Animations are everywhere on the web. They make things feel alive. Think of a button that changes color when you hover over it, or a loading spinner that keeps you company while something loads. These little movements aren’t just pretty. They guide users, make things clearer, and sometimes even add a bit of fun.
There are two main ways to create these animations: CSS and JavaScript. Both get the job done, but they work very differently, and choosing the right one can save you time and frustration and even make your website run faster.
In this article, we break it all down: when it’s better to stick with CSS, when JavaScript shines, and how to decide which one to use for your project. Don’t worry, we’ll keep things simple and straight to the point. Ready? Let’s get started.
The Basics of CSS Animations
CSS animations let you create smooth, eye-catching effects without much effort. They’re declarative, meaning you simply tell the browser what should happen, and it handles the how. This makes CSS animations lightweight and straightforward to use.
You’ll mostly work with two tools:
transition
– Great for simple changes like fading or sliding.animation
– Paired with@keyframes
for more complex effects that involve multiple steps.
Here’s an example to show how simple it is:
button {
background-color: blue;
color: white;
transition: background-color 0.3s ease;
}
button:hover {
background-color: green;
}
In the code above, the transition
tells the browser to make the background color change smoothly over 0.3 seconds when the user hovers over the button. That’s all it takes!
Secondly, for animations that involve multiple stages, @keyframes
comes into play. Think of it as creating a timeline for your animation.
Here’s an example:
@keyframes slideIn {
from {
transform: translateX(-100%);
opacity: 0;
}
to {
transform: translateX(0);
opacity: 1;
}
}
.element {
animation: slideIn 1s ease-out;
}
In the code above, the from
state sets the element off-screen and invisible, the to state moves it into place and fades it in and the animation
property ties it all together, running the effect over 1 second.
The Basics of JavaScript Animations
JavaScript animations give you full control over how elements move and behave, making them perfect for dynamic and interactive effects. Unlike CSS, where you define animations in stylesheets, JavaScript lets you animate elements directly through code, making it more flexible.
There are two main ways to create animations with JavaScript:
Manual animations – You update an element’s properties over time using JavaScript.
Animation libraries – Tools like GSAP, Anime.js, and Lottie make animations smoother, easier to manage, and more powerful.
Here’s a simple example of moving an element:
const box = document.querySelector(".box");
let position = 0;
function moveBox() {
position += 5;
box.style.transform = `translateX(${position}px)`;
if (position < 200) {
setTimeout(moveBox, 50);
}
}
moveBox();
This moves the element 5 pixels to the right every 50 milliseconds.
However, writing animations manually can get complicated, especially when dealing with easing, sequences, or complex motion effects. That’s where animation libraries come in.
GSAP (GreenSock Animation Platform) is one of the most powerful JavaScript animation libraries, widely used for professional-grade animations:
With just one line of code, you get a smooth, optimized animation. Other libraries like **Anime.js** are also great for handling multiple animations at once, while **Lottie** is perfect for rendering vector animations from After Effects.
Key Differences Between CSS and JavaScript Animations
Both CSS and JavaScript animations have their strengths, and the right choice depends on what you're trying to achieve. CSS animations are great for simple, performance-friendly effects, while JavaScript shines when you need interactivity, complex motion, or fine-grained control.
Here’s a side-by-side comparison of the key differences:
Feature | CSS Animations | JavaScript Animations |
---|---|---|
Ease of Use | Simple to implement with transitions and keyframes. | Requires scripting but allows more flexibility. |
Performance | Optimized by the browser, runs on the compositor thread. | Can be optimized, but may cause lag if not handled well. |
Control | Limited to predefined keyframes and timing. | Full control over every frame and animation state. |
Interactivity | Hard to integrate with user input. | Can respond to clicks, scrolls, and other user actions. |
Complexity | Best for basic to moderate animations. | Ideal for advanced animations and physics-based motion. |
If you need quick, lightweight effects like hover animations, CSS is the best choice. But if you want animations that react to user actions or involve complex sequences, JavaScript is the way to go.
