Imagine starting every new web project by writing the same basic CSS from scratch: resetting browser styles, setting up a grid system, and configuring typography. It’s repetitive and time-consuming. This is where a CSS boilerplate comes in.
In this article, we’ll explore what a CSS boilerplate is, why it’s valuable, and how it can supercharge your workflow.
Let’s dive in!
What Is a CSS Boilerplate?
A CSS boilerplate is a pre-written collection of CSS styles designed to serve as a foundation for new projects. It typically includes rules for resetting browser defaults, establishing consistent typography, and setting up a basic layout structure.
Think of it as a template that ensures your CSS starts with a clean slate, free of browser quirks, and ready for customization.
How It Works
When you include a boilerplate in your project, it provides a predefined set of styles you can either use as-is or modify to fit your design needs. These styles focus on universal requirements, like ensuring all browsers render elements consistently.
For example, here’s a simple snippet from a boilerplate like Normalize.css:
/* Resetting the default box-sizing for better consistency */
html {
box-sizing: border-box;
}
*, *::before, *::after {
box-sizing: inherit; /* Ensures all elements use the same box-sizing model */
}
/* Improve readability by adjusting default font settings */
body {
font-family: Arial, sans-serif;
line-height: 1.5;
color: #333;
margin: 0;
padding: 0;
}
Without these boilerplate rules, browsers would apply their styles, leading to unpredictable results across different devices. By normalizing these styles, a boilerplate makes your project behave consistently.
Boilerplate vs. Framework
It’s easy to confuse a boilerplate with a CSS framework like Bootstrap or Tailwind CSS, but they serve different purposes:
CSS boilerplate: A lightweight starting point focusing on resets and basic styles. It’s meant to be extended and customized.
CSS framework: A comprehensive toolkit with pre-designed components, utility classes, and grid systems. Frameworks like Bootstrap are often heavier and opinionated.
For instance, a boilerplate might provide this basic grid setup:
.container {
width: 100%;
max-width: 1200px;
margin: 0 auto;
padding: 0 20px;
}
.row {
display: flex;
flex-wrap: wrap;
}
.col {
flex: 1;
padding: 10px;
}
This serves as a foundation for your layout, unlike a framework that might already have styled buttons, modals, and cards.
Why Use a CSS Boilerplate?
A CSS boilerplate might seem like just another tool in the developer’s arsenal, but it can significantly improve your workflow in several ways.
Here are key reasons why using a CSS boilerplate is a smart choice.
1. Speeds Up Development
Starting from scratch means setting up base styles every time, which is repetitive and time-consuming. A CSS boilerplate eliminates this step by providing a ready-to-use foundation.
Example: Imagine you need to set up a project with basic typography and a consistent margin. Without a boilerplate, you might write something like this:
body {
font-family: Arial, sans-serif;
line-height: 1.6;
margin: 0;
}
h1, h2, h3, h4, h5, h6 {
margin: 0 0 10px;
font-weight: bold;
}
A boilerplate already includes these types of rules, saving you precious time during setup.
2. Ensures Consistency
Different browsers apply unique default styles, which can lead to inconsistent rendering. A boilerplate normalizes these differences, giving your project a uniform starting point.
Example: Without normalization, a <button>
might look different in Chrome versus Firefox. A boilerplate like Normalize.css ensures consistency:
button {
background: none;
border: none;
padding: 0;
font: inherit;
cursor: pointer;
}
This ensures buttons across all browsers have the same baseline styling, so you don’t have to troubleshoot inconsistencies.
3. Simplifies Scaling
As your project grows, managing styles can become challenging. A CSS boilerplate provides a modular and organized structure that makes it easier to scale. For example, a boilerplate might define reusable utility classes for common tasks:
.mt-10 {
margin-top: 10px;
}
.text-center {
text-align: center;
}
Instead of writing repetitive CSS rules, you can reuse these utility classes, keeping your codebase clean and manageable.
4. Reduces Bugs
Boilerplates are crafted by experienced developers who have addressed common pitfalls, such as browser quirks or accessibility issues. Using one helps you avoid subtle bugs.
Example: Accessibility-focused boilerplates might include ARIA roles or keyboard navigation rules by default:
a:focus {
outline: 2px solid #005fcc;
}
This ensures interactive elements are accessible to keyboard users without requiring you to write custom code.
