Websites provide different functionalities for different purposes, enhancing and increasing user engagement. A mortgage calculator is a valuable tool that can be added to a website's functionality.
A mortgage calculator can be mostly found on real estate websites, mortgage broker sites, finance websites, real estate investment platforms, etc.
How can you add a mortgage calculator to a website? A mortgage calculator can be added to a website in various ways, and we’ll discuss a couple in this article.
Let’s briefly go through what a mortgage calculator is and then the steps required to add one to a website.
What is a Mortgage?
A mortgage is a loan used to purchase real estate, such as a home, land, etc. This transaction is between a borrower and a lender. The loan is borrowed from a financer, and the lender agrees to pay back the loan in installments over a while with interest.
If the borrower fails to repay the loan, the real estate in question is repossessed by the lender or financer. Also, there are different types of mortgages depending on their features and benefits.
What is a Mortgage Calculator?
Individuals who want to purchase a home or refinance an existing mortgage can use a mortgage calculator. Here are a couple of reasons why a mortgage calculator is necessary;
Budgeting: A mortgage calculator can help potential homeowners create a budget by understanding how much they can afford to borrow, to make monthly payments based on the loan amount, interest rate, and terms.
Loan Rate Comparison: A mortgage calculator can help to compare loan offers from different financers. By calculating the interest rate you can see which financer or lender’s offer is beneficial
Time-Saving: A mortgage calculator helps you calculate much faster instead of doing the calculations manually. You can apply different loan amounts, and compare the results to know which loan amount works better for you.
Convenience: Users can calculate mortgage payments easily without the help of a financial adviser, to first understand what it entails before communicating with the advisor. This ensures that the potential real-estate buyer is going in with understanding and having an estimate for mortgage payments.
How to add Mortgage Calculator to your Website
WordPress Site
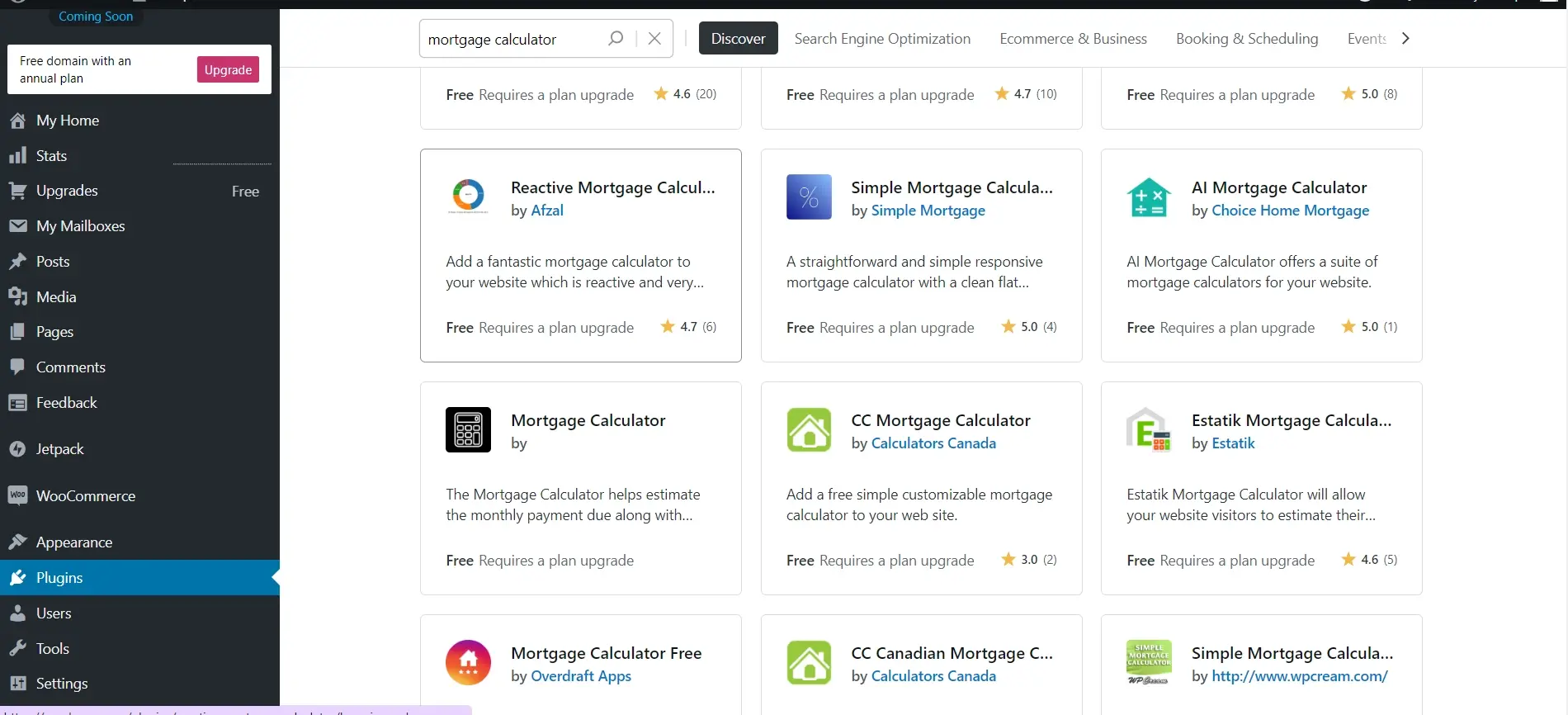
If you created a website using WordPress, add a mortgage calculator by going to the WordPress admin dashboard, navigating to plugins, searching for "mortgage calculator", choosing a calculator, and selecting install now to activate.
