The process of developing a web application involves various steps, which include the application's requirements, the architecture, databases, backend implementation services, etc.
Websites or web applications that are data-driven usually require backend services that manage and process data. Databases for instance are responsible for the storing and retrieval of data.
When building an application or website - especially those that handle large amounts of data (e.g. e-commerce, social media, content, and media streaming platforms) scalability should always be top of mind.
The reason why a business should think of scaling or ensuring that its websites or apps are built to scale is to accommodate growth. One moment, there are 200 users registered on a web application; the next, there may be about 200,000 people registered on the same platform.
How can we ensure that the infrastructure doesn't break when large users are interacting with the application or website?
In this article, we’ll be looking at a platform developed by Google called Firebase that helps developers build scalable web and mobile applications, and we’ll also be creating a simple CRUD operation using Firebase.
Let’s get started!
What is Firebase?
Simply defined on the platform itself, Firebase is an app development platform that helps you build and grow apps and games users love. It is also used for web development projects and is not exclusive to mobile and web applications.
Firebase is Google’s client-side and server-side development platform based on Google Cloud, which offers a suite of tools and services for building and scaling web and mobile applications.
Firebase also integrates with other Google tools like, Ads and Analytics to gain insights into users' interactions and allow business to monetize their applications through in-app advertising, etc.
Firebase is built on Google’s infrastructure and provides backend services for building and managing web and mobile applications. It’s built to be scalable, its cloud infrastructure is robust including databases, servers, networking capabilities, etc.
Firebase supports multiple client app platforms and provides SDKs for various programming languages, including IOS (Swift, Objective-C), Android (Java, Kotlin), and web development (JavaScript, TypeScript). It also offers support for frameworks such as Angular, React, and Vue.
Importance of building Scalable Applications
Growth: An application built to scale can handle large user traffic, and data volume without major glitches in performance. This means that the application can grow as users grow without experiencing setbacks.
User Experience: When an application is built to be scalable, it functions smoothly and is designed with fail-safe mechanisms to reduce downtime for an expanding user base, thereby impacting or enhancing user experience.
Cost Efficiency: Scalable applications are cost-efficient because they can accommodate a larger user base without requiring additional hardware or resources. Implementing scalability techniques in the early stages helps avoid costly modifications as the user base grows.
Competitive Edge: Building scalable applications can provide businesses with a competitive advantage by enabling fast responses to changes in market demand, quick addition of features, and adaptation to overall customer needs. The ability to scale keeps businesses on top of their game and prepared for growth opportunities
Advantages of Using Firebase
Here are a few advantages of using Firebase;
Large Databases: Firebase is built on Google’s infrastructure, and it uses its resources to offer robust databases for web and mobile applications.
Safe and Fast Hosting: Firebase offers secure, fast hosting services that accommodate various types of content, including web applications, static, and dynamic content.
Google analytics Tool: Google Analytics tools are offered by Google and can be integrated with Firebase. This tool provides developers with insights into the behavior of mobile and web applications.
Additionally, Firebase Analytics is efficient in enhancing the retention and engagement rate of users using your application, as it gives insights into the behavior of users.
Firebase Testing Services: Firebase has comprehensive testing services that provide different testing solutions to ensure reliable and efficient software applications. The different types of testing services offered by firebase include;
- Firebase Crashlytics
- Firebase Test Lab
- Firebase Performance Monitoring
- Firebase App Distribution,
Machine Learning Ability: Firebase incorporates Firebase Machine Learning, also known as ML Kit, which offers specialized APIs that developers can utilize for tasks such as text recognition, image labelling, face detection, barcode scanning, and more.
Additionally, Firebase ML provides ready-to-use cloud-based APIs powered by Google Cloud's ML technology, ensuring accuracy and reliability in machine learning operations.
Cross-Platform Messaging: Firebase Cloud Messaging provides an affordable way to send messages across different platforms. It helps apps send notification messages and allows for targeting specific target audiences with messages within the app or web app environment.
Let’s Create a Simple CRUD Operation Using Firebase
In Firebase, we'll use a tool called the real-time Database to perform CRUD (Create, Read, Update, and Delete) operations. The real-time Database enables real-time synchronization of data, facilitating seamless interaction with the database and ensuring that any changes made to the database are quickly reflected across all connected users.
Step 1: Create an account on firebase, click on get started to create a project. When your project is ready, it would look like the image below. Click continue to move to the next step.
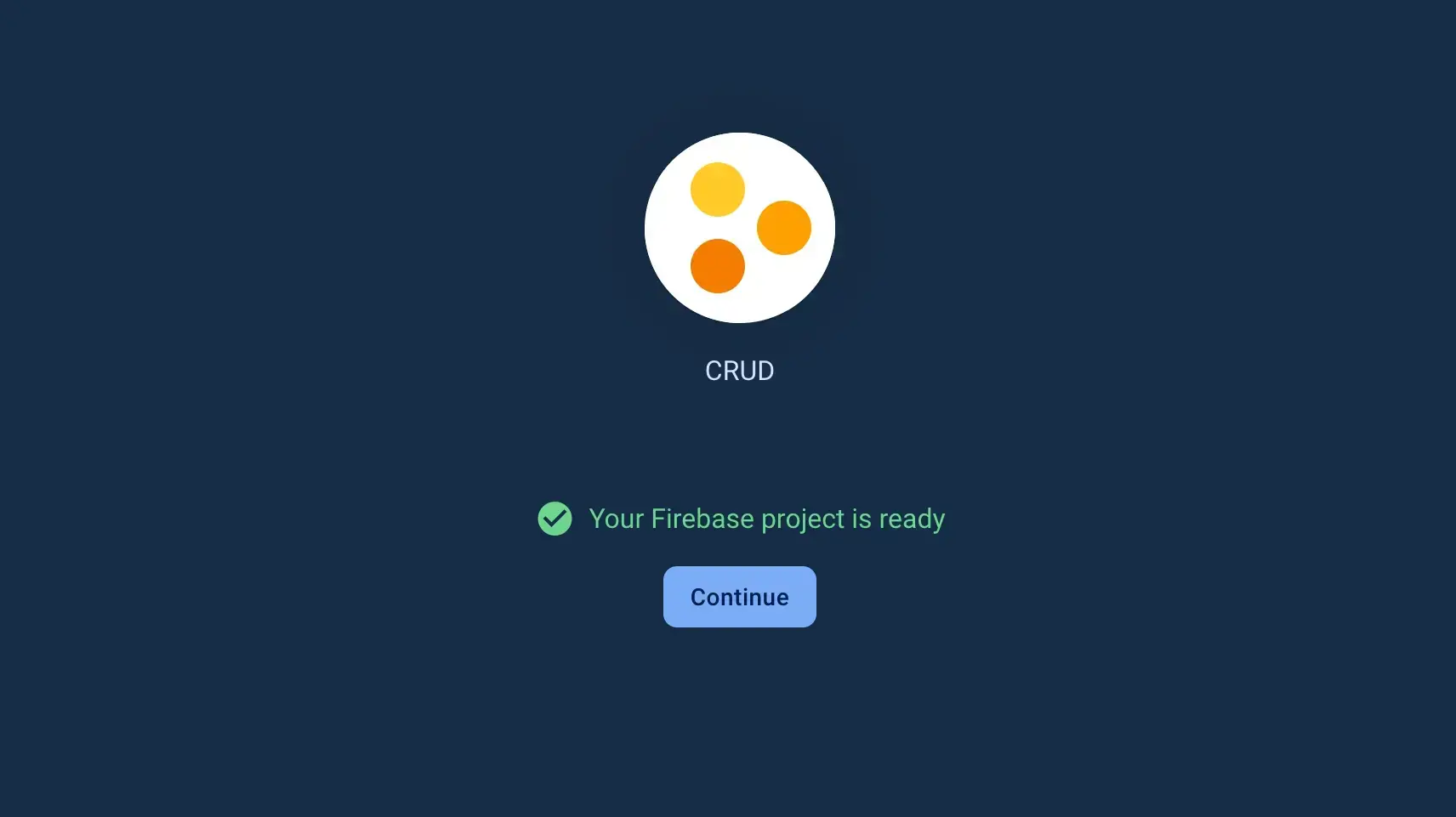
Step 2: After you click on continue, you’ll be directed to a firebase console.
