Cross-Site Request Forgery (CSRF) is a serious web security threat that exploits a user's browser trust, executing unauthorized actions such as compromising accounts, causing financial losses, identity theft, security bypass, and manipulating user data.
To stay safe, it is crucial to beef up security measures. Imagine a hacker tweaking request settings and using some social trickery to hijack a user account or even create an admin account, potentially causing chaos for your business. That's the kind of trouble CSRF attacks can brew.
This article will address how to protect against cross-site request forgery, emphasizing the collaborative effort needed to establish a secure online environment for all stakeholders.
Understanding Cross-Site Request Forgery
Cross-Site Request Forgery (CSRF) is a web security vulnerability where an attacker tricks an authenticated user into performing undesired actions on a web application.
This is achieved by executing malicious code or making unauthorized requests on behalf of the user, leading to actions like changing account settings, initiating financial transactions, or performing other sensitive operations.
In 2007, a CSRF vulnerability was discovered in Gmail. An attacker could send an email to a Gmail user with a malicious link. If the user clicks the link while logged into Gmail, the attacker could change the user's email settings without their knowledge.
How CSRF Works
The user logs into a web application and receives an authentication token or session cookie.
The attacker tricks the victim into accessing a web page containing malicious code or clicking on a crafted link.
The malicious code or link triggers a request to a vulnerable web application, using the victim's authenticated session. This request could be, for example, changing the victim's email address or password.
Since the request is sent with the victim's authenticated session, the web application may mistakenly perceive it as a legitimate action initiated by the authenticated user.
Types of Applications
1. Web Applications with State-Changing Operations
Any web application that relies on user authentication such as updating user profiles, changing account settings, or making financial transactions is vulnerable to CSRF attacks. An attacker can trick a user into unknowingly executing actions that modify the user's account settings or perform transactions without their consent.
2. Online Banking and Financial Transactions
Online banking platforms and financial transaction applications are high-value targets for CSRF attacks. An attacker could initiate fund transfers, change account details, or conduct unauthorized financial transactions using the victim's authenticated session.
3. Social Media Platforms
Social media platforms that allow users to post content, update profiles, or connect with others are often vulnerable to CSRF attacks. Attackers can manipulate a victim's social media account, posting unauthorized content, changing privacy settings, or connecting with malicious accounts.
4. Email and Messaging Systems
Email systems or messaging platforms allow users to change email settings, and passwords, or perform actions without reauthentication. An attacker can abuse a victim's email account to send malicious emails, change settings, or compromise the confidentiality of communication.
5. Content Management Systems (CMS)
CMS platforms that allow content creation, modification, or deletion. Attackers could manipulate website content, deface pages, or delete critical information if a user with administrative privileges falls victim to a CSRF attack.
Consequences of a Successful CSRF Attack
Unauthorized Actions: A successful CSRF attack allows an attacker to perform actions on a web application as if they were the authenticated user. This can include modifying account settings, changing passwords, or initiating transactions.
Data Manipulation: CSRF attacks can lead to unauthorized modification or deletion of data stored on a web application. This can result in data loss, data corruption, or the injection of malicious content.
Financial Loss: For applications dealing with financial transactions, CSRF attacks can lead to unauthorized fund transfers, purchases, or changes to payment information, resulting in financial losses for the victim.
Identity Compromise: CSRF attacks may compromise the identity of users, allowing attackers to impersonate them, post malicious content on their behalf, or engage in other activities that damage their online reputation.
Privacy Violations: CSRF attacks on applications involving communication, such as email or messaging systems, can result in privacy violations. Attackers may gain access to sensitive messages, contacts, or other private information.
Legal and Regulatory Consequences: Successful CSRF attacks can have legal and regulatory repercussions, especially if the compromised application involves sensitive data or compliance requirements. Organisations may face fines or legal action for failing to protect user data.
Identifying and understanding these risks is crucial for implementing effective countermeasures and mitigating the potential impact of CSRF attacks on various types of web applications.
