What makes an API effective? Is it its performance, documentation, or developer experience? In this article, I will explain the difference between GraphQL and REST API, two of the most popular request-response APIs. We will compare them to see what makes them different, but let's start by defining an API first.
What is an API?
An API (Application Programming Interface) is a set of rules and protocols that defines how computers communicate with each other.
By using APIs, different applications can access the functionality of a software library or expose data and services for developers or businesses to use. The application that accesses the resource is referred to as the client, and the application, which contains the resources, is called the server. Now that we have defined APIs, let's explore them in more detail.
What is REST?
REST stands for Representational State Transfer, an architectural pattern for requesting and retrieving data over HTTP. REST is a set of rules for building web applications or an architecture style used to design network applications.
Web services are made such that resources are stored on a server in different states. When there's a request by a user, the information received by the user represents the state of the resource, not how the resource is stored on the server.
The representation of the State, which is the resource data, can be in different formats, either in JSON, HTML or XML, etc. JSON is used the most for sending and receiving data because it’s easy to understand.
REST API helps client-server communication by sending and retrieving responses, which also means it conforms to REST constraints. It is stateless and concedes separation between a client and server, or we may say it relies on the client-server protocol(mostly HTTP).
In REST architecture, clients and servers are independent because they are not concerned with each other’s internal parts. On the condition that the interface is the same, they can go through changes independently without affecting each other, which makes it flexible, scalable, and reliable.
If you’ve heard REST and RESTful in the same sentence and are wondering what the difference is, they are the same. A RESTful API follows a set of rules known as the Representational State Transfer (REST), which has been the standard for API development since the 2000s. In other words, REST is the set of rules, while RESTful refers to the API complying with the set of rules.
RESTful API organizes resources into a set of unique URI known as Uniques Resources Identifiers to separate the different types of requests on the server or tell the server what to do with the requests. It comprises four parts, often referred to as Anatomy. Here are some of the components of the REST anatomy:
Endpoint: The endpoint consists of the URI (Unique Resource Identifier), which tells us how and where to find resources on the server. For a client to access the resource, an Identifier is needed. Assuming we’re using an HTTP Protocol, the identifier of our resource would look like this; http://www.example.com/user/1 - In this identifier, we have the domain name, the user, and the ID.
Methods: If a client requests certain data to an endpoint via HTTP, the request follows specific methods or HTTP VERB. The HTTP VERB specifies the action that needs to be performed to access or communicate with the resource, and these methods include;
POST - A POST request means we want to create a new resource. When we fill out a form on the web by entering our name, password, DOB, etc. It also means we’re sending data to the server.
