AJAX is one of those terms we often hear in the world of web development. The term can be confusing at first because AJAX is not a programming language or a stand-alone product. Instead, it’s a technology that uses existing languages and technologies in a new way. As a result, it speeds up certain tasks on your website and improves the user experience. Fortunately, AJAX isn’t difficult to use with WordPress. In this post, we’ll explore what AJAX is, see how it works, help you decide if or when you need it, and see how to implement it on your WordPress website.
What is AJAX?
AJAX, or Asynchronous JavaScript and XML, is a programming concept that describes web development techniques to create asynchronous web applications. This allows a website to send and receive data from the server in the background without having to refresh the page. It now uses JSON in place of XML, but no one wants to change the name to AJAJ, so we still call it AJAX.
The information can be marked up and styled with HTML and CSS and can be dynamically displayed with JavaScript. Users can receive and interact with that information dynamically. This adds a higher level of quality and usability to the website. For more information about dynamic websites, see the Verpex article Static vs Dynamic Website.
Why We Need AJAX (with a brief history of the web)
AJAX was created to solve the problem of page loading on HTML websites. In the early 1990s, every time something on the page changed, including interactions where a user made a choice, the entire page was reloaded. This greatly limited bandwidth and server load.
To help with this, Internet Explorer introduced the iFrames tag in 1996. It worked asynchronously and paved the way for AJAX in 1998 which appeared in Internet Explorer 5.0 in 1999. It then appeared in most of the popular web browsers. Google and several other companies started using it and it became popular among developers. W3C began standardizing AJAX in 2005. The latest standardization is from 2016. With AJAX as a standard, it can work cross-browser and cross-platform to deliver a seamless user experience.
One of the main advantages of adding AJAX to a WordPress website is it makes the website more user-friendly. For more information about making your website user-friendly, see the article User-Friendly Website Tips here on the Verpex blog.
Data Transfer Without AJAX
Without AJAX, the process of transferring the data to the user would look like this:
User interaction with the browser (such as a search)
The HTTP request is sent to the server
The server gets the data from the database
HTML and CSS are sent to the browser
The browser refreshes to show the new HTML page
Data Transfer With AJAX
With AJAX, the process of transferring the data to the user would look like this:
User interaction with the AJAX engine (such as a search)
The AJAX engine requests data from the server
The server gives the data from the database
The data is sent to the AJAX engine
The browser shows the information along with the proper HTML and CSS data without refreshing the page
Why AJAX is Better
At first, the number of steps seems to be the same. However, the for AJAX steps are simpler, faster, and more efficient. The web browser doesn’t need to rebuild the entire page with all of the HTML and CSS to create it. Only the data in the AJAX engine (such as a search result) is refreshed. The amount of data transferred is reduced and the data transfer is faster.
The Concept of AJAX
AJAX isn’t a markup or programming language. Instead, it’s a technology concept. As a concept, AJAX uses several current technologies that implement applications that interact with the server in the background. So, instead of being a programming language, it uses programming languages. Here are some of the main technologies that make AJAX work and what they do:
XMLHttpRequest
This is the object used for asynchronous communication between the browser and the server.XML or JSON
This is used for data interchange. Most modern AJAX implementations use JSON (JavaScript Object Notation) in place of XML (Extensible Markup Language). You can also use text files or preformatted HTML.DOM (Document Object Model)
Dynamically displays the data and the interaction with that data.HTML and CSS
Hyper Text Markup Language and Cascading Style Sheets work together to present the data to the user.JavaScript
This brings all the technologies together.
With AJAX, events are data-driven rather than page-driven. It can be triggered with a button, an enter key, or even a mouse hover.
Technologies That Use AJAX
Anyone that’s spent more than a few days on the web has interacted with AJAX. If you’ve interacted with something and received some sort of data without the page refreshing, chances are high that you’ve interacted with AJAX. AJAX is everywhere.
For example, if you enter text into the search box here at the Verpex blog you’ll see some search suggestions appear in the search box as you type. The search engine is asynchronously sending your inquiry to the server and displaying options for you to choose from. This search box uses AJAX to provide suggestions, which makes the search faster and more efficient for the user. This feature is only viable with technology like AJAX.
You can see this in the image below. I’ve searched for WordPress and the search box has provided lots of article titles in the Verpex blog for me to choose from. This happened immediately without the page reloading.