Go over to your plugins to check that it’s added, then go to the calculator setting to adjust the calculator options e.g. loan term, interest rate, currency, etc. depending on the type of calculator you choose.
After making changes to the settings, click on view details, you’ll see a short code provided by the plugin that would be used to plug the mortgage calculator into a page.
Copy the code, navigate to “pages” on the dashboard, create a new page to display the calculator, or add it to an existing page.
The short code is added in the content area, usually, there’s a + sign with the option “short code”. When you click on “short code” an input box is provided. This is where you paste the short code provided by the plugin and then publish or update the page. After publishing you can preview the page to see what it looks like on a webpage.
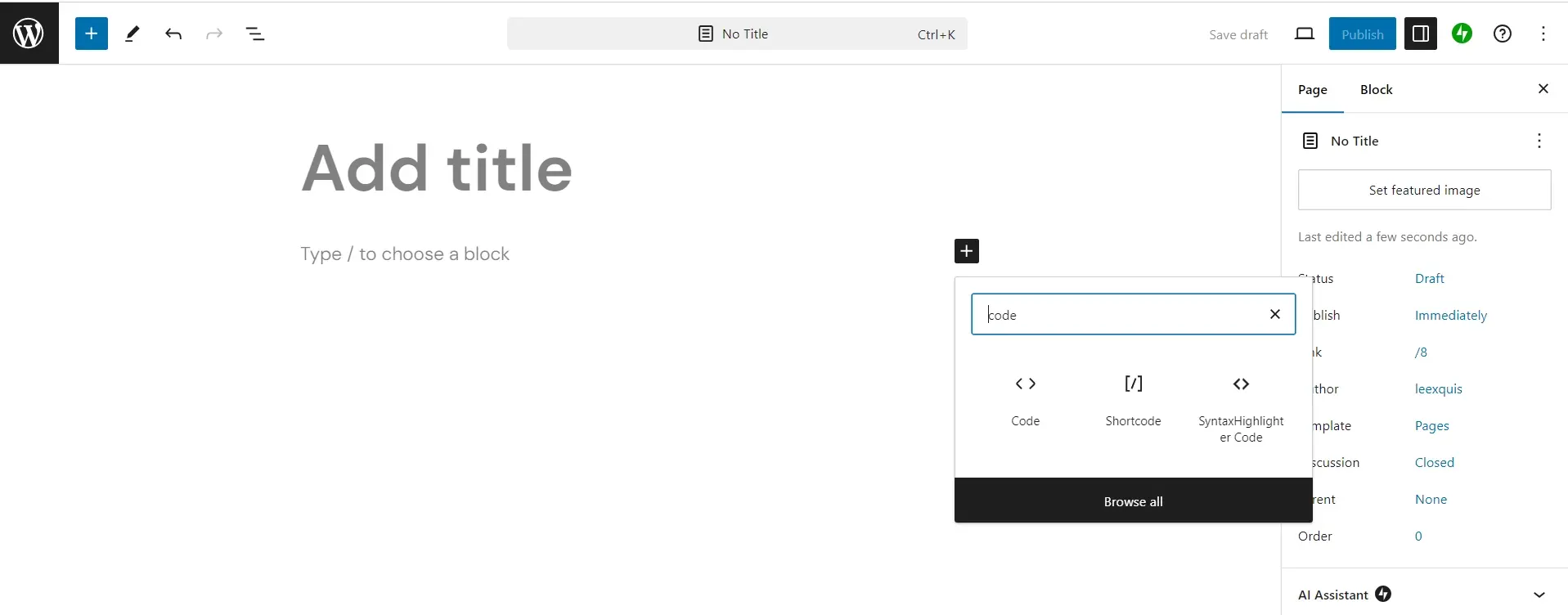
This is the easiest way to add a mortgage calculator to a web page, it’s not difficult to configure, and it can be applied by anyone without technical skills.
Custom HTML / JavaScript Code
Creating custom with HTML, CSS, and JavaScript requires the following;
Create a form where users can input loan amount, interest rate, loan term, and a button to trigger the calculator.
Style the form using CSS (Cascading Style Sheet) to make it more visually appealing.
Create a JavaScript functionality to capture user input and also create a formula for calculating monthly mortgage payments and displaying the result on the webpage.
Here’s a simple way to create a mortgage calculator, and add it to a website. In this case, we’ll assume we already have a website using ReactJs and want to add a mortgage calculator to the website.