GET - GET means we want to read the data of an existing result. We make GET requests whenever we enter a URL in our web browser to retrieve data from a specified resource.
PUT - PUT means to update an existing resource.
DELETE - This means to delete or remove existing resources.
Headers: Headers provide information between the client and server. They are also used for authentication purposes.
Data: The data or body is used to send information to the server. It tells the server when you want to add, replace or delete data.
There are many HTTP verb methods. However, those mentioned above are frequently used.
REST API Design Principle
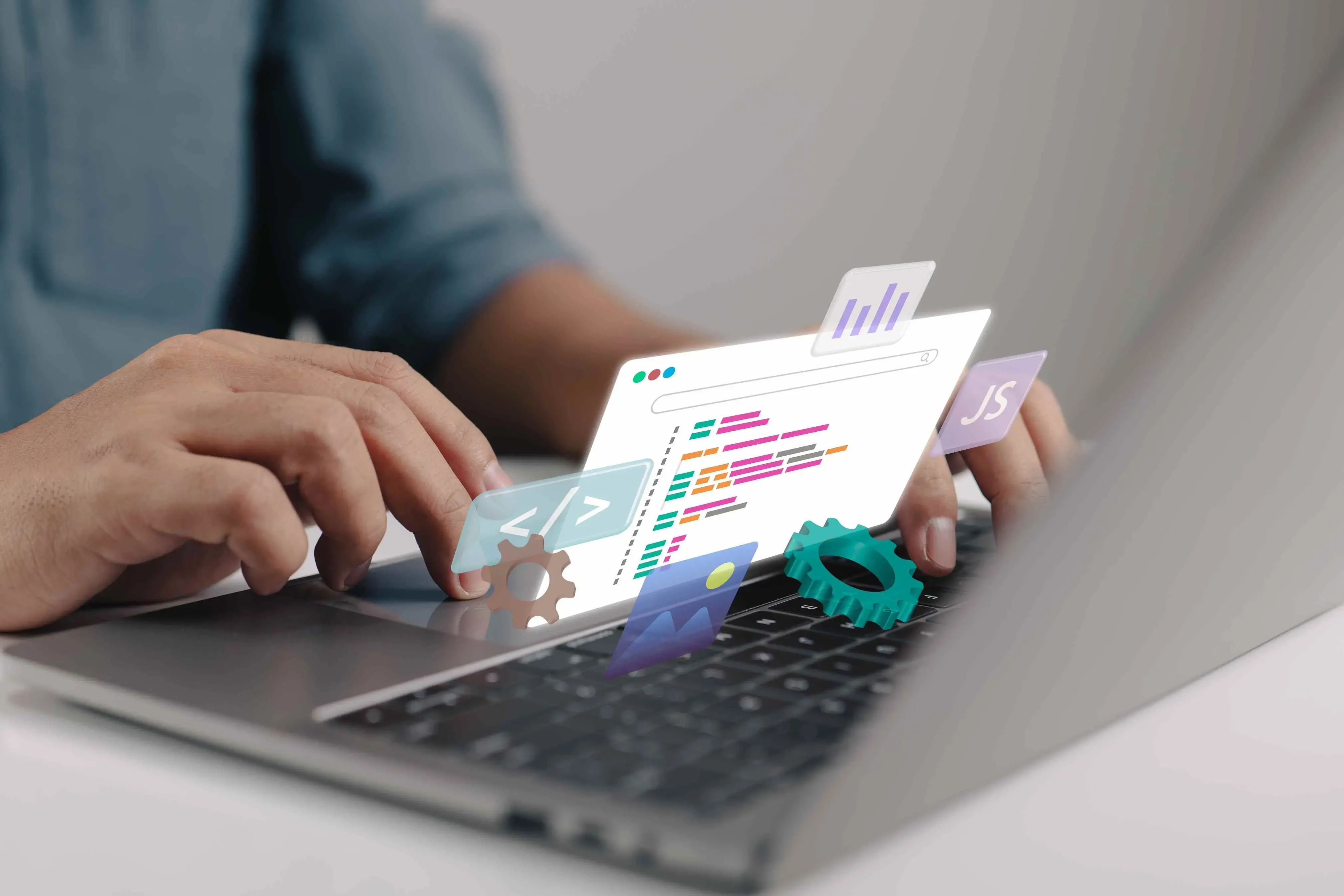
The following six REST design principles must be followed by an API to be considered RESTFUL:
Client-Server Architecture: This implies that the server is not selective of the type of client serves, either mobile, tablet, or desktop. For instance, if we want to play a video from a website, the client will communicate with the website server to play the video, irrespective of the type of device.
Statelessness: When the client sends an HTTP request to the server, the server doesn’t store any information about the request. For the server to understand the request, the client must send every bit of data needed by the server to provide a corresponding response.
Cacheability: Resources shared by the client and server must be cacheable, implying that the resources shared between the client and server are stored in memory for easy retrieval, which helps improve the efficiency of data retrieval.
Layered Architecture: The client and server do not communicate directly. An intermediary server is a means through which communication is handled, and this supports load balancing.
Code On-Demand: Code-on-demand is the ability for the server to send code to the client as part of the API response to perform an action or additional functions. In other words, if a client requests to get the list of novels from the server, the server can also send additional code to allow the client to filter the list of novels.
Uniform Interface: The uniform interface constraint is defined by the ability of the client and server to communicate over a network using standard HTTP methods(e.g., GET, POST, PUT, DELETE). Rest Uniform Interface constraints must be followed for a system to be considered “RESTFul.”
Limitations of REST APIs
Over-fetching data: Due to the numerous endpoints that return fixed data, REST is prone to over-fetching data. For example, suppose we want to get only a user's name in a resource. In that case, The API will return all the user entities or the entire resource, including other attributes about the user. In this case, we get a lot of unnecessary data that we don't need.
Data under-fetching issues: Data under-fetching is less common than data over-fetching. Clients may be required to include additional requests or specify the identifier of the particular entity when making requests to endpoints.
Interaction between client and server is not saved: Because of its statelessness, there’s a separation of concern between client and server. This means that information is not stored, and the transaction status does not persist. Imagine going to the coffee shop and making the same order daily. It’s logical for the barrister to always give you the same order because this individual already has your order in memory. In the case of REST, you’ll always have to make the same request, even if you’re a regular.
