“What is code without error”? No code at all - just an empty file! The likelihood of errors in any code is 100%, which is why JavaScript provides different methods for handling them.
Before an error is handled, there must be a cause. Errors in JavaScript can be of different types, and can stem from the simplest things which can be quite infuriating.
There are various types of errors that can cause a program to fail and they can be handled in different ways. We’ll go through the possible causes of errors, the different types of errors and how they can be handled.
Let’s get started!
Errors in JavaScript
The term error simply means a deviation of accuracy or correctness. An error in JavaScript is a statement that disrupts the proper operation of a program. Errors can be caused by different reasons;
Errors can occur if there is a missing semicolon, or brackets, within a program.
Errors can occur if a variable that was undefined is called in the code.
Errors can be caused when modules or dependencies are missing, imported or spelt incorrectly.
Compatibility Issues with browsers that may not support certain JavaScript features can lead to errors when the code runs.
Passing incorrect arguments to a function can cause errors.
Unexpected errors can occur when interacting with third-party libraries or APIs that have bugs.
Network Issues or errors might occur if there’s server downtime.
Security errors occur when there's attempts to gain unauthorised access into a system, and other security threats.
Errors can either be the developer’s fault, whether unknowingly or deliberately. These faults may include semantic, logical, and range errors, etc.
Furthermore, there are program errors which may involve network downtime, runtime errors, security issues, and more.
Types of Errors in JavaScript include;
Syntax Error
Syntax error occurs when you do not adhere to the rules of the predefined syntax of JavaScript language. For instance, the incorrect use of quotation marks, omitting semicolons, missing parenthesis, using incorrect operators, and more.
Example of a syntax;
Let y =;
This would throw an unexpected token error.
Declaring a variable with 'Let' is incorrect because JavaScript is case-sensitive. Additionally, the statement is incomplete because including the '=' operator expects a value on the right side.
The correct way would be:
let y;
or
let y= "Hey there"
Extensions like prettier, a popular code formatter that formats code by adding missing quotation marks, spacing, and ensuring that the code style is consistent can help fix this error quickly.
However, it cannot fix logical errors, type errors or blocks of code with incorrect syntax, in such cases ESLint which is a linter that forces developers to follow coding standards can be combined with Prettier to catch both syndtax and logical errors.
Logic Error
Logic errors occur when logic of a program is written incorrectly. It could be using the wrong equation sign when performing arithmetic tasks, etc.
Example;
function isEvenNum(num){
if(num % 2 = 0){
return true;
}else{
return false;
}
}
}
console.log(isEvenNum(4))
Runtime Error
Runtime occurs during the execution of a program, or while the program is running. Syntax errors are usually detected during code compilation however runtime errors are detected when the code with the error is executed.
Here are common types of runtime errors that can occur in JavaScript, including;
Reference Error
Reference error occurs when the code references a variable, object or function that hasn't been initialised using the right keywords (let, var, const) or exists at all.
For instance, Logging a variable x that hasn’t been declared
console.log (x)
we’re attempting to log the value of x to the console

It’s important to check that a variable is declared properly, and uses the right keywords.
Type Error
Type error occurs when a value is used outside the scope or context of its data type. This could happen if a keyword was spelt incorrectly or if you try to access a property or method of an undefined object.
Example;
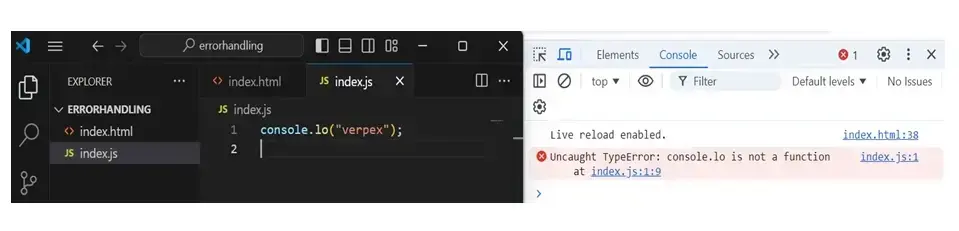
In the image above, console.lo("Verpex")
is attempting to log the string “Verpex” and you can see in the console that it says console.lo is not a function.