Basic Principles of CSRF Protection
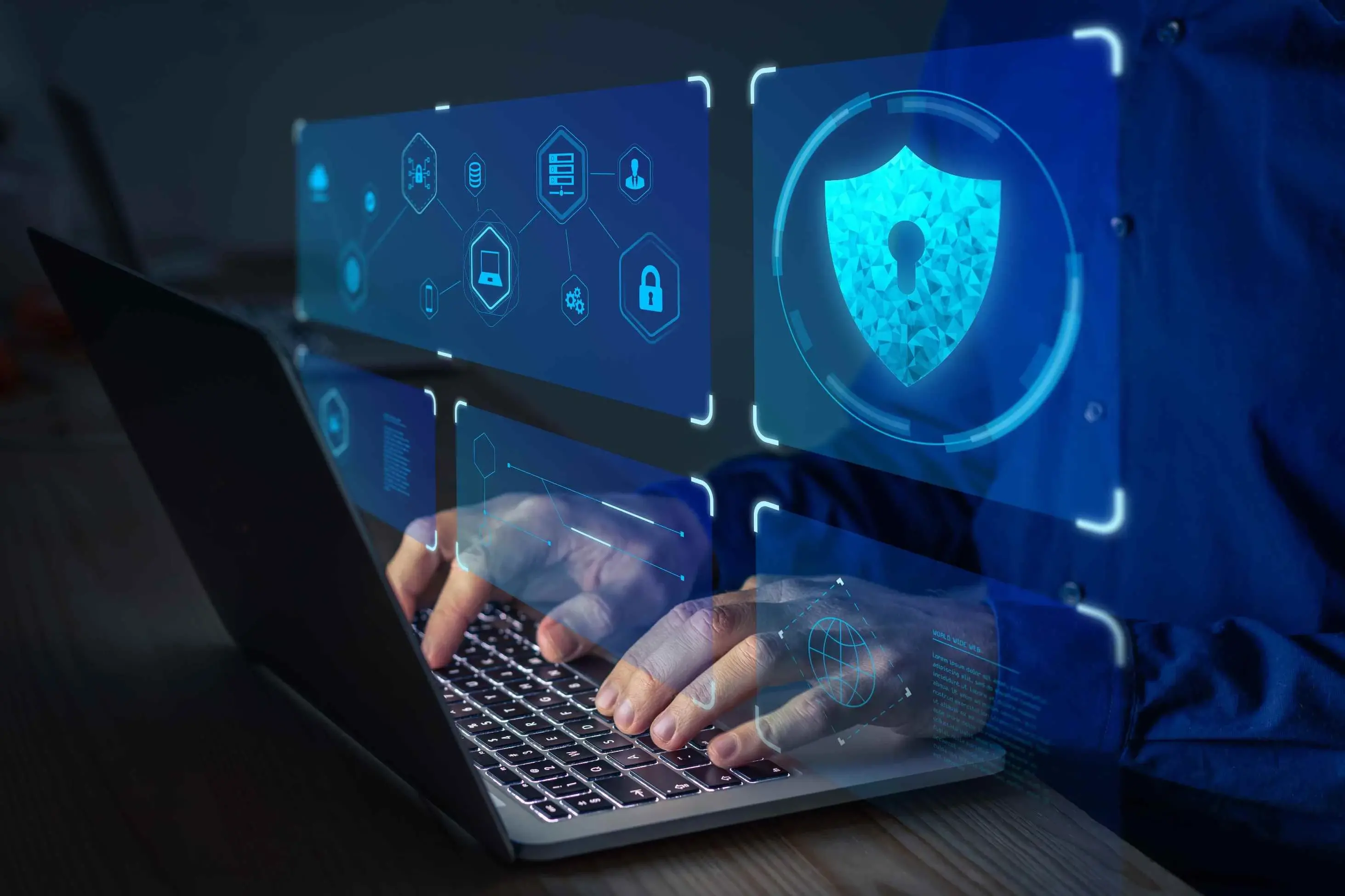
Anti-CSRF Tokens: Implement anti-CSRF tokens to validate the legitimacy of requests, preventing attackers from tricking users into executing unauthorized actions.
Same-Origin Policy: Leverage the Same-Origin Policy, a security measure implemented by web browsers. This policy restricts web pages from requesting a different domain than the one that served the web page. CSRF attacks often involve unauthorized requests from a different origin, and the Same-Origin Policy helps prevent such requests.
SameSite Cookie Attribute: Utilize the SameSite cookie attribute to control when cookies are sent with cross-site requests. Setting the SameSite attribute to "Strict" or "Lax" helps prevent cookies from being sent in cross-origin requests, reducing the risk of CSRF attacks.