This is what the website looks like;
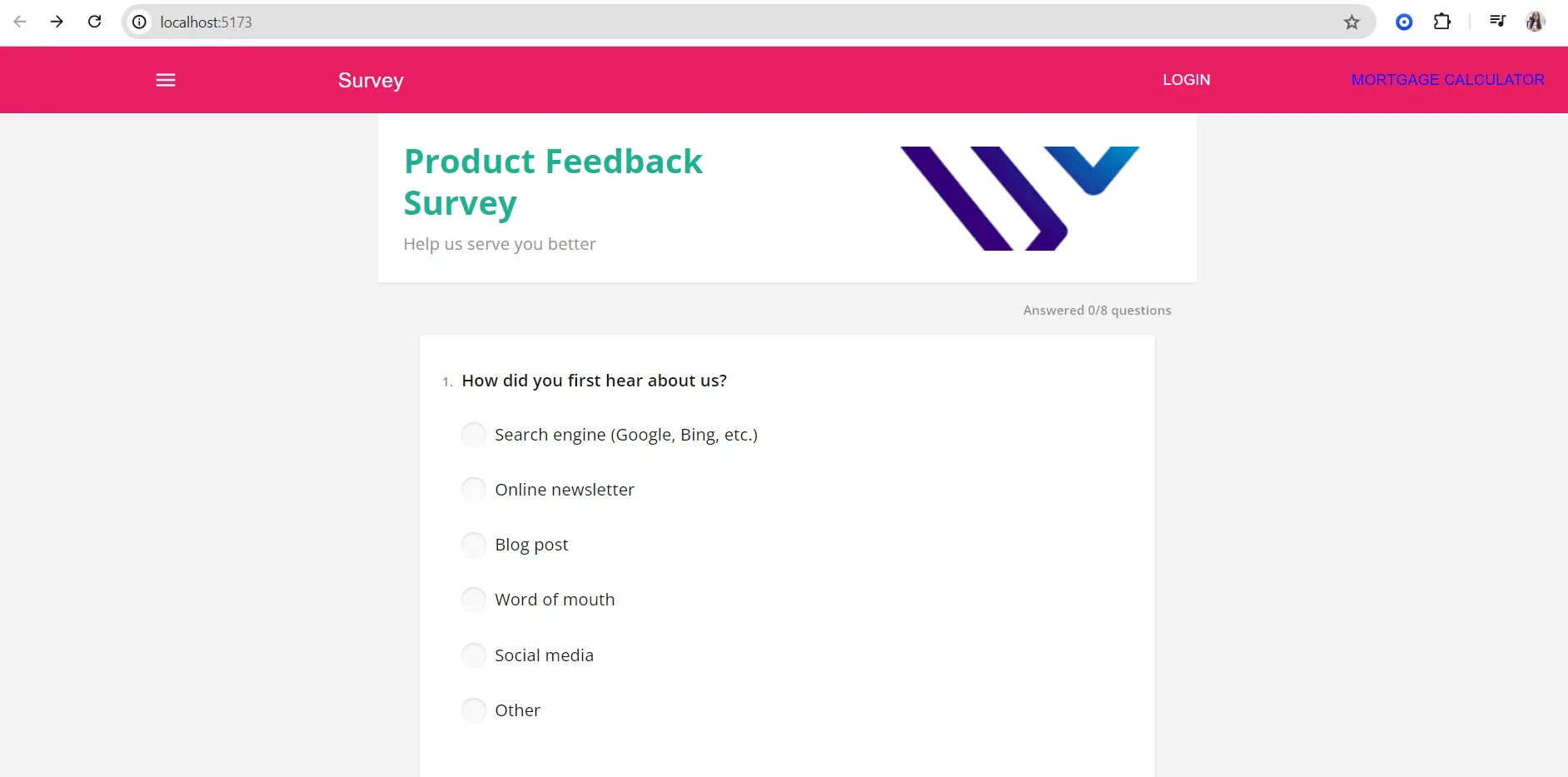
we added a link called “Mortgage Calculator” to the Menu bar. The mortgage calculator will be located in a component called Mortgage Calculator.
When we click on “Mortgage Calculator”, it will direct us to a URL of our local server with a path http://localhost:5173/calculator specifying the route (specific page) where our mortgage calculator resides.
To achieve this we used react-router-dom to create a path from the main page to the mortgage calculator page.
Here’s how to achieve this using createBrowserRouter and RouterProvider from react-router-dom.
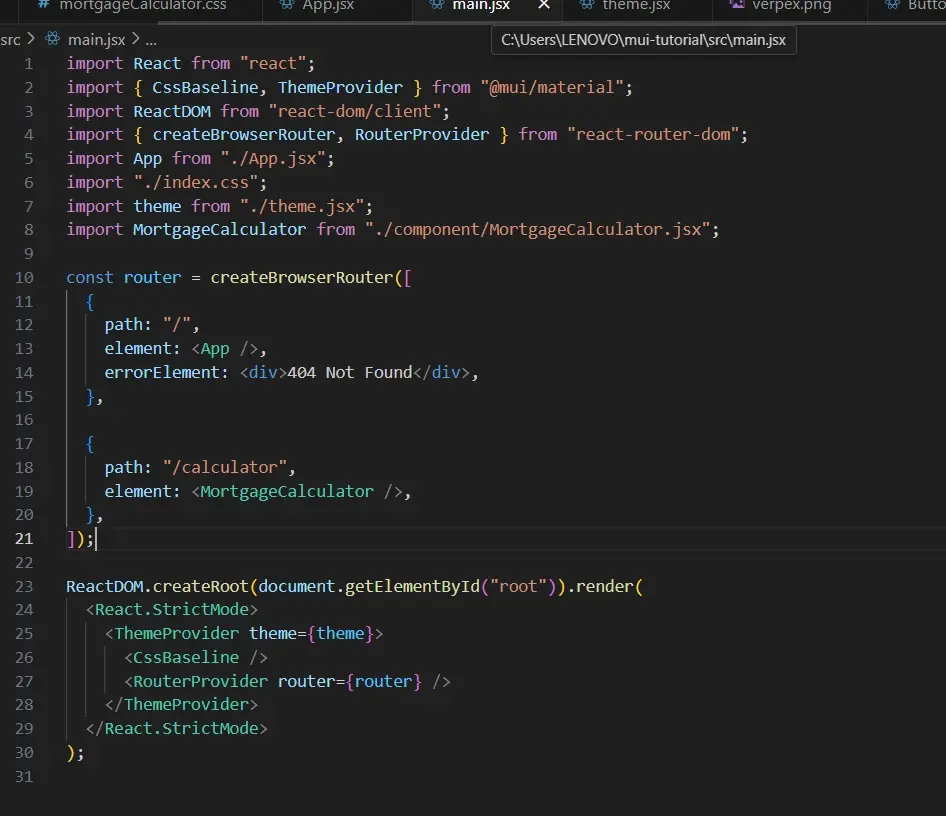
Import createBrowserRouter and RouterProvider from react-router-dom which is used to set up client-side routing in React applications, it allows users to navigate between different pages of an application
import { createBrowserRouter, RouterProvider } from "react-router-dom";
Import the mortgage calculator component to render it on a specific route.
import MortgageCalculator from "./component/MortgageCalculator.jsx";
Provide <RouterProvider router={router} />
to the root of your application enabling routing functionality according to specific paths.