5. Promotes Best Practices
Most CSS boilerplates are designed with modern standards in mind, incorporating best practices like responsive design and modular CSS.
Example: A boilerplate might include a responsive grid system to get you started:
.row {
display: flex;
flex-wrap: wrap;
}
.col-6 {
flex: 0 0 50%;
}
With this setup, you can build layouts that adapt to different screen sizes without extra effort.
Common Features of CSS Boilerplates
CSS boilerplates are designed to simplify the foundational setup for web projects. They come with a variety of built-in features that handle repetitive tasks, making it easier to start your project with a clean and functional base.
Let’s explore the most common features you’ll find in a CSS boilerplate.
Browser Style Resets
Browsers apply their own default styles to elements, which can lead to inconsistencies in design. Boilerplates include resets or normalization to override these defaults and create a consistent starting point.
Example: Here’s a typical reset rule:
/* Remove default padding and margin for all elements */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
This ensures every element starts with the same baseline styles, regardless of the browser.
Cross-Browser Compatibility
Boilerplates address quirks in how browsers render specific elements, helping you avoid spending time troubleshooting compatibility issues.
Example: A boilerplate might include fixes for consistent form input styles across browsers:
input, textarea, select, button {
font-family: inherit;
font-size: 100%;
margin: 0;
}
This ensures inputs and buttons look consistent across Chrome, Safari, and Firefox.
Typography Defaults
Typography is a key part of web design, and boilerplates often include default font settings to improve readability.
Example: Here’s a basic typography setup you might find:
body {
font-family: 'Helvetica Neue', Arial, sans-serif;
line-height: 1.5;
color: #333;
}
h1, h2, h3, h4, h5, h6 {
margin: 0 0 10px;
font-weight: bold;
}
These rules provide a visually appealing and legible starting point for text.
Grid Systems
Many boilerplates include a simple grid system to help you create responsive layouts quickly.
Example: Here’s a basic grid system setup:
.container {
width: 100%;
max-width: 1200px;
margin: 0 auto;
padding: 0 20px;
}
.row {
display: flex;
flex-wrap: wrap;
}
.col {
flex: 1;
padding: 10px;
}
This structure allows you to create flexible, responsive designs without building a grid from scratch.
Utility Classes
Boilerplates often include utility classes for commonly used styles, making it easy to apply them without writing custom rules.
Example:
.text-center {
text-align: center;
}
.mt-20 {
margin-top: 20px;
}
.hidden {
display: none;
}
These classes save time and help keep your styles consistent throughout the project.
Responsive Design Helpers
Modern boilerplates include styles and utilities that help you build responsive designs that adapt to different screen sizes.
Example:
@media (max-width: 768px) {
.col {
flex: 0 0 100%;
}
}
With this, your grid system automatically adjusts for smaller screens.
Modular and Maintainable File Structure
A good boilerplate provides an organized CSS file structure to keep your styles modular and easy to maintain.
Example structure:
styles/
├── base.css /* Resets and basic styles */
├── layout.css /* Grid system and layout rules */
├── components.css /* Buttons, forms, etc. */
This separation ensures you can quickly locate and update specific styles.
Popular CSS Boilerplates
CSS boilerplates are not one-size-fits-all, and the right choice depends on your project needs.
Below are some of the most popular CSS boilerplates, along with a brief overview of their purpose, features, and ideal use cases.
Normalize.css
Normalize.css is one of the most widely used CSS boilerplates, known for its minimalism and effectiveness. Instead of resetting all browser styles to a blank slate, it normalizes them, preserving useful defaults while addressing inconsistencies.