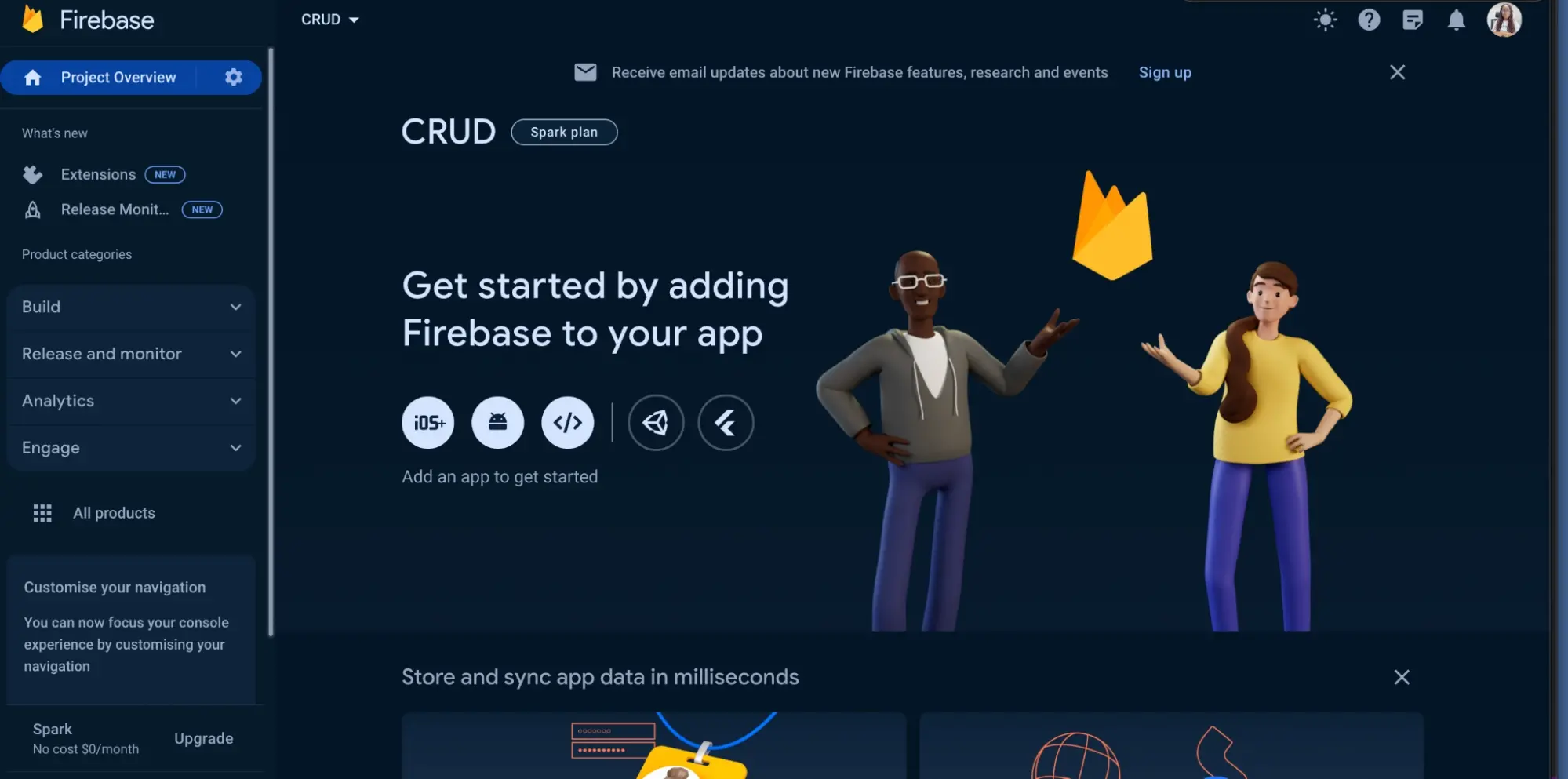
Step 3: Click on build and select the option “real-time database”
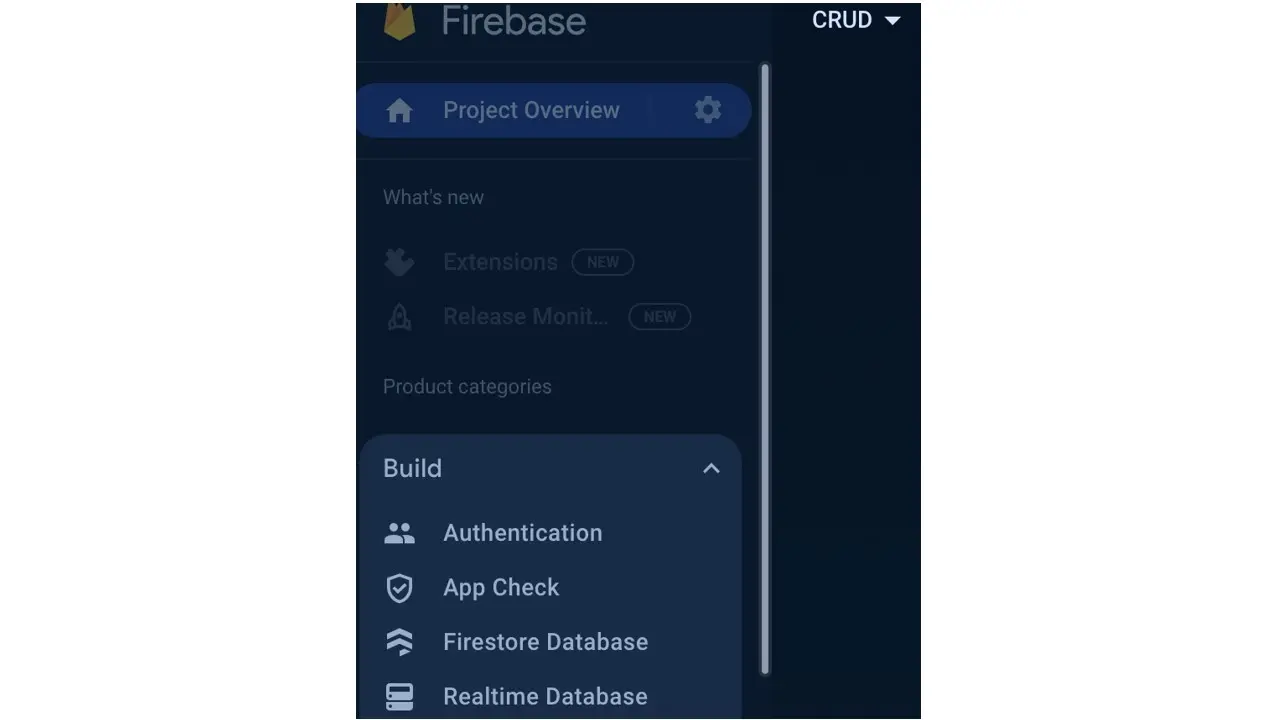
Step 4: Click on Create Database
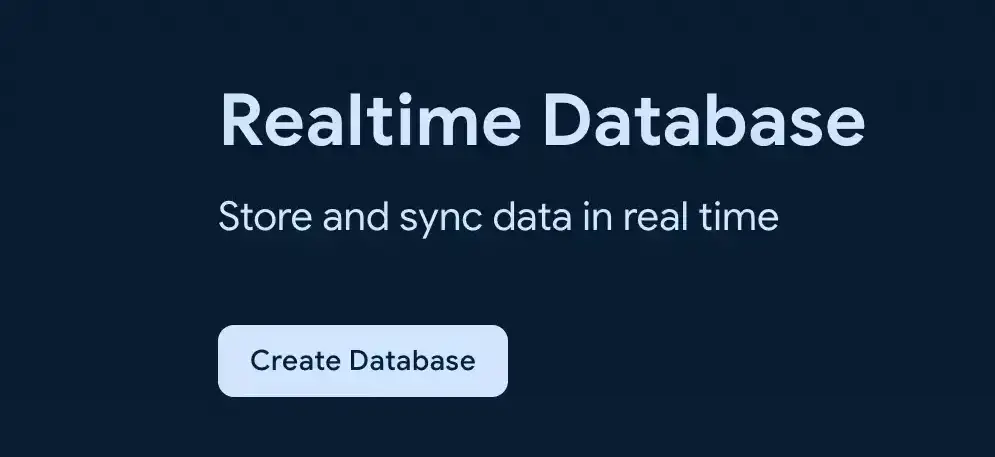
Step 5: After clicking create Database, click next, select the option start in test mode, and click the enable button.
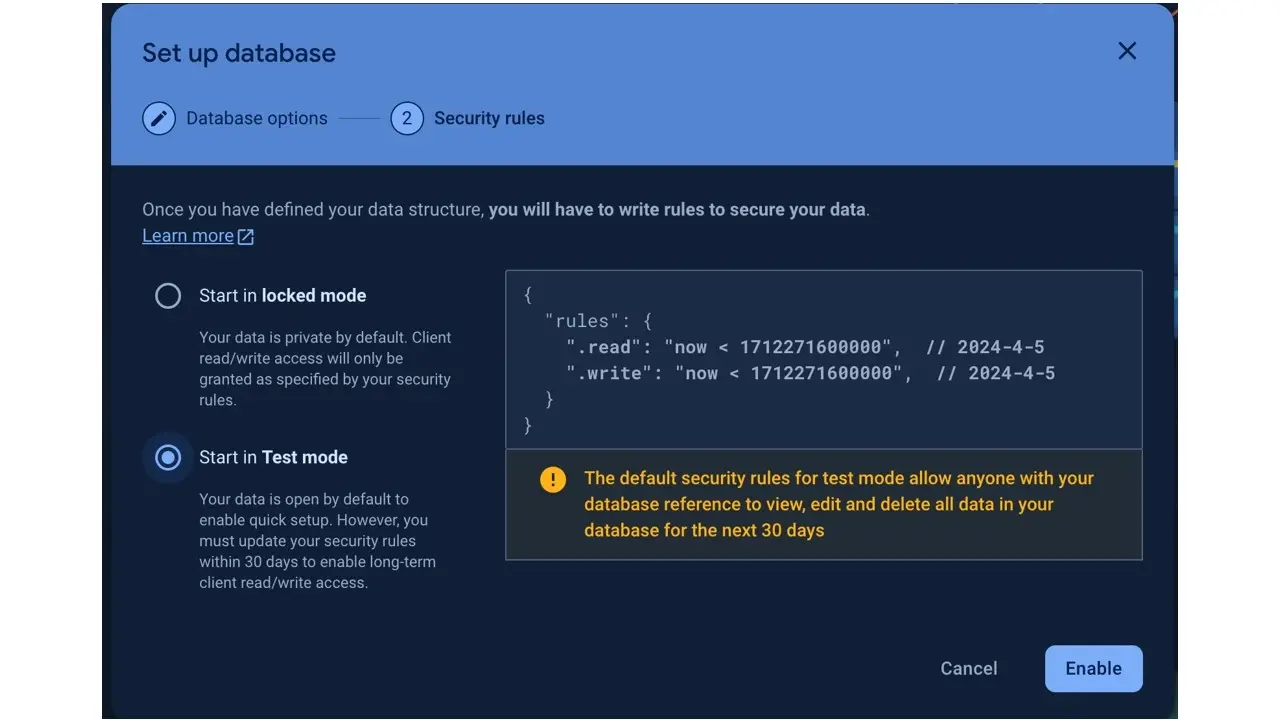
Step 6: The next view would look like so;
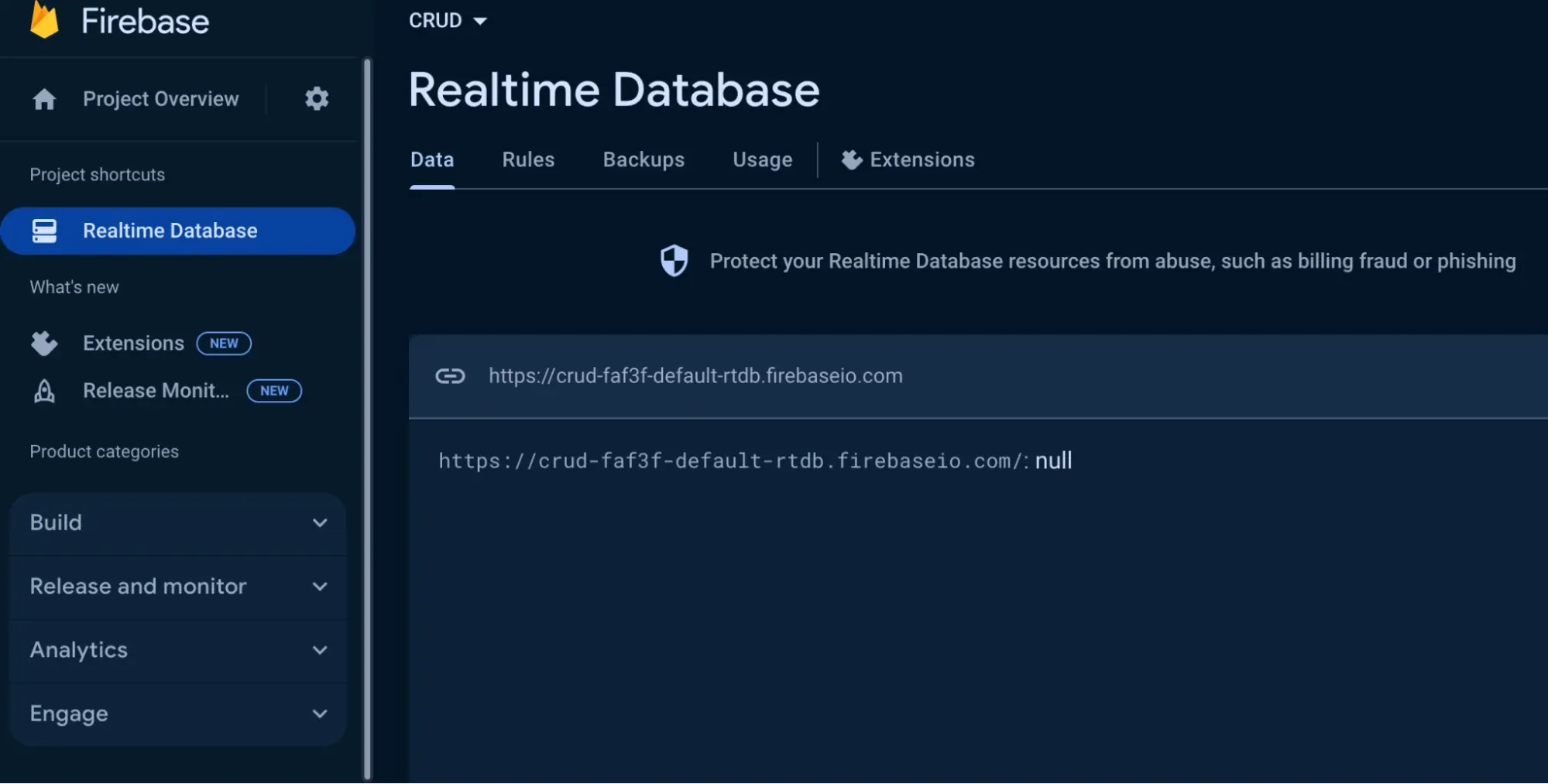
Step 7: At the top of the page, beside the project overview is a settings gear, click on it and select the option “project settings”.