Create a router using createBrowserRouter
with a path: /
(Entry point of our application), element
(which defines what users see when navigating different paths or links within the application), and errorElement
for error handling.
{
path: "/",
element: <App />,
errorElement: <div>404 Not Found</div>,
},
A calculator route with a path:/calculator, and element where we render the MortgageCalculator component.
{
path: "/calculator",
element: <MortgageCalculator />,
},
We'll use the link <Link> </Link>
component to help us navigate from the main page to the mortgage calculator page.
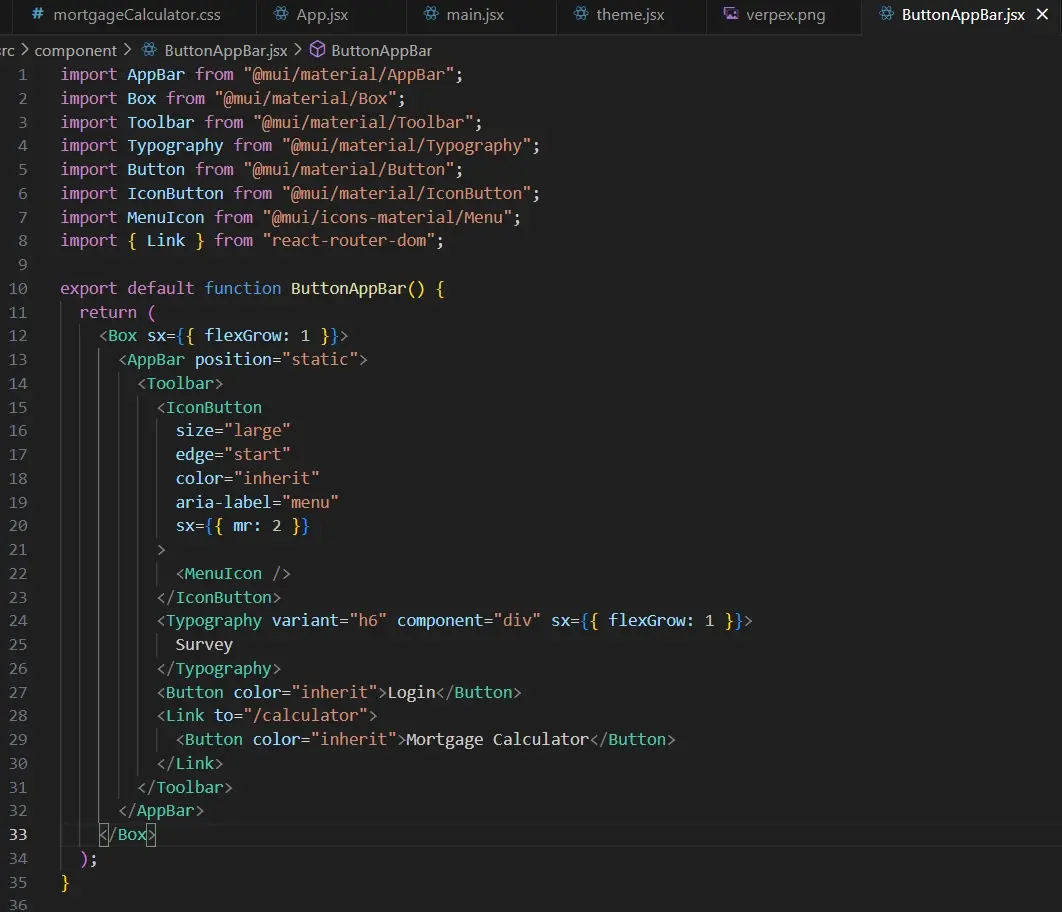
Import Link from “react-router-dom”
import {Link } from "react-router-dom";
Wrap the mortgage calculator button in <Link > </Link>
component
<Link to="/calculator">
<Button color="inherit">Mortgage Calculator</Button>
</Link>
The <Link to =/calculator >
specifies the path we want to navigate to which is the "mortgage calculator" on the main page. This is used instead of the <a>
tag we are familiar with when creating links in HTML.
Create a Simple Mortgage Calculator
Step One: Create a form in the MortgageCalculator component
Let’s create a form in the component called “mortgage calculator” like so;

See the code below;
<div className="container">
<div className="calculator-container">
<p>Mortgage Calculator</p>
</div>
<form onSubmit={handleSubmit}>
<div className="form-group">
<label htmlFor="propertyPrice">Property Amount</label>
<input
type="number"
placeholder="loan amount"
onChange={handleChange}
id="propertyPrice"
name="propertyPrice"
value={formData.propertyPrice}
/>
</div>
<div className="form-group">
<label htmlFor="interestRate">Interest Rate</label>
<input
type="number"
placeholder="interest rate"
onChange={handleChange}
id="interestRate"
name="interestRate"
value={formData.interestRate}
/>
</div>
<div className="form-group">
<label htmlFor="loanTerm">Loan Term (Years) </label>
<input
type="number"
placeholder="loan term"
onChange={handleChange}
id="loanTerm"
name="loanTerm"
value={formData.loanTerm}
/>
</div>
</div>
<button type="submit">Calculate</button>
</form>
</div>
This code contains a div which is the main wrapper for the form, it contains a;
paragraph
<p> </p>
element named "Mortgage Calculator" with a className "calculator-container" for stylingA form element that contains input fields with labels and a submit button. Inside the form element, different divs contain the label and input field with className "form-group".