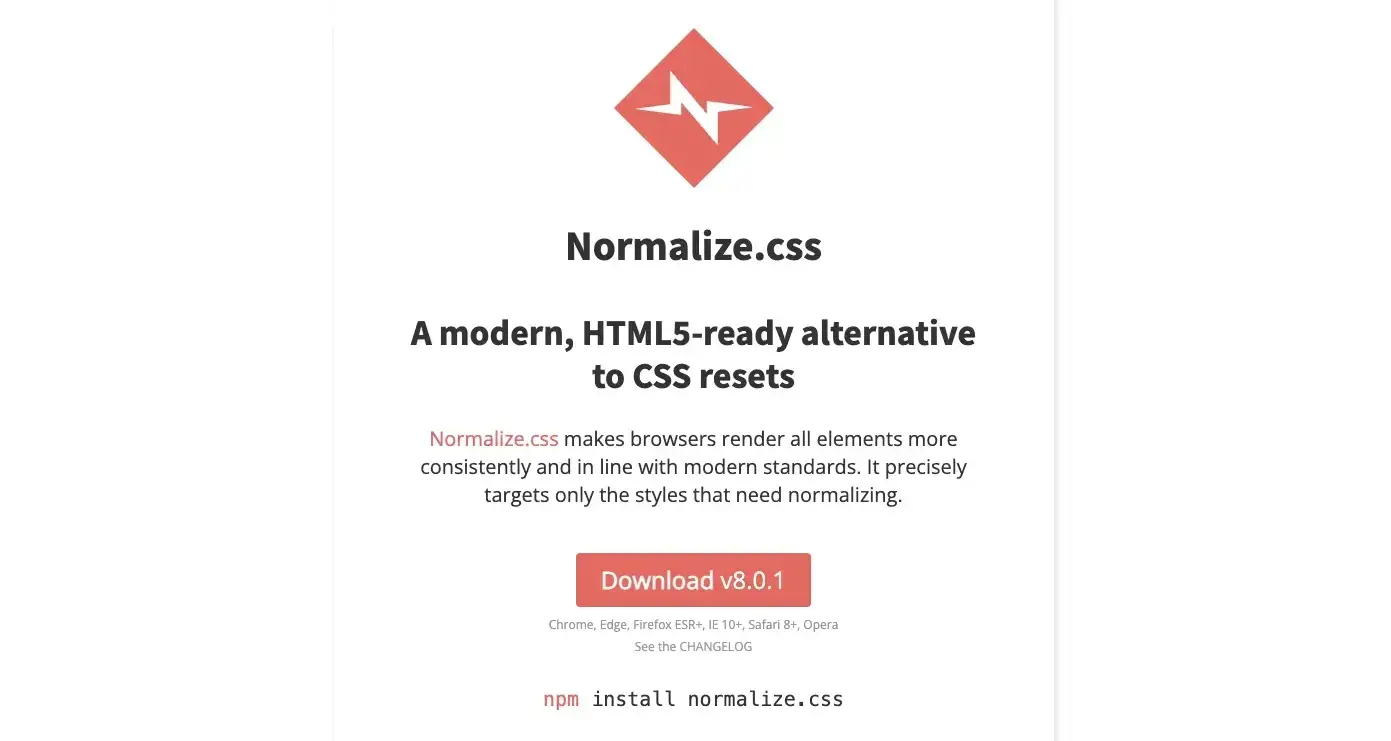
For example, it ensures that <h1>
tags render consistently across browsers while leaving sensible styles like clickable link underlines intact.
Developers prefer Normalize.css because it’s lightweight and easy to integrate into any project. It’s perfect for developers who want to focus on building their custom styles without dealing with browser quirks.
A typical use case for Normalize.css is ensuring form elements, such as buttons and inputs, render consistently across browsers like Chrome, Safari, and Firefox. This makes it a reliable choice for projects where simplicity and cross-browser compatibility are priorities.
Skeleton
Skeleton is a tiny CSS boilerplate designed for simplicity and speed. It provides just enough tools to get started with responsive layouts and typography, making it ideal for small projects or quick prototypes.
