Middleware is a critical concept in Express.js, a popular web application framework for Node.js. It enables the modular and extensible handling of HTTP requests and responses.
In the context of Express.js, middleware functions are key players in the request-response cycle, allowing developers to incorporate logic and operations at different stages of the process.
Express.js middleware consists of functions with access to the request object (req), the response object (res), and a special function called "next." These functions can modify the request and response objects, terminate the request-response cycle, or pass control to the next middleware in the stack.
The primary purpose of middleware is to enhance application functionality by incorporating custom logic, performing preprocessing tasks, and managing various aspects of the request-response flow.
Middleware in the Request-Response Cycle
Express middleware functions are executed sequentially in the order they are added to the application. Each middleware function can modify the request and response objects or terminate the cycle.
The next function is used to pass control to the next middleware in the stack. This mechanism allows developers to compartmentalise different aspects of the application logic and make it more maintainable.
Key Concepts: req, res, and next
req (Request Object): Represents the incoming HTTP request and contains information about the client's request, such as parameters, headers, and the request body.
res (Response Object): Represents the outgoing HTTP response and is used to send the response back to the client. Developers can modify this object to customise the response.
next (Next Function): A callback function provided by Express to pass control to the next middleware in the stack. Invoking next() is essential for the request-response cycle to proceed to the next middleware.
Why Do We Use Middleware in JS?
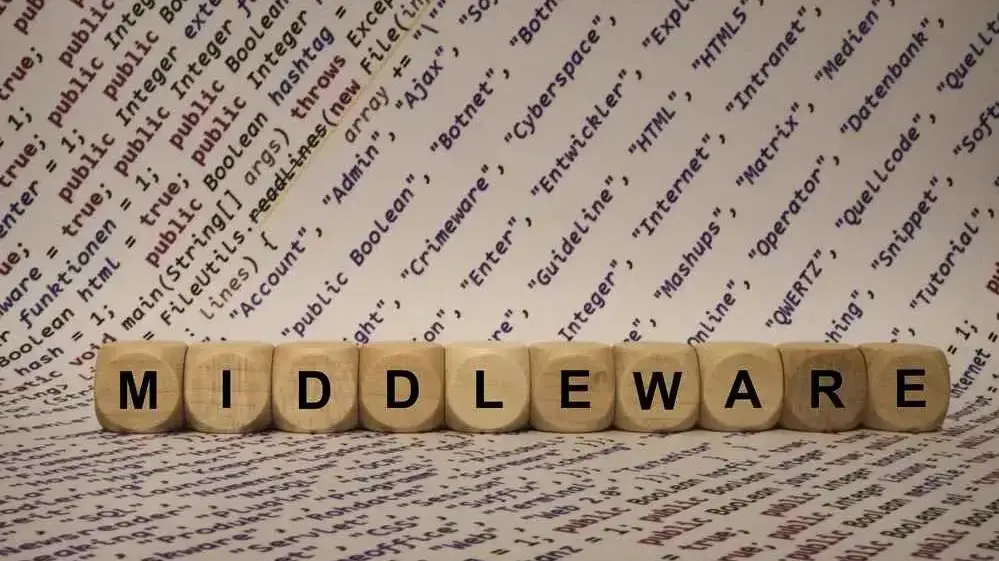
Middleware in JavaScript, especially in frameworks like Express.js, serves several essential purposes:
Modularity: Middleware allows developers to modularize and organize code by breaking down the application logic into smaller, manageable functions.
Request-Response Processing: Middleware functions can intercept and modify both incoming requests and outgoing responses, enabling tasks such as authentication, logging, and data validation.
Extensibility: Developers can easily extend and enhance the functionality of an application by adding or removing middleware functions.
Reusability: Middleware functions are reusable components that can be applied to different routes or across multiple applications, promoting code reuse.
Error Handling: Middleware can handle errors globally or for specific routes, improving the overall robustness of the application.