Input elements - where the users type in data. It consists of ;
Type ( type="number") attribute that accepts only numeric values
Placeholder ( placeholder="monthly payment") - an instruction that tells the user what should be typed in the input field.
onChange ( onChange={handleChange}) attribute that listens for changes in the input field and calls the handleChange function when a user enters values in the input field.
Id ( id="monthlyPayment" ) attribute that assigns a unique to the input element which can be used for targeting the element e.g. for styling
Name attribute ( name="monthlyPayment" ) that assigns a name to the input element, it is a unique identifier for each input field in the form
Value attribute ( value={formData.monthlyPayment} )that binds the input field value to the property in the formData state.
Step 2: Style DIV
Add styling to the div element, here’s the CSS code below;
.container {
display: flex;
flex-direction: column;
align-items: center;
color: black;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.3);
width: 50%;
margin: 10% auto;
}
.calculator-container {
display: flex;
flex-direction: column;
align-items: center;
color: white;
padding: 20px;
margin-bottom: 20px;
border: 1px solid #ddd;
width: 100%;
font-size: 25px;
border-radius: 8px;
background-color: #45a049;
}
form {
display: flex;
flex-direction: column;
align-items: center;
}
.form-group {
display: flex;
flex-direction: column;
align-items: flex-start;
width: 100%;
max-width: 300px;
margin-bottom: 10px;
}
label {
margin-bottom: 5px;
color: black;
}
input {
width: 100%;
padding: 10px;
border: 1px solid #cccccccd;
border-radius: 4px;
}
button {
width: 100%;
max-width: 300px;
padding: 10px;
background-color: #51ba55;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
margin-top: 10px;
}
button:hover {
background-color: #45a049;