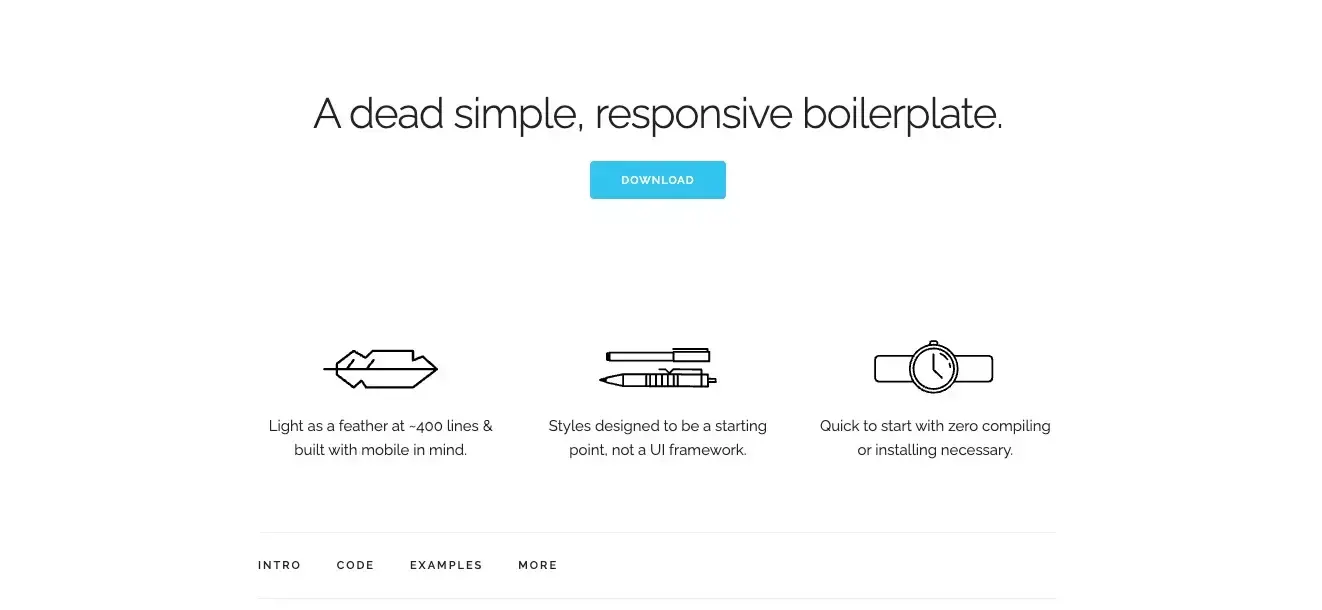
At its core, Skeleton includes a lightweight 12-column grid system, predefined typography styles, and basic elements like buttons and forms. It’s not a full-fledged framework, so it doesn’t come with pre-designed components like modals or navigation bars, which gives developers the freedom to customize their designs from the ground up.
Skeleton is particularly suited for developers who want a quick-start CSS setup without unnecessary bulk. For instance, you can create responsive layouts with minimal effort using its grid system while keeping the overall page load time low.
HTML5 Boilerplate
HTML5 Boilerplate goes beyond CSS and provides a complete starting template for web projects. It includes Normalize.css for styling resets, JavaScript, caching rules, and performance optimizations.
It’s an excellent choice for developers who want a robust starter kit for modern web applications, as it includes built-in mobile-friendly responsive settings.
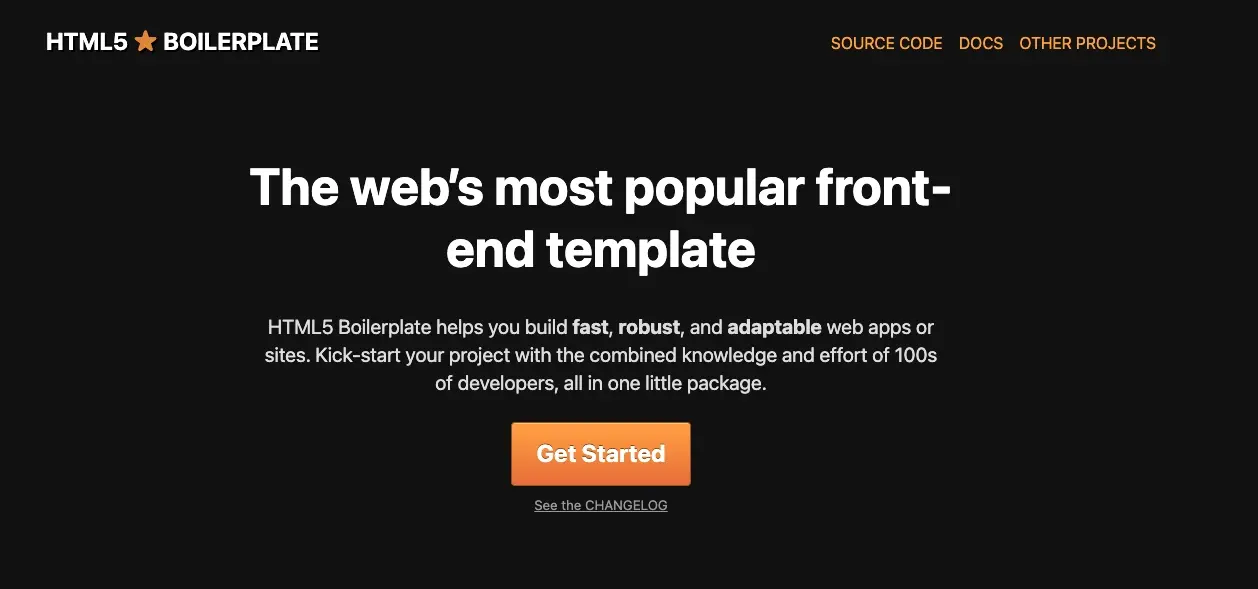
For example, HTML5 Boilerplate includes base styles for typography and layout, but it also integrates other web development essentials, such as a default favicon setup, CSS and JavaScript minification hints, and optimized cross-domain loading techniques.
