After playing with polygon shapes in the previous article, we are going to create a heart shape using CSS. You are probably saying: “Why another article about the heart shape? it was already done a thousand times!”. We are not going to only build a heart shape but we will do it with modern CSS tricks. Forget the pseudo-element with border-radius and rotation. We are also going to transform images into hearts!
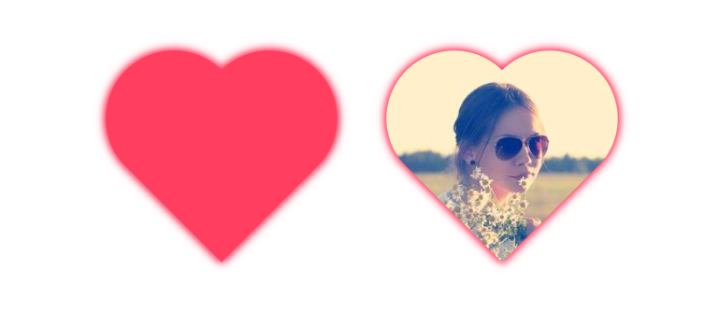
Using CSS Gradients
Let’s jump straight into the first method where we will rely on multiple gradients to create a heart shape.
.heart {
--c: red;
width: 200px;
aspect-ratio: 1;
background:
/* 1 */ radial-gradient(circle at 60% 65%,var(--c) 64%,#0000 65%) top left/50% 50%,
/* 2 */ radial-gradient(circle at 40% 65%,var(--c) 64%,#0000 65%) top right/50% 50%,
/* 3 */ conic-gradient(from -45deg at 50% 85.5%,var(--c) 90deg,#0000 0) bottom/100% 50%;
background-repeat: no-repeat;
}
We define a square element using aspect-ratio: 1
. Then, we use three gradient layers to create the final shape. Here is an illustration to understand each gradient
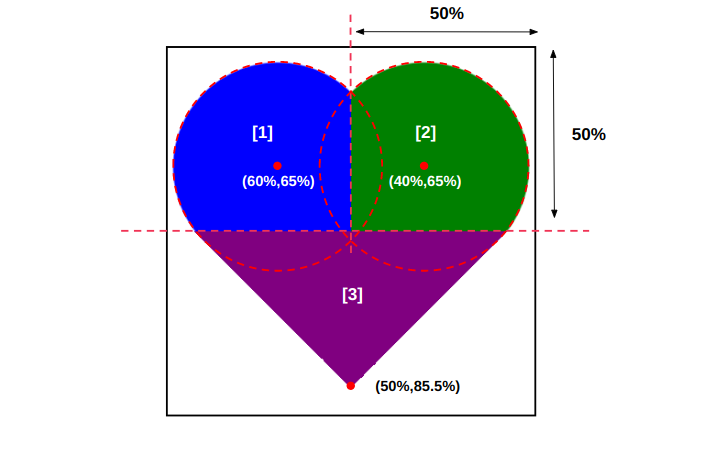
Each radial-gradient()
will take a quarter of the element at the top to create the circular part of the shape. The conic-gradient()
will create the triangular part at the bottom.
I won’t detail the math logic behind the different values and you don’t need to update them. All you have to do is to adjust the width value to control the size of the heart and the CSS variables to change the color.
It is worth noting that we can find different syntax to achieve the same result using gradients.
Here is another one with less code:
.heart {
--c: red;
width: 200px;
aspect-ratio: 1;
background:
radial-gradient(at 70% 31%,var(--c) 29%,#0000 30%),
radial-gradient(at 30% 31%,var(--c) 29%,#0000 30%),
conic-gradient(from -45deg at 50% 84%,var(--c) 90deg,#0000 0)
bottom/100% 50% no-repeat;
}
It’s always the same configuration: two radial-gradient()
and one conic-gradient()
but written differently.
Adding Clip-path
Sometimes, we may face some anti-aliasing issues with the conic-gradient()
and we get jagged edges. To avoid this we can introduce clip-path
:
.heart {
--c: red;
width: 200px;
aspect-ratio: 1;
background:
radial-gradient(at 70% 31%,var(--c) 29%,#0000 30%),
radial-gradient(at 30% 31%,var(--c) 29%,#0000 30%),
linear-gradient(var(--c) 0 0) bottom/100% 50% no-repeat;
clip-path: polygon(-43% 0,50% 91%, 143% 0);
}
I replaced the conic-gradient()
with a linear-gradient()
to cover the bottom area with the main color. Then the clip-path
will clip the element and create the triangular shape:
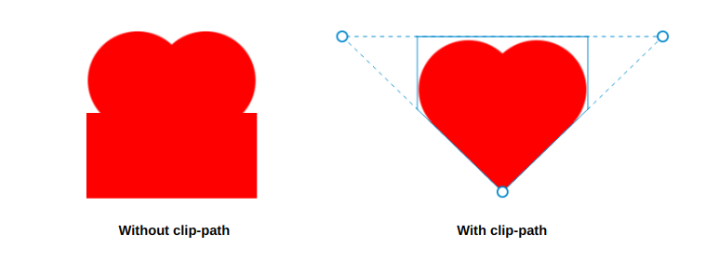
Using border-image
We can optimize our code and replace the three gradients with only one. Yes, it’s possible! For this, we will rely on border-image
using radial-gradient()
.