}
This is what the calculator looks like;
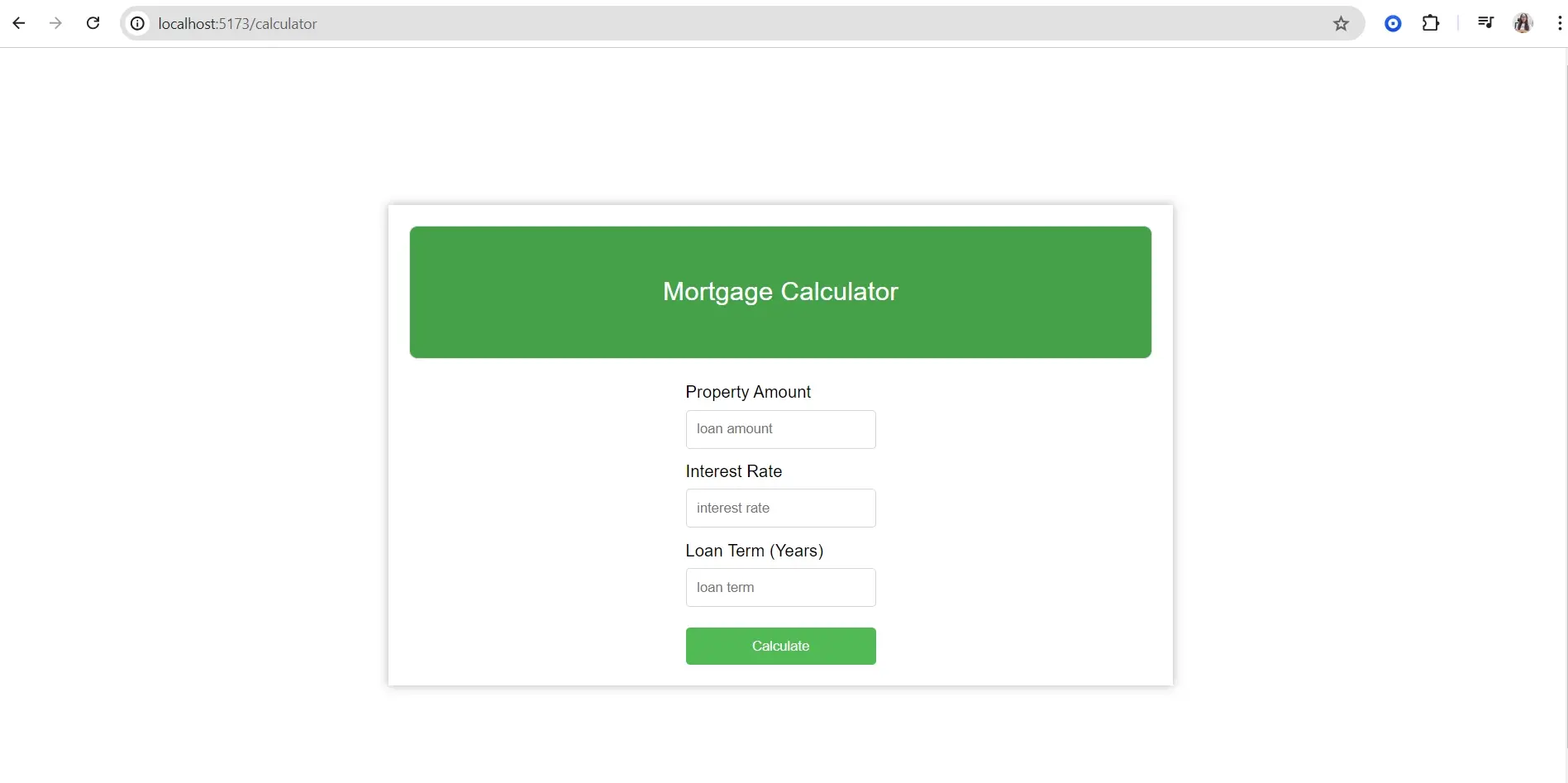
Step 3: Create state, handle change function, and handle submission for our form;
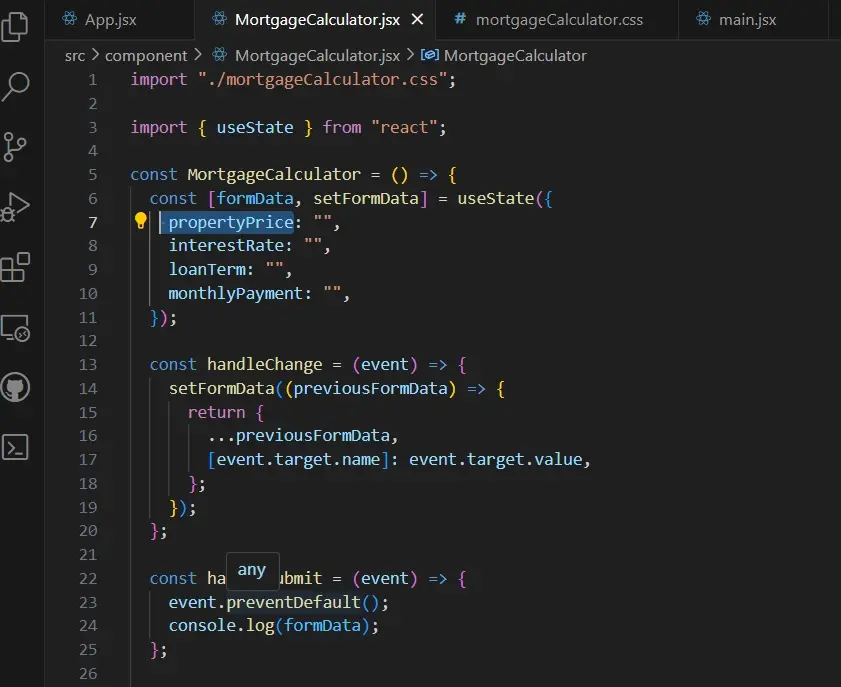
See code below;
const [formData, setFormData] = useState({
propertyPrice: "",
interestRate: "",
loanTerm: "",
monthlyPayment: "",
});
const handleChange = (event) => {
setFormData((previousFormData) => {
return {
...previousFormData,
[event.target.name]: event.target.value,
};
});
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(formData);
};
Code Explanation
Initialize State
- We use a "useState" hook to create a state variable name "formData and a setter function named "setFrormData to update the state.
- The initial state is an object with four properties namely propertyPrice: " ", interestRate: " ", loanTerm: " ", monthlyPayment: " ", with an empty string which will hold the values entered into the input field.
Handle Change Function
The handle change function is called when changes are applied in the input field
The (event) parameter is an object passed to the function when changes to the input are made.
The setFormData function updates the state. While the previousFormData receives the previousState receiving a new state object.
the ...previousFormData, copies existing state properties into the new state object.
event.target.name updates the properties in the state object based on the name attribute of the input field.
event.target.value is the value entered into the input field by the user.
Handle Form Submission
The handleSubmit function is executed when a user submits the form.
event.preventDefault () prevents default form submission behavior which usually refreshes the page.
console.log (formData) logs the current state of "formData" to the console, which is the values typed in the input field by the user. This is to check that the form is working as it should.
