Real-time communication on the web refers to the ability of web applications to deliver and receive information instantly, without noticeable delays. This capability is essential for applications like messaging platforms, online gaming, collaboration tools and financial systems.
What are WebSockets?
WebSockets are a communication protocol that establishes a bidirectional, full-duplex communication channel over a single, long-lived connection between a client (typically a web browser) and a server. This enables real-time, asynchronous communication, allowing data to be sent between the client and server in both directions at any time after the initial connection.
This communication protocol is useful for applications that require low-latency and real-time updates, such as chat applications, online gaming, financial trading platforms, and live data streaming.
Technical Overview of WebSockets
Handshake: The communication begins with a WebSocket handshake initiated by the client, usually through a standard HTTP request. The server responds to this handshake, and once the connection is established, it remains open, facilitating ongoing communication.
Full-Duplex Communication: WebSockets allow data to be sent in both directions simultaneously. Either the client or the server can initiate communication, making it suitable for applications that require low latency and real-time updates.
Data Frames: Data in WebSockets is transmitted in small, independent frames. These frames can carry various types of data, and the lightweight structure allows for efficient communication.
Low Latency: WebSockets significantly reduce latency compared to traditional HTTP connections, as there is no need to repeatedly establish connections for each request-response cycle. This makes WebSockets ideal for applications where timely updates are critical.
Comparison Between WebSockets and Traditional HTTP Connections
Feature | WebSockets | Traditional HTTP |
---|---|---|
Communication Model | Bidirectional, full-duplex | Request-Response |
Connection Persistence | Persistent | Stateless (new connection for each request) |
Latency | Lower latency due to a continuous open connection | Higher latency, as a new connection is established |
Data Format | Supports multiple data formats (text, binary) | Typically uses JSON or XML |
Overhead | Lower overhead, as headers are minimal | Higher overhead, due to headers in each request |
Usage | Real-time applications (chat, gaming, live updates) | Standard web applications, static content |
Scalability | Scalable with a large number of concurrent connections | Scalable, but may require additional infrastructure |
Firewalls and Proxies | Can be affected; some may block WebSocket connections | Generally supported, as HTTP traffic is more common |
Usage Scenarios | Interactive and dynamic applications | Static content, forms, occasional dynamic elements |
Implementation Complexity | Requires handling events, but can simplify real-time apps | Simpler, as it follows a request-response model |
WebSockets are well-suited for real-time applications, while traditional HTTP is often sufficient for static content and occasional dynamic interactions. Some applications may even use a combination of both technologies depending on the use case.
The Role of WebSockets in Real-Time Communication
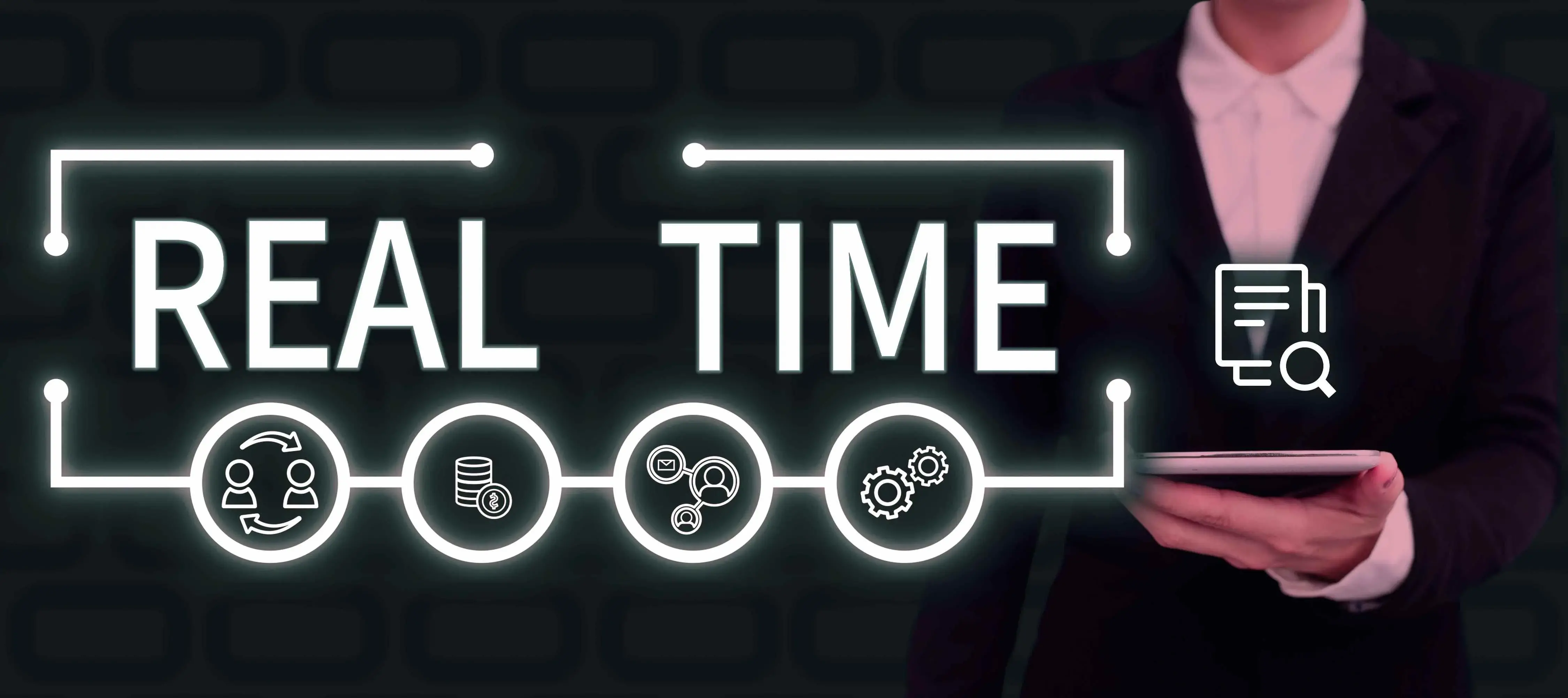