Referrer Policy: Implement and enforce a strict Referrer Policy on the server side. Check the Referer header to ensure that requests are coming from expected sources. However, note that the effectiveness of this method relies on the browser sending the Referer header.
Content-Type Header Validation: Verify the Content-Type header of incoming requests to ensure that it matches the expected value for the specific request. This can help protect against certain types of CSRF attacks that rely on manipulating the request's content type.
Double-Submit Cookies: In addition to Anti-CSRF tokens, implement double-submit cookies. Set a cookie value that must match a corresponding value in the request parameters. This provides an additional layer of protection against CSRF attacks.
User Authentication and Authorization: Ensure robust user authentication and authorization mechanisms. Strong passwords, multi-factor authentication, and proper user privilege management can reduce the risk of unauthorized actions, even if a CSRF attack is attempted.
Security Headers: Utilize security headers, such as Content Security Policy (CSP), to to fortify overall web application security.
Implementing Anti-CSRF Tokens
Generate a unique and unpredictable token on the server side for each user session. This token should be associated with the user's session and stored securely.
Use a cryptographically secure random number generator to create a unique token for each user. The token can be a random string of characters, and its length should be sufficient to provide a high level of entropy.
Embed the generated token in HTML forms or include it in other request parameters, such as in the headers or as part of the request payload.
Include a hidden form field within HTML forms to store the anti-CSRF token. For example:
<form action="/process" method="post">
<input type="hidden" name="csrf_token" value="generated_token_here">
<!-- Other form fields -->
<button type="submit">Submit</button>
</form>
Use server-side code to dynamically include the anti-CSRF token in form templates. This ensures that each form has a unique token associated with the user's session.
For AJAX requests, include the anti-CSRF token in the headers or payload. This may involve modifying the JavaScript code responsible for making the requests.
var xhr = new XMLHttpRequest();
xhr.open("POST", "/process", true);
xhr.setRequestHeader("Content-Type", "application/json");
xhr.setRequestHeader("X-CSRF-Token", "generated_token_here");
xhr.send(JSON.stringify({ data: "example" }));
When processing a form submission or handling any other request, validate the anti-CSRF token on the server side. Compare the submitted token with the one stored in the user's session.