When to Use CSS Animations
CSS animations are best suited for scenarios where simplicity, performance, and efficiency matter. Since they run directly in the browser’s rendering engine, they minimize the impact on the main thread, making them ideal for lightweight transitions and effects that don’t require scripting.
Here are the key situations where CSS animations should be your first choice:
1. Simple, State-Based Animations
CSS is perfect when an animation only needs to reflect a change in state, such as an element appearing, disappearing, or changing styles when hovered, focused, or active.
For example: Imagine a notification banner that smoothly fades into view when it appears on the page.
.notification {
opacity: 0;
transform: translateY(-20px);
animation: fadeIn 0.5s ease-out forwards;
}
@keyframes fadeIn {
from {
opacity: 0;
transform: translateY(-20px);
}
to {
opacity: 1;
transform: translateY(0);
}
}
Here, the animation starts with the notification slightly above its final position and fully transparent. Over 0.5 seconds, it moves into place and fades in. The forwards
keyword ensures it stays visible once the animation completes.
This works well because there’s no need for JavaScript to manage the timing or trigger the effect—it runs automatically when the element appears in the DOM.
2. Performance-Critical Animations
CSS animations are optimized by the browser, particularly when using transform
and opacity
. These properties can be handled by the GPU instead of causing unnecessary reflows and repaints, making them ideal for performance-sensitive effects.
For example: A simple loading animation can be handled entirely in CSS without affecting the page’s responsiveness.
.loader {
width: 40px;
height: 40px;
border: 5px solid #ccc;
border-top-color: #007bff;
border-radius: 50%;
animation: spin 1s linear infinite;
}
@keyframes spin {
to {
transform: rotate(360deg);
}
}
This continuously rotates the element, creating a smooth spinning effect. Since it only modifies transform
, it runs efficiently on the GPU without interfering with the main thread.
If this same animation were done using JavaScript, it would require manual timing updates, which could introduce performance issues if not optimized correctly.
3. Automatically Running Effects
Some animations don’t need to be triggered by user interaction—they should just run as soon as the page loads or loop indefinitely. CSS is the best option for these cases since it requires no extra logic.
For example: A website header can have a subtle, animated background effect that continuously shifts colors to create visual interest.
@keyframes gradientShift {
0% {
background-position: 0% 50%;
}
50% {
background-position: 100% 50%;
}
100% {
background-position: 0% 50%;
}
}
.animated-bg {
background: linear-gradient(45deg, #ff7eb3, #ff758c, #ff7eb3);
background-size: 200% 200%;
animation: gradientShift 5s infinite ease-in-out;
}
Here, the gradient background shifts position over five seconds in an infinite loop, creating a seamless animated effect without the need for JavaScript.
This is useful for non-interactive animations that improve aesthetics without needing to respond to user actions.
When Should You Use CSS Over JavaScript?
CSS animations are the right choice when:
The animation is tied to a state change (like an element appearing, disappearing, or transitioning between styles).
Performance is a priority, especially when animating
transform
oropacity
.The animation runs automatically without user interaction.
A lightweight, maintainable solution is preferred over scripting.
JavaScript is often the better tool for more complex animations that require interaction, sequencing, or physics-based motion. But for simple, efficient effects, CSS remains the fastest and easiest approach.
When to Use JavaScript Animations
JavaScript animations are the best choice when you need more control, interactivity, or complexity than what CSS alone can provide. Unlike CSS, which is limited to predefined keyframes and simple transitions, JavaScript lets you dynamically adjust animations based on user input, physics-based effects, and real-time data.
Here are the key situations where JavaScript is the better option, along with examples:
1. Complex Animations That Require Precise Control
When an animation involves multiple stages, chaining effects, or needs to be fine-tuned dynamically, JavaScript is the better tool.
For example: A physics-based bounce effect cannot be easily done with CSS alone, as it requires custom easing and dynamically adjusting the animation speed.
const ball = document.querySelector(".ball");
let position = 0;
let velocity = 10;
let gravity = 0.5;
function bounce() {
position += velocity;
velocity -= gravity;
if (position <= 0) {
velocity = -velocity * 0.7; // Lose some energy on bounce
}
ball.style.transform = `translateY(${Math.max(position, 0)}px)`;
requestAnimationFrame(bounce);
}
bounce();
Here, JavaScript calculates the ball’s position in real-time, simulating gravity and bounce effects. This kind of physics-based movement isn't possible with CSS alone.