WebSockets plays a crucial role in enabling real-time communication on the web by providing a bidirectional, full-duplex communication channel over a single, long-lived connection.
This design is particularly advantageous for applications requiring instant and seamless data transfer between clients and servers.
How WebSockets Facilitate Real-Time Data Transfer
Persistent Connection: WebSockets establish a persistent connection between the client and server, eliminating the need to repeatedly open and close connections for each exchange of data. This persistent connection significantly reduces latency, making it well-suited for real-time scenarios.
Bidirectional Communication: WebSockets support full-duplex communication, meaning that both the client and server can send messages independently at any time. This bidirectional flow of data enables instant updates and responses without waiting for a request or predefined intervals.
Low Latency: The elimination of connection setup overhead and the ability to send data in real-time contribute to low-latency communication. This is particularly advantageous for applications where timely information is critical, such as live chats, collaborative editing, online gaming, financial trading platforms, and more.
Advantages of Using WebSockets
Reduced Latency
WebSockets significantly reduce latency compared to traditional HTTP connections. The persistent connection allows for instant communication without the need to repeatedly establish connections for each data exchange.
Bi-Directional Communication
WebSockets facilitate full-duplex communication, allowing both the client and server to send messages independently. This bidirectional flow of data supports interactive and dynamic web applications.
Collaborative tools, like Google Docs, utilize WebSockets to enable multiple users to edit the same document simultaneously. Changes made by one user are instantly propagated to others, creating a seamless collaborative experience.
Scalability
WebSockets are highly scalable, making them suitable for applications with a large number of concurrent connections. The ability to handle numerous simultaneous connections efficiently is crucial for applications with widespread usage.
Social media platforms use WebSockets to manage real-time notifications, comments, and updates for millions of users simultaneously. This ensures that users receive timely information without overloading the server.
Efficient Resource Utilization
WebSockets use a single, long-lived connection, reducing the overhead associated with repeatedly opening and closing connections. This efficient resource utilization is advantageous for both servers and clients.
Online multiplayer games leverage WebSockets to enable real-time player interactions. The persistent connection ensures that game events are communicated instantly, enhancing the gaming experience without unnecessary resource consumption.
Cross-Platform Compatibility
WebSockets are supported by modern web browsers and are compatible with various programming languages, making them a versatile choice for building real-time applications that can run on different platforms.
Messaging applications like WhatsApp use WebSockets to provide real-time chat across different devices and platforms. Users can seamlessly switch between devices without losing the continuity of their conversations.