If the tokens do not match or if no token is provided, reject the request as it may be a potential CSRF attack. This validation should occur before processing any state-changing operations.
Example (in a web framework like Express.js)
const express = require('express');
const session = require('express-session');
const app = express();
// Middleware to generate and store anti-CSRF token in the session
app.use((req, res, next) => {
if (!req.session.csrfToken) {
req.session.csrfToken = generateCSRFToken();
}
next();
});
// Middleware to validate anti-CSRF token on each request
app.use((req, res, next) => {
const submittedToken = req.body.csrf_token || req.get('X-CSRF-Token');
if (submittedToken !== req.session.csrfToken) {
return res.status(403).send('Invalid CSRF token');
}
next();
});
// Route handling the form submission
app.post('/process', (req, res) => {
// Process the request only if the CSRF token is valid
res.send('Request processed successfully');
});
// Function to generate a random CSRF token
function generateCSRFToken() {
// Implementation to generate a random token
}
Utilizing SameSite Cookies to Prevent CSRF
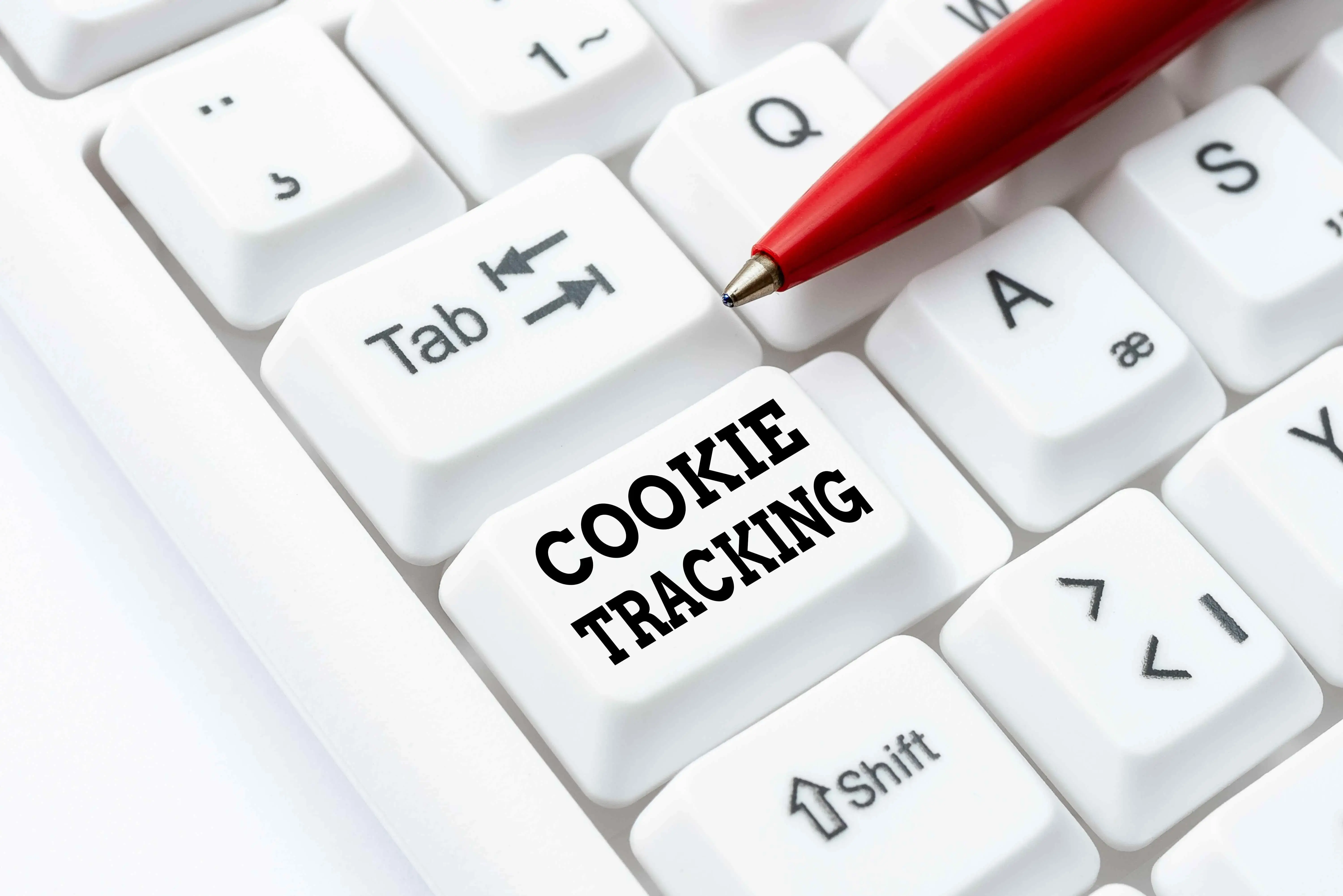
The SameSite attribute is a property that can be set for cookies to control their behaviour in cross-site requests. It helps mitigate the risk of CSRF attacks by preventing cookies from being sent in cross-origin requests, which are often exploited by attackers to impersonate users.
SameSite=None
When a cookie has the SameSite attribute set to "None," it can be sent in cross-origin requests initiated by third-party websites. However, this should only be done when the cookie is intended to be accessed across different sites, and the cookies is secure (transmitted over HTTPS).
SameSite=Lax or SameSite=Strict
When the SameSite attribute is set to "Lax" or "Strict," the cookie is not sent with cross-site requests initiated by third-party websites. This helps prevent CSRF attacks because even if an attacker manages to trick a user into requesting a malicious site, the browser won't include the SameSite=Lax or SameSite=Strict cookies in that request.
Configuring SameSite Cookies Correctly
- SameSite=None; Secure: Use this configuration when you have cookies that need to be accessed from different sites, and the cookie is transmitted securely over HTTPS. This is suitable for scenarios like cross-site single sign-on (SSO) where the cookie is shared across multiple sites.
Set-Cookie: myCookie=myValue; SameSite=None; Secure
- SameSite=Lax or SameSite=Strict: Use these configurations for cookies that should not be sent with cross-origin requests. This is suitable for cookies that are meant for site-specific operations and should not be accessible to third-party websites.