This makes it more than just a CSS boilerplate—it’s a comprehensive toolkit for building fast, responsive websites.
How to Get Started with a CSS Boilerplate
Getting started with a CSS boilerplate is straightforward. Whether you’re working on a small static website or a dynamic application, integrating a boilerplate can set you up with a solid foundation.
Here’s a step-by-step guide to help you get started:
1. Choose the Right Boilerplate
The first step is selecting a boilerplate that matches your project’s needs. To make an informed choice, consider factors like the size of your project, your preferred level of customization, and whether you need additional tools like grid systems or performance tweaks.
2. Add the Boilerplate to Your Project
Once you’ve chosen a boilerplate, there are several ways to include it in your project:
Option 1 — Use a CDN (Content Delivery Network): This is the quickest method. Add a link to the boilerplate’s stylesheet in your HTML file.
Example for Normalize.css:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/8.0.1/normalize.min.css">
Option 2 — Download the File: Visit the official website or GitHub repository of the boilerplate, download the CSS file, and add it to your project directory.
project/
├── css/
│ └── normalize.css
├── index.html
Then link it in your HTML:
<link rel="stylesheet" href="css/normalize.css">
Option 3 — Install via package managers: If you’re using npm or yarn, you can install the boilerplate as a dependency.
Example for Normalize.css:
npm install normalize.css
Then import it in your CSS or JavaScript file:
@import "normalize.css";
3. Customize the Boilerplate
Most boilerplates are designed to be extended or customized to fit your project’s specific needs. After adding the boilerplate, you can start writing your own CSS to override or add styles.
Example:
/* Normalize.css included in the project */
/* Add custom styles */
body {
font-family: 'Roboto', sans-serif;
background-color: #f4f4f4;
}
.container {
max-width: 1200px;
margin: 0 auto;
padding: 20px;
}
4. Test and Iterate
Once you’ve integrated the boilerplate and added your custom styles:
Test your project on different browsers (Chrome, Firefox, Safari, Edge) to ensure consistency.
Validate your CSS using tools like the W3C CSS Validator to catch errors or unsupported properties.
Continuously refine the styles to suit your design as the project evolves.
5. Maintain and Scale
As your project grows, keep your styles modular and organized. A good practice is to separate the boilerplate from your custom styles. For example:
project/
├── css/
│ ├── normalize.css /* Boilerplate */
│ ├── base.css /* Your resets and base styles */
│ ├── layout.css /* Grid and layout styles */
│ └── components.css /* Buttons, forms, etc. */
This structure ensures the boilerplate remains untouched, while your customizations stay modular and easy to maintain.
By following these steps, you can seamlessly integrate a CSS boilerplate into your workflow, save time, and build consistent and scalable styles for your project.
Conclusion
A CSS boilerplate is more than just a starting point—it’s a powerful tool that saves time, reduces repetitive work, and ensures consistency across your projects.
Whether you’re a beginner trying to simplify the setup process or an experienced developer aiming to streamline your workflow, a boilerplate can be a valuable addition to your toolkit.
As you integrate a boilerplate into your workflow, remember to:
Choose the one that best fits your project’s needs.
Customize it to align with your design goals.
Regularly test and refine your styles for better results.
Have fun coding!
Frequently Asked Questions
What security measures are essential for CSS hosting?
Key security measures for CSS hosting include SSL/TLS encryption, regular software updates, firewalls, and protection against DDoS attacks. These features safeguard your CSS files and the overall integrity of your website.
Can free HTML editors support other languages like CSS and JavaScript?
Yes, free HTML editors often support languages like CSS and JavaScript, enabling integrated web development.
What is the impact of server location on CSS hosting performance?
Server location significantly affects the loading speed of CSS files. Hosting your CSS closer to your user base ensures faster download times, reducing website latency and improving user experience.