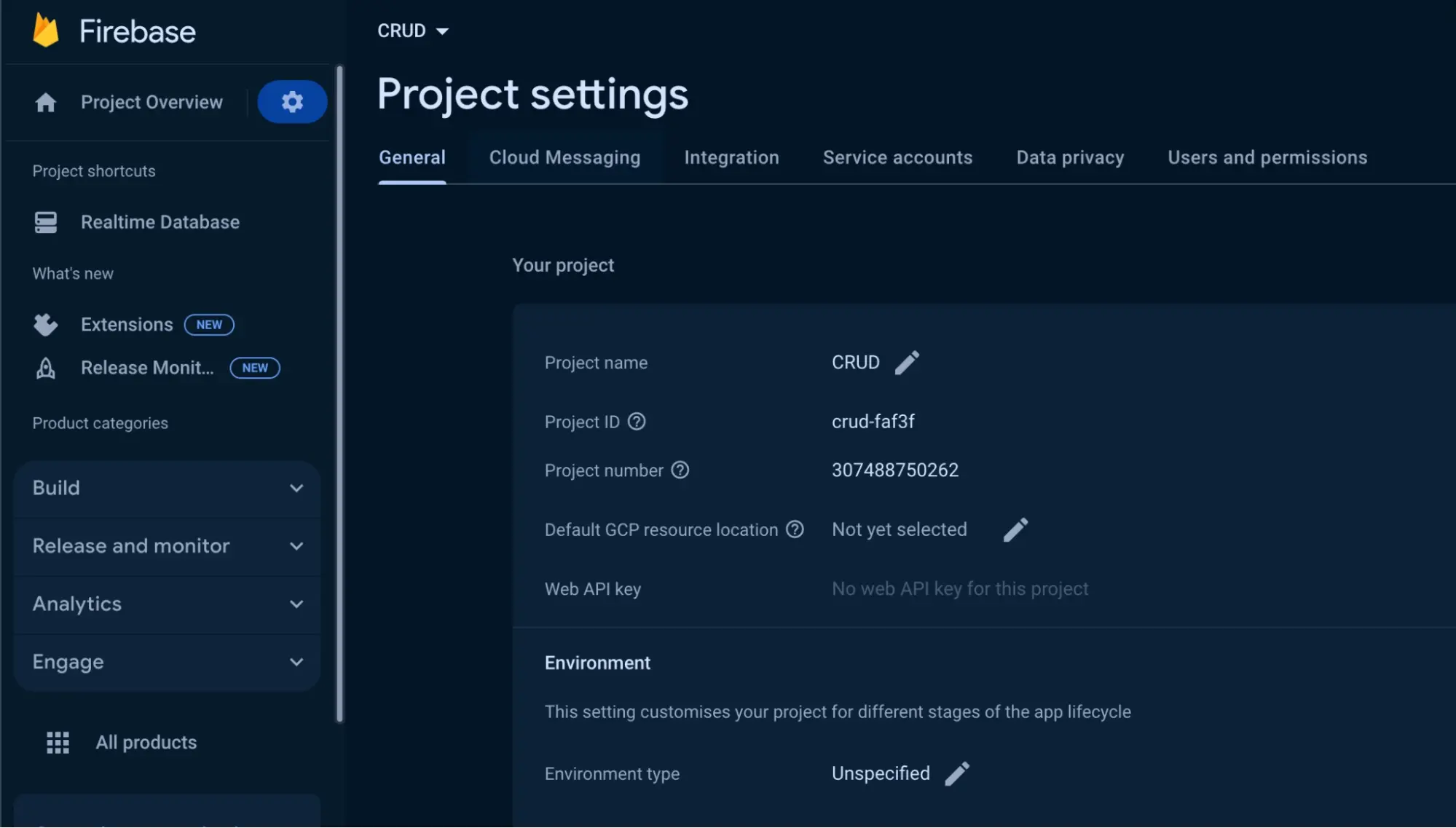
Step 8: In project settings, scroll to the section of the page with title “your apps”, and select the web app option represented by the closing angle bracket </> to add a web app.
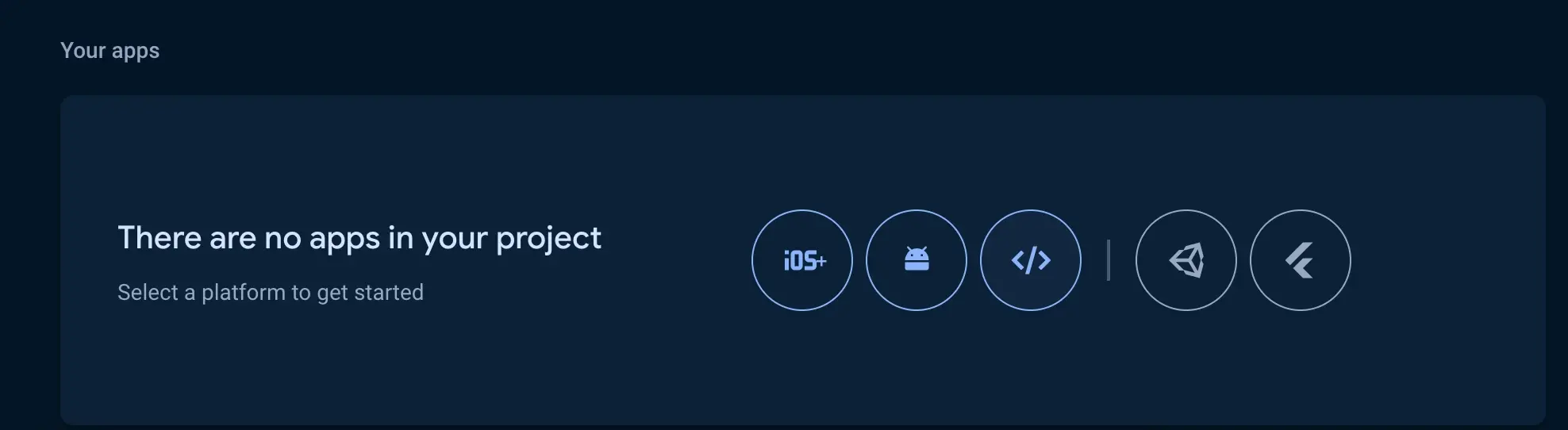
Step 9: To add firebase to a web app, you’ll need to provide an app nickname, and then click on the register app button.
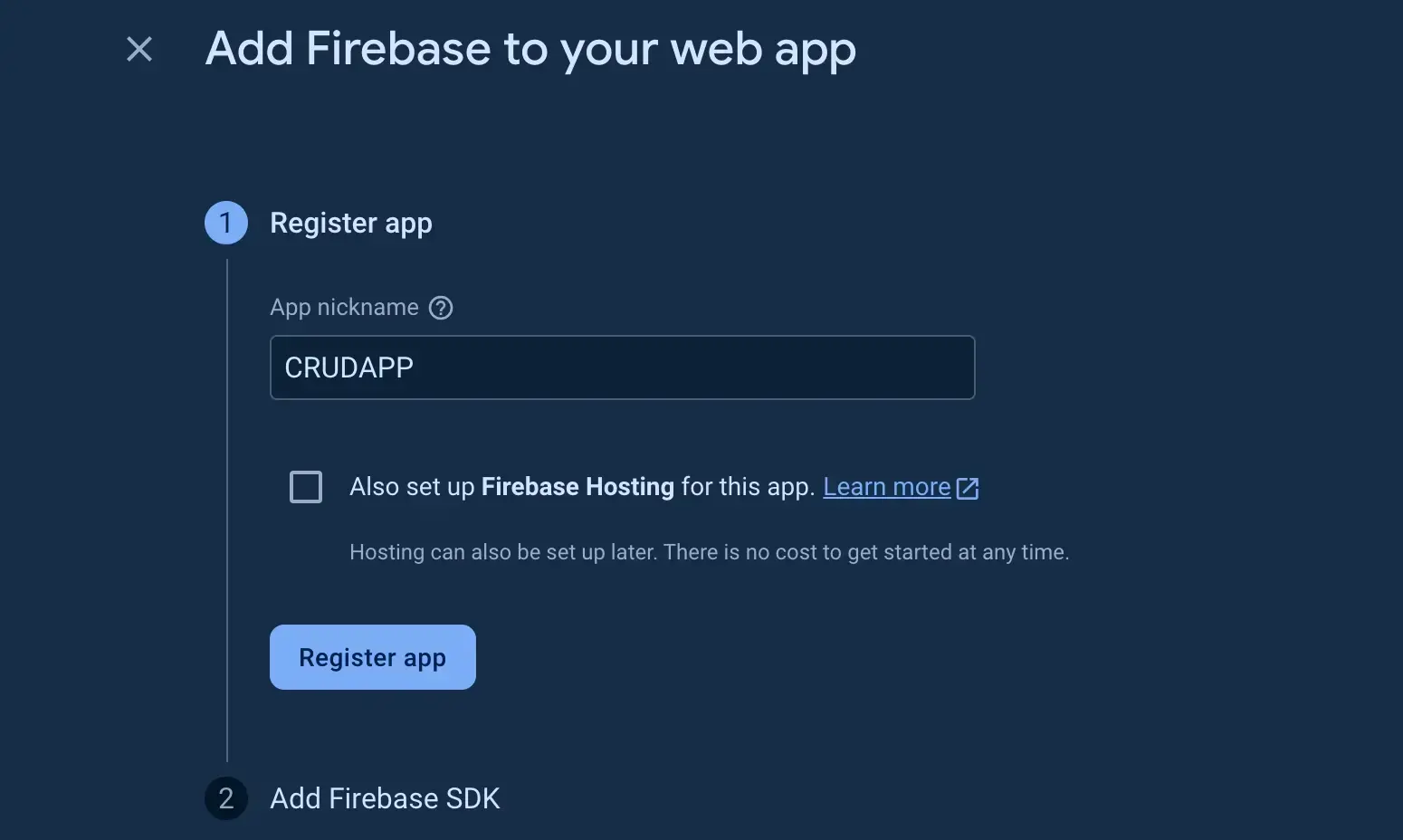
Step 10: After you click on “Register App”, you’ll see another option that says “add firebase SDK” scroll down and click on the “continue to the console” button.