Set-Cookie: myCookie=myValue; SameSite=Lax
- Setting SameSite Attribute in Code (JavaScript): When setting cookies through client-side JavaScript, you can include the SameSite attribute:
document.cookie = "myCookie=myValue; SameSite=Lax";
Considerations
- Ensure that your website is served over HTTPS to use the "Secure" attribute effectively.
- Keep in mind that some older browsers may not fully support the SameSite attribute, so it is crucial to test and ensure compatibility.
- Regularly check and update your web application to address any changes in browser behaviour or security standards.
Double Submit Cookie Technique in CSRF Defense
The double submit cookie technique is a method used to mitigate Cross-Site Request Forgery (CSRF) attacks by associating a randomly generated token with both a cookie and a request parameter. The server verifies that these two tokens match during each request, providing an additional layer of security against CSRF exploits.
How the Double Submit Cookie Technique Works
A unique and unpredictable token is generated on the server side and associated with the user's session.
The token is then set as a cookie in the user's browser and included as a hidden field in HTML forms or as a parameter in request headers.
When the user submits a form or makes a request, the server checks that the token in the cookie matches the token in the request parameters.
If the tokens match, the request is considered legitimate, as it indicates that the user's browser is submitting a token associated with their session. If the tokens do not match or if no token is provided, the request is rejected to prevent potential CSRF attacks.
Implementing the Double Submit Cookie Technique
- Generate a unique token on the server side and associate it with the user's session.
const userToken = generateToken();
// Set the token as a cookie
res.cookie('csrf_token', userToken, { httpOnly: true, secure: true });
- Include the token in HTML forms as a hidden field.
<form action="/process" method="post">
<input type="hidden" name="csrf_token" value="userToken">
<!-- Other form fields -->
<button type="submit">Submit</button>
</form>
- Validate the token on the server side before processing the request.
const submittedToken = req.body.csrf_token || req.headers['x-csrf-token'];
const userToken = req.cookies.csrf_token;
if (submittedToken !== userToken) {
return res.status(403).send('Invalid CSRF token');
}
// Process the request since the CSRF token is valid
Security Considerations
- Ensure that your website is served over HTTPS to secure the transmission of cookies.
- Regularly update and patch your web application to address any security vulnerabilities.
- Consider the overall security of your web application, including other measures like SameSite cookie attributes and anti-CSRF tokens.
Leveraging Framework-Specific CSRF Protection
Web development frameworks often come with built-in features to address common security issues, including Cross-Site Request Forgery (CSRF). Here are examples of CSRF protection features in popular web development frameworks:
Django (Python)
Django includes a middleware called django.middleware.csrf. CsrfViewMiddleware that adds protection against CSRF attacks. This middleware generates and validates a CSRF token for each form submission.CSRF protection is enabled by default in Django. You can use the {% csrf_token %} template tag in your HTML forms to include the CSRF token.
<form method="post" action="/submit">
{% csrf_token %}
<!-- Other form fields -->
<button type="submit">Submit</button>
</form>
Ruby on Rails
Rails provides built-in CSRF protection in controllers through the protect_from_forgery method. This method includes a CSRF token in forms and verifies its presence in non-idempotent requests.
In your application controller, include the protect_from_forgery line.
class ApplicationController < ActionController::Base
protect_from_forgery with: :exception
end
ASP.NET (C#)
ASP.NET provides an AntiForgery class and the ValidateAntiForgeryToken attribute to protect against CSRF attacks. The ValidateAntiForgeryToken attribute can be applied to controller actions to ensure the presence and validity of an anti-forgery token.
In your controller, apply the ValidateAntiForgeryToken attribute to actions that require CSRF protection.
[ValidateAntiForgeryToken]
public ActionResult SubmitForm(FormModel model)
{
// Process the form submission
}
Express.js (Node.js)
The csurf middleware in Express.js helps protect against CSRF attacks by generating and validating anti-CSRF tokens. It adds a csrfToken property to the request object.
Install the csurf middleware and include it in your Express.js application.
const csrf = require('csurf');
const csrfProtection = csrf({ cookie: true });
app.use(csrfProtection);
app.get('/form', (req, res) => {
res.render('form', { csrfToken: req.csrfToken() });
});
These are just a few examples of CSRF protection features in popular web development frameworks. When using a framework, it is essential to understand and leverage its built-in security features to enhance the overall protection of your web application against common vulnerabilities like CSRF.