Implementing WebSockets
Below is a basic guide on how to set up a WebSocket connection using JavaScript. This example will cover both the client-side and server-side implementations.
Client-Side (JavaScript):
- Create a new WebSocket instance by providing the server's WebSocket URL.
- Listen for the 'open' event to confirm that the connection has been successfully established.
- Send messages to the server using the send method.
- Listen for the 'message' event to receive and handle messages from the server.
- Listen for the 'close' event to handle the case when the connection is closed.
// Create a WebSocket connection
const socket = new WebSocket('ws://example.com/socket');
// Connection opened
socket.addEventListener('open', (event) => {
console.log('Connected to WebSocket');
// Send a message to the server
socket.send('Hello Server!');
});
// Listen for messages from the server
socket.addEventListener('message', (event) => {
console.log('Message from server:', event.data);
});
// Connection closed
socket.addEventListener('close', (event) => {
console.log('Connection closed');
});
Server-Side (Node.js using the ws library):
- Create a WebSocket server using the ws library.
- Handle incoming connections using the 'connection' event.
- Listen for 'message' events to receive and handle messages from clients.
- Send responses back to clients using the send method.
- Handle the 'close' event to manage client disconnections.
const WebSocket = require('ws');
const server = new WebSocket.Server({ port: 3000 });
// Handle incoming WebSocket connections
server.on('connection', (socket) => {
console.log('Client connected');
// Listen for messages from the client
socket.on('message', (message) => {
console.log('Message from client:', message);
// Send a response back to the client
socket.send('Hello Client!');
});
// Handle disconnection
socket.on('close', () => {
console.log('Client disconnected');
});
});
Challenges and Considerations in WebSocket Implementation
Security Concerns
WebSockets can be vulnerable to security issues, including cross-site scripting (XSS) attacks and potential abuse of open connections.
To mitigate this, implement secure coding practices, validate and sanitize user input, use secure WebSocket protocols (wss://), and employ authentication mechanisms to ensure that only authorized users can establish connections.
Browser Compatibility
While modern browsers generally support WebSockets, there might be variations in implementation or issues with older browser versions.
To ensure cross-browser compatibility, check and test WebSocket functionality on different browsers. Additionally, provide fallback mechanisms, such as using alternative communication methods (like long polling), for browsers that don't support WebSockets.
Network Constraints
Firewalls and proxy servers might restrict WebSocket connections, especially in corporate networks.
To enhance the likelihood of successful connections,cConfigure WebSocket connections to work over standard ports (80 for HTTP, 443 for HTTPS). It is important to communicate with network administrators to ensure that WebSocket traffic is allowed through firewalls.
Scaling and Load Balancing
Scaling WebSocket applications to handle a large number of concurrent connections can be complex.
To address this, utilize load balancing techniques to distribute WebSocket connections across multiple servers. Additionally, consider implementing a message broker or a distributed architecture to effectively manage state and synchronize data between servers.
Resource Management
Maintaining a large number of open connections can strain server resources.
To address this challenge, implement connection limits, enforce proper resource cleanup on disconnections, and consider implementing connection pooling. Additionally, optimize server configurations to effectively handle a high volume of concurrent connections.
Limited Protocol Support
Not all intermediaries (like load balancers and proxies) fully support the WebSocket protocol.
To address this, work with WebSocket-aware infrastructure components, and if needed, configure proxies to support WebSocket connections. Additionally, implement WebSocket protocol negotiation mechanisms to handle situations where WebSocket connections may need to be upgraded from HTTP.