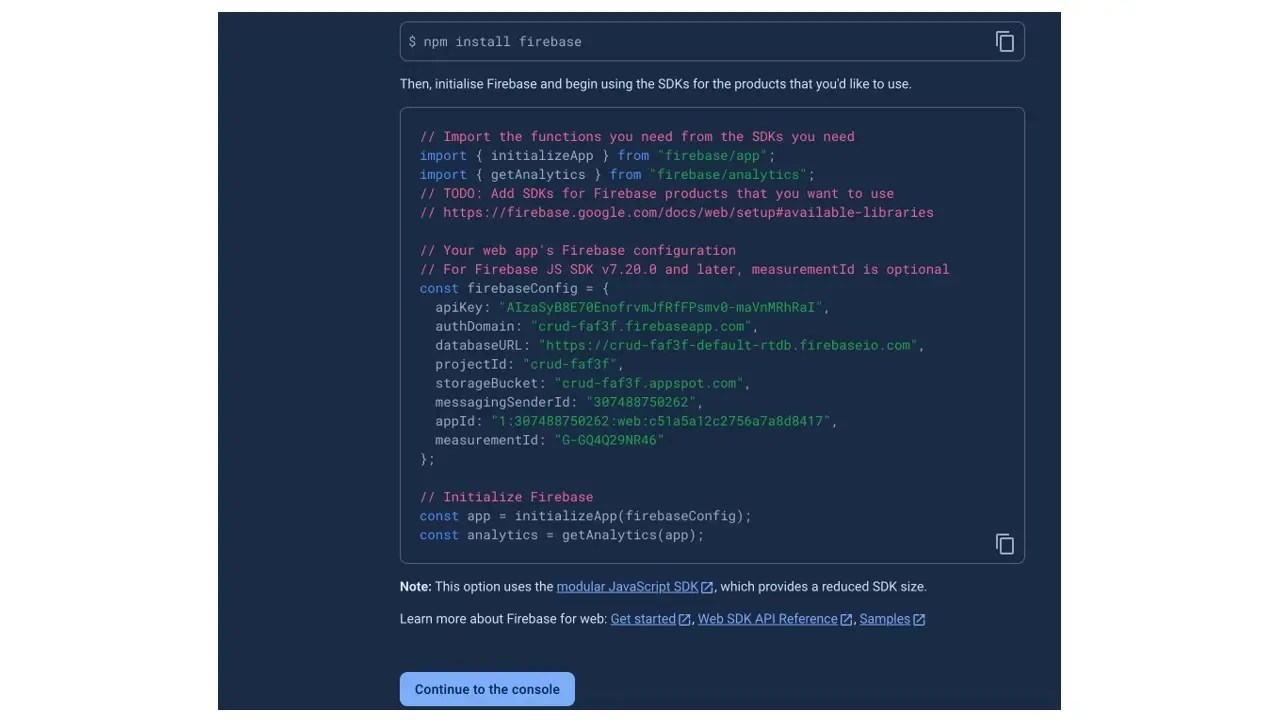
Step 11: In VSCode let’s create a simple form that accepts different data types that include; String, Boolean, Objects, so that we can perform a simple CRUD operation.
Step 12: Create a simple form with different data types.
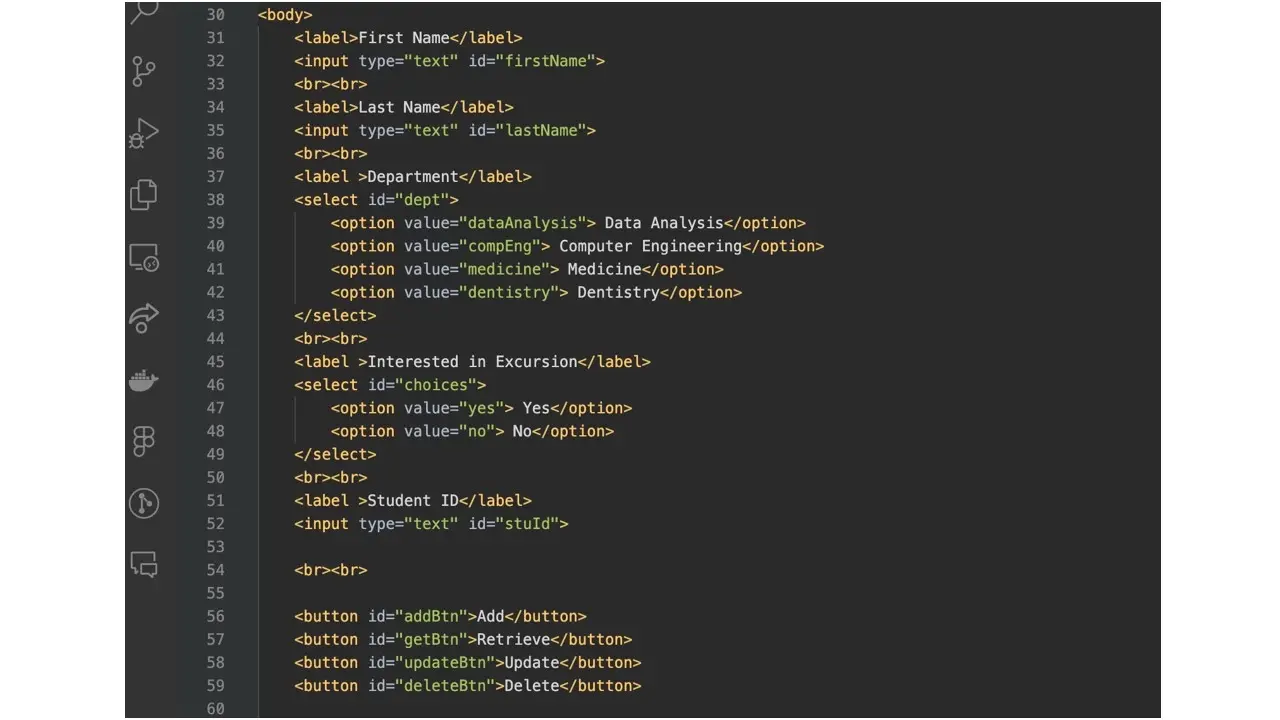
You can include your own CSS style at inside the <head> </head>
of the document. This is what our form looks like;
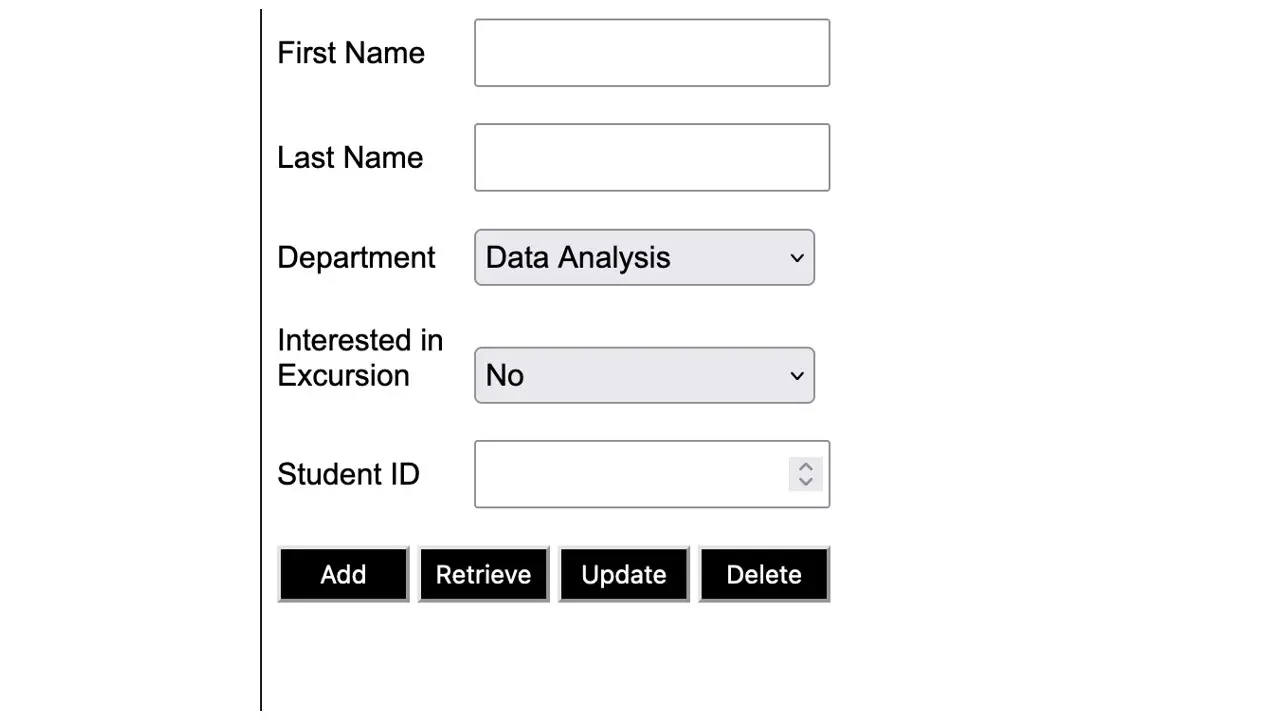
Fire Base Configuration
Step 13: To configure firebase, we need to add a script into our document- in firebase settings, click on CDN and copy the entire script.