Types of Middleware
1. Application-Level Middleware
Application-level middleware is bound to the entire application and is executed for every incoming request. Examples include logging, parsing request bodies, and setting headers that are common to the entire application.
app.use(express.json()); // Application-level middleware for parsing JSON
2. Router-Level Middleware
Router-level middleware is specific to a particular route or group of routes. It is applied using app.use()
or within a specific router.
const router = express.Router();
router.use(myMiddleware); // Router-level middleware
3. Error-Handling Middleware
Error-handling middleware is used to catch errors that occur during the processing of a request. It has four parameters (err, req, res, next) and is defined with the app.use()
method.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
4. Third-Party Middleware
Third-party middleware are external packages that provide additional functionality to an Express application. These can be easily integrated using the app.use()
method.
const helmet = require('helmet');
app.use(helmet()); // Third-party middleware for securing HTTP headers
Implementing Middleware
In Express.js, middleware can be implemented using the app.use()
method. You can use built-in middleware, third-party middleware, or create your custom middleware functions.
1. Using Built-in Middleware
const express = require('express');
const app = express();
// Built-in middleware for parsing JSON
app.use(express.json());
// Built-in middleware for parsing URL-encoded data
app.use(express.urlencoded({ extended: true }));
2. Creating Custom Middleware
You can create custom middleware functions by using the app.use()
method. A middleware function takes three parameters: req (request), res (response), and next (a callback function to pass control to the next middleware).
// Custom middleware function
const myMiddleware = (req, res, next) => {
console.log('This is my middleware');
next(); // Pass control to the next middleware
};
// Use the custom middleware
app.use(myMiddleware);
3. Middleware Execution Order
Middleware functions are executed in the order they are added to the application. The order of middleware registration matters and each middleware function can modify the request and response objects or terminate the request-response cycle.
app.use((req, res, next) => {
console.log('Middleware 1');
next();
});
app.use((req, res, next) => {
console.log('Middleware 2');
next();
});
// Route handler
app.get('/', (req, res) => {
res.send('Hello, World!');
});
In this example, "Middleware 1" will be logged before "Middleware 2" because they are registered in that order.
4. Chaining Middleware
Middleware functions can be chained together using the app.use()
method. This is useful for organising and separating concerns in your application.
// Middleware 1
const middleware1 = (req, res, next) => {
console.log('Middleware 1');
next();
};
// Middleware 2
const middleware2 = (req, res, next) => {
console.log('Middleware 2');
next();
};
// Use middleware 1 and middleware 2 for a specific route
app.use('/route', middleware1, middleware2);
// Route handler
app.get('/route', (req, res) => {
res.send('Hello, Middleware!');
});
In this example, both middleware1 and middleware2 will be executed in order when a request is made to the '/route' endpoint.
Understanding the order of execution and how to chain middleware is crucial for building a well-structured and modular Express.js application.
Functions of Middleware in Express js
Middleware in Express.js plays a crucial role in enhancing security and developing various features by allowing developers to insert logic at different stages of the request-response cycle.
1. Request Processing
Middleware functions seamlessly handle incoming requests, validating data and performing necessary transformations before reaching the route handlers. This type of middleware focused on request processing, guards against common security vulnerabilities like SQL injection or cross-site scripting (XSS).
2. Response Modification
Middleware functions can modify the response before it is sent back to the client. This includes adding headers, compressing responses, or customising the payload. This type of middleware is useful for implementing features like response compression, adding custom headers for security, or transforming responses into a standardised format.
3. Authentication and Authorization
Middleware can handle authentication by verifying user credentials and ensuring that only authorised users have access to certain routes or resources, thereby protecting sensitive resources.
4. Logging and Monitoring
Middleware functions can log information about incoming requests, responses, and errors, providing valuable data for monitoring, debugging, and feature development. Crucial for understanding application behaviour, logging middleware is also beneficial for identifying and responding to security incidents.
5. Error Handling
Specialized error-handling middleware can catch and process errors, providing a consistent way to handle errors across the application. This proper error handling helps prevent information leakage to attackers by presenting generic error messages to users and logging detailed error information internally.
6. Routing and Routing Protection
Middleware defines routes and protects specific routes with authentication or authorization checks. Developers can create a structured and secure application by organizing routes and protecting them with middleware. One practical instance is protecting admin routes from unauthorized access.
7. Security Measures
Middleware can enforce security measures such as setting secure HTTP headers, preventing Cross-Site Request Forgery (CSRF) attacks, and securing against common web vulnerabilities. Implementing security middleware helps protect the application from a wide range of security threats, making it more resilient against attacks.