Best Practices in User Authentication and Session Management
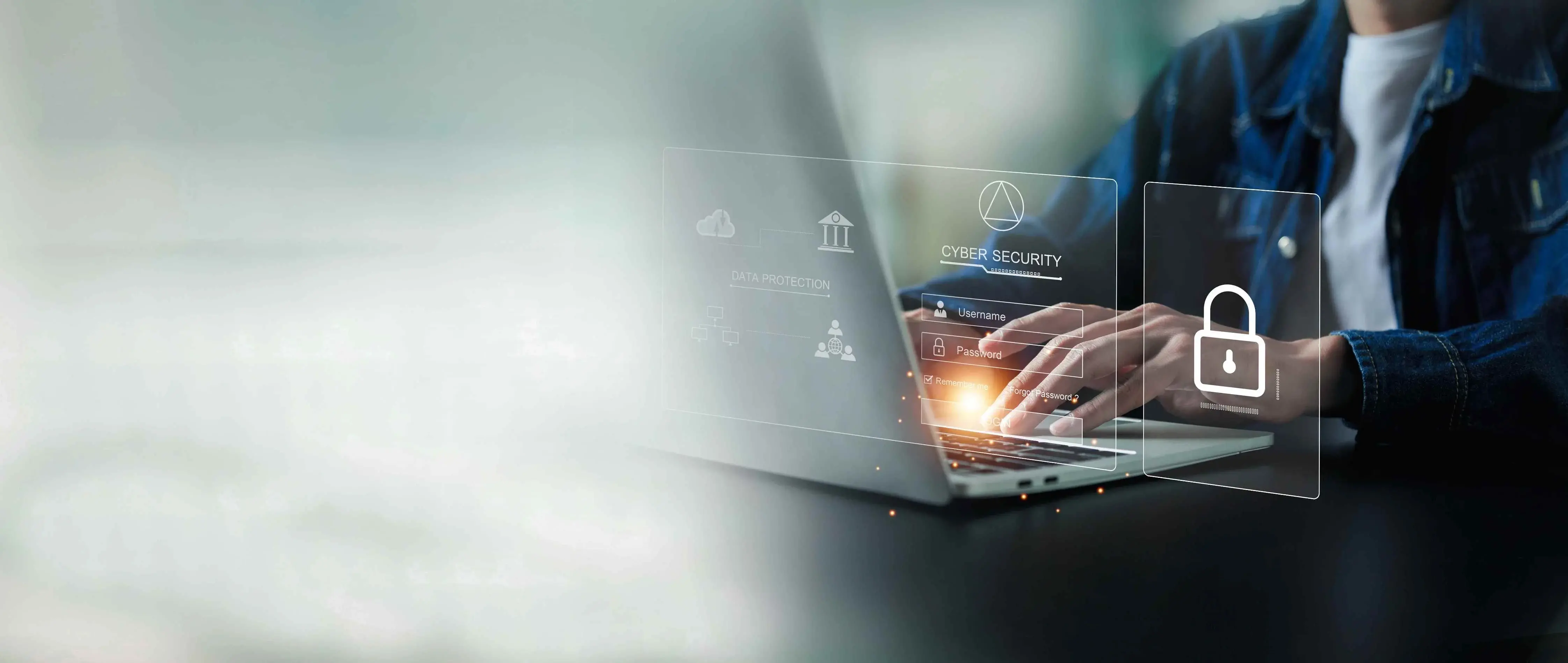
1. Secure Authentication
- **Use Strong Password Policies: Enforce the use of strong and unique passwords. Encourage users to create passwords with a mix of uppercase and lowercase letters, numbers, and special characters.
- **Multi-Factor Authentication (MFA): Implement multi-factor authentication to add an extra layer of security. MFA requires users to provide multiple forms of identification, such as a password and a temporary code sent to their mobile device.
- **Account Lockout Policies: Implement account lockout policies to protect against brute force attacks. Lock user accounts after a specified number of failed login attempts, preventing attackers from guessing passwords.
2. Session Management
- **Use Secure Session Cookies: Ensure that session cookies are set with secure attributes. Cookies should be marked as HTTP-only and secure, meaning they are only transmitted over HTTPS connections, reducing the risk of interception by attackers.