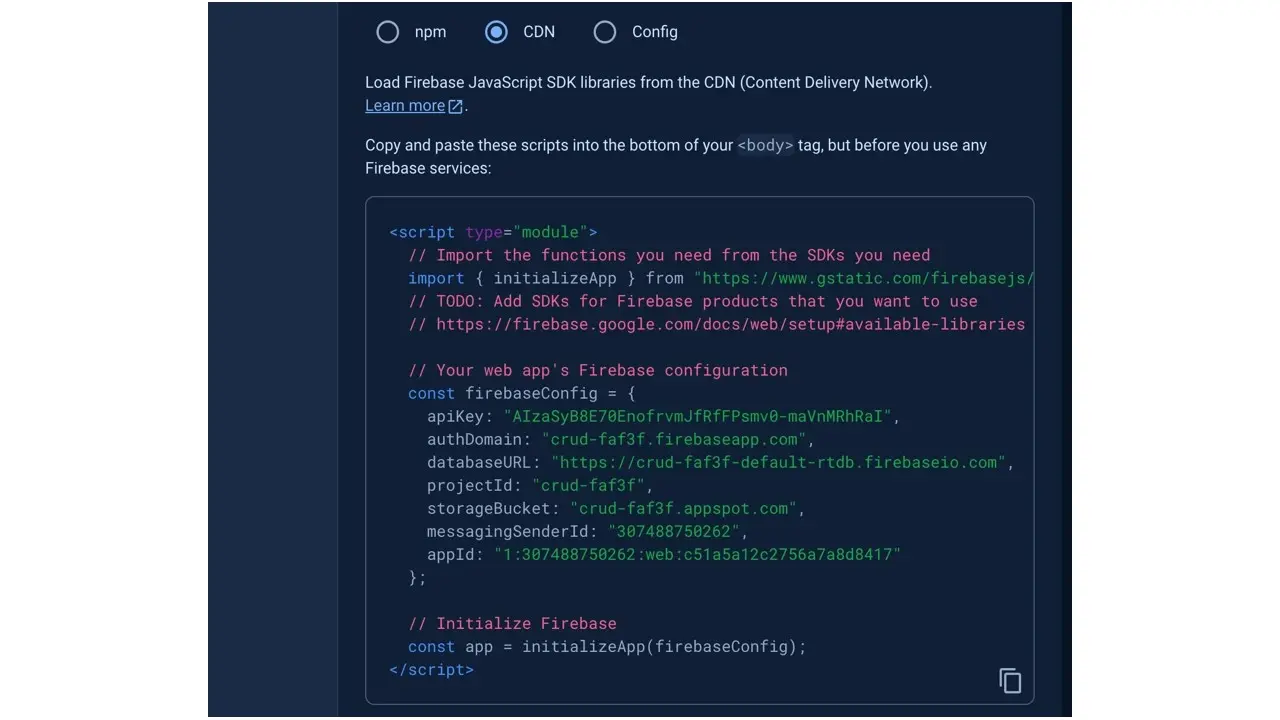
Step 14: Add the script in your html file, and remove all comments.
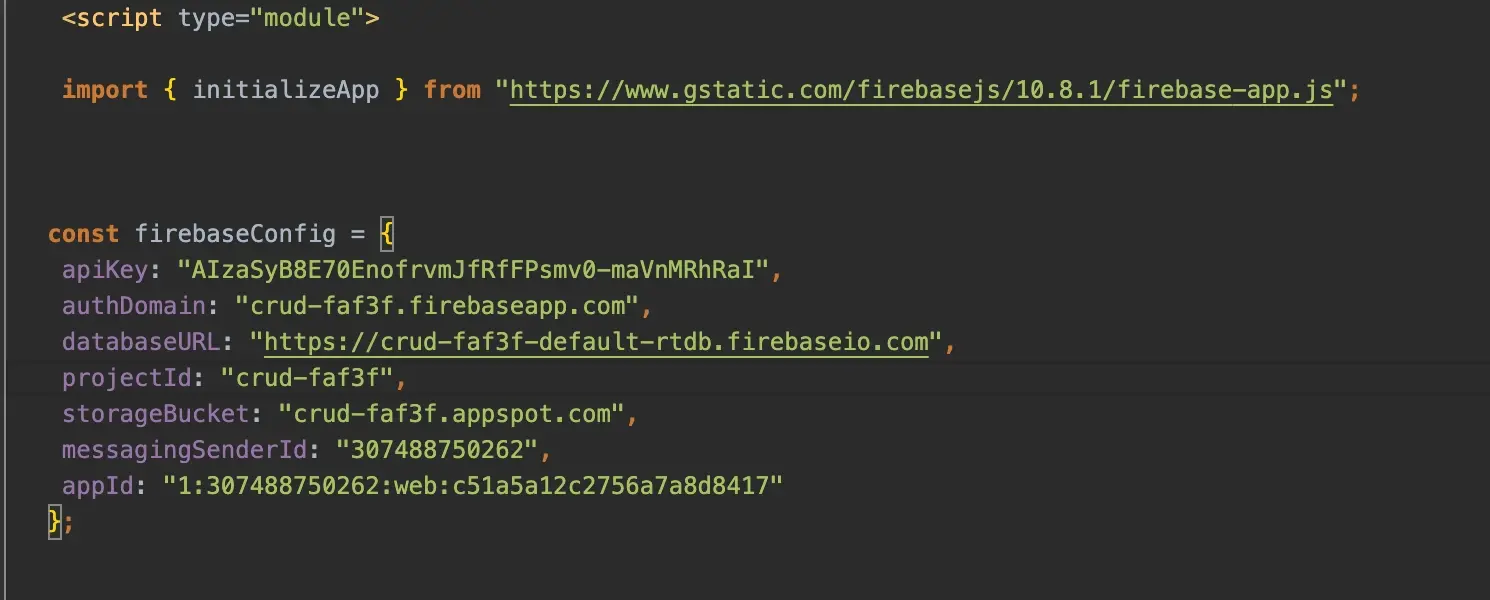
Step 15: Define another import statement, and import from firebase database like so;

Step 16: Define a constant to get the database.
const db = getDatabase();

Step 17: Get the reference(id) of all the defined HTML input like so;
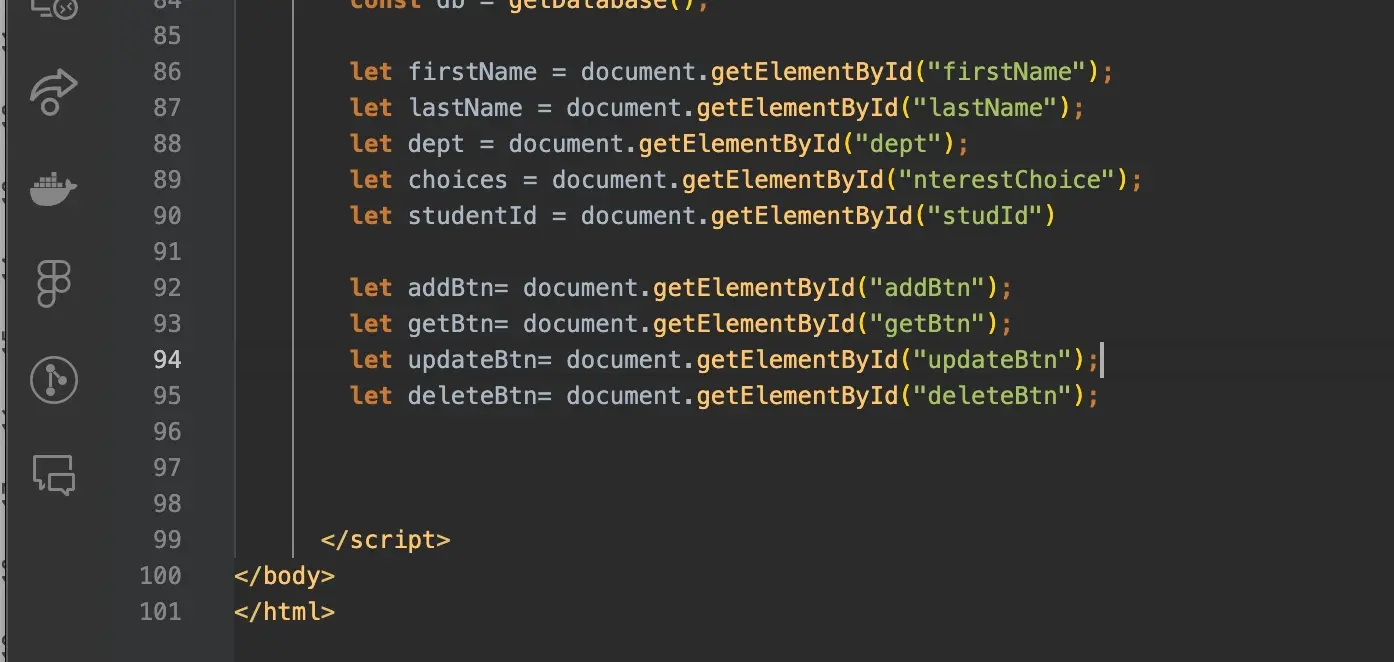
Firebase functions
Step 18: To create the add function, we’ll use firebase set function which requires two arguments - ref which contains the db constant we created and a path, and a second argument which is an object that holds data we want to save in the database. The object is usually in a key value pair.
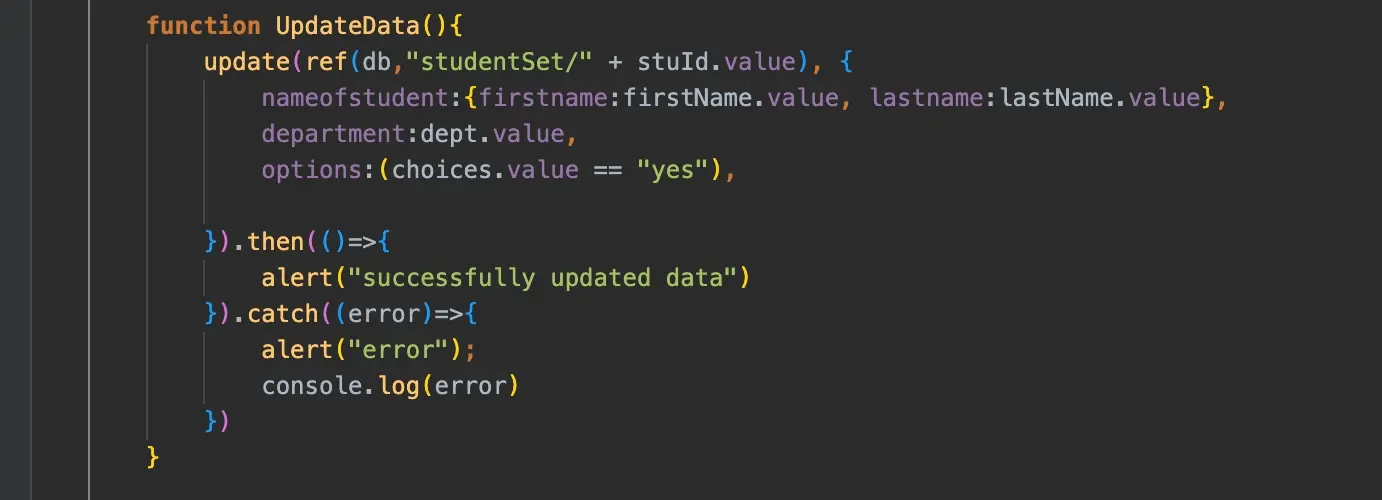
Step 19: Let’s create a retrieve data function, to grab the data stored in the firebase database.