Real-World Use Cases
1. Improve Performance by Compressing Server Responses
- Install Compression Middleware
npm install compression
- Use Compression Middleware in Express App
const express = require('express');
const compression = require('compression');
const app = express();
// Use compression middleware
app.use(compression());
2. Manage User Sessions and Store Session Data
- Install and Configure express-session Middleware
npm install express-session
- Use express-session Middleware in Express App
const express = require('express');
const session = require('express-session');
const app = express();
// Configure express-session middleware
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: true,
}));
3. Validate Incoming Data from Requests
- Use Express Validator Middleware
npm install express-validator
- Use Express Validator in Express App
const express = require('express');
const { body, validationResult } = require('express-validator');
const app = express();
// Use express-validator middleware for request validation
app.post('/submit', [
body('username').isEmail(),
body('password').isLength({ min: 5 }),
], (req, res) => {
// Check for validation errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(422).json({ errors: errors.array() });
}
// Process the request
// ...
});
4. Integrate GraphQL into an Express.js Application
- Install Required Packages
npm install express express-graphql graphql
- Create a GraphQL Schema and Resolver
const { GraphQLSchema, GraphQLObjectType, GraphQLString } = require('graphql');
const schema = new GraphQLSchema({
query: new GraphQLObjectType({
name: 'RootQueryType',
fields: {
hello: {
type: GraphQLString,
resolve: () => 'Hello, GraphQL!',
},
},
}),
});
- Integrate GraphQL Middleware into Express App
const express = require('express');
const expressGraphQL = require('express-graphql');
const app = express();
// Use express-graphql middleware to handle GraphQL requests
app.use('/graphql', expressGraphQL({
schema: schema,
graphiql: true, // Enable GraphiQL for development
}));
These steps provide a general overview of how you can achieve each use case. Remember to adapt them to your specific project needs and requirements.
Testing Middleware
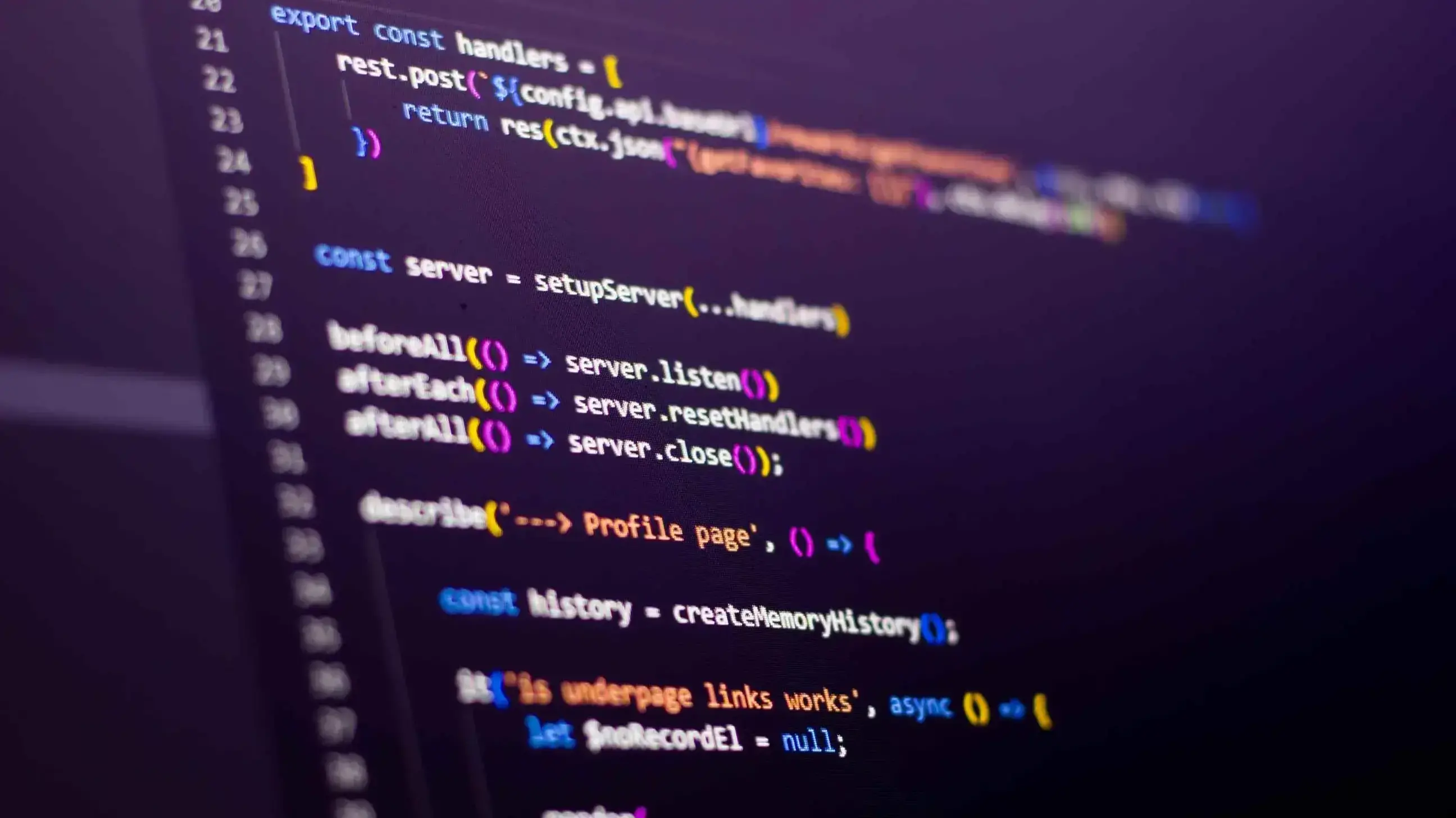
Testing middleware functions in Express.js involves both unit testing and integration testing.
1. Unit Testing Middleware Functions
Unit testing involves testing individual units of code in isolation to ensure they work as expected. For middleware functions, you want to test the behaviour of each middleware separately.
Example: Testing a Logging Middleware.
- Assuming you have a logging middleware function like this:
// loggerMiddleware.js
const loggerMiddleware = (req, res, next) => {
console.log('Request received at:', new Date());
next();
};