- **Implement Session Timeout: Set a reasonable session timeout to automatically log users out after a period of inactivity. This reduces the window of opportunity for attackers to exploit an active session.
- **Regenerate Session IDs: Regenerate session IDs after a user logs in or changes their authentication status. This helps mitigate session fixation attacks, where an attacker sets a user's session ID to a known value.
3. Anti-CSRF Measures
- **Use Anti-CSRF Tokens: Implement anti-CSRF tokens as an additional layer of defense. These tokens should be associated with user sessions and submitted with each request. Verify the token's validity on the server side before processing any state-changing operations.
- **SameSite Cookie Attribute: Utilize the SameSite cookie attribute to control when cookies are sent in cross-site requests. Setting it to "Lax" or "Strict" helps prevent CSRF attacks by limiting cookie transmission to same-site or first-party requests.
4. Regular Security Audits
- **Conduct Regular Security Audits: Regularly audit your authentication and session management mechanisms for vulnerabilities. Perform security assessments, code reviews, and penetration testing to identify and address potential weaknesses.
- **Stay Informed About Security Best Practices: Keep up-to-date with the latest security best practices and standards. Follow security blogs, subscribe to relevant mailing lists, and participate in the security community to stay informed about emerging threats and mitigation techniques.
5. User Education
- **Educate Users About Security Best Practices: Provide users with information on security best practices. Encourage them to use strong passwords, enable multi-factor authentication, and be cautious about clicking on links or accessing sensitive information over unsecured networks.
- **Notify Users of Suspicious Activity: Implement mechanisms to notify users of suspicious activity, such as multiple failed login attempts or logins from unfamiliar locations. This empowers users to take action if their accounts are compromised.
A holistic approach to security that combines technical measures with user education is crucial for building robust defenses against various threats.
Importance of Regular Security Testing and Audits for CSRF Vulnerabilities
1. Manual Testing: Conduct manual tests to identify CSRF vulnerabilities by analyzing how the application handles user sessions and processes requests. Manually submitting forms with manipulated tokens and analyzing server responses can reveal potential vulnerabilities.
2. Automated Scanning Tools: Use automated scanning tools like OWASP ZAP, Burp Suite, and Acunetix to identify common CSRF vulnerabilities. These tools can help identify and report potential issues by simulating various attack scenarios.
3. Code Reviews: Perform regular code reviews with a focus on security. Analyze the application's source code to identify potential vulnerabilities related to how it handles session management and anti-CSRF token implementation.
4. Penetration Testing: Conduct penetration testing to simulate real-world attack scenarios. Ethical hackers can attempt to exploit CSRF vulnerabilities by manipulating requests and exploiting weaknesses in the application's session management and anti-CSRF defenses.
5. Security Headers Analysis: Analyze security headers, such as Content Security Policy (CSP), to ensure they are configured correctly. Properly configured headers can help prevent various types of attacks.
6. Framework-Specific Tools: Some web development frameworks offer specific tools for security testing. For example, Django Debug Toolbar for Django applications provides insights into potential CSRF vulnerabilities during development.
7. Regular Security Audits: Conduct regular security audits that include a comprehensive examination of the application's security controls. This involves reviewing configuration settings, access controls, and the effectiveness of anti-CSRF measures.
8. Compliance Scanning: Use compliance scanning tools that focus on specific security standards (e.g., PCI DSS, HIPAA) to ensure that the application meets regulatory requirements related to CSRF and session management.
9. Threat Modeling: Implement threat modeling to identify and assess potential CSRF threats and attack vectors. This proactive approach helps organizations anticipate and address security concerns during the design and development phases.
By combining manual testing, automated tools, code reviews, and other methodologies, organizations can effectively identify and mitigate CSRF vulnerabilities, enhancing the security and trustworthiness of their web applications.
Conclusion
Protecting web applications against CSRF is an ongoing process that requires a combination of technical measures, user education, and adherence to security best practices. Proactive and comprehensive security measures are crucial in maintaining the integrity, confidentiality, and availability of web applications
This approach not only builds user trust but also guards against evolving cybersecurity threats. Organizations can significantly enhance the security posture of their web applications and reduce the risk of CSRF attacks by adopting these strategies
Frequently Asked Questions
What is the difference between a CSRF token and a JWT (JSON Web Token)?