Multiple HTTP Roundtrips: You'll have to make separate calls if you want to get a resource and a sub-resource. You have to get to the resource before accessing the sub-resource. Neither request can be combined simultaneously.
What is GraphQL?
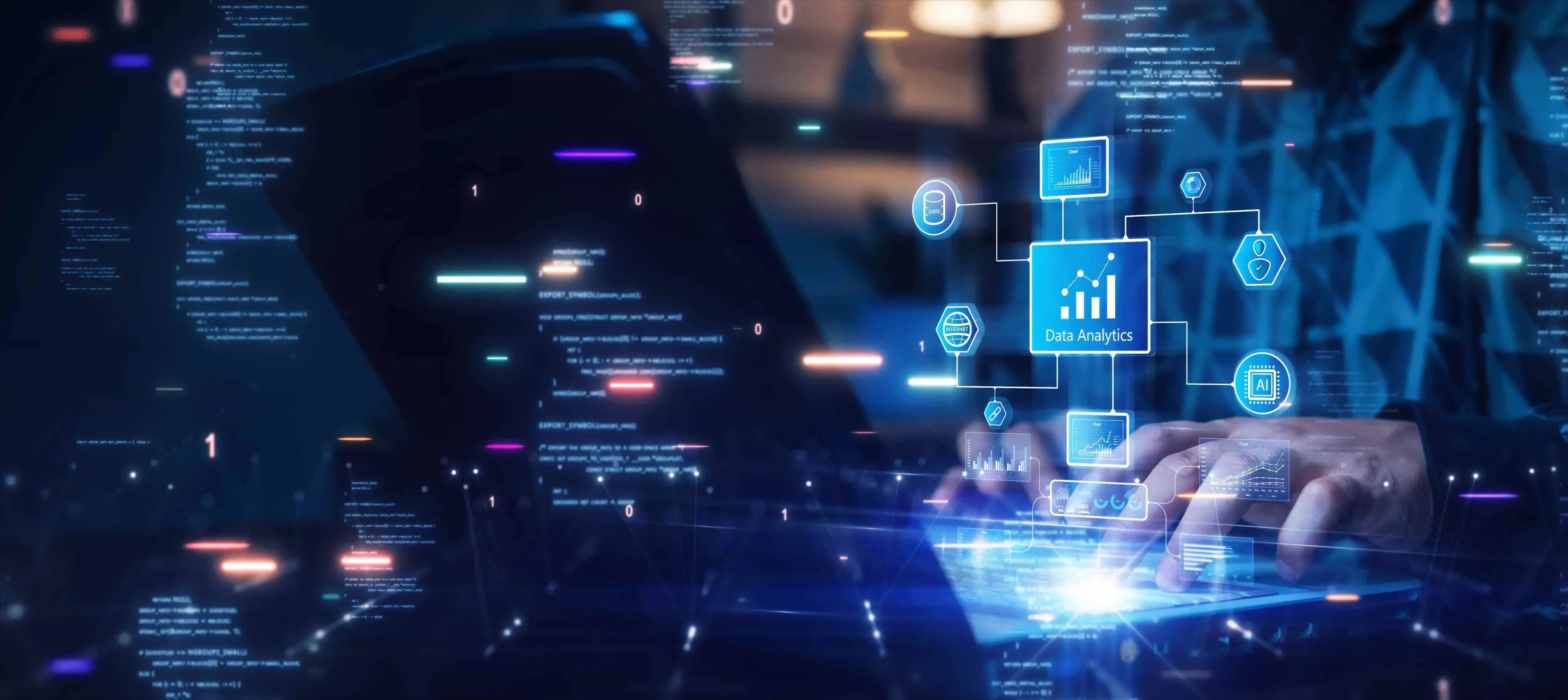
GraphQL is a query language for your APIs. It is used for querying and modifying data and was developed as an alternative to REST APIs.
GraphQL offers a system where you can specify the exact data you need, allowing flexibility for querying data more efficiently than REST APIs, where you will be required to create a fixed set of endpoints for different data types. These endpoints will often return more data than the client needs.
The GraphQL protocol was developed by Meta, formerly Facebook, when it introduced the Facebook mobile app in 2012. The inspiration came from a persistent issue they encountered with building REST APIs at scale. At the time, they realized that the mobile app ecosystem was evolving, and they needed to build a system that caters to this change. The team realized that REST APIs aren’t as flexible and often require multiple requests to different endpoints to deliver data to their users. This led to the creation of GraphQL, which allows querying data to be more flexible and efficient.