module.exports = loggerMiddleware;
- Using a testing library like Mocha and Chai, you can create a unit test for this middleware.
// loggerMiddleware.test.js
const chai = require('chai');
const chaiHttp = require('chai-http');
const app = require('../yourApp'); // Import your Express app
const loggerMiddleware = require('./loggerMiddleware');
chai.use(chaiHttp);
const expect = chai.expect;
describe('Logger Middleware', () => {
it('should log the request timestamp', (done) => {
const req = {};
const res = {};
const next = () => {
// Assert that the log message was printed
// You may need to adjust this based on your logging mechanism
expect(console.log.calledWith('Request received at:')).to.be.true;
done();
};
// Assuming you are using a mocking library like sinon to spy on console.log
sinon.spy(console, 'log');
// Call the middleware with mocked req, res, and next
loggerMiddleware(req, res, next);
});
});
Make sure to adjust the test based on your logging mechanism and testing library.
2. Integration Testing with Middleware
Integration testing involves testing the collaboration of different parts of the system. For middleware, this includes testing how middleware functions work together within the context of your Express application.
Example: Testing Authentication Middleware.
- Assuming you have an authentication middleware that checks if a user is logged in:
// authMiddleware.js
const authMiddleware = (req, res, next) => {
if (req.isAuthenticated()) {
return next();
}
res.status(401).send('Unauthorized');
};
module.exports = authMiddleware;
- You can create an integration test to ensure this middleware protects a route correctly.
// authMiddleware.test.js
const chai = require('chai');
const chaiHttp = require('chai-http');
const app = require('../yourApp'); // Import your Express app
const authMiddleware = require('./authMiddleware');
chai.use(chaiHttp);
const expect = chai.expect;
describe('Authentication Middleware', () => {
it('should allow access to protected route when user is authenticated', (done) => {
// Assuming you have a test user or a way to authenticate a user for testing
const authenticatedUser = { /* ... */ };
chai.request(app)
.get('/protected-route')
.set('Cookie', 'yourAuthCookie=' + authenticatedUser.cookie) // Adjust based on your authentication mechanism
.end((err, res) => {
// Assert that the response status is 200 (OK)
expect(res).to.have.status(200);
done();
});
});
it('should deny access to protected route when user is not authenticated', (done) => {
chai.request(app)
.get('/protected-route')
.end((err, res) => {
// Assert that the response status is 401 (Unauthorized)
expect(res).to.have.status(401);
done();
});
});
});
Ensure that your tests cover various scenarios and edge cases, and adapt them based on your specific middleware and application logic.