CSRF tokens are used to protect against Cross-Site Request Forgery attacks by validating the origin of requests, while JWTs are authentication tokens often used for user identity verification.
How does a CSRF attack differ from a Cross-Site Scripting (XSS) attack?
CSRF involves unauthorized actions performed on behalf of a user, leveraging their active session, whereas XSS injects malicious scripts into a website to target other users.
Can CSRF protection mechanisms impact the performance of a web application?
Yes, they can add overhead due to token generation and verification, potentially impacting performance.
How do Content Security Policies (CSP) help in mitigating CSRF risks?
CSP helps by restricting the sources from which a page can load resources, preventing the execution of malicious scripts injected via CSRF.
Are there any specific challenges in implementing CSRF protection in single-page applications (SPAs)?
Yes, SPAs face challenges due to dynamic content loading and the need to ensure proper token handling during asynchronous operations.
How should CSRF tokens be securely stored and managed on the client side?
CSRF tokens should be stored in a secure, HttpOnly cookie to prevent access by malicious scripts and transmitted over secure channels.
Are mobile applications vulnerable to CSRF attacks in the same way as web applications?
Yes, mobile applications can be susceptible to CSRF attacks, especially if they use web-based authentication methods.
How can I ensure third-party plugins or libraries used in my application do not introduce CSRF vulnerabilities?
Regularly update and review third-party components, ensuring they follow secure coding practices and do not introduce CSRF vulnerabilities.
Is it necessary to implement CSRF protection for APIs, and if so, how does it differ from standard web applications?
Yes, it is necessary. API protection often involves token-based authentication and validation, similar to web applications.
Can CORS (Cross-Origin Resource Sharing) settings affect the efficacy of CSRF protections?
Yes, proper CORS settings can enhance CSRF protection by controlling which domains are allowed to make requests.
How do I handle CSRF protection in a distributed or microservices-based architecture?
Ensure consistent implementation of CSRF protection across microservices and use centralized mechanisms for token validation.
What are some common mistakes developers make when implementing CSRF protection?
Common mistakes include inadequate token validation, improper storage, and failure to protect against token leakage.
How frequently should CSRF tokens be rotated or refreshed in a session?
Regularly refreshing CSRF tokens adds an extra layer of security; the frequency depends on the application's sensitivity.
How do stateless applications handle CSRF protection differently from stateful ones?
Stateless applications often rely on token-based authentication for CSRF protection, while stateful ones may use session-based mechanisms.
Can browser extensions or add-ons assist in protecting against CSRF attacks?
Browser extensions can enhance security, but they are not a primary defense against CSRF; proper application-level protection is crucial.
What role do HTTP methods (GET, POST, PUT, DELETE) play in CSRF attacks and protection strategies?
CSRF attacks often target state-changing HTTP methods; protection involves validating tokens and ensuring sensitive actions use secure methods.
How does CSRF protection work in serverless architectures?
In serverless architectures, CSRF protection is still necessary and often involves token validation and secure handling of stateless requests.
Can implementing a CAPTCHA system help in preventing CSRF attacks?
While CAPTCHA can add an extra layer of security, it is not a direct solution for CSRF prevention.
What are some emerging trends or technologies in web security that might impact CSRF protection strategies in the future?
Emerging trends may include advanced tokenization methods, AI-driven threat detection, and more sophisticated browser security features.
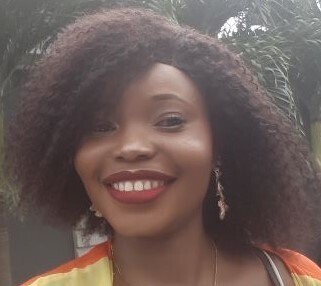
Yetunde Salami is a seasoned technical writer with expertise in the hosting industry. With 8 years of experience in the field, she has a deep understanding of complex technical concepts and the ability to communicate them clearly and concisely to a wide range of audiences. At Verpex Hosting, she is responsible for writing blog posts, knowledgebase articles, and other resources that help customers understand and use the company's products and services. When she is not writing, Yetunde is an avid reader of romance novels and enjoys fine dining.
View all posts by Yetunde Salami