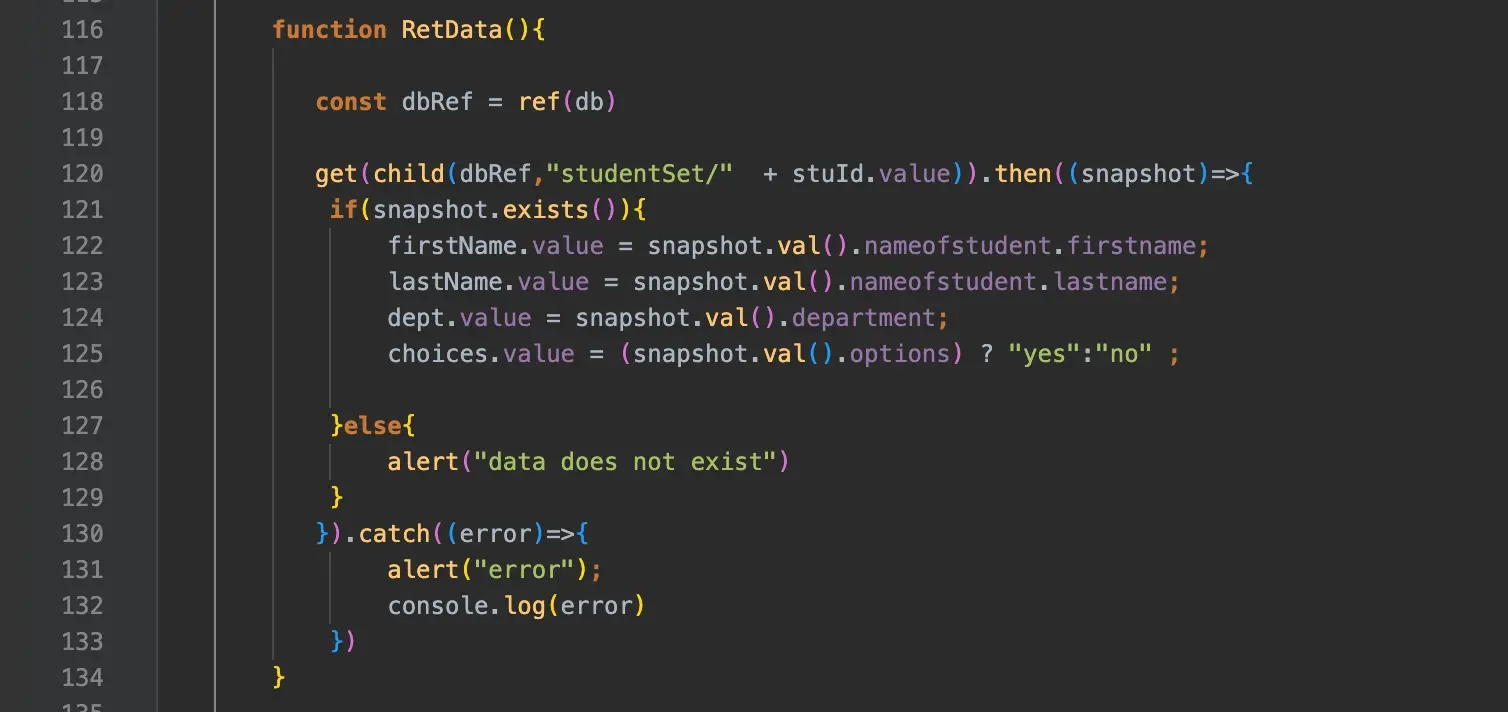
get(Child(dbRef, “studentSet/” + studId.value)) - retrieves data from firebase. “dbRef” references the database, the child navigates to the specific child node in the database, fetching data from the studentSet path, and the studId.value specifies the student data to retrieve.
.then((snapshot)=>{...} The snapshot is a promise that when the data is recovered the function is executed with a snapshot of the data.
This code fetches data from the real-time database with the studentId and updates the fields in the UI with the data.
Step 20: Let’s create an update data function, the update function is used to modify data in a specific location in the database.
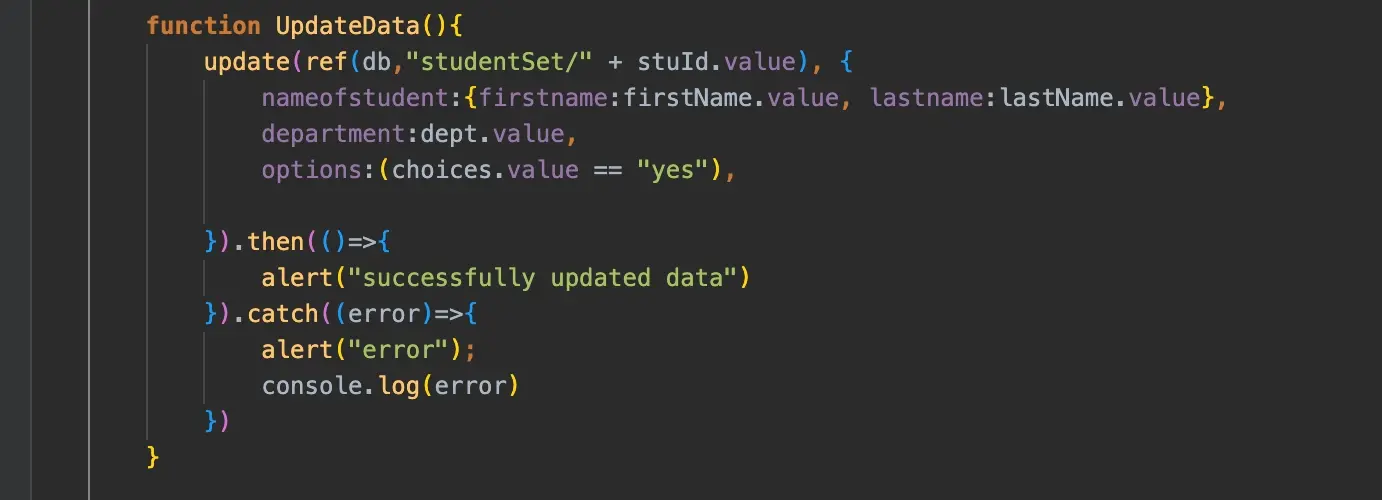
update(ref(db,"studentSet/" + stuId.value),{...} this line of code calls the update function, it takes two arguments; (ref(db,"studentSet/" + stuId.value) the first argument references the location in the database to be updated, the stuId.value is the unique identifier that points to the data to be updated.
The second argument is the object that contains the data to be updated e.g. students firstName, lastName,dept,options.
This function updates student data in the database and also handles success and error conditions providing feedback using alerts
Step 21: Let's create a delete function
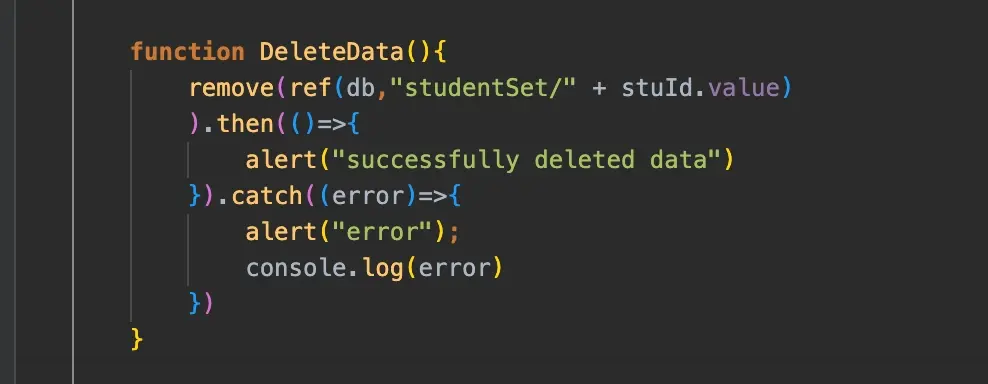
This function deletes data of students from the database. It uses the remove function to delete data from a specific location in the database. "studentSet/" is the path where the students data are located and the + stuId.value represents the students unique identifier.
Step 22: Create event listeners to execute the functions
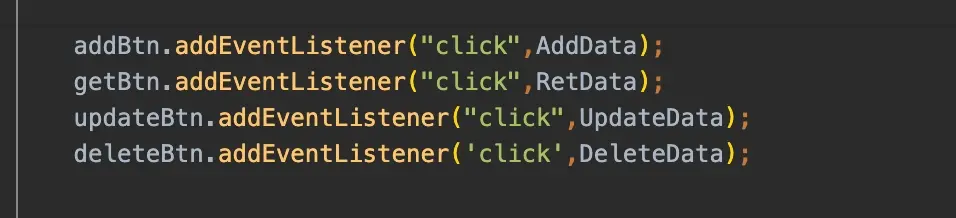
These event listeners trigger the specific functions that correspond with the button when they are clicked. E.g the addBtn.addEventListener(“click”,AddData); executes AddData function when the “add” button is clicked.
Step 23: Let’s test to see that our code works, and send data to firebase from our form. Fill out the form and click on the Add button, you’ll see an alert that says successfully added data.
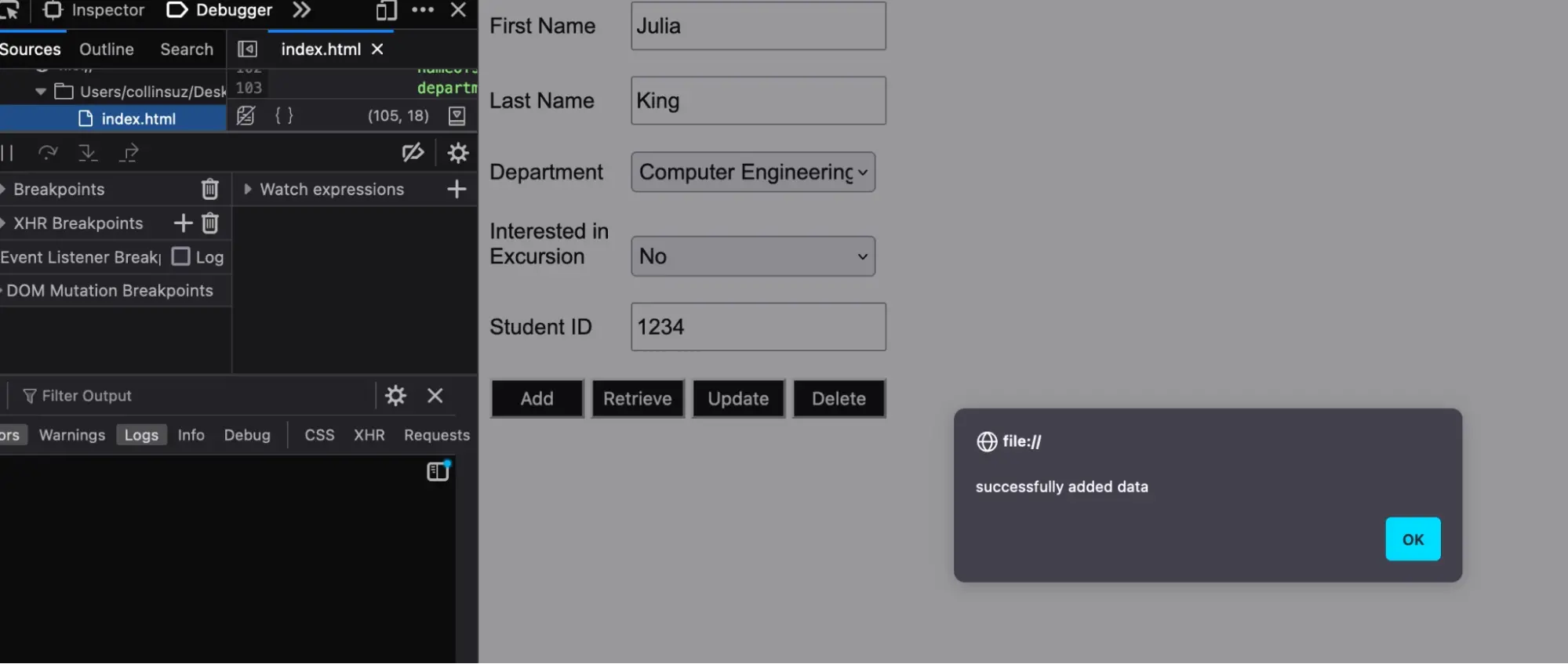
Step 24: Check firebase console to see if the data has been sent successfully. In the image below you can see that the data from the form has been sent to firebase database.