How does CSS hosting handle scalability for high-traffic websites?
CSS hosting typically employs scalable infrastructure, like cloud hosting, which can dynamically allocate resources to handle increased traffic. This ensures your site remains fast and reliable, even during traffic surges.
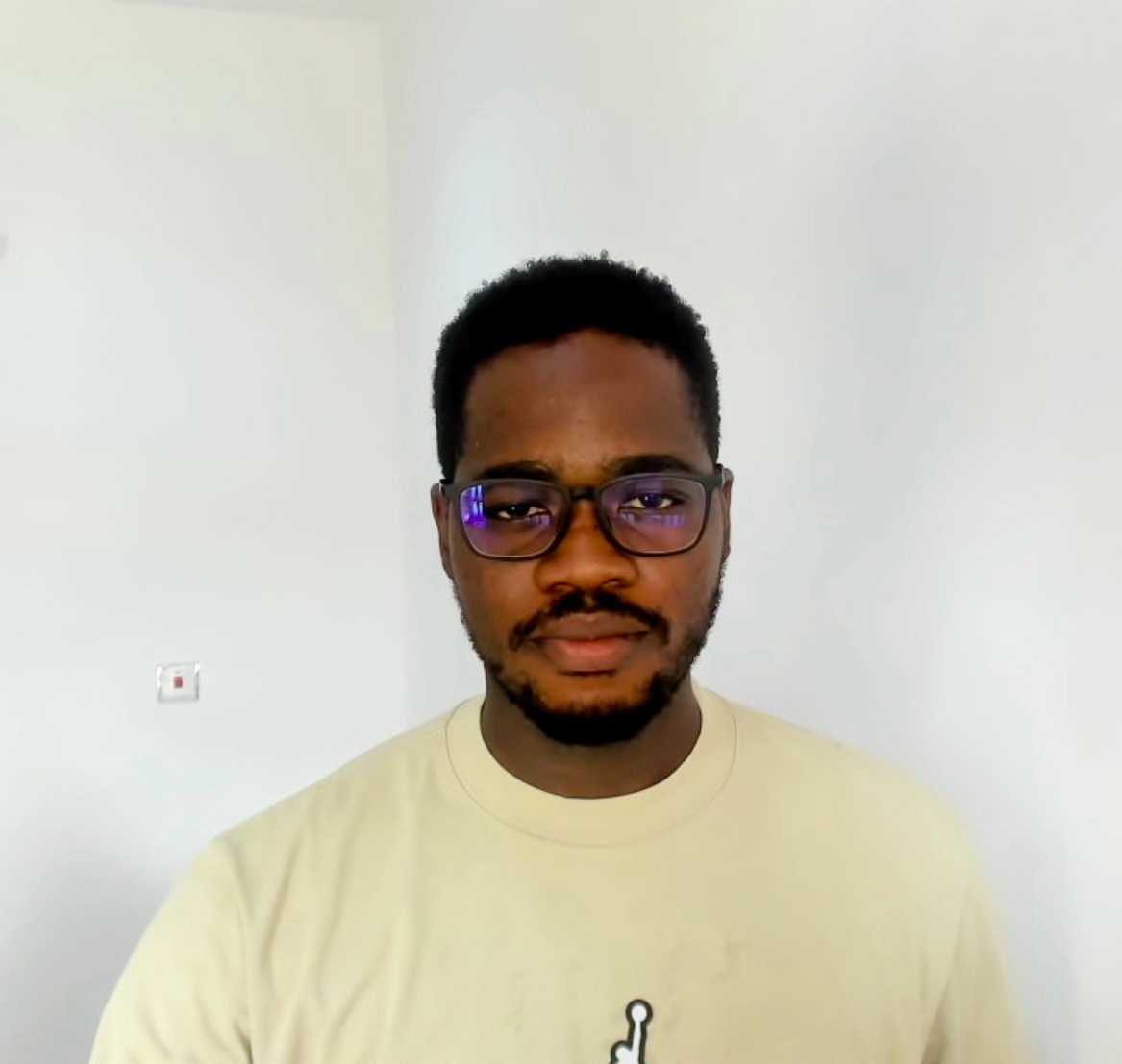
Joel Olawanle is a Software Engineer and Technical Writer with over three years of experience helping companies communicate their products effectively through technical articles.
View all posts by Joel Olawanle