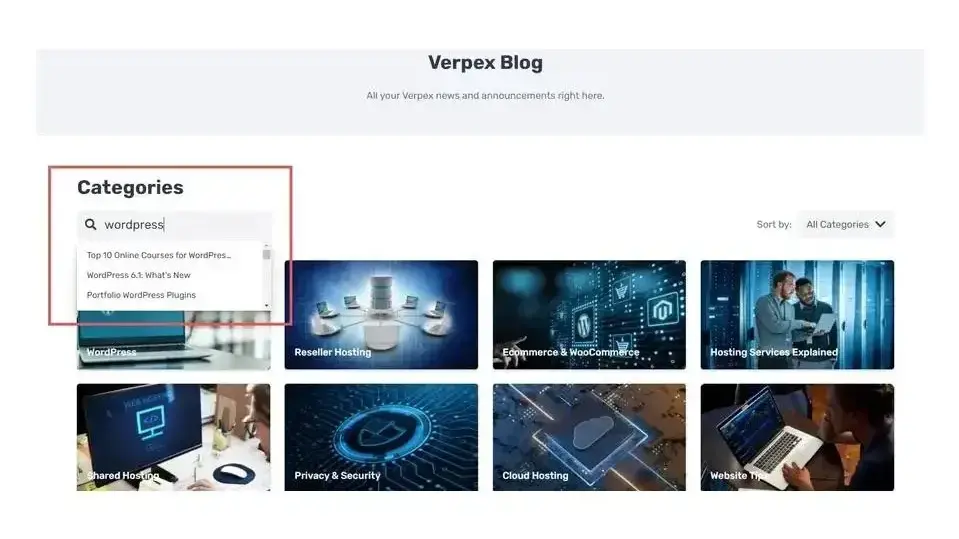
There are many other uses for AJAX that we encounter daily. Web technologies that utilize AJAX include:
Login forms
Shopping carts
Search boxes with auto-suggest
Product filter
Contact forms
Infinite scroll
Comments
Chat
Calendar
Load more button
Add to cart
Mini cart
Navigation
Pagination
Site reviews
Maps
File upload
Domain checker
Hits counter
Popular posts
Polls
Surveys
Product variation swatches
Photo gallery
Product tables
Wishlist
Job listings
Font directories
Status updates
Notifications
This list shows just a few of the many uses of AJAX with WordPress.
How to Implement AJAX in WordPress
AJAX can be implemented in several ways with WordPress websites. It can be coded manually into your content (such as a Code Block), or you can find plugins that add or use AJAX, or AJAX could be coded into your child theme.
Coding AJAX in WordPress
Coding AJAX requires knowledge of HTML, CSS, JSON, and JavaScript. I only recommend hand-coding AJAX if you’re familiar with these markup and programming languages. Unless you’re a programmer and want to code your own products, choosing plugins with AJAX features is a better option.
A full tutorial on coding with AJAX would take more than what we can cover in this article, but we’ll step through a simple example. To show how it works, let’s look at the example from W3schools.
First, we need something to interact with on the front end. This can be a form or many other types of interactive elements. W3Schools uses HTML to display text and a button with this code:
<!DOCTYPE html>
<html>
<body>
<div id="demo">
<h2>Let AJAX change this text</h2>
<button type="button" onclick="loadDoc()">Change Content</button>
</div>
</body>
</html>
You can see the text and button in the image below.

When the user clicks the button, it runs the function “loadDoc ()”. This function requests data from the server. You can see the function below.
function loadDoc() {
var xhttp = new XMLHttpRequest();
xhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("demo").innerHTML = this.responseText;
}
};
xhttp.open("GET", "ajax_info.txt", true);
xhttp.send();
}
The function gets the data from the server and displays it in place of the original text and removes the button, as you can see in the image below. This happens quickly without refreshing the page.
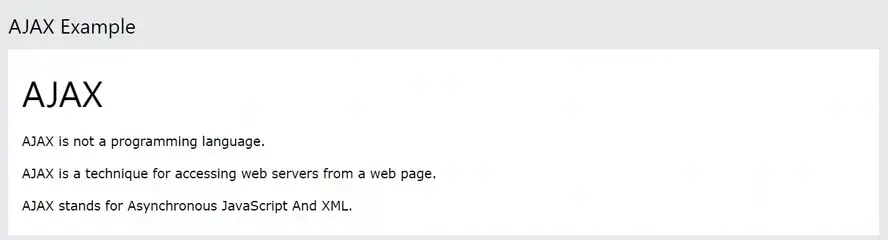
7 Most Popular WordPress Plugins with AJAX
The easiest way to add AJAX to your WordPress website is by using a plugin that already includes it. For example, rather than using an element, such as a form, and then adding AJAX to it, simply find a form plugin that includes AJAX.
There are many WordPress plugins available that include AJAX. Here’s a look at a few of the most popular. I’ve selected plugins in a wide range of categories. There are a lot more in many categories, but this list should give you an idea of the types of plugins that are available with AJAX. To find more, search the WordPress repository for the type of plugin you need and include the keyword “AJAX”.