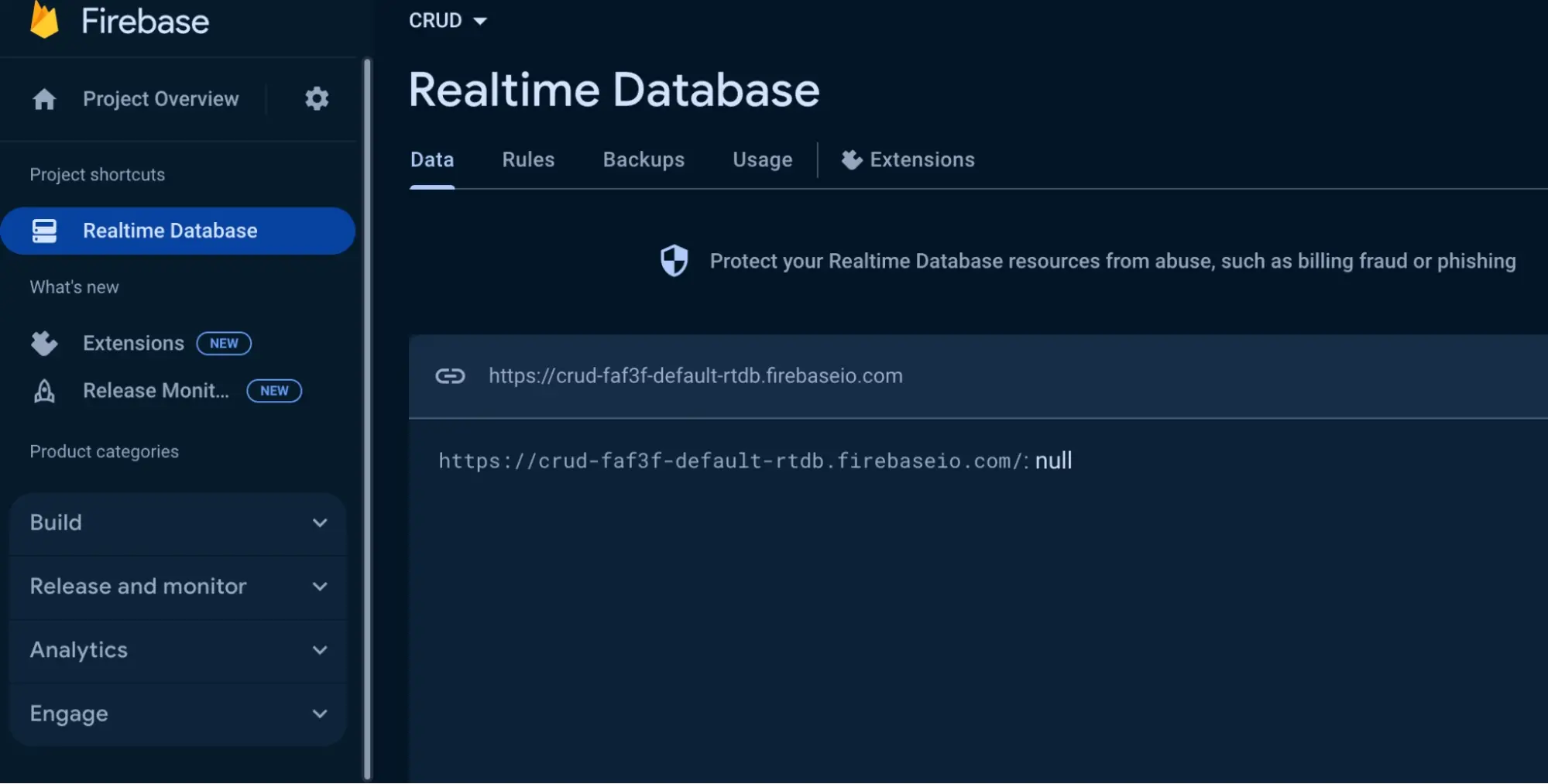
Step 25: Retrieve Data from firebase database. To perform this action, enter the id number of the data you want to retrieve and click the retrieve data button. The form will be filled with the data registered with the id.
Step 26: To update data, simply make the changes from the form using the id that’s linked to the data you want to change. In our form we changed the department from computer engineering to medicine.
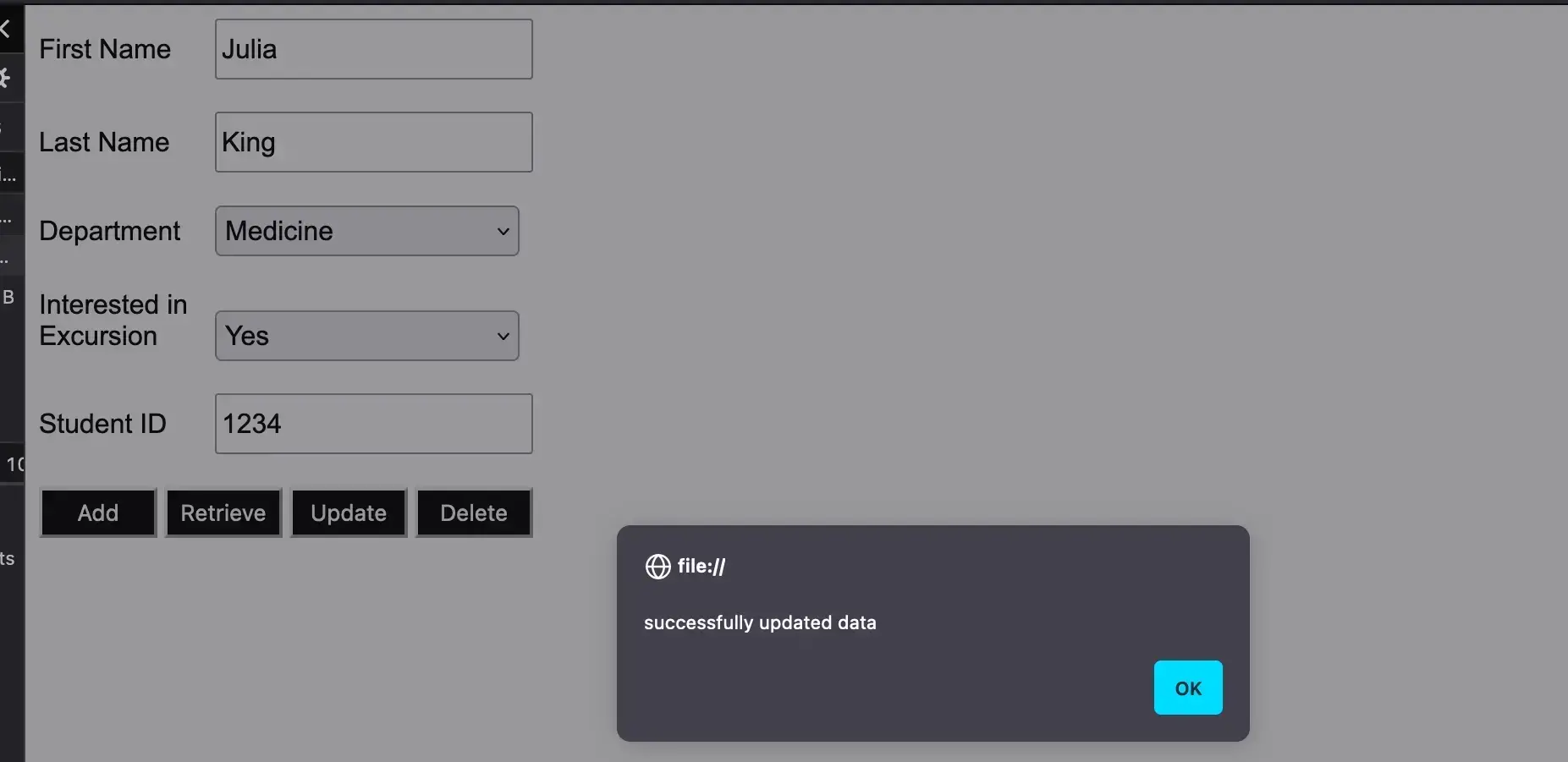
This is the result in firebase;
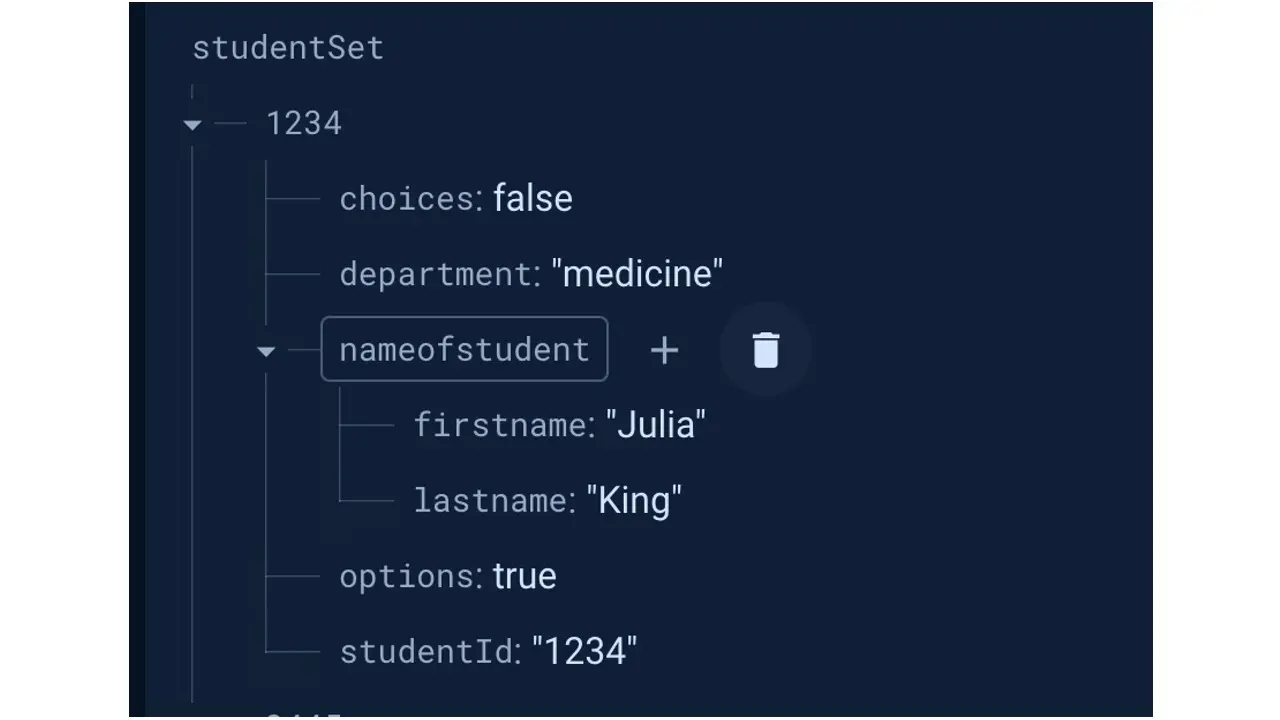
Step 27: Let’s delete the data with student id “1234”. To achieve this enter the id of the data you want to delete from the database in the input and click the delete button. In firebase you’ll notice that we no longer have the data with the id “1234”
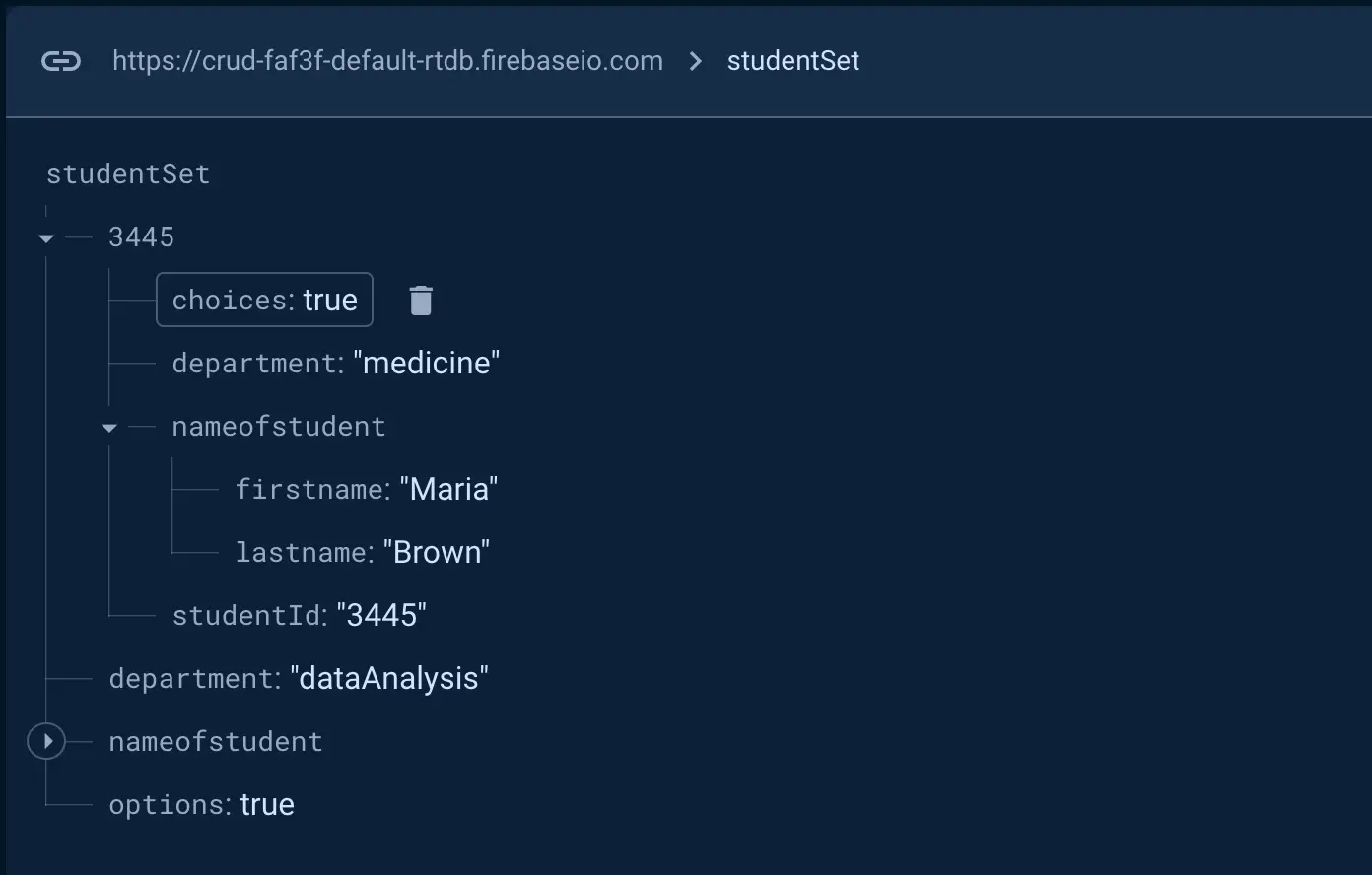
Summary
Firebase collection of tools for developers solves the issue of scalability and performance of web applications and mobile applications.
The real-time Database on the web makes it easy, with just a few simple lines of code, to store and sync data in real-time using a NoSQL cloud database