Range Error
RangeError occurs when you try to pass a value as an argument to a function and the value is not within the acceptable range of values that the function accepts.
Example:
You can use RangeError to handle cases where the input doesn't meet the criteria of an operation.
const checkIfLegal = (age) => {
if (age >= 18 && age <= 60) {
return age;
} else {
throw new RangeError("Not allowed in, too young to drink!");
}
};
console.log(checkIfLegal(16));
This is what it looks like in the developers’ console;

The purpose of the error is to ensure that the input meets the criteria, and you can use try...catch block to catch and handle the error so it prevents the program from crashing.
try {
console.log(checkIfLegal(16));
} catch (e) {
console.log("Error:", e.message);
}
After adding the try…catch block, this is what it looks like in the developers’ console.
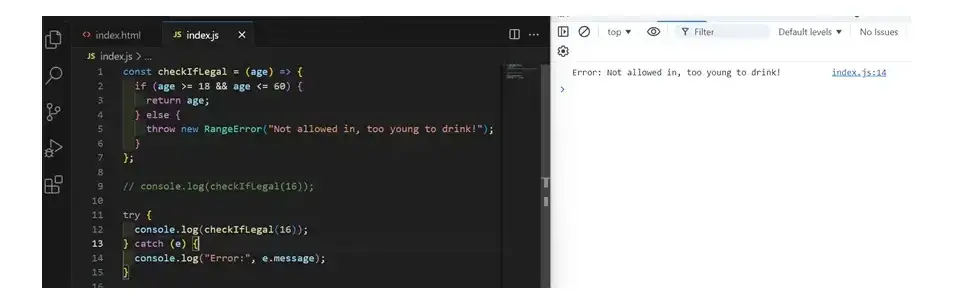
It’s also recommended to use console.error instead of console.log. console.error will highlight the errors by logging them to the console and provide a clear message about the error, which is useful for debugging.
URI (Uniform Resource Identifier) Error
URI error occurs when there is an issue with how the URI (uniform resource identifier) is used in a web application. For instance, using the wrong characters in a URI function.
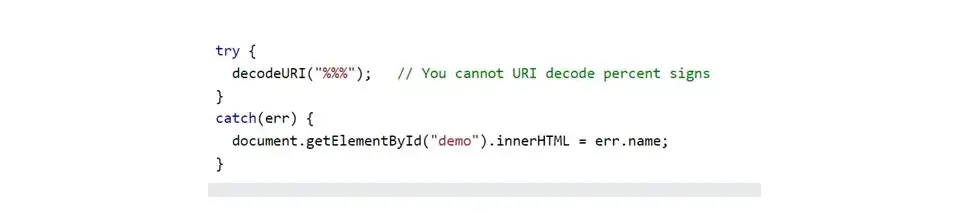
What are Exceptions?
Exceptions are errors thrown at runtime that disrupt the execution of a program, they can be caught and handled using several techniques.
The difference between errors and exceptions is that errors simply refer to the general issues that disrupt the execution of a program, while exceptions refer to errors that occur during runtime.
There are techniques used to catch and handle exceptions in a controlled manner. Examples include; throwing, catching, etc.
The different techniques for handling exceptions in JavaScript include;
Try… Catch
try{} - The try block encloses code that has the potential to cause an error. For instance, trying to establish a connection with an external API. If requesting the API fails and it’s not handled when failure occurs, it can disrupt an entire program.
This is an example of how to wrap our code with the try block;
const getMovies = async () => {
try {
const res = await axios.get("url");
console.log(res);
}
};
After wrapping the code in a try block, you will need a catch block.
catch{}: The catch block catches and handles any thrown errors from try block, it has a parameter named "e" or "error" which represents an error object containing details about the error.
catch (e) {
console.error("Error:", e);
}
Here’s what it looks like together;
const getMovies = async () => {
try {
const res = await axios.get("url");
console.log(res);
} catch (e) {
console.error("Error:", e);
}
};
Try…Catch and Finally
You can also use finally block, which always executes regardless of whether an error occurred.