Step 4: Test
In the developer tool, we can check to see if the value typed in by the user is displayed in the console.
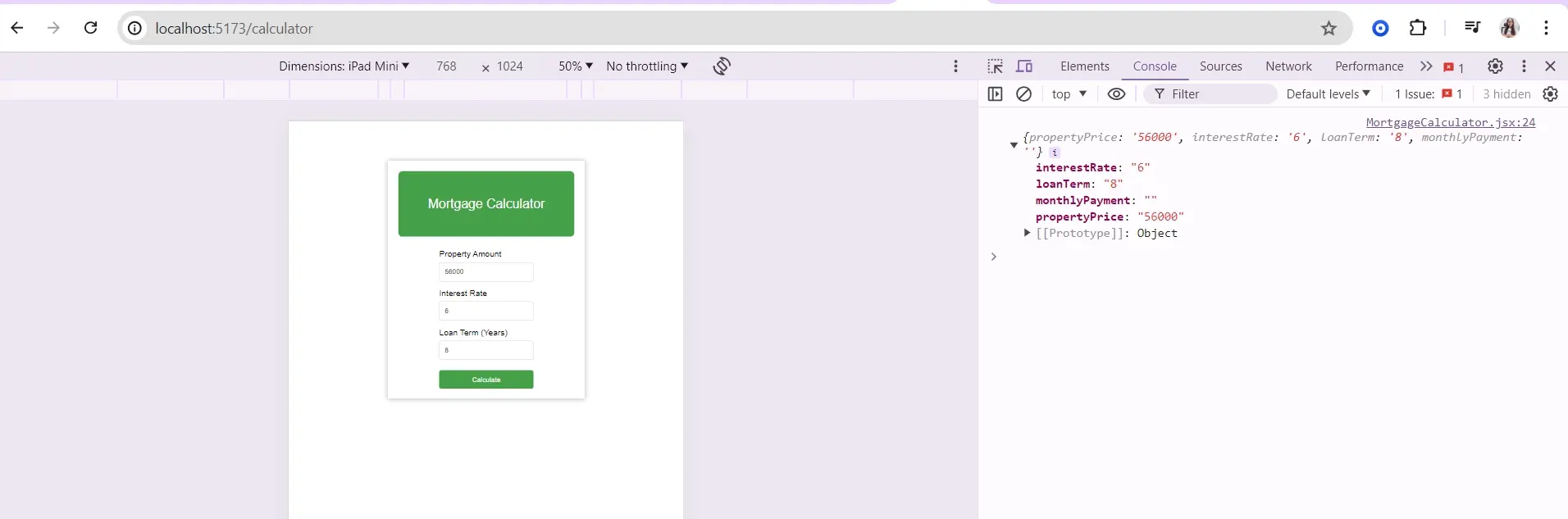
Step 5: Calculate Monthly Payment and Total Payment
To calculate monthly payment, let’s use the formula below;
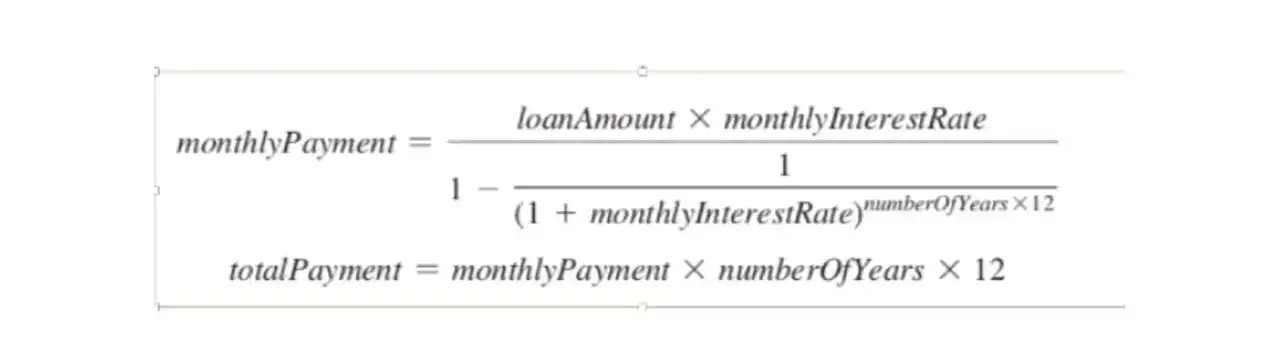
const monthlyPayment =
(principal * monthlyInterestRate) /
(1 - 1 / Math.pow(1 + monthlyInterestRate, loanTermYears * 12));
const totalPayment = monthlyPayment * loanTermYears * 12;
formulaSrc: https://gist.github.com/letrongtanbs/d29354da30f12784bc8453af4e4fb6ff
See code below;
const [formData, setFormData] = useState({
propertyPrice: "",
interestRate: "",
loanTerm: "",
monthlyPayment: "",
});
const handleChange = (event) => {
setFormData((previousFormData) => {
return {
...previousFormData,
[event.target.name]: event.target.value,
};
});
};
const handleSubmit = (event) => {
event.preventDefault();
console.log(formData);
monthlyPaymentCalculation();
};
const monthlyPaymentCalculation = () => {
const { propertyPrice, interestRate, loanTerm } = formData;
const principal = parseFloat(propertyPrice);
const annualInterestRate = parseFloat(interestRate);
const loanTermYears = parseFloat(loanTerm);
const monthlyInterestRate = annualInterestRate / 100 / 12;
const monthlyPayment =
(principal * monthlyInterestRate) /
(1 - 1 / Math.pow(1 + monthlyInterestRate, loanTermYears * 12));
const totalPayment = monthlyPayment * loanTermYears * 12;
setFormData((previousFormData) => ({
...previousFormData,
monthlyPayment: monthlyPayment.toFixed(2),
totalPayment: totalPayment.toFixed(2),
}));
};
What our code looks like in VS Code;
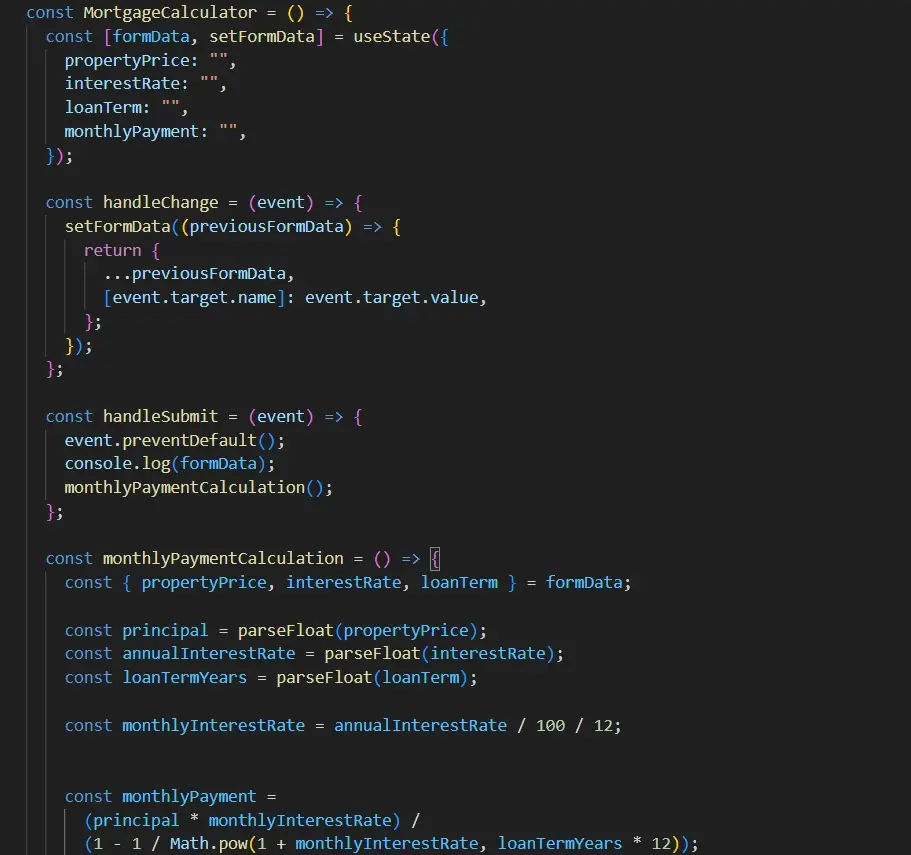
Test
Type in different values to check that the calculator works.

