the difference in the outcome shown in the image below.

The error here says parameter “a” implicitly has an “any” type - This error occurred because I didn’t specify any type. Now I'll add a “Number” type to both parameters

Now, the error tells me that the argument of type “string” is not assignable to the parameter of type number, which simply means I cannot assign a string when my parameter is assigned the type number.
Now I can spot the error in my code and fix it immediately.
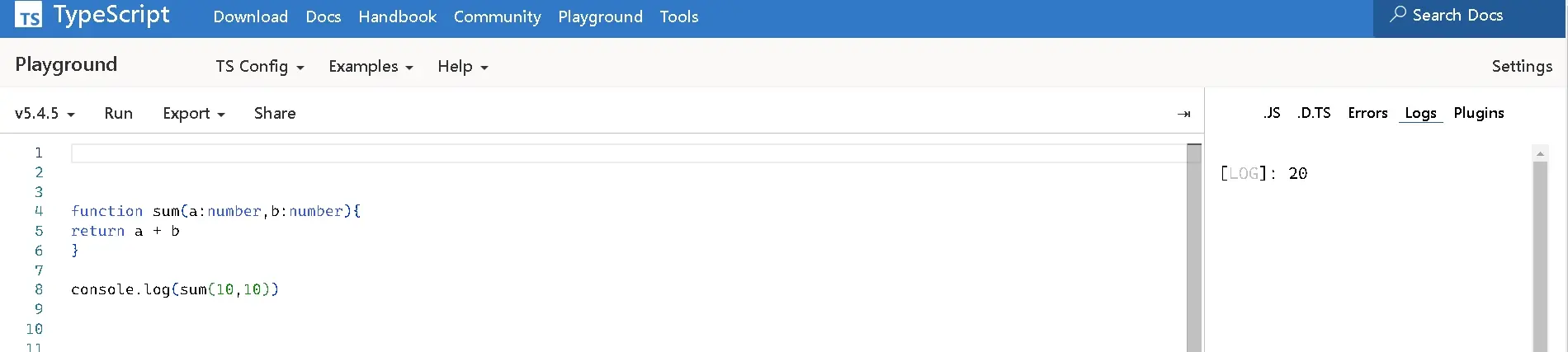
Example 2:
Let's write the same function in JavaScript, but this time, omit an argument. In the JavaScript console, we’ll get NAN - Not a Number. However, this error won’t be displayed when we write the code, but after we run the code.
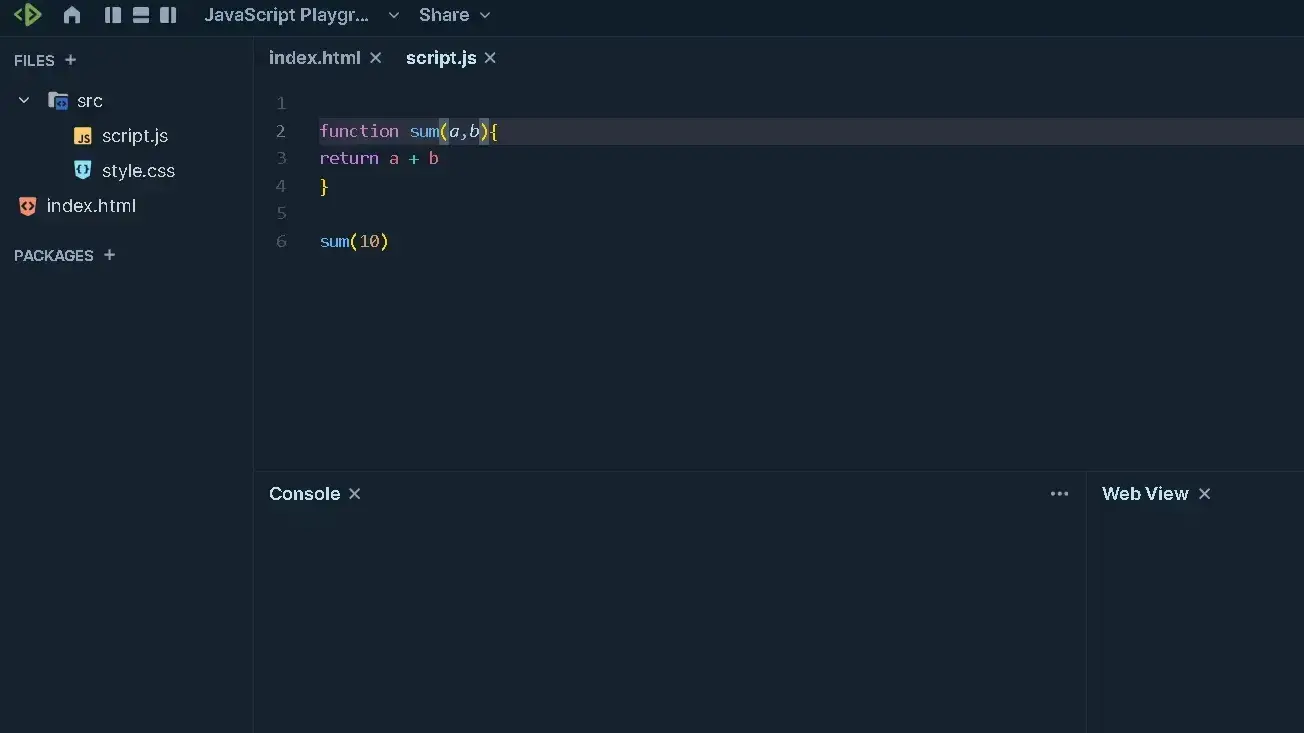
After the code runs,
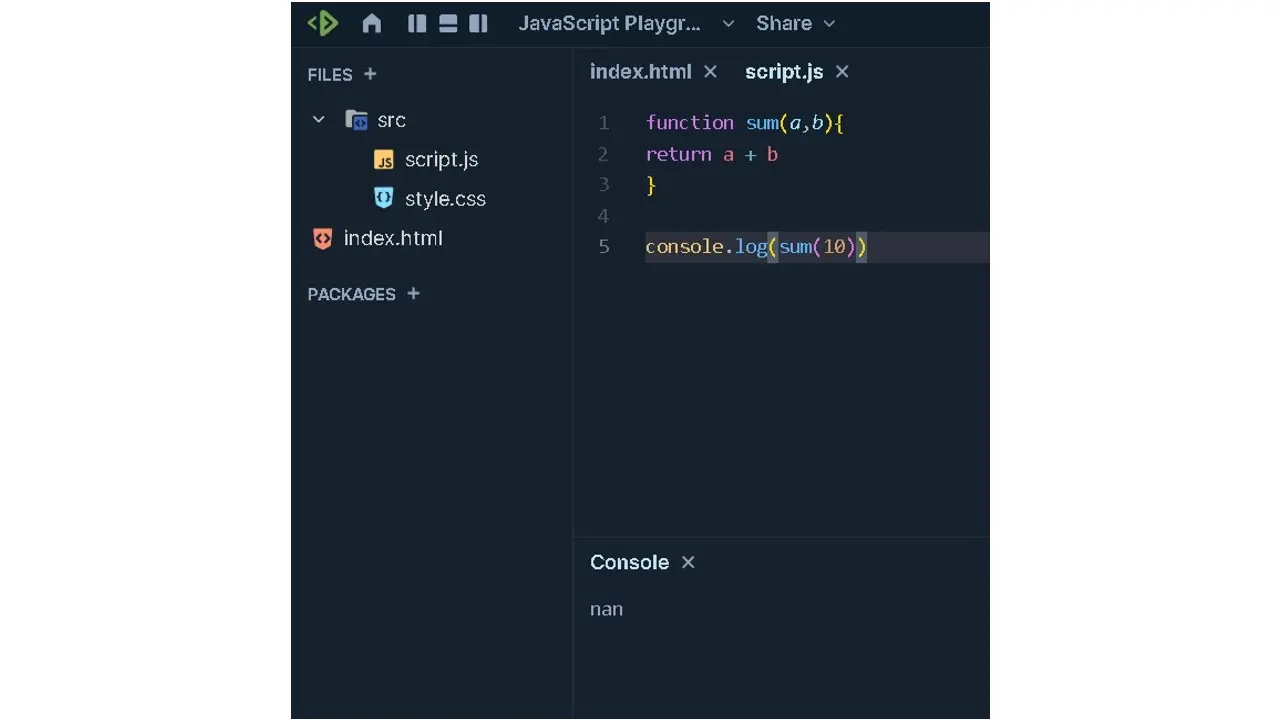
since we didn’t provide b with a value, it’s assigned the value “undefined”. When the number 10 is added to “undefined” it becomes NaN.