.heart {
--c: red;
width: 200px;
aspect-ratio: 1;
border-image: radial-gradient(var(--c) 69%,#0000 70%) 84.5%/50%;
clip-path: polygon(-42% 0,50% 91%, 142% 0);
}
I know that the border-image
syntax is a bit crazy but let’s dissect it. It’s the shorthand for:
border-image-source: radial-gradient(var(--c) 69%,#0000 70%);
border-image-slice: 84.5%;
border-image-width: 50%;
We need a radial-gradient()
to create a circular shape so the border-image-source
is trivial.
We need the shape to cover the whole element so the width needs to be as big as possible. 50%
is the minimum value but we can go bigger and the result will always be the same. Less than 50%
will give us a gap. If for example, you use 45%
you will have a gap equal to 100% - 2*45% = 5%
in the middle.
The trickiest value is the border-image-slice
. When you use 100%
in the slice, you will have a circle on each corner. Using 50%
will give you a full circle covering the whole element. If you manually play with the value between 50%
and 100%
you can get the shape we need. The value is 84.5%
. I have a detailed article about border-image
that I invite you to read for more detail.
Finally, we apply the clip-path and we get the heart shape:
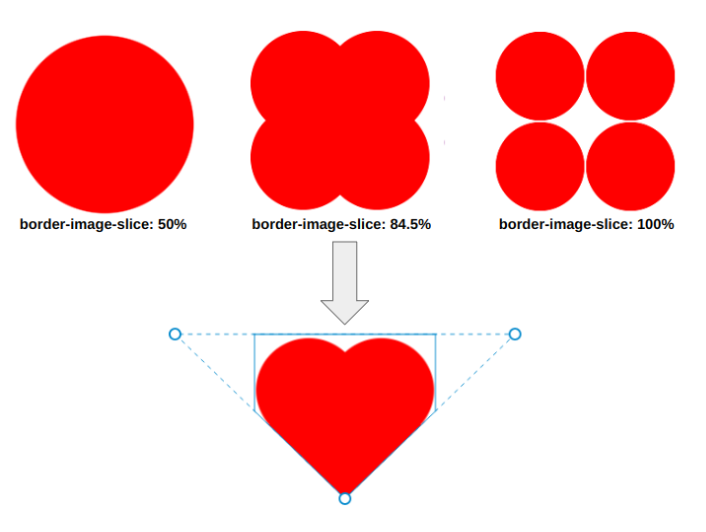
Turning an image into a heart
Having a heart shape is good but putting an image inside it is better! For this, we are going to reuse the previous code with masking. The method that uses background
will rely on mask
and the one with border will rely on mask-border
.
Why not use only the last version which is the shortest?
That’s what I recommend for the future but for now, mask-border
has a very low browser support so will rely on the mask property as a fallback.
img {
width: 250px;
aspect-ratio: 1;
--_m: radial-gradient(#000 69%,#0000 70%) 84.5%/50%;
-webkit-mask-box-image: var(--_m);
mask-border: var(--_m);
clip-path: polygon(-42% 0,50% 91%, 142% 0);
}
/* fallback until better support for mask-border */
@supports not (-webkit-mask-box-image: var(--_m)) {
img {
--_m:
radial-gradient(at 70% 31%,var(--c) 29%,#0000 30%),
radial-gradient(at 30% 31%,var(--c) 29%,#0000 30%),
linear-gradient(#000 0 0) bottom/100% 50% no-repeat;
-webkit-mask: var(--_m);
mask: var(--_m);
}
}
TADA! We turned our image into a heart shape with a small code. The code looks a bit lengthy due to the prefixes and browser support but in the future it will only be this one:
img {
width: 250px;
aspect-ratio: 1;
mask-border: radial-gradient(#000 69%,#0000 70%) 84.5%/50%
clip-path: polygon(-42% 0,50% 91%, 142% 0);
}
And why not a cool hover effect to reveal your image:
In the above, I am using the outline technique I am detailing in a previous article (in this article as well)
Conclusion
Like I said in the introduction: no pseudo-element, no border-radius, and no rotation. We used modern CSS tricks to create a heart shape using one element and a few lines of code. I will end this article with a CSS pattern. We have used gradients to create the heart so we can easily build a pattern with it!
Frequently Asked Questions
Can free HTML editors be used for professional web development?
Yes, free HTML editors like Visual Studio Code and Atom offer advanced features and are suitable for professional web development.
Does Verpex provide any tutorials or resources for web developers using their hosting service?
Verpex offers a comprehensive knowledge base and blog featuring tutorials, resources, and best practices to help web developers make the most of their hosting services.
Is there a money-back guarantee for Verpex Web Development Hosting services?
Yes, Verpex Web Development Hosting offers a 45-day money-back guarantee, allowing you to try our services risk-free and assess if they meet your needs.
Are there any limitations on the type of web development projects I can host with Verpex?
Verpex Web Development Hosting supports a wide range of web development projects. However, we do not allow projects that promote illegal activities, violate intellectual property rights, or engage in malicious activities.
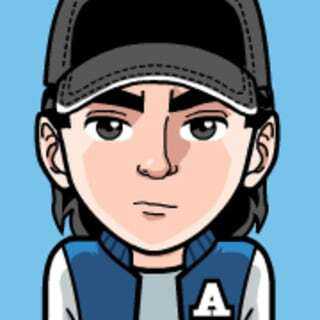
Temani Afif is an expert web developer, a content creator, and a CSS addict. He is the mastermind behind CSS Loaders, CSS Generators, CSS Tip and a lot of CSS stuff.
View all posts by Temani Afif