PyTorch is an open-source machine learning library developed by Meta AI and now maintained under the Linux Foundation. It’s widely used for tasks like computer vision and natural language processing, thanks to its dynamic computation graphs, simplicity, and GPU acceleration.
Alongside TensorFlow, PyTorch is one of the most popular deep learning frameworks, empowering both researchers and developers.
This guide will introduce you to PyTorch’s key concepts and walk you through building your first machine-learning model, step by step.
Key Features of PyTorch
PyTorch stands out as a flexible and powerful machine learning framework, offering features designed to simplify deep learning tasks:
Tensors: PyTorch provides multi-dimensional arrays called tensors, similar to NumPy arrays, with the added advantage of seamless GPU acceleration. This makes it easy to handle numerical computations on both CPUs and GPUs.
Automatic differentiation (Autograd): The
autograd
module enables automatic computation of gradients, essential for optimizing models through backpropagation. This feature simplifies training neural networks and supports custom gradient calculations.Neural network building blocks: PyTorch’s
torch.nn
module offers pre-built components like layers, loss functions, and optimizers. These building blocks make it straightforward to design and train complex neural networks with minimal effort.Dynamic computation graphs: Unlike static frameworks, PyTorch builds computation graphs dynamically at runtime. This allows for intuitive debugging and flexible experimentation, making it ideal for research and prototype development.
Versatile applications: PyTorch integrates seamlessly with libraries for tasks such as natural language processing (NLP), computer vision, and reinforcement learning, making it a popular choice for diverse machine learning applications.
Getting Started with PyTorch
Getting started with PyTorch involves setting up your environment, installing the library, and optionally enabling GPU support. Follow these steps to get everything ready.
1. Installing PyTorch
Installing PyTorch is straightforward, and the framework supports both CPU and GPU configurations. Visit the official PyTorch website for an installation command tailored to your system.
If you’re using pip
, you can install the latest stable version of PyTorch with the following command:
pip install torch torchvision torchaudio
For GPU support, ensure that you have a compatible CUDA version installed on your system. Replace cu118
in the command below with the version of CUDA you’re using (e.g., cu117
or cu116
):
pip install torch torchvision torchaudio --index-url https://download.pytorch.org/whl/cu118
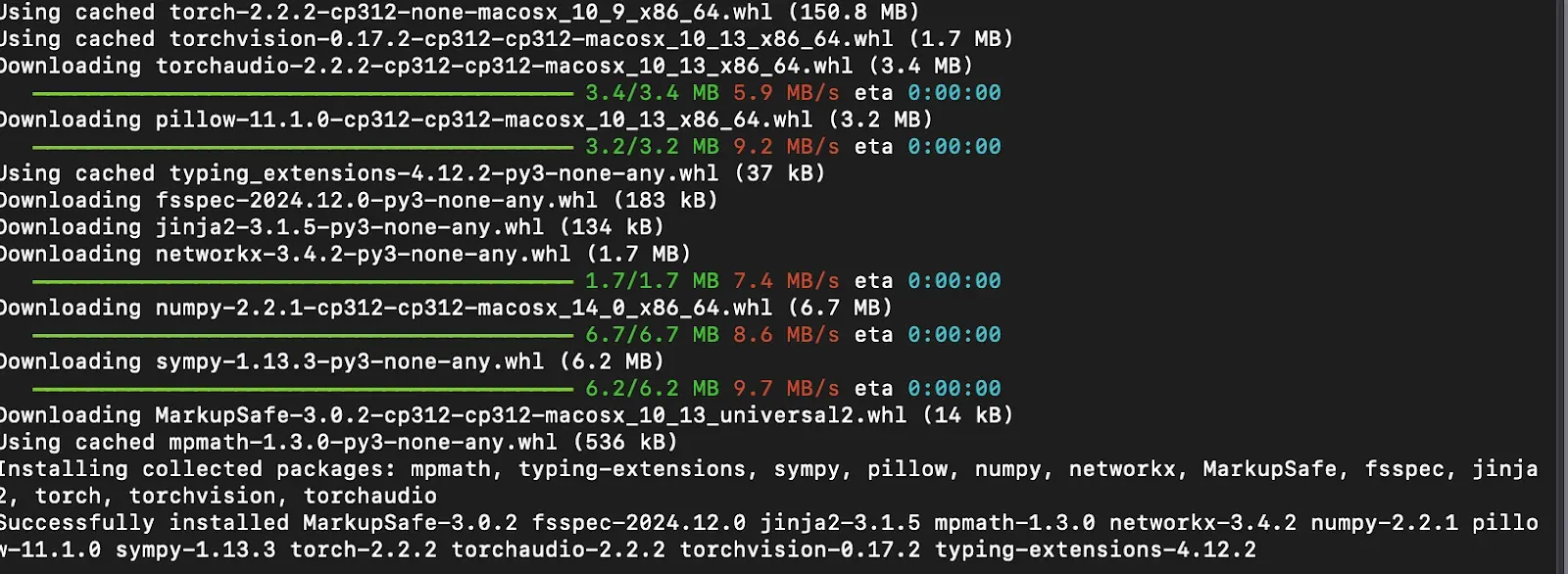
Alternatively, if you prefer managing dependencies with conda
, you can install PyTorch using this command:
conda install pytorch torchvision torchaudio pytorch-cuda=11.8 -c pytorch -c nvidia
Visit the official PyTorch website for more installation details, including instructions tailored to specific configurations.