In GraphQL, a concept called Schema defines what type of data can be queried and the operations that can be performed. The schema serves as a contract between the client and the server and defines the shape of the data that the client can request. Schemas are written in GraphQL’s SDL(Schema Definition Language) format. Let’s see an example of a GraphQL Schema:
type Book {
title: String!
author: String!
}
The schema above defines a Book type with two fields title and author. They both have required fields, which are indicated with the (!) symbol. To write a query for this schema in GraphQL, we can do so using the syntax:
{
book(title: "Half of a Yellow Sun") {
title
author
}
}
This query returns a JSON response that includes the data associated with the keys: Author and Title.
{
"book": {
"Author": "Chimamada Adichie",
"Title": "Half of a Yellow Sun"
}
}
Advantages and Disadvantages of GraphQL
Advantages
Disadvantages
Advantages of GraphQL
More efficient data querying: GraphQL schema allows clients to ask for exactly what they need. Furthermore, users can query for multiple resources at once using a single endpoint.
Improved Performance: The capability to request data in a form that's required improves performance when working with complex data structures. This reduces the workload on the server, eliminating the need to make repetitive requests.
Autogenerated Documentation: GraphQL keeps the documentation up-to-date as the API changes. Whenever a field or query is updated in the codebase, it automatically reflects in the documentation, making it easier to work with. When new changes are introduced, the developers spend less time updating the documentation.
Strongly Typed System: GraphQL is a strongly typed language. Each field has a type against which it will be validated. When you make a GraphQL query, it will only return the expected data type. For example, the query should also return a string if a field has a string type. This strongly typed system ensures the data returned is reliable and reduces the chances of errors in the API.
Improved Developer Experience: GraphQL data structure is precise, making it easier for developers to work with. In addition, it has abundant tools and libraries that can help developers build, test, and debug their applications.
Disadvantages of GraphQL
No Built-In Support for Caching: GraphQL doesn't have a built-in cache capability, so developers have to implement caching strategies to optimize API performance.
Complex Query: The flexibility of GraphQL can be both an advantage and a disadvantage. Performing complex queries can be time-consuming and labor-intensive, resulting in poor performance
REST VS. GRAPHQL
There are some similarities between GraphQL and REST, as well as some differences. Let’s look into some differences:
REST | GRAPHQL |
---|---|
REST is an architectural concept used to develop new APIs for network-based software. | Data in GraphQL is organized into a graph and follows a Schema structure with nodes structure objects. |
REST has over-fetching, and under-fetching issues, which is getting too much or too little data, leading to a response with redundant data. | In GraphQL, clients can request only the data they need, solving the issue of over-fetching and under-fetching. |
In REST, several endpoints are called to fetch resources. | Resources can be fetched from a single request. Fetching data in GraphQL is achieved by queries. This allows you to obtain specific data from the GraphQL server. |
REST implements caching during its operations. | Automatic Caching is unavailable in GraphQL |
Debugging in REST is easy and can be achieved using HTTP Status Codes. | Identifying errors in GraphQL is more complex. |
Different API versions are supported by REST | GraphQL does not support API versions. |
REST supports various formats to represent data. (JSON, XML, etc.) | JSON is the only way to represent data in GraphQL. |
To ensure security, REST APIs use API authentication methods like HTTP authentication. | GraphQL integrates data validation. However, users are in charge of ensuring authorization and authentication measures. |
One function communicates with one request. | One request activates many functions. |
REST assigns a URL to every data resource to make a request or retrieve a resource. The APIs send a request to the address, it is processed by REST, and then a JSON document is returned. | GraphQL works with a defined object and a query which is a means to retrieve information. |
Wrapping Up
Choosing between REST and GraphQL depends on the type of software you’re building. They both have limitations and advantages through which you can determine how they may affect your work. If you’re working on software that fetches specific data, GraphQL is a great option, but if your software is for general data, then REST is the better option.
Frequently Asked Questions
Can I easily integrate third-party tools and APIs with Verpex Web Development Hosting?
Yes, Verpex Web Development Hosting allows for easy integration of third-party tools and APIs, enabling you to streamline your workflow and enhance your project's functionality.
How does Verpex Web Development Hosting cater to developers' needs?
Verpex Web Development Hosting caters specifically to developers' needs by offering support for a wide range of programming languages, pre-installed development tools, and scalable resources to accommodate the growth of your project.
Do I need web developer skills to use Shopify or WooCommerce?