2. Animations That Depend on User Interaction
If an animation needs to respond to events like clicks, drags, or scrolling, JavaScript is the best approach because it can handle dynamic updates in response to user actions.
For example: A common effect is making elements fade in as the user scrolls down the page.
const element = document.querySelector(".fade-in");
window.addEventListener("scroll", () => {
const rect = element.getBoundingClientRect();
if (rect.top < window.innerHeight * 0.8) {
element.classList.add("visible");
}
});
.fade-in {
opacity: 0;
transform: translateY(20px);
transition: opacity 0.5s ease, transform 0.5s ease;
}
.fade-in.visible {
opacity: 1;
transform: translateY(0);
}
Here, JavaScript listens for the scroll event and applies a CSS class when the element enters the viewport. While CSS handles the actual animation, JavaScript is necessary to trigger it based on user behavior.
3. Sequential or Chained Animations
If an animation needs to happen in a specific sequence—such as elements appearing one after another—JavaScript allows better control over timing.
For example: Using GSAP, a JavaScript animation library, you can animate elements in a sequence effortlessly.
This line of code smoothly animates multiple `.word` elements, fading them in one after another with a short delay. Achieving this level of sequencing with pure CSS would be significantly more complex.
###4. Cross-Browser and Complex SVG Animations
JavaScript animations are often more reliable across different browsers, especially when working with SVGs or canvas-based graphics.
**For example:** Animating an [SVG](https://verpex.com/blog/wordpress-hosting/using-svg-in-wordpress-a-beginners-guide) drawing effect requires dynamically updating stroke properties, something that CSS cannot do as effectively.
```
const path = document.querySelector(".line");
path.style.strokeDasharray = path.getTotalLength();
path.style.strokeDashoffset = path.getTotalLength();
gsap.to(path, { strokeDashoffset: 0, duration: 2 });
```
This creates a drawing effect, where an SVG line gradually appears over time.
### When Should You Use JavaScript Over CSS?
JavaScript animations are the right choice when:
- The animation requires precise control over timing, easing, or physics.
- The animation is driven by user interaction, such as clicks, scrolling, or dragging.
- Multiple animations need to run in sequence or be dynamically triggered.
- You're working with SVGs, canvas elements, or complex effects that CSS alone can't handle.
CSS is excellent for simple and performance-efficient effects, but JavaScript unlocks the full potential of web animations, making it essential for interactive, engaging experiences.
Wrapping Up!
Both CSS and JavaScript animations have their place in web development. CSS is the best choice for simple, performance-friendly effects like transitions, hover animations, and keyframe-based movements. It’s easy to implement and optimized by the browser.
JavaScript, on the other hand, is essential for complex, interactive, or physics-driven animations. It gives you precise control, allowing animations to respond to user actions, run in sequence, or integrate with external data.
The key takeaway? Use CSS when possible for efficiency, and turn to JavaScript when you need flexibility and advanced control.
Frequently Asked Questions
How does Verpex Hosting handle databases for JavaScript hosting?
We support both SQL (like MySQL, PostgreSQL) and NoSQL (such as MongoDB) databases for JavaScript hosting, ensuring seamless integration and management for Node.js applications.
Can free HTML editors support other languages like CSS and JavaScript?
Yes, free HTML editors often support languages like CSS and JavaScript, enabling integrated web development.
How does CSS hosting handle scalability for high-traffic websites?
CSS hosting typically employs scalable infrastructure, like cloud hosting, which can dynamically allocate resources to handle increased traffic. This ensures your site remains fast and reliable, even during traffic surges.
Are there specific hosting requirements for JavaScript frameworks like React, Angular, or Vue.js?
Answer: Generally, hosting for client-side JavaScript frameworks like React, Angular, or Vue.js does not require specialized servers since the frameworks run in the user's browser.