2. Setting Up a Virtual Environment
To avoid dependency conflicts, it’s recommended to use a virtual environment. You can create one using Python’s built-in venv
module:
python -m venv pytorch_env source pytorch_env/bin/activate # On Linux/Mac pytorch_env\Scripts\activate # On Windows
If you’re working with Anaconda, use the following commands to set up an isolated environment:
conda create -n pytorch_env python=3.9 conda activate pytorch_env
Always activate your virtual environment before installing PyTorch or running your projects.
3. Verifying Installation
Once installed, you can verify that PyTorch is set up correctly by opening a Python shell and running the following:
import torch
print(torch.__version__) # Check PyTorch version
print(torch.cuda.is_available()) # Check if GPU is detected
If the GPU check returns True
, you’re ready to leverage GPU acceleration in your PyTorch projects. Otherwise, PyTorch will default to using your CPU.
PyTorch Basics
Understanding the foundational components of PyTorch is essential for building machine learning models. This section explores tensors, autograd, and efficient data handling using PyTorch.
1. Tensors: The Building Block of PyTorch
Tensors are multi-dimensional arrays, similar to NumPy arrays, but with the added advantage of GPU acceleration. They are used to encode data, model parameters, and operations efficiently.
Here are some common tensor operations:
import torch
# Creating tensors
tensor1 = torch.tensor([1, 2, 3])
print("Tensor from list:", tensor1)
tensor2 = torch.zeros(2, 3)
print("Tensor of zeros:", tensor2)
tensor3 = torch.rand(3, 2)
print("Random tensor:", tensor3)
# Basic operations
tensor1_broadcasted = tensor1.unsqueeze(0) # Adding a dimension
result_add = tensor1_broadcasted + tensor2
print("Addition result:", result_add)
result_mul = tensor2 * 5
print("Multiplication result:", result_mul)
result_matmul = torch.matmul(tensor2, tensor3)
print("Matrix multiplication result:", result_matmul)
This code demonstrates creating tensors, broadcasting dimensions, and performing common operations like addition, multiplication, and matrix multiplication.
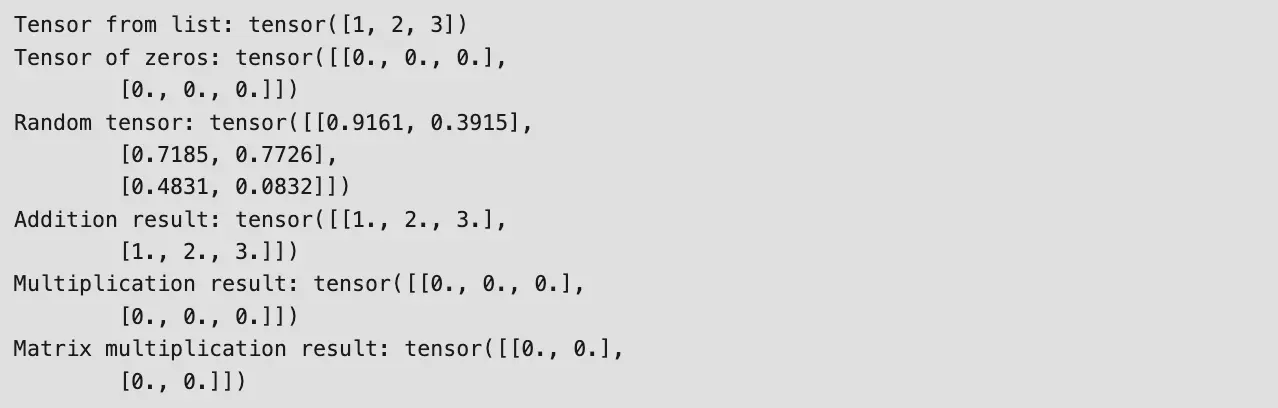
2. Autograd: Automatic Differentiation in PyTorch
PyTorch’s autograd module simplifies gradient computation, which is essential for optimizing machine learning models. Gradients represent the rate of change of a function with respect to its inputs, helping to minimize the loss during training.