1. FiboSearch – Ajax Search for WooCommerce
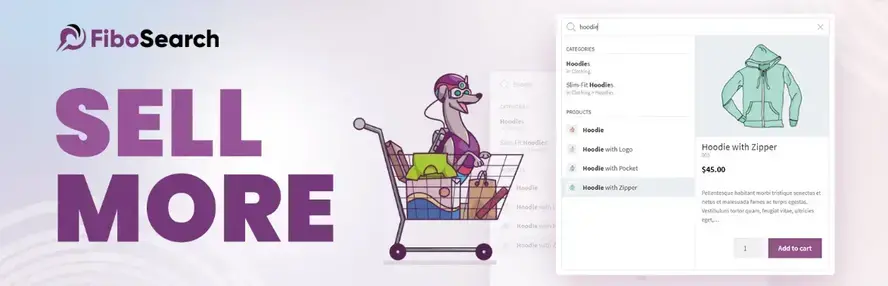
FiboSearch is a smart and powerful WooCommerce search with live product suggestions. It can search by title, long and short descriptions, categories, and tags. The results display the product image, price, description, and SKU. The search results are automatically grouped by type. It supports Google Analytics and multilingual services such as WPML, Polylang, and qTranslate-XT. Customize the search bar and autocomplete suggestions.
2. WordPress Infinite Scroll – Ajax Load More
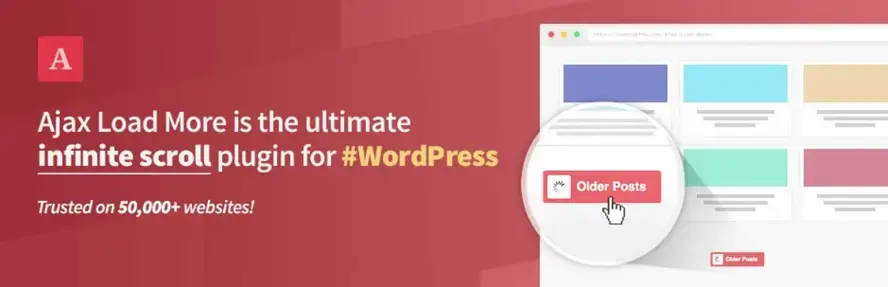
WordPress Infinite Scroll provides a lazy load for infinite scrolling for posts, pages, comments, etc. It can query based on the content type including the post type, category, date, tags, custom taxonomies, authors, search terms, and more. You can place multiple “load more” instances on a single page, create a custom template that matches your website, and filter the results. It’s multisite compatible.
3. Side Cart WooCommerce (Ajax)
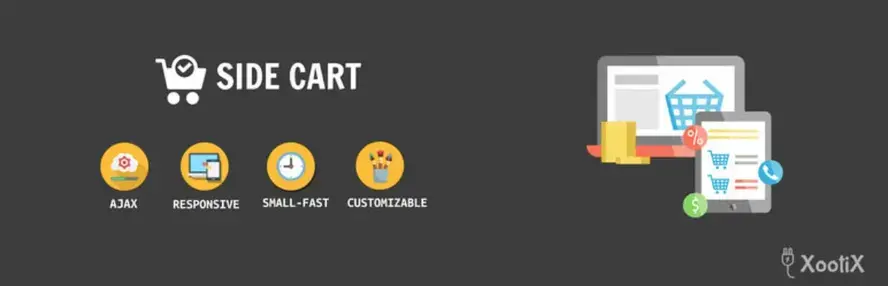
Side Cart WooCommerce allows users to access their shopping cart from any page. Users can add or remove items from their carts from anywhere. It’s fully customizable so you can choose what to show in the cart, hide the cart on specific pages, and design the look of the cart.
4. Login With Ajax
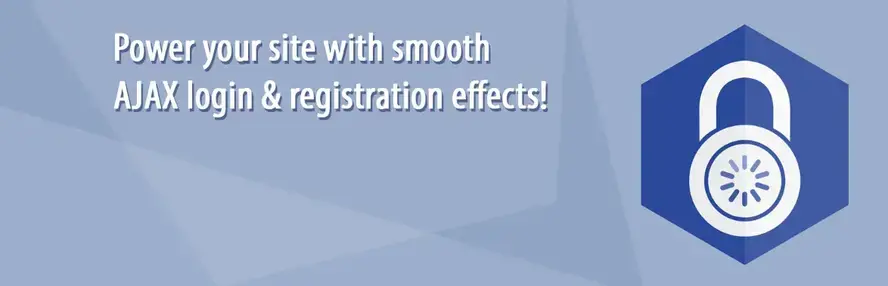
Login With Ajax adds a login widget to the sidebar and Gutenberg blocks that make logging in quick and easy. Features include login, registration, and password remember and reset. It includes multiple templates to choose from, modals and popups, individual base color options, and several display methods including blocks and shortcodes. You can also redirect the user if you want. Redirect includes custom URLs and URLs based on the user roles.