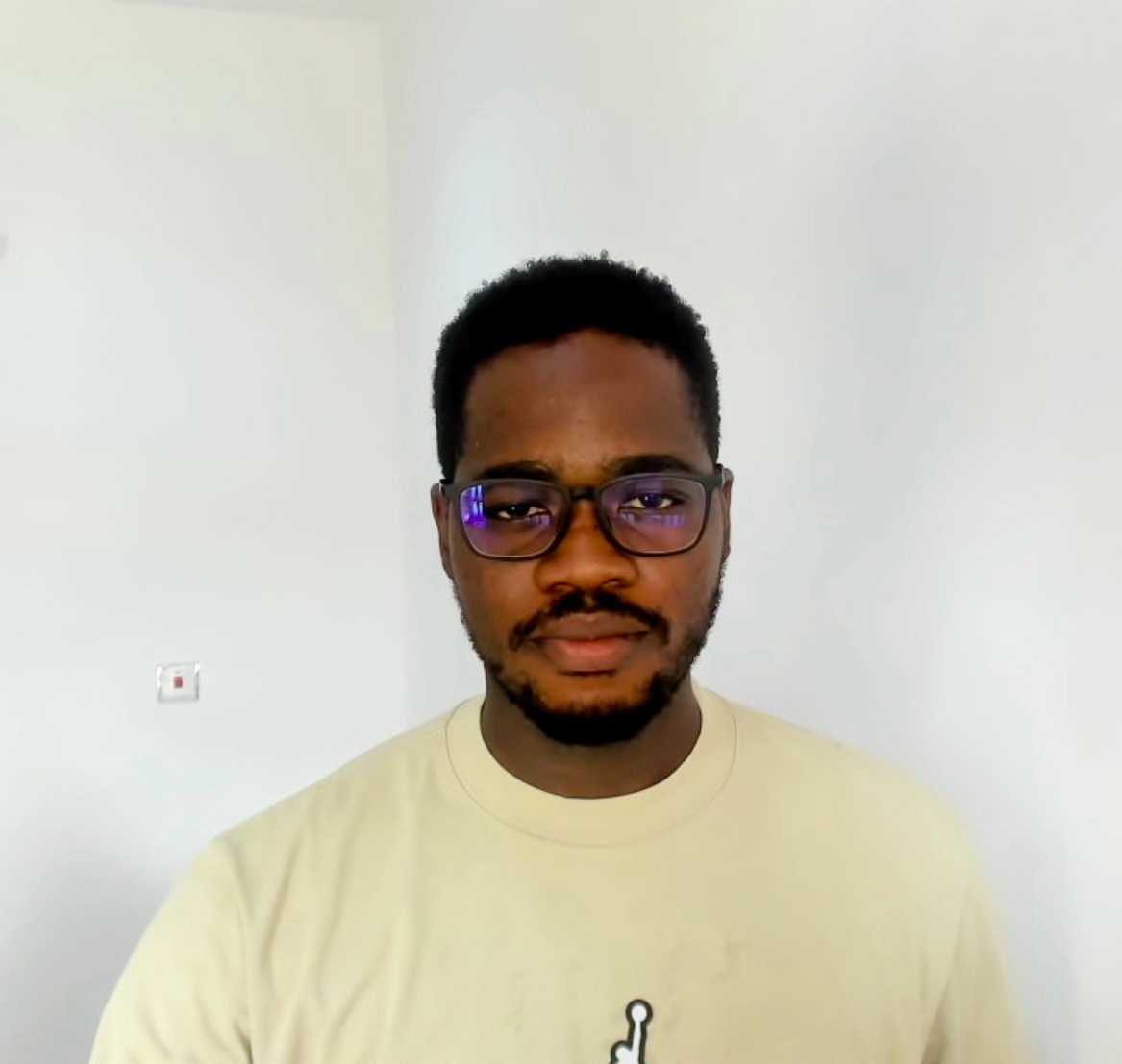
Joel Olawanle is a Software Engineer and Technical Writer with over three years of experience helping companies communicate their products effectively through technical articles.
View all posts by Joel Olawanle