Example:
# Defining tensors with gradient tracking
x = torch.tensor(2.0, requires_grad=True)
y = torch.tensor(3.0, requires_grad=True)
# Perform a computation
z = x**2 + y**3
print("Output tensor z:", z)
# Compute gradients
z.backward()
print("Gradient of x:", x.grad) # ∂z/∂x = 2x
print("Gradient of y:", y.grad) # ∂z/∂y = 3y^2
Output:
z=x2+y3=4+27=31z = x^2 + y^3 = 4 + 27 = 31z=x2+y3=4+27=31
∂z∂x=2x=4\frac{\partial z}{\partial x} = 2x = 4∂x∂z=2x=4
∂z∂y=3y2=27\frac{\partial z}{\partial y} = 3y^2 = 27∂y∂z=3y2=27
PyTorch automatically tracks operations for backpropagation through the grad_fn
property.
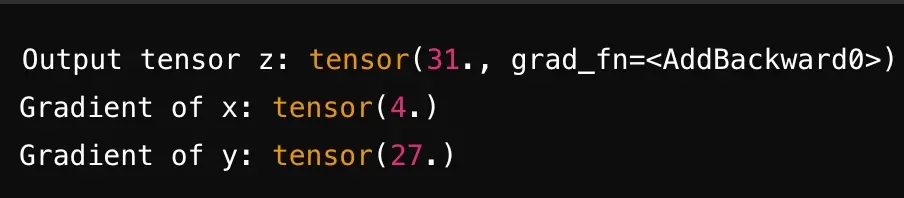
3. Working with Data in PyTorch
Efficient data handling is a crucial aspect of machine learning. PyTorch provides two key classes:
Dataset:
An interface for custom datasets that you can extend.DataLoader:
A utility to load and batch data efficiently, with support for shuffling and parallel processing.
Example:
from torch.utils.data import DataLoader, Dataset
# Define a custom dataset
class CustomDataset(Dataset):
def __init__(self, data, targets):
self.data = data
self.targets = targets
def __len__(self):
return len(self.data)
def __getitem__(self, idx):
return self.data[idx], self.targets[idx]
# Sample data and labels
data = torch.randn(100, 3, 32, 32) # Random 32x32 images with 3 channels
targets = torch.randint(0, 10, (100,)) # Random labels for 10 classes
# Create dataset and dataloader
custom_dataset = CustomDataset(data, targets)
data_loader = DataLoader(custom_dataset, batch_size=32, shuffle=True, num_workers=4)
# Iterate through batches
for batch_idx, (inputs, labels) in enumerate(data_loader):
print(f"Batch {batch_idx+1}: Inputs shape: {inputs.shape}, Targets shape: {labels.shape}")
The code above creates a new PyTorch dataset class to handle picture data and target labels. It employs a DataLoader
to efficiently load data in batches for training or processing. It generates a dataset of random images and labels, then iterates over each batch, outputting the input and target forms.
Below is the output of the above code:

The dataset contains 100 samples, and with a batch size of 32, it is divided into:
3 full batches: Each containing 32 samples.
1 final batch: Containing the remaining 4 samples.
The structure of the data is as follows:
Inputs: Shapes are
[batch_size, channels, height, width
], representing the 3-channel 32x32 image data. For example, a batch of 32 images would have a shape oftorch.Size([32, 3, 32, 32])
.Targets: Shapes correspond to the batch size. For instance, for a batch of 32 samples, the target labels have a shape of
torch.Size([32])
.
Since shuffling is enabled in the DataLoader
, the sequence of the batches changes in every epoch, but the overall structure remains consistent.
Summary
PyTorch is a flexible and powerful framework for building machine learning models. This guide introduced its key features, including tensors, automatic differentiation, and data handling, showing how they simplify tasks like image recognition and natural language processing.
With its intuitive design, PyTorch makes experimentation and development straightforward, giving you the tools to start creating your own machine-learning projects.
Frequently Asked Questions
Is Fedora VPS hosting suitable for machine learning?
Yes, Fedora VPS supports popular libraries like TensorFlow and PyTorch, making it a strong choice for lightweight machine learning projects.
Can small-scale dropshippers also benefit from AI and Machine Learning?
Yes, small-scale dropshippers can benefit from AI and Machine Learning by using scalable, cloud-based AI tools that offer cost-effective solutions for automating processes, analyzing data, and enhancing customer experiences.