5. WooCommerce Ajax Cart Plugin

WooCommerce Ajax Cart Plugin changes how the standard WooCommerce cart page works. When the buyer changes the quality of the product, the total price is recalculated automatically. This keeps them from having to update the cart to see the new total. It includes plus and minus buttons so they can easily change the quantity. It displays a confirmation if they choose a quantity of zero.
6. Comments – wpDiscuz
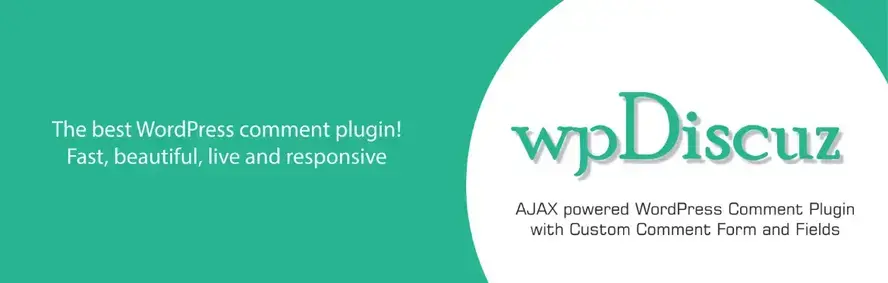
wpDiscuz is a real-time commenting system with a custom form and fields. An interactive commenting box is added to your content types, and it includes inline commenting and feedback. Allow or disallow commenting on specific posts or post types. Other features include social commenting with social login options, post rating, comment list sorting, nested comments, lazy loading, multiple line breaks, author notifications, anti-spam, and lots more. It has three layouts to choose from.
7. Simple Ajax Chat
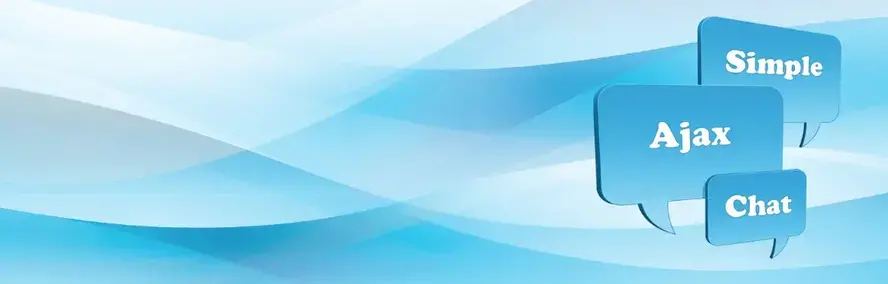
Simple Ajax Chat adds an interesting way for your site visitors to chat with each other on your website. The chat can be placed anywhere with a shortcode, and you can customize the style to match your website with CSS. It includes anti-spam website security. There are lots of options to choose from such as banning certain words, playing a sound alert, enabling browser notifications, sorting, custom content placement, and more.
Ending Thoughts
That’s our look at AJAX for the web. AJAX is a technology that brings HTML, CSS, JSON, and JavaScript together in a new way to improve web interaction. AJAX is more efficient than running web applications without it. Since it’s asynchronous, AJAX can get data from the server and update the page without having to reload the page.
Only the requested data updates, so the rest of the page does not need to refresh. Because of this, web applications that use AJAX are faster and reduce server load.
We want to hear from you. Do you use AJAX with your WordPress website? Let us know about your experience in the comments.
And if you want to ensure your WordPress website is built on a solid platform, consider using Verpex web hosting. Our hosting plans for WordPress are optimized for speed and excellent performance.
Frequently Asked Questions
Do I need web developer skills to use Shopify or WooCommerce?
No. Both platforms welcome beginners, but you might get more out of WooCommerce’s extensions if you’ve got some previous experience.
Do I need extensive developer skills to operate BuddyPress?
No, you don't need extensive developer skills to operate BuddyPress. It's designed to be user-friendly, with many features accessible through a graphical interface.
That said, basic knowledge of WordPress and some familiarity with coding can help you customize and extend its functionality.
Are WordPress plugins free?
WordPress has loads of plugins you can install, some of them are free, but some of them you will need to pay for. You can learn how to use WordPress Plugins on our blog.
How do I keep WordPress plug-ins up to date?
In most cases, your plug-ins will scan for updates automatically, but it’s always worth logging into your dashboard on a regular basis and performing a manual scan. This can usually be done in just a few clicks.
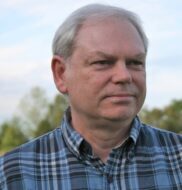
Randy A. Brown is a freelance writer from east TN specializing in WordPress and eCommerce. He's a longtime WordPress enthusiast and loves learning new things and sharing information with others. If he's not writing or reading, he's probably playing guitar.
View all posts by Randy A. Brown