No. Both platforms welcome beginners, but you might get more out of WooCommerce’s extensions if you’ve got some previous experience.
Does Verpex provide any tutorials or resources for web developers using their hosting service?
Verpex offers a comprehensive knowledge base and blog featuring tutorials, resources, and best practices to help web developers make the most of their hosting services.
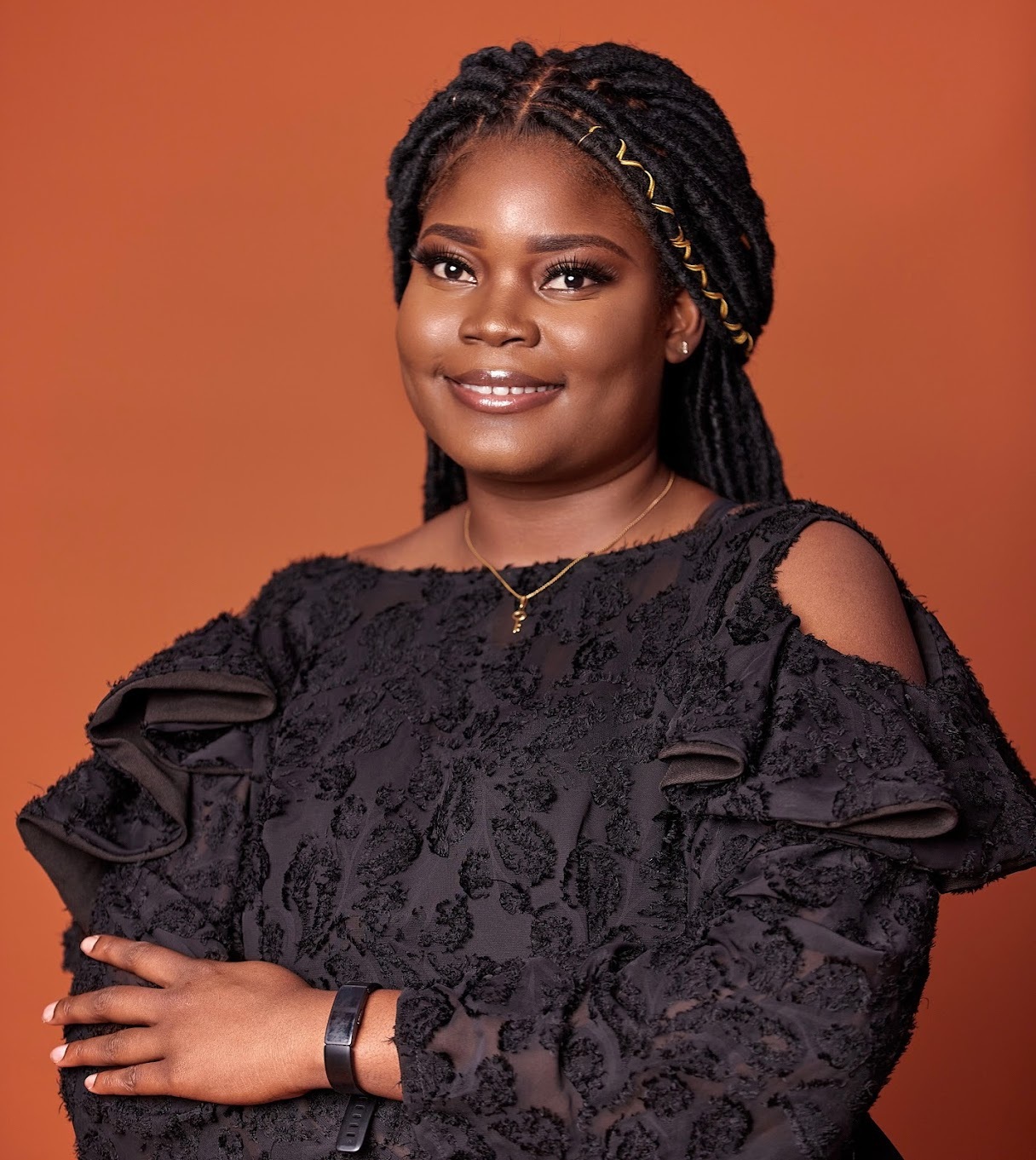
Gift Egwuenu is a developer and content creator based in the Netherlands, She has worked in tech for over 4 years with experience in web development. Her work and focus are on helping people navigate the tech industry by sharing her work and experience in web development, career advice, and developer lifestyle videos.
View all posts by Gift Egwuenu