We’ll write the same function in the TypeScript playground as well. An error message is displayed, which indicates that we are missing one argument - this occurs before we run the code.
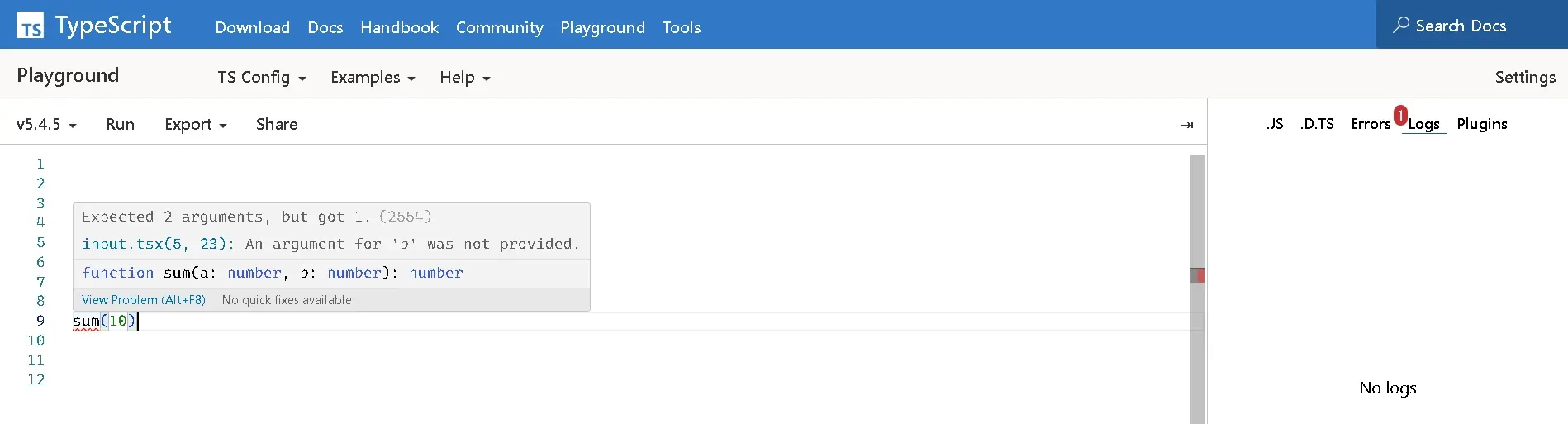
These are just basic examples to differentiate between the two; however, it’s more complex when working with on projects.
TypeScript VS JavaScript
JavaScript
TypeScript
Static or optional static typing - you can determine
Fixing errors is much easier because it can be seen during design time or compile time.
Object-oriented language
The steep learning curve for Beginners. It is easier for individuals who already understand JavaScript.
Great for enterprise or any large-scale project
Limited tools, libraries, and frameworks.
TypeScript is a compiled language
Requires more code - sometimes considered to be verbose
Summary
JavaScript and TypeScript have advantages and disadvantages.As a beginner, JavaScript is much easier to learn as it doesn’t follow strict data type rules. Starting with TypeScript as a beginner is a great choice. The reason is that many developers who learned JavaScript have to learn TypeScript, as many companies require it.
In summary, TypeScript is still JavaScript except for the strict typing rules, which means that JavaScript is valid TypeScript code. Choosing one or the other depends on your need. However, it is advised to understand TypeScript as it is fast becoming essential for developers.
if you're working on JavaScript projects, our JavaScript Hosting is here to provide reliable support and performance. Explore our hosting options to find the perfect fit for your project’s needs.
Frequently Asked Questions
How does Verpex Hosting handle databases for JavaScript hosting?
We support both SQL (like MySQL, PostgreSQL) and NoSQL (such as MongoDB) databases for JavaScript hosting, ensuring seamless integration and management for Node.js applications.
Does Verpex Hosting support automatic scaling for JavaScript hosting?
Yes, we offer auto-scaling capabilities for JavaScript hosting, particularly in its cloud-based hosting solutions. This feature allows hosting resources to dynamically adjust in response to your application's demand, ensuring efficient performance during periods of high traffic.
Can free HTML editors support other languages like CSS and JavaScript?
Yes, free HTML editors often support languages like CSS and JavaScript, enabling integrated web development.