WebSockets vs. Other Real-Time Technologies
Feature | WebSockets | AJAX | Long Polling | Server-Sent Events (SSE) |
---|---|---|---|---|
Communication Model | Bidirectional, full-duplex | Unidirectional, initiated by client | Unidirectional, initiated by client | Unidirectional, initiated by server |
Connection Persistence | Persistent | Short-lived, reopens for each request | Can be long-lived or short-lived, depending on server response | Persistent |
Latency | Low latency | Moderate latency, higher than WebSockets | Moderate latency, lower than traditional polling | Low latency |
Data Format | Supports multiple data formats (text, binary) | Typically JSON or XML | Typically JSON or XML | Text-based, supports only text data |
Overhead | Lower overhead, minimal headers | Moderate overhead due to headers in each request | Moderate to high overhead due to request-response cycle | Moderate overhead due to headers in each response |
Usage Scenarios | Real-time applications, interactive features | Asynchronous updates, dynamic content | Real-time applications, chat, gaming | Streaming updates, real-time notifications |
Scalability | Scalable with a large number of concurrent connections | Scalable but may require additional infrastructure | Scalable, but challenges with a large number of open connections | Scalable with a large number of concurrent connections |
Event-Driven Model | Yes | Yes | Yes | Yes |
Browser Support | Widely supported in modern browsers | Supported in all browsers | Supported in most modern browsers | Widely supported in modern browsers |
When to Use | Real-time applications, live data updates | Asynchronous updates, dynamic content | When real-time updates are crucial but WebSockets are not supported | Real-time notifications, streaming updates |
Consider Alternatives When | Compatibility with older browsers is a concern | Lower-level of real-time requirement, compatibility issues | Compatibility with older browsers is a concern | Need a simpler setup, and real-time updates are not critical |
WebSockets are often preferred for applications that require low-latency, bidirectional communication, and real-time updates. However, alternative technologies like AJAX, Long Polling, and Server-Sent Events may be suitable for specific use cases based on the characteristics mentioned in the table.
Future of Real-Time Communication with WebSockets
Widespread Adoption in Various Industries
The use of WebSockets is likely to expand across diverse industries like healthcare, education, IoT (Internet of Things), and more.WebSockets offer a reliable and efficient means of real-time communication, making them a valuable technology for applications beyond the traditional realms of gaming and messaging.
Integration with Edge Computing
As edge computing gains prominence, WebSockets may play a crucial role in facilitating real-time communication between devices and edge servers. Edge computing relies on minimizing latency, and the low-latency nature of WebSockets aligns well with the requirements of edge computing applications, such as IoT and smart cities.
Integration with WebAssembly (Wasm)
The growing adoption of WebAssembly allows developers to run code written in languages other than JavaScript in the browser. WebSockets may evolve to seamlessly integrate with WebAssembly, enabling more efficient and diverse real-time applications that leverage the performance benefits of languages like C and Rust.
Standardization of WebSocket APIs
Ongoing efforts to standardize WebSocket APIs across different platforms and frameworks aim to simplify development and promote interoperability. As WebSockets become more ubiquitous, having standardized APIs can contribute to a more consistent and developer-friendly experience.
WebRTC Integration
WebRTC (Web Real-Time Communication) is becoming a standard for browser-based real-time communication.
Future developments may see closer integration between WebSockets and WebRTC, offering a comprehensive solution for various real-time communication scenarios such as video conferencing, voice calls, and collaborative applications.
Increased Use in Progressive Web Apps (PWAs)
The rise of Progressive Web Apps, which combine the best features of web and mobile applications, is likely to drive increased use of WebSockets.WebSockets provide a responsive and interactive experience, making them well-suited for PWAs that aim to deliver app-like experiences in web browsers.
Conclusion
WebSockets play a crucial role in shaping the landscape of real-time communication on the web. This technology offers a persistent, bidirectional communication channel, allowing for low-latency, full-duplex data exchange between clients and servers.
The advantages of reduced latency, efficient resource utilization, and support for real-time, interactive applications position WebSockets as a cornerstone of modern web development.
As a key enabler for real-time applications, WebSockets empowers developers in various domains, facilitating seamless and responsive user experiences in chat applications, live sports updates, collaborative editing, and online gaming.
As real-time communication evolves, exploring the potential of WebSockets becomes crucial. Delving into this technology allows developers to enhance the responsiveness and interactivity of their web applications.
Frequently Asked Questions
What are the limitations of using WebSockets in mobile applications?
WebSockets face limitations in mobile applications such as potential issues with unreliable network conditions, increased battery consumption due to persistent connections, and challenges with background operation. Additionally, some mobile networks or proxies might restrict WebSocket connections.
How do WebSockets handle network interruptions or reconnects?
WebSockets can handle network interruptions by implementing automatic reconnection mechanisms. Developers often use strategies like exponential backoff, which gradually increases the time between reconnection attempts. Heartbeats can also be employed to detect and handle silent disconnections.
Can WebSockets be used in conjunction with other communication protocols like MQTT or AMQP?