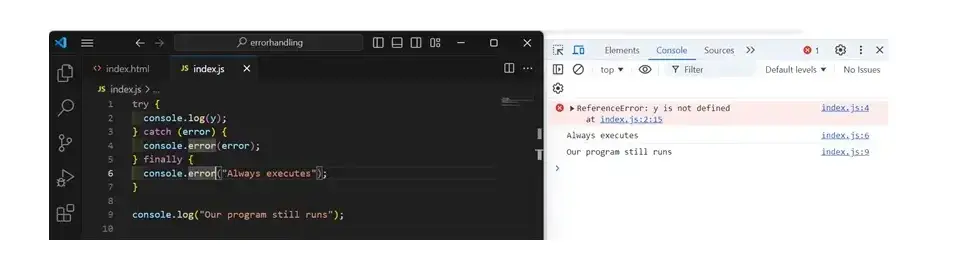
Custom Error (Throw)
When there's an error in a code, JavaScript generates an error message. Throw statement allows you to create your own custom error to provide more specific information about why the code or program failed.
const getAPIs = async () => {
try {
let response = await axios.get("url");
if (!response.ok) {
throw new Error("failed to fetch data");
}
console.log(response.data);
} catch (e) {
console.error(`custom error: ${e.message}`);
}
};
Global Error Handling
Global error handling allows you to catch and handle unhandled exceptions and errors that occur in your application. It can be used to display error messages to users, clean-up tasks, log errors, etc.
There are two methods to set up global error handling, and they are;
window.onerror: This is a global event handler that can be used to handle JavaScript runtime error. It allows you to catch and handle errors at the global level.
Window.onerror consists of five parameters that provide details about the error that occurred. They include;
message: The message associated with the thrown exception
source: The url of the script where error occurred
lineno: The line number where the error was thrown
colno: The column number where the error was thrown
error: The error object that contains information about the error
Example;
You can create a function and save it in window.onerror
window.onerror = function (message, source, lineno, colno, error) {
//perform other actions
console.log("lineNumber:", lineno);
};
window.addEventListener("error",handler): You can use the addEventListener()
method on the window object to listen for errors , and handle them in a flexible manner.
The error event object also comes with properties like message, filename, colno, lineno, and error which provides detailed information about the error.
Example
window.addEventListener("error", function (event) {
//perform other actions
console.error(
" error:",
event.message,
event.filename,
event.lineno,
event.colno,
event.error
);
});
Error Object
In JavaScript there are built-in-error objects such as error, syntaxError, ReferenceError, TypeError, etc which can either be thrown or caught. Error object allows you to handle different types of errors like syntax error, reference error, type error, etc.
try {
//code that might throw an error
undefinedVariable.exponent();
} catch (error) {
if (error instanceof TypeError) {
console.error("TypeError:", error.message);
} else {
console.error("An error occured:", error.message);
}
}
In the above example, the code in the ‘try’ block might throw a TypeError or Reference error when the exponent function is called on undefinedVariable, which is not defined.
The catch block catches the error, the instanceof TypeError checks if the caught error is a TypeError and then logs the TypeError message, else, it logs a more generic error message.
Summary
Errors interrupt the flow of JavaScript programs. There are a ton of scenarios where you may encounter these errors. These examples are just a pin in a sack full of hay, however, they show common errors that developers encounter daily which sometimes can be as minute as using an incomplete equality sign or forgetting to add a comma (,).
It’s important to get familiar with common errors, and how to handle them properly. You would encounter new errors as long as you're working with programs. Thankfully, there are a ton of resources from developers that have encountered these errors before which helps to make solving or handling them easier.
Frequently Asked Questions
Does SSR affect client-side JavaScript functionality?
SSR does not negatively impact client-side JavaScript functionality; both can work together seamlessly.
What makes Astro.js different from traditional JavaScript frameworks?
Astro.js is a static site generator that prioritizes static HTML generation and minimal client-side JavaScript. Unlike traditional JavaScript frameworks, it focuses on faster performance, improved SEO, and seamless integration with multiple front-end frameworks.
Can I use a CDN with JavaScript hosting?
Yes, it's common to integrate a CDN (Content Delivery Network) with JavaScript hosting to improve load times and reduce latency for users across different geographical locations. Verpex hosting includes CDN services in their plans.
How does JavaScript hosting differ from traditional web hosting?
Traditional web hosting is typically optimized for hosting static websites and may not offer the necessary resources or environment for running JavaScript-based applications, especially on the server side. JavaScript hosting, on the other hand, is specifically optimized for JavaScript applications, offering features like Node.js support, database integration, and specialized security measures.