What is the role of artificial intelligence and machine learning in ethical hacking?
AI and machine learning enhance ethical hacking by automating tasks, identifying patterns, and improving threat detection. Ethical hackers leverage these technologies to analyze large datasets and enhance security measures.
How does Machine Learning assist in inventory management for a dropshipping business?
Machine Learning assists in inventory management by analysing sales data, seasonal trends, and market changes to predict inventory needs, thereby helping maintain optimal stock levels and reducing the risks of overstocking or stockouts.
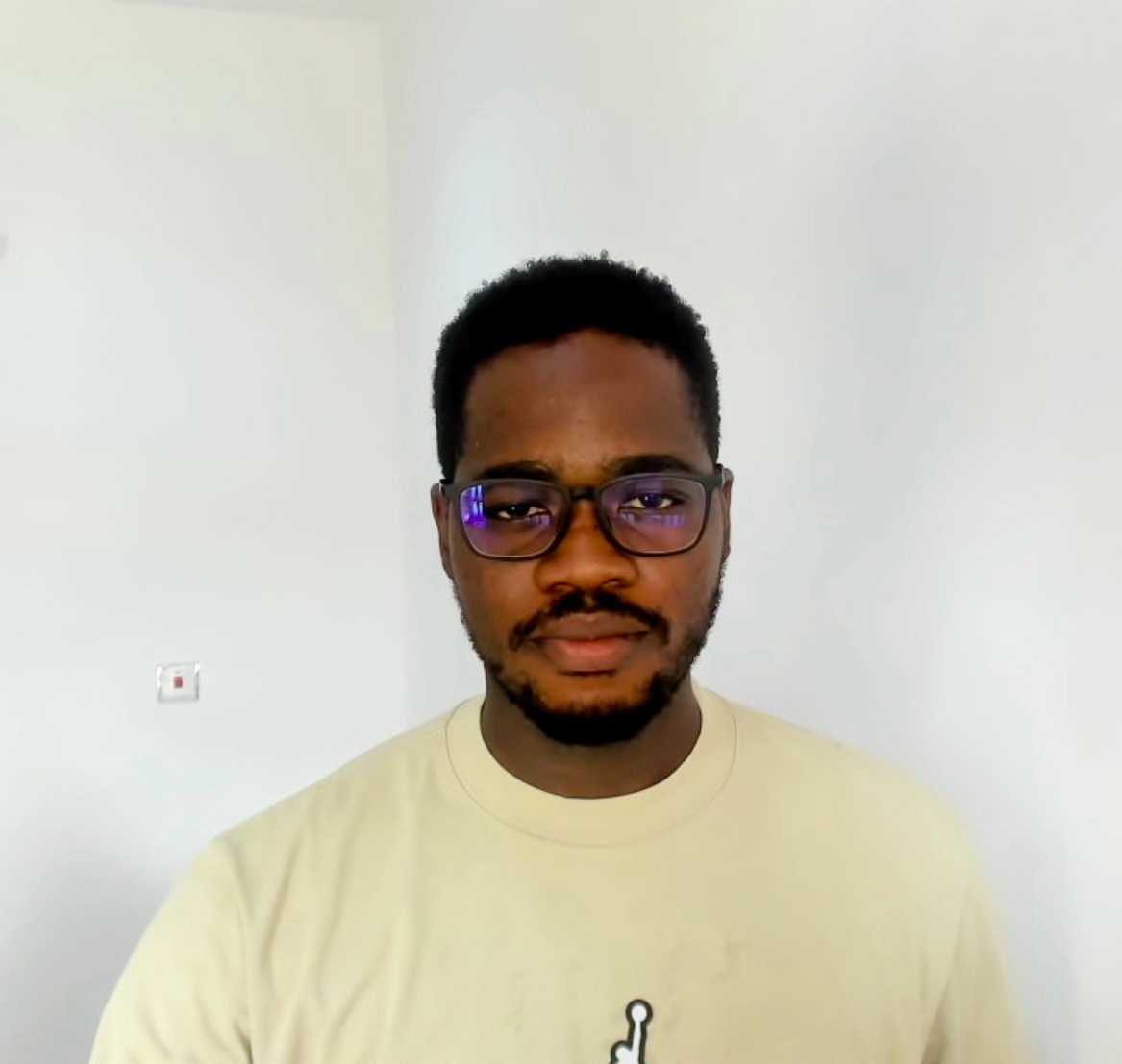
Joel Olawanle is a Software Engineer and Technical Writer with over three years of experience helping companies communicate their products effectively through technical articles.
View all posts by Joel Olawanle