This is just a simple example of how to add a mortgage calculator to your website.
Summary
Adding a mortgage calculator to a website can be done differently, and there are several factors to consider like ease, complexity, type of loan, compliance, performance, etc.
Working with WordPress for instance makes it easier to add a mortgage calculator to a website, there’s also an option to embed an already-made mortgage calculator or create one from scratch.
Ultimately, these options are dependent on what the scope of the project is, and the preference of the owner or developer in question.
Frequently Asked Questions
Is a website on WordPress safe?
Websites on WordPress are safe, however to avoid hacking keep your website up to date.
Can you migrate my existing website over?
Yes, and without issue. Regardless of how many websites you’re managing, we’ll bring all of them under the Verpex wing free of charge. Just send us the details of your websites when you’ve signed up to get started.
What is AI for creating a website?
AI for creating a website refers to the use of artificial intelligence technologies to automate various aspects of the website development process, including design, content creation, and functionality.
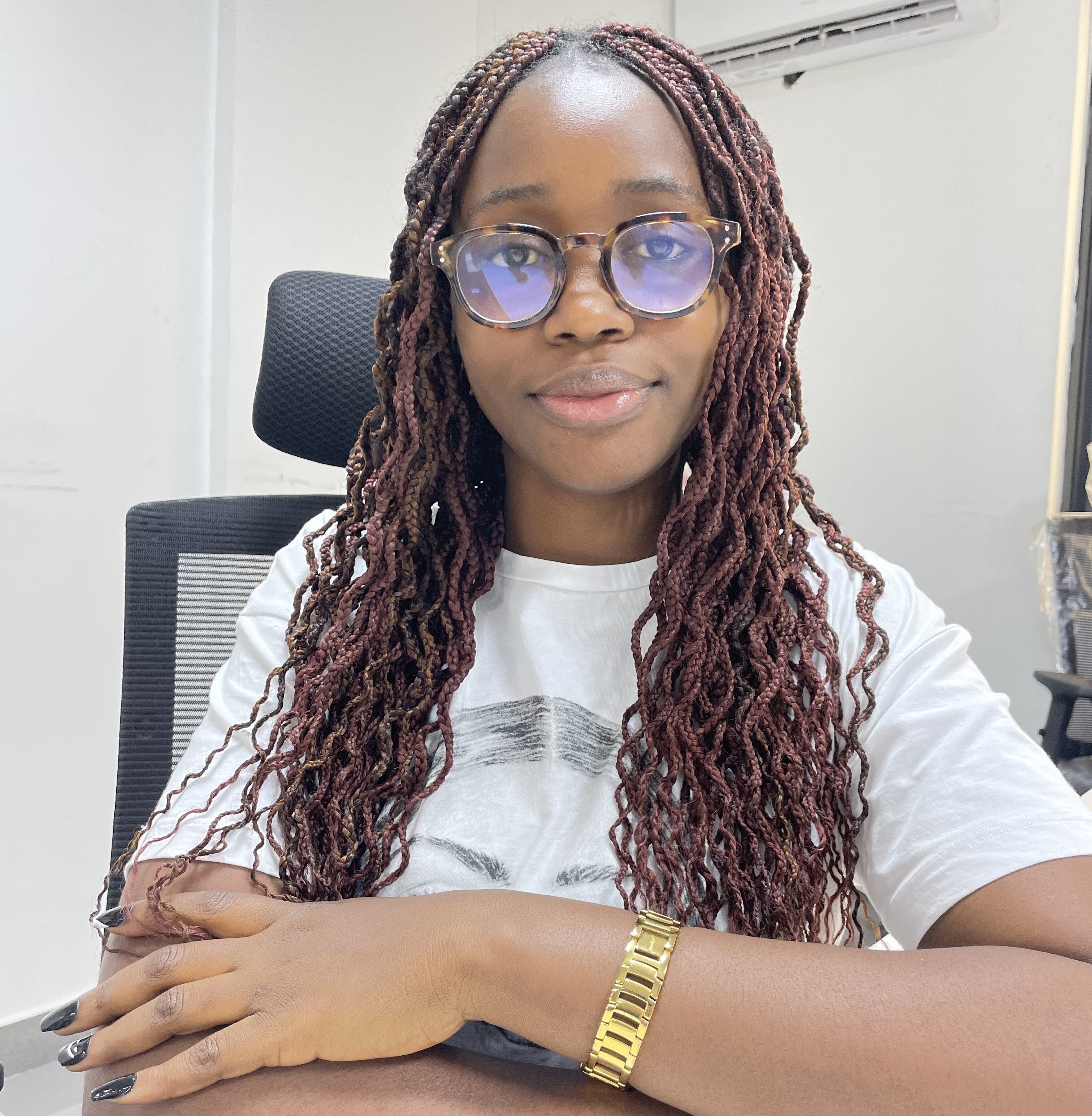
Jessica Agorye is a developer based in Lagos, Nigeria. A witty creative with a love for life, she is dedicated to sharing insights and inspiring others through her writing. With over 5 years of writing experience, she believes that content is king.
View all posts by Jessica Agorye