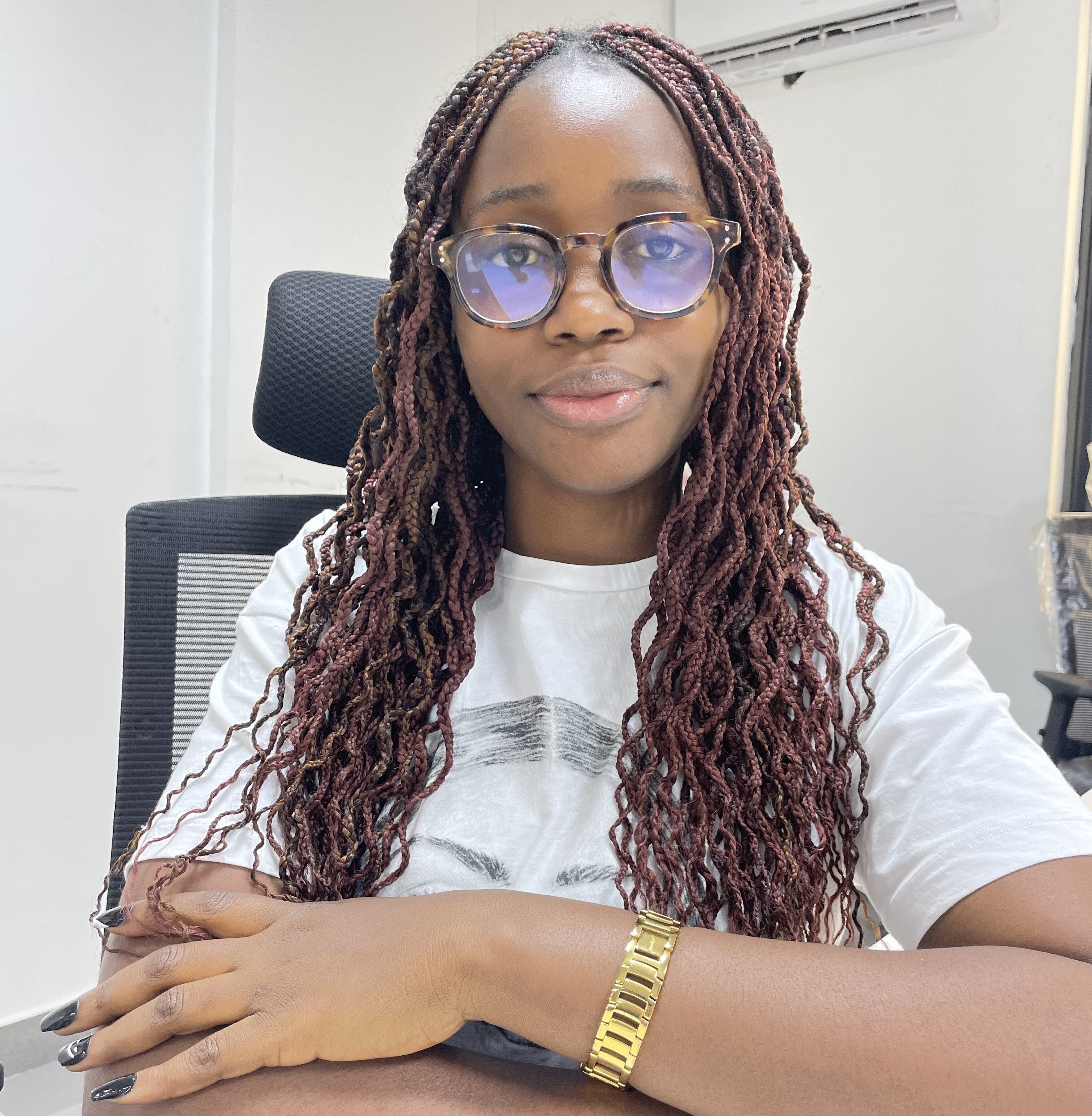
Jessica Agorye is a developer based in Lagos, Nigeria. A witty creative with a love for life, she is dedicated to sharing insights and inspiring others through her writing. With over 5 years of writing experience, she believes that content is king.
View all posts by Jessica Agorye