Data is stored in JSON format and is synced across all users and available offline. Firebase manages the infrastructure of the database system by handling building, maintaining and hosting the database.
It’s a great tool to use because of how efficient and convenient it is.
Frequently Asked Questions
How does AR affect mobile commerce or shopping via mobile apps?
AR enhances mobile commerce by offering interactive experiences, increasing engagement and sales.
Is SSR suitable for mobile web applications?
Yes, SSR is suitable for mobile web apps as it improves load times, which is crucial for users on slower mobile networks.
How do CSPs interact with mobile applications or progressive web apps?
CSP can be applied to both mobile applications and progressive web apps by configuring policies to control content sources and enhance security on these platforms.
Can Server-Side Rendering slow down my web application?
While it can introduce slight server overhead, SSR often enhances web application performance rather than slowing it down.
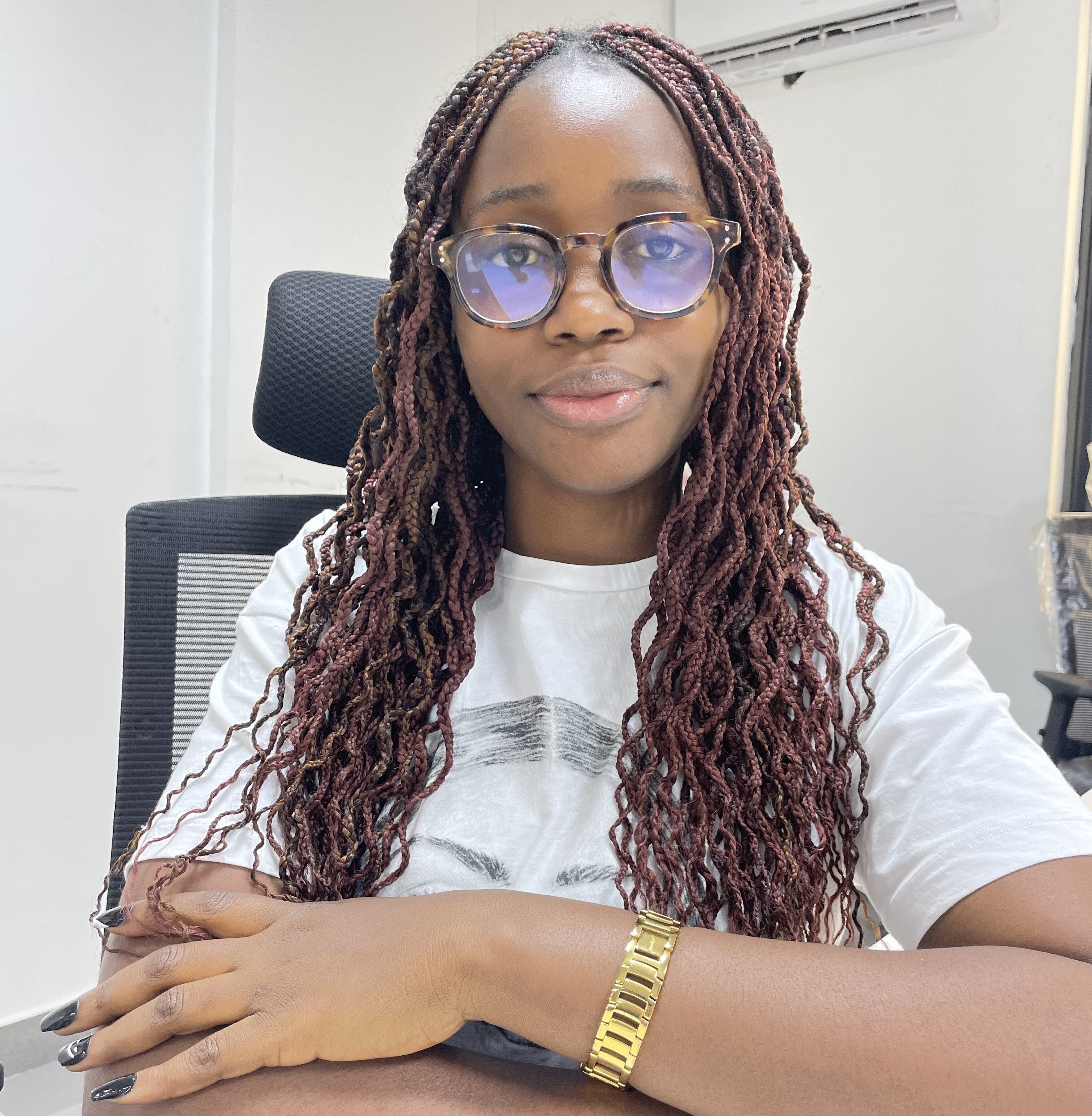
Jessica Agorye is a developer based in Lagos, Nigeria. A witty creative with a love for life, she is dedicated to sharing insights and inspiring others through her writing. With over 5 years of writing experience, she believes that content is king.
View all posts by Jessica Agorye