Performance Considerations
When working with middleware in Express.js, it is essential to consider the performance implications to ensure that the client's application remains fast and responsive.
Middleware Execution Order
The order in which middleware functions are executed matters. Middleware functions are executed in the order they are added to the application. Consider the placement of middleware to ensure that critical operations are performed efficiently.
Sequential Execution: Middleware functions are executed sequentially in the order they are added to the application. Ensure that critical middleware, such as authentication and security checks, is placed early in the stack.
Error-Handling Middleware: Error-handling middleware should be defined last. It will only be invoked if there is an error in the previous middleware or route handlers. Placing it early might result in it capturing errors that could be handled by subsequent middleware.
Efficient Middleware
Keep middleware functions efficient. Inefficient middleware can introduce bottlenecks and degrade application performance. Optimize code, avoid unnecessary computations, and be mindful of the resources consumed by middleware.
Minimize Blocking Operations: Avoid synchronous operations that could block the event loop. If a middleware involves I/O operations, use asynchronous patterns (callbacks, Promises, async/await) to prevent blocking.
Optimize Data Processing: Middleware often processes request and response data. Optimize data processing to only perform necessary operations. For example, if parsing a JSON body, only parse what is needed for the current request.
Use Caching: Consider caching results of expensive operations when appropriate. If a middleware performs a computation that doesn't change frequently, caching can reduce the workload on subsequent requests.
Asynchronous Middleware
Some middleware operations may involve asynchronous tasks, such as database queries or API calls. Ensure that asynchronous middleware functions effectively use callbacks, Promises, or the async/await pattern to avoid blocking the event loop and maintain responsiveness.
Use Promises or Async/Await: For asynchronous tasks, use Promises or the async/await syntax. This helps maintain the asynchronous nature of Node.js and prevents blocking the event loop.
Handle Errors Properly: Asynchronous middleware should handle errors appropriately. Use try/catch blocks or ensure that Promises are rejected with meaningful error information.
Middleware Dependencies
Be cautious about dependencies between middleware functions. If one middleware depends on the result of another, ensure that the order of execution is appropriate. Circular dependencies or unnecessary dependencies can impact performance.
Order of Execution: Be mindful of the order in which middleware functions are executed. Ensure that dependencies are met and critical operations happen before dependent middleware functions.
Minimize Dependencies: Avoid unnecessary dependencies between middleware. Each middleware should ideally be self-contained and not rely on the internal state of another middleware unless it is intentional and necessary.
Middleware Design
Design middleware functions with performance in mind. Minimize unnecessary data processing, and only execute operations that are essential for the current request-response cycle. Consider breaking down complex middleware into smaller, focused functions.
Single Responsibility: Follow the principle of having each middleware function with a single responsibility. This makes it easier to reason about, test, and maintain middleware.
Modularity: Break down complex middleware into smaller, modular functions. This allows for better code organization and makes reusing or replacing specific functionalities easier.
Middleware Updates
Regularly update third-party middleware to benefit from performance improvements and security updates. Check for updates in the middleware libraries you use, and keep your dependencies up to date to leverage optimizations made by the library maintainers.
Stay Informed: Keep track of updates and releases for your middleware libraries. Subscribe to newsletters or follow community channels to stay informed about improvements and security patches.
Scheduled Maintenance: Plan scheduled maintenance to review and update dependencies. Ensure that updates are thoroughly tested in a development environment before applying them to a production system.
It is crucial to balance the need for functionality to maintain optimal performance throughout the request-response cycle.
Conclusion
Middleware in Express.js serves as a powerful tool, seamlessly integrating into the request-response cycle to enhance web application functionality, security, and structure.