Can I use Astro.js with other JavaScript libraries and frameworks?
Yes, Astro.js is designed to work with various frontend frameworks and libraries, allowing you to leverage your preferred tools alongside its performance optimizations.
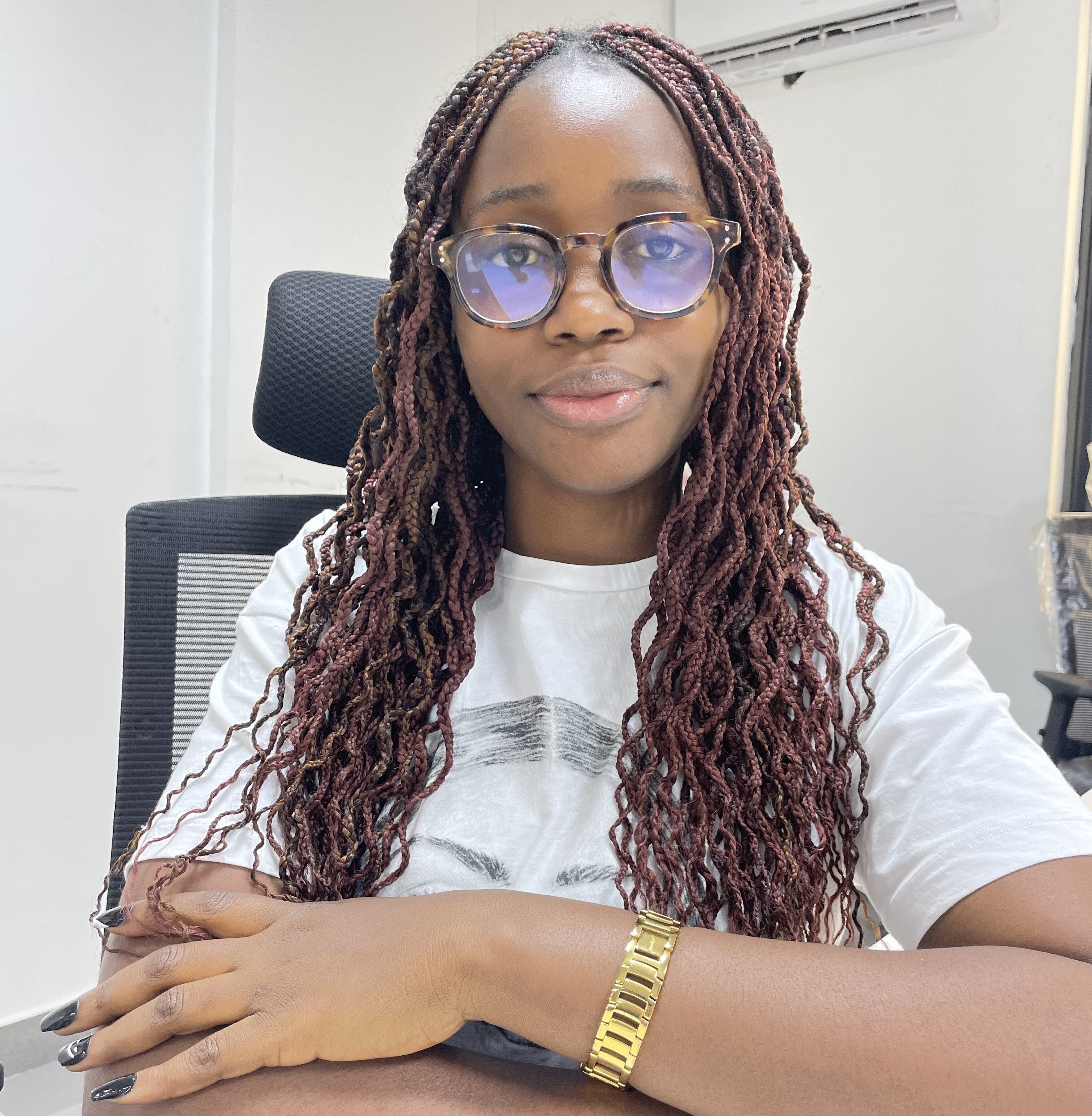
Jessica Agorye is a developer based in Lagos, Nigeria. A witty creative with a love for life, she is dedicated to sharing insights and inspiring others through her writing. With over 5 years of writing experience, she believes that content is king.
View all posts by Jessica Agorye