Yes, WebSockets can be used in conjunction with other communication protocols like MQTT (Message Queuing Telemetry Transport) or AMQP (Advanced Message Queuing Protocol). This hybrid approach allows leveraging the strengths of each protocol for specific use cases.
What are the differences between WebSocket and WebRTC for real-time communication?
WebSocket provides a bidirectional communication channel for real-time data transfer, while WebRTC is designed specifically for real-time audio and video communication. WebRTC includes features for peer-to-peer media streaming and is commonly used for applications like video conferencing.
How do WebSockets support broadcasting messages to multiple clients?
WebSockets support broadcasting messages to multiple clients by maintaining a list of connected clients on the server. When a message is sent, it is pushed to all connected clients. This enables real-time updates and simultaneous communication with multiple clients.
How do WebSockets handle binary data transmission efficiently?
WebSockets handle binary data efficiently by supporting the transmission of binary frames. This allows the efficient exchange of data such as images, audio, and other binary formats without the need for additional encoding or conversion.
Can WebSockets be integrated with RESTful APIs, and if so, how?
Yes, WebSockets can be integrated with RESTful APIs. While REST is commonly used for request-response interactions, WebSockets complement this by providing real-time, bidirectional communication. Developers often use both technologies in conjunction, with REST for initial data retrieval and WebSockets for real-time updates.
What are the best practices for scaling WebSocket connections in a large-scale application?
Best practices for scaling WebSocket connections include implementing load balancing, using horizontal scaling across multiple servers, optimizing server configurations for handling a high volume of connections, and considering the use of a message broker or distributed architecture.
How does backpressure management work in WebSockets?
Backpressure in WebSockets is managed by implementing flow control mechanisms. If a client or server is unable to handle the incoming data rate, it can signal backpressure to slow down the data transmission. This helps prevent overwhelming the system and ensures smoother operation under heavy loads.
What are the best practices for ensuring data consistency over WebSocket connections?
Ensuring data consistency over WebSocket connections involves implementing proper error handling, validating incoming data on the server, and using acknowledgments to confirm successful message delivery. Additionally, maintaining a consistent application state on both the client and server contributes to overall data consistency.
Can WebSockets be used effectively in serverless architectures?
Yes, WebSockets can be used effectively in serverless architectures. Serverless platforms, such as AWS Lambda, support WebSocket connections. Using services like AWS API Gateway with WebSocket support, developers can implement serverless WebSocket solutions that scale dynamically based on demand.
What are the considerations for using WebSockets in cross-origin scenarios?
Cross-origin WebSocket connections may require server configurations to allow cross-origin requests. Using the appropriate headers, such as CORS (Cross-Origin Resource Sharing) headers, is essential for enabling cross-origin WebSocket communication while considering security implications.
Are there any specific compliance or regulatory considerations when using WebSockets?
Compliance and regulatory considerations depend on the industry and the nature of the data being transmitted. It is crucial to adhere to data protection regulations, encryption standards, and any industry-specific compliance requirements when implementing WebSocket communication.
How can fallback mechanisms be implemented if WebSockets are not supported?
Fallback mechanisms can be implemented by detecting whether the browser supports WebSockets. If not, alternative communication methods like long polling or Server-Sent Events can be used. This ensures that real-time functionality is maintained even in environments where WebSockets are not supported.
How do WebSocket sessions work, and can they be persisted?
WebSocket sessions are maintained through a unique identifier associated with each connection. While WebSocket itself doesn't inherently support persistence, developers can implement mechanisms to persist session information, such as using databases or other storage solutions, to maintain state across server restarts or reconnect.
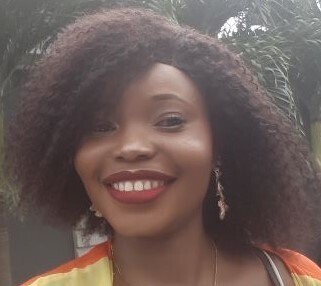
Yetunde Salami is a seasoned technical writer with expertise in the hosting industry. With 8 years of experience in the field, she has a deep understanding of complex technical concepts and the ability to communicate them clearly and concisely to a wide range of audiences. At Verpex Hosting, she is responsible for writing blog posts, knowledgebase articles, and other resources that help customers understand and use the company's products and services. When she is not writing, Yetunde is an avid reader of romance novels and enjoys fine dining.
View all posts by Yetunde Salami