Developers leverage middleware to preprocess requests, handle authentication, enforce security, and organize routes, fostering modular and resilient applications.
Whether it is logging information, handling errors, or implementing security measures, middleware plays a pivotal role in shaping the behaviour of an Express.js application.
Testing at the unit and integration levels ensures the reliability and proper functioning of middleware functions.
As a result, Express.js middleware emerges as a fundamental component, empowering developers to build robust and scalable web applications while efficiently managing the request-response flow.
Frequently Asked Questions
How do you use multiple middleware in Express JS?
In Express.js, you can use multiple middleware functions by chaining them using the app.use() method.
const express = require('express'); const app = express();
// Middleware 1 app.use((req, res, next) => { console.log('Middleware 1'); next(); });
// Middleware 2 app.use((req, res, next) => { console.log('Middleware 2'); next(); });
// Route handler app.get('/', (req, res) => { res.send('Hello, World!'); });
app.listen(3000, () => { console.log('Server is running on port 3000'); });
Why Express JS is called middleware?
Express.js is named middleware due to its capability to incorporate functions (middleware) into the request-response processing pipeline. These middleware functions, equipped with access to the request object (req), the response object (res), and the next function, possess the ability to adjust the request and response and influence the flow of the request-response cycle.
Can I use middleware to serve static files in Express.js?
Yes, you can use the built-in express.static middleware to serve static files in Express.js. This middleware is designed to serve static assets, such as HTML, CSS, images, and JavaScript files.
const express = require('express'); const app = express();
// Serve static files from the 'public' directory app.use(express.static('public'));
app.listen(300
Can middleware be used for route protection in Express.js?
Absolutely. Middleware can be employed to protect specific routes by implementing authentication or authorization checks. This ensures that only authorized users have access to certain routes or resources, contributing to the application's overall security.
Can middleware be dynamically added or removed in Express.js?
Yes, middleware in Express.js can be dynamically added or removed. Developers can use the app.use method to add middleware globally or apply middleware to specific routes.
How does Express.js middleware contribute to the maintainability of code?
Express.js middleware enhances code maintainability by promoting a modular and organized structure. Developers can compartmentalize specific functionalities into middleware functions, making managing and updating individual components easier.
Can middleware be used to implement caching strategies in Express.js?
Yes, middleware in Express.js can be employed to implement caching strategies. Developers can create custom middleware to cache responses based on specific criteria, reducing the load on the server and improving overall application performance.
How does middleware support cross-cutting concerns in Express.js development?
Middleware in Express.js addresses cross-cutting concerns, such as logging, authentication, and error handling, by allowing developers to inject logic across various parts of the application. This avoids the need to duplicate code and ensures a consistent approach to handling common functionalities, simplifying development and maintenance.
Can middleware functions access external services or APIs in Express.js?
Yes, middleware functions in Express.js can access external services or APIs. This capability is valuable when additional data or functionality from external sources is required during the request-response cycle.
How does error-handling middleware contribute to application robustness?
Error-handling middleware significantly contributes to application robustness by providing a centralized mechanism to catch and process errors. This prevents unexpected failures from disrupting the entire application.
How does middleware contribute to Express.js being a flexible and unopinionated framework?
Middleware contributes to the flexibility of Express.js by allowing developers to shape the behaviour of the framework according to specific application requirements. Express.js, being unopinionated, provides the freedom for developers to choose and apply middleware as needed, adapting the framework to diverse use cases without imposing strict conventions.
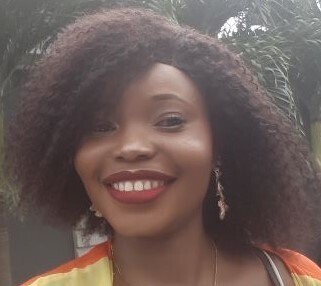
Yetunde Salami is a seasoned technical writer with expertise in the hosting industry. With 8 years of experience in the field, she has a deep understanding of complex technical concepts and the ability to communicate them clearly and concisely to a wide range of audiences. At Verpex Hosting, she is responsible for writing blog posts, knowledgebase articles, and other resources that help customers understand and use the company's products and services. When she is not writing, Yetunde is an avid reader of romance novels and enjoys fine dining.
View all